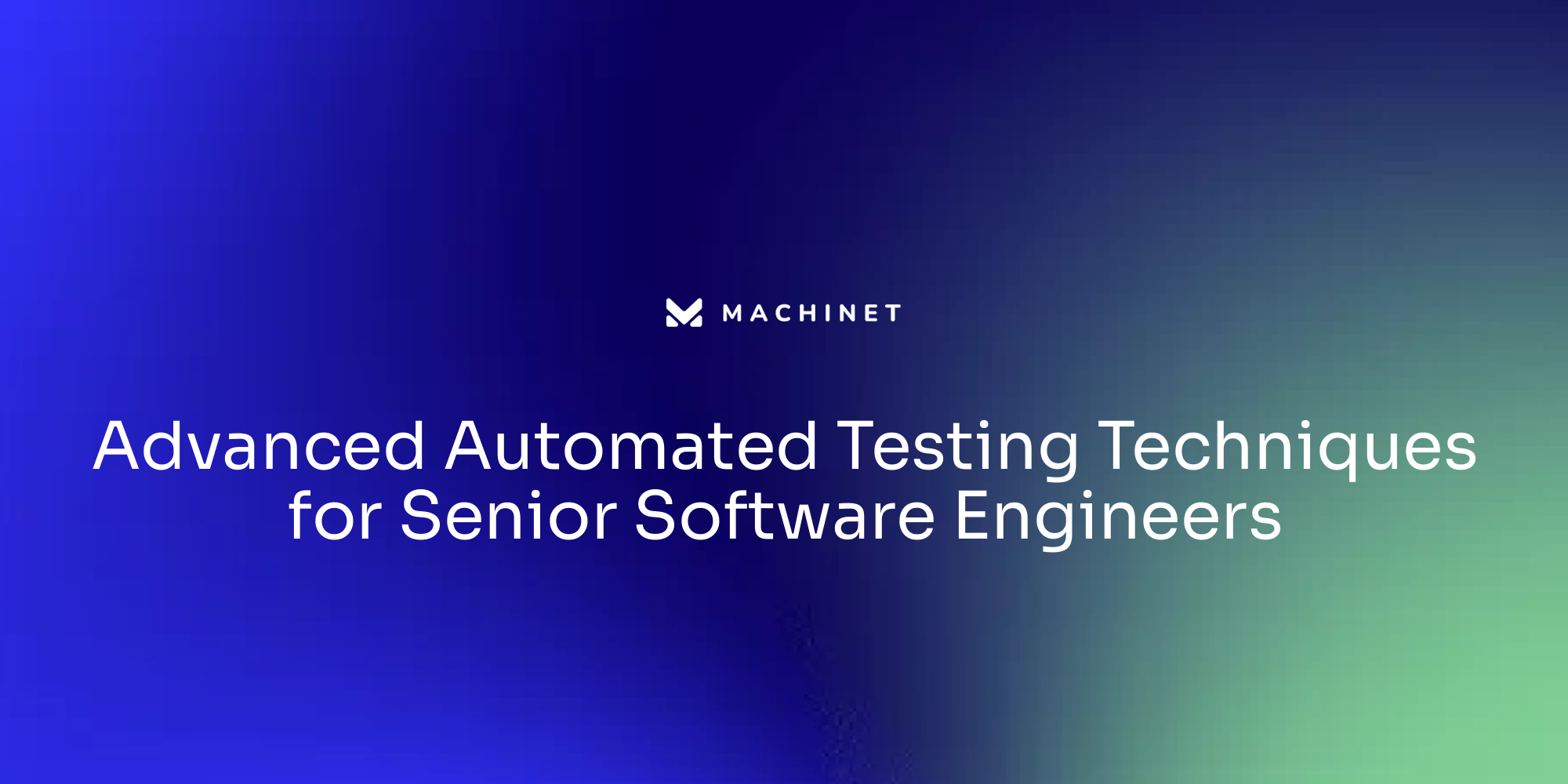
Table of contents
- Understanding the Importance of Automated Testing
- Exploring Different Types of Automated Tests
- The Role of Unit Tests in Software Development
- Best Practices for Test Automation
- Techniques for Testing Callback Methods in Java
- Strategies to Manage Technical Debt and Legacy Code
- Adapting to Changing Requirements with Robust Testing Frameworks
- Balancing Workload and Deadlines in Software Testing
Introduction
Testing is an essential aspect of software development, ensuring that the code functions as expected and meets the desired quality standards. However, managing the testing process can be challenging, especially when faced with changing requirements and project deadlines. In this article, we will explore strategies and best practices for managing technical debt and legacy code, adapting to changing requirements with robust testing frameworks, and balancing workload and deadlines in software testing.
First, we will delve into the importance of addressing technical debt and legacy code in software development. We will discuss strategies such as regular code refactoring, automated testing, and the role of senior engineers in managing technical debt. Additionally, we will explore real-world examples of companies effectively managing technical debt.
Next, we will examine how robust testing frameworks can help teams adapt to changing requirements. We will explore different types of automated tests, such as unit tests, functional tests, and regression tests, and how they contribute to maintaining high-quality software products. We will also discuss techniques for testing callback methods in Java and best practices for test automation.
Finally, we will discuss strategies for balancing workload and deadlines in software testing. We will explore the importance of test case prioritization based on criticality, complexity, and potential bug discovery. We will also discuss the role of automation in reducing the workload on testing teams and ensuring efficient testing processes.
By implementing these strategies and best practices, software development teams can effectively manage technical debt, adapt to changing requirements, and balance workload and deadlines in software testing. This ultimately leads to the delivery of high-quality software products that meet customer expectations
1. Understanding the Importance of Automated Testing
In the realm of software development, automated testing is a key player, serving as an essential tool to swiftly detect bugs and glitches. This ensures the delivery of a software product that meets high-quality standards. Techniques encompassing unit, integration, and system testing form a robust framework that aids in validating the functionality, security, and performance of software applications.
Unit testing is a technique that enables developers to verify individual units of source code, determining whether they are fit for use. This process isolates a section of code and tests it for correctness. As such, it helps to uncover and rectify bugs early in the development process. To optimize this process, it's recommended that developers write testable code by keeping the code modular and loosely coupled, which facilitates easier isolation and testing of individual units. Equally important, developers should create test cases that cover all possible scenarios and edge cases to ensure that the code functions correctly in different situations. For Java applications, a unit testing framework such as JUnit can automate these test cases, allowing for easy and frequent testing of the code during development.
Integration testing, another technique, focuses on the interfaces between components, interactions between different parts of the system, and potentially the entire system itself. This approach is designed to detect any issues with the relations between different software modules. It plays a crucial role in identifying any issues that arise when different components of the software interact with each other, ensuring that the integrated system functions as expected and that all components work harmoniously. Integration testing also uncovers any compatibility issues between different modules or systems, allowing developers to address them before the final release.
System testing, the third type of automated testing, validates the complete and fully integrated software product. It ensures that the entire system functions as expected and verifies whether the application meets the specified requirements. This type of testing typically includes testing the interactions between different modules, subsystems, and the overall system. It aims to verify that the software application functions as expected in real-world scenarios and meets the desired quality standards.
Automated testing plays a significant role in continuous integration and continuous delivery (CI/CD). It allows developers to integrate their changes back to the main branch as frequently as possible. By doing so, you can detect and locate errors quickly and easily. To integrate automated testing in CI/CD, a pipeline that automates the build, test, and deployment processes should be implemented. This pipeline should be triggered whenever changes are pushed to the version control system. Automated tests should be included in the pipeline, and a test framework that is compatible with the application or system should be selected. This framework should support automated execution of tests and provide necessary reporting capabilities.
Moreover, automated testing contributes to enhancing productivity in the software development lifecycle. It reduces the time and effort required for testing and allows for more focus on the design and implementation of software. To improve the efficiency of automated testing, it is important to prioritize test cases based on their impact and criticality, automate repetitive and time-consuming tasks, review and update test cases regularly, leverage parallel execution techniques, and implement proper test case management and reporting tools.
In conclusion, automated testing is a powerful tool for ensuring software quality, providing a robust framework for software validation, and its role in CI/CD accelerates the software development lifecycle
2. Exploring Different Types of Automated Tests
Automated testing has become an indispensable pillar in the realm of current software development, serving as a potent tool to ensure both efficiency and quality in the testing process. Diverse categories of automated tests exist, each with a distinctive function and focus.
Unit tests, the most granular type of automated testing, concentrate on individual code units. These tests are typically developed by the software engineers who write the code, thus integrating them into the development process. Unit testing, as a technique, enables the independent testing of system components to ensure correct functionality. By writing unit tests, developers can verify the behavior of each component in isolation, allowing early detection of bugs or issues, thereby bolstering the reliability of your code.
Functional tests simulate user actions to validate the system's behavior against expected outcomes. They play a pivotal role in ensuring the software functions as intended, thus offering a reliable user experience. The efficiency and effectiveness of the testing process are significantly enhanced by automation tools like Wetest, which offers automation for functional testing, performance testing, regression testing, and more.
Regression tests are engineered to confirm that previously identified defects have been addressed and that no new issues have been introduced by modifications. These tests are instrumental in maintaining software stability as it evolves.
Smoke testing serves as a quick litmus test to verify the core functionalities of the software. It is usually conducted as a preliminary test to ascertain the software's basic functionality before proceeding with more detailed testing.
Performance tests assess the software's behavior under various load conditions. They are crucial in ensuring that the software can handle the expected load and perform optimally under different usage conditions.
Parallel to these, we have integration and system tests. Integration tests focus on validating the interactions between different components or modules. They help identify any issues related to data exchange and communication between these components.
System tests evaluate the software in its entirety, assessing its adherence to specified requirements and its ability to operate in its intended environment. To evaluate whether the system meets the specified requirements and standards, it is necessary to analyze and compare the system's functionality, features, and performance against the desired requirements.
To conclude, while manual testing retains its place in ensuring software quality, the efficiency and effectiveness of automated testing make it a crucial component in modern software development. The key lies in understanding which tests to automate and selecting the most suitable test automation strategy based on the project's requirements and goals
3. The Role of Unit Tests in Software Development
Unit testing is a fundamental element of software development, particularly within the realm of Java. These tests offer immediate feedback to developers, enabling them to spot and address errors promptly. A key benefit of unit tests lies in their ability to facilitate refactoring, ensuring that modifications do not disrupt existing functionalities. This is because unit tests serve as a code safety net, making refactoring a routine process rather than an avoided one.
In the context of Java development, unit tests extend beyond mere debugging tools; they also offer valuable insights into API interactions and usability. They help assess the API's functionality and ease of use, offering early feedback and allowing for iteration. Hence, unit tests can also be deemed as an API design tool.
Moreover, unit tests function as a form of documentation, detailing the expected behavior of the system. This documentation aspect is particularly beneficial as unit tests ensure that the tests reflect the most recent state of the API, providing examples of its usage. This positions unit tests as an effective documentation mechanism for the code.
Additionally, unit tests play a substantial role in maintaining the software's longevity and maintainability. They enforce a modular design and endorse good coding practices, which in turn helps to prevent the codebase from becoming cluttered and difficult to comprehend.
A real-world example of the importance of unit tests comes from Roland Weisleder, a freelance Java developer, who noticed that changes in the Java version can affect date parsing and currency formatting. His experiences underscore the necessity of updating unit tests when upgrading the Java version to prevent unforeseen errors.
In summary, unit tests are an integral tool in Java development that enables developers to deploy safe code at a faster pace. They ensure that the code functions as expected, capture bugs early, and allow developers to experiment with different scenarios. Furthermore, they provide a safety net for code changes, serve as an API design tool, and function as a documentation mechanism, thereby making the development process quicker and more efficient."
In the context of software development, unit tests play an essential role in ensuring software reliability and quality by testing individual units or components of the code. They facilitate the early identification of bugs and errors in the development process, allowing developers to rectify them before they become more complex and costly to resolve. Moreover, unit tests serve as documentation and provide examples of how the code should be used, simplifying the process for other developers to understand and work with the software.
Unit tests offer numerous benefits in software development. They facilitate the early identification and rectification of bugs, reducing the cost and effort required for debugging and maintenance. Unit tests enhance code quality and readability by enforcing modular and well-structured code. Additionally, unit tests function as code documentation, simplifying the process for other developers to comprehend and work with the codebase. They also facilitate code refactoring and ensure that modifications to the codebase do not introduce new bugs. Overall, unit tests improve software development projects' reliability, maintainability, and efficiency.
Unit tests aid developers in software development by providing a means to verify that individual units of code, such as functions or methods, behave as expected. By writing and executing unit tests, developers can catch bugs and errors early in the development process, ensuring that the code functions correctly before integrating it with other components. This helps reduce the overall number of bugs in the software and enhances the overall quality of the code. Additionally, unit tests serve as a form of documentation, providing examples of how the code should be used and what outputs can be expected in different scenarios. Overall, unit tests aid developers in building reliable, maintainable, and robust software applications.
To write effective unit tests, it is important to adhere to certain best practices. These include writing tests that are focused and cover a specific piece of functionality or behavior, ensuring that tests are independent and do not rely on the state of other tests, using meaningful and descriptive test names that clearly indicate what is being tested, incorporating test-driven development (TDD) principles where tests are written before the implementation code, including both positive and negative test cases to ensure that the code handles expected and unexpected scenarios, mocking or stubbing external dependencies to isolate the code being tested, regularly re-running tests to catch any regressions or breaking changes, and writing tests that are easy to understand and maintain, with clear assertions and minimal test setup. By adhering to these guidelines, developers can write effective unit tests that instill confidence in the correctness and robustness of their code.
Unit tests in software development are used to verify the functionality and correctness of individual units or components of the software.
They are typically written by developers and are automated to run frequently during the development process. Unit tests are designed to test small, isolated pieces of code and can help identify bugs and errors early on. Examples of unit tests in software development may include testing individual functions or methods within a class, testing different scenarios and edge cases, checking for expected outputs given specific inputs, and ensuring proper error handling and exception handling. Unit tests also serve as documentation for how a particular unit or component should behave.
In software development, unit tests can serve as a form of documentation. By writing thorough and descriptive unit tests, developers can provide clear examples of how their code is intended to be used and what expected outcomes should be. This documentation through unit tests can help other developers understand the functionality of the code and how to interact with it correctly. Additionally, unit tests can act as a safety net, alerting developers to potential issues or regressions if any changes are made to the codebase. Overall, documentation through unit tests is a valuable practice in software development that promotes code understanding and maintainability.
Modular design and unit tests are important aspects of software development. A modular design allows for the separation of different components or modules of a software system, making it easier to develop, test, and maintain. Unit tests, on the other hand, are small tests that validate the functionality of individual units or components of the software. These tests help identify bugs and ensure that the units are working as expected. By incorporating modular design and unit tests into the software development process, developers can improve the overall quality and reliability of their software
4. Best Practices for Test Automation
Test automation, correctly implemented, significantly boosts the efficiency and effectiveness of software testing processes. A major component of this is maintaining an immaculate test environment, ensuring the reliability of test outcomes. Tests should be designed independently, allowing for any sequence of execution without impacting their results.
Every test case should be laser-focused, clear, and concise, tackling a single functionality to prevent confusion and ensure precision. Regular review and updates of these test cases are essential to guarantee their ongoing relevance and effectiveness in the dynamic world of software development.
A blend of manual and automated testing optimizes results, as not all tests are ideally automated. For example, regression tests, including smoke tests, sanity tests, and test cases uncovering defects from previous testing cycles, are well-suited for automation. Automating end-to-end testing, which ensures the application functions correctly across a variety of user tasks, can accelerate release times.
Collective ownership of automated tests is a best practice that promotes transparency and knowledge sharing, while eliminating potential work disruptions due to dependency on a single team member. Detailed planning, including test selection, addressing the root causes of failures, and retrospective analysis, is a vital component of successful test automation.
Choosing the right automation tools is a critical factor that can substantially influence the quality of the automation process. Monitoring automation progress through metrics can assist in identifying areas for improvement and adjusting testing strategies accordingly.
It's advisable to find a balance between testing on emulators, simulators, and actual devices to maximize product quality and cost-effectiveness. Starting tests early in the software development process aids in identifying critical bugs and enhancing usability. It's also crucial to keep all tests updated and remove unstable tests from regression packages to maintain high test coverage and product quality.
Using linting rules for tests can help illuminate errors and ensure consistency in test code. Code repetition can be avoided by using before/after each blocks and utility functions to encapsulate shared logic. Grouping related tests in describe blocks can help organize test files and encapsulate set-up/tear-down logic. Each unit test should only have one reason to fail, adhering to the single responsibility principle. Tests should be kept independent to avoid dependencies and ensure reliable and predictable results. Testing a variety of input parameters can cover all possible code paths and verify edge cases.
Leveraging automated testing tools and frameworks can significantly streamline the testing process and improve test coverage. By adhering to these best practices, software engineers can maximize the benefits of test automation, leading to more efficient and effective testing processes, and ultimately, higher quality software products
5. Techniques for Testing Callback Methods in Java
The task of testing callback methods in Java can be daunting due to their inherent asynchronous nature. However, several strategies have been developed to effectively test these methods.
One such strategy is the use of a CountDownLatch, a synchronization aid that allows one thread to pause until other threads complete their tasks. This is especially useful in scenarios where one operation is dependent on the completion of others. To implement this, a CountdownLatch object is created with an initial count of 1. The callback method then calls the countDown() method on the CountdownLatch object to decrement the count. In the test method, an instance of the class containing the callback method is created. Before invoking the method that triggers the callback, the await() method on the CountdownLatch object is called to block the test thread. After invoking the method, the callback method is executed and the count is decremented. The await() method is called again on the CountdownLatch object to block the test thread until the count reaches zero. The expected result is then asserted in your test method. This effectively synchronizes the test thread with the callback method, ensuring the callback has been executed before proceeding with the assertions in the test method.
Future objects, which represent the outcome of an asynchronous computation, can also be utilized in testing. By using a Future object, the result of a computation performed asynchronously can be retrieved, allowing the callback method to be tested by verifying the expected result returned by the Future object.
Another effective strategy involves the use of mocking frameworks such as Mockito. Mockito allows for the simulation of callback method behavior and facilitates the verification of interactions with other components. A spy is used instead of a mock and the spy is annotated with @Spy. The test method, named testsuccessfullogin, performs a login with arbitrary credentials and then verifies that the saveinsession method was called. This verification is done using the Mockito verify method, which takes the spy as a parameter and checks that the specified method was called. The saveinsession method is given a package-level modifier to allow the testing of non-public methods. Furthermore, the verify method can validate if the method was called with specific parameters, such as any customer object.
In addition, the asynchronous nature of callback methods in Java can be tested using techniques such as CompletableFuture, CountDownLatch, or Awaitility. These techniques allow for the waiting of the callback method to complete before asserting the expected result, ensuring the callback method is executed asynchronously and its behavior is tested accordingly.
Overall, these techniques provide a comprehensive approach to testing callback methods in Java, ensuring the stability and reliability of the code
6. Strategies to Manage Technical Debt and Legacy Code
Technical debt and legacy code are inherent in the realm of software development. Technical debt refers to the complexities and challenges that arise due to the use of outdated technology or suboptimal coding practices. Despite their profitability, legacy applications often become burdensome as they age, necessitating a transition to more contemporary technologies.
Addressing these challenges requires a strategic approach. One such strategy includes setting aside regular time to tackle pressing issues. This could involve organizing dedicated events like hackathons or "quality weeks" to focus solely on these issues. Striking a balance between minimizing the creation of new technical debt and maintaining the existing status quo is essential to manage existing debt effectively.
Refactoring is a key component of this strategy. Instead of resorting to temporary fixes or surface-level solutions, refactoring and replacing outdated technology should be prioritized. This not only enhances the code's structure and readability but also bolsters the system's performance and maintainability.
Automated testing is an indispensable tool in this process. By preserving a comprehensive suite of tests, it ensures that refactoring or replacing legacy code doesn't introduce new bugs, thereby maintaining the system's functionality.
The task of managing technical debt is not for novices. It necessitates the expertise of senior engineers who possess a thorough understanding of the system. These individuals are entrusted with the continual assessment of the current status versus the risk of migrating to newer systems. They should not hesitate to create temporary code that may later be discarded, as this could be a beneficial step in the transition process.
AppsFlyer, an engineering company that prioritizes code craftsmanship and engineering excellence, provides a valuable example of effective technical debt management. The company has successfully navigated the challenges of managing technical debt, even taking risks such as changing core data stores and rewriting mission-critical services. Their experience highlights the necessity of continuous effort in maintaining minimal technical debt and fostering a culture that values engineering excellence.
The importance of team culture, engagement, and motivation in managing legacy code cannot be overstated. Hiring and onboarding processes should prioritize diversity and inclusion, and distributed teams should be managed with effective communication and collaboration.
Lastly, it is vital to alter the perception of legacy code. Often seen as a career dead-end, working with legacy code can provide valuable learning opportunities and a chance to enhance code quality. By fostering a positive team culture around legacy code and investing in improving infrastructure and onboarding processes, what is often viewed as a challenge can be transformed into an opportunity for growth and improvement
7. Adapting to Changing Requirements with Robust Testing Frameworks
In the continuously evolving world of software development, the ability to adapt to changing requirements is a crucial aspect. Agile teams are well-known for their resilience and adaptability, as they routinely integrate these modifications into their product and processes. The key to maintaining high-quality output amidst these changes lies in the effective utilization of robust testing frameworks.
These frameworks provide a structured, systematic, and planned approach to testing, enabling teams to revise test cases promptly in response to evolving requirements[^2^]. Ranging from unit testing to functional testing, these frameworks support a variety of tests, thus enabling teams to validate new functionalities and assess the impact of changes on existing functionalities[^5^].
The importance of these frameworks extends beyond structured testing and validation. They significantly contribute to test automation, thereby reducing the time and effort required for testing, and facilitating a more efficient process[^7^][^8^]. For instance, frameworks like JUnit, TestNG, and Mockito offer features like test annotations, assertions, test runners, and mocking capabilities, which can help write effective and reliable test cases[^3^].
In the case of a significant production outage, conducting retrospectives, also known as postmortems, is suggested. Along with brainstorming sessions, these can be instrumental in understanding and prioritizing important challenges. This understanding can then drive changes in the testing process to prevent similar outages in the future.
Visual collaboration tools, such as root cause analysis tools and fishbone diagrams, can further enhance the exploration and addressing of issues. These tools can help identify the underlying causes of problems and develop effective solutions.
Teams are encouraged to conduct 'small frugal experiments' to make progress towards a goal. The outcomes and learnings from these experiments can then be applied to adapt the product and processes.
Teams can also formulate and test hypotheses to address specific challenges or achieve goals. For instance, instrumenting the code to capture events leading to 500 errors can expedite the diagnosing and fixing of these errors in production. Similarly, collaborating with designers in usability testing new feature releases can lead to customers trying new features right away.
These practices, combined with robust testing frameworks, can aid software development teams in managing changing requirements effectively.
However, the key to success lies in continual improvement. Teams should use concrete measurements to show progress towards a goal and continually refine their processes to ensure the successful delivery of high-quality software products. As aptly put, "Continual improvement is an important way to measure progress and success in agile testing
8. Balancing Workload and Deadlines in Software Testing
The need to balance software testing demands with project deadlines forms a crucial part of the software development lifecycle. A key tactic in managing this balance is test case prioritization, which should be anchored on factors such as criticality, complexity, and the potential to uncover bugs.
Prioritizing test cases based on importance and complexity is a vital part of test planning. Factors like the criticality of the functionality being tested, the impact on end-users, and the complexity of the test case itself can be considered. This ensures that the most critical scenarios are tested first, leading to early detection of issues and better allocation of testing resources.
Test impact analysis is a method that prioritizes tests according to their code coverage. This systematic approach ensures that the most critical parts of the software are thoroughly tested. It also helps identify tests more likely to uncover bugs, thus warranting a higher priority.
Another important factor in test case prioritization is the use of metrics related to testing and debugging. Metrics such as the bug lifecycle and test records can provide valuable insights into the effectiveness of various tests, aiding in the prioritization process. For instance, tests that have recently failed or have a high bug finding score should be given higher priority, as they are more likely to uncover new issues.
In some cases, dynamic test case prioritization may be employed. This technique involves reordering pending tests during a test campaign to maximize efficiency. While advanced methods such as rule mining and artificial intelligence can be used for dynamic test case prioritization, simpler approaches can also yield effective results. Indeed, prioritizing tests with shorter execution times can significantly improve efficiency and help address the common problem of tests taking too long.
Automation plays a critical role in balancing workload and deadlines in software testing. Automating repetitive and time-consuming tests can significantly reduce the workload on testing teams, freeing up resources for more complex and critical tests. Test automation tools and frameworks can be used to automate tests, reducing the time and effort required for testing and allowing the team to focus on more critical tasks.
Furthermore, it's crucial to ensure that there is adequate time allocated for testing in the project schedule. A testing strategy should be regularly reviewed and adjusted as necessary to ensure alignment with project deadlines and objectives.
Balancing workload and deadlines in software testing is a complex task requiring careful planning and strategic decision-making. However, with the right approach to test case prioritization, automation, and time management, it is possible to ensure that testing efforts are both effective and efficient
Conclusion
In conclusion, this article has explored various strategies and best practices for managing technical debt and legacy code, adapting to changing requirements with robust testing frameworks, and balancing workload and deadlines in software testing. The importance of addressing technical debt and legacy code in software development was highlighted, along with strategies such as regular code refactoring and automated testing. The article also discussed the role of robust testing frameworks in adapting to changing requirements and maintaining high-quality software products. Additionally, strategies for balancing workload and deadlines were examined, emphasizing the importance of test case prioritization and automation.
The ideas discussed in this article have significant implications for software development teams. By effectively managing technical debt and legacy code, teams can improve the maintainability and reliability of their software products. Adapting to changing requirements with robust testing frameworks allows teams to stay agile and deliver high-quality software that meets customer expectations. Balancing workload and deadlines through effective test case prioritization and automation helps optimize productivity and ensure efficient testing processes. By implementing these strategies and best practices, software development teams can navigate the challenges of software development more effectively, leading to the delivery of high-quality software products.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.