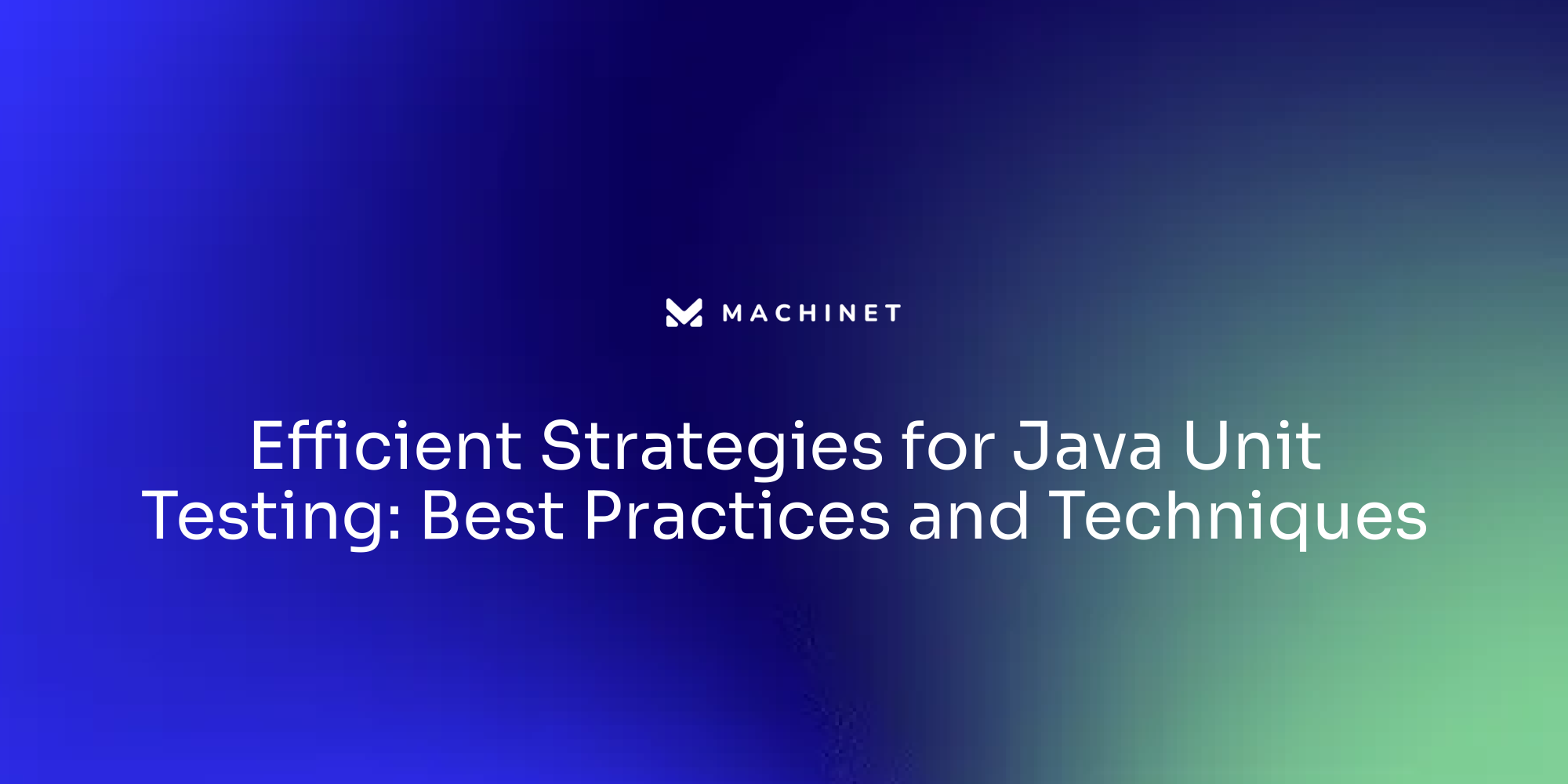
Table of Contents
- Understanding the Basics of Unit Testing in Java
- Writing Testable Code: Key Principles and Techniques
- Setting Up Your Java Unit Tests: An Overview
- Running a Unit Test in Java: A Step-by-Step Guide
- Leveraging JUnit 5 for Effective Java Unit Testing
- Dealing with Changing Requirements: Implementing Robust and Flexible Testing Frameworks
- Balancing Workload and Deadlines in Unit Testing Efforts
- Refactoring and Improving Existing Test Suites: Strategies and Best Practices
Introduction
Unit testing is a critical aspect of software development, ensuring the reliability and robustness of code. In the realm of Java, unit testing is often empowered by frameworks like JUnit and Mockito, which streamline the testing process and enable developers to mimic interactions with external components. The core of unit testing in Java lies in thorough examination of individual code components, such as methods and classes, and comparing expected outcomes with actual results. By mastering the art of unit testing in Java, software engineers can enhance the quality of their code and detect bugs early in the development process.
In this article, we will delve into the basics of unit testing in Java, exploring the process of setting up tests using frameworks like JUnit and Mockito. We will discuss the significance of assertions in determining the success or failure of tests and how to construct meaningful test cases. We will also examine best practices for organizing and structuring unit tests, as well as strategies for handling complex scenarios through method stubbing and mocking. By understanding these fundamentals, developers can optimize their Java unit testing efforts and ensure the reliability and functionality of their code
1. Understanding the Basics of Unit Testing in Java
Unit testing is a cornerstone of software development, acting as a safeguard to detect potential issues and preserve the robustness of your code. The core of unit testing is the thorough examination of individual code components including methods, classes, and components, ensuring their readiness for deployment.
In the Java universe, unit testing is often empowered by libraries such as JUnit and Mockito. JUnit provides a structure for crafting and executing tests, while Mockito assists in mocking external dependencies and stubbing methods.
Enhance your unit testing with JUnit and Mockito in combination with Machinet for code generation.
These tools enable developers to streamline the testing process by mimicking interactions with external components like database connections or network requests.
Breaking down the process of unit testing in Java, we initiate with setting up the necessary parameters for the test. This leads to the creation of mock objects using Mockito and the stubbing of methods to replicate their behavior. The final step encompasses invoking the method under test with the initialized parameters.
The pivot of unit testing is assertions, which are utilized to determine the success or failure of a test. This is accomplished by contrasting the expected return value of a method with the actual return value. This comparison shapes the foundation of the test cases, which are composed to evaluate the output of a method against its expected outcome.
Unit tests are typically constructed in a separate test class and are annotated with the @Test annotation. Post writing the tests, they can be executed by selecting "Run Test" from the context menu. The result of the test is subsequently exhibited in the test results window, indicating the pass or fail status of the tests.
Unit testing is not confined to simple functions. It can also be implemented in intricate scenarios, such as testing a function that assigns special permissions based on a user's role or a function that sorts an array.
Experience the power of Machinet for complex unit testing scenarios and code generation.
In these circumstances, method stubbing and mocking can be particularly handy.
For example, when testing functions that incorporate database queries, mock objects and stub methods can be created to simulate the behavior of the database. This enables the method to return dummy data, thereby eliminating the need for actual database connections.
In essence, unit testing is a potent tool for enhancing the quality of your code. By detecting bugs and issues early in the development process, it ensures that your code complies with the quality standards established by your organization. Hence, mastering the art of unit testing in Java is an indispensable skill for any software engineer.
To optimize Java unit testing, it's crucial to consider factors such as code organization, test coverage, and test design. Proper code organization involves structuring the test code in a way that promotes understanding and maintenance. It's advisable to group tests based on the functionality they're testing and use descriptive names for test methods.
High test coverage is another essential practice, implying that unit tests should strive to cover as much of the code as possible. All potential branches and edge cases should be tested to ensure that the code operates correctly under various scenarios.

Test design is another critical component of effective unit testing. Tests should be designed to be independent of each other, meaning that the outcome of one test does not influence the outcome of another. This promotes easier debugging and maintenance of the tests.
It's beneficial to use mocking frameworks, such as Mockito, to isolate the code under test from its dependencies. This aids in creating more focused and reliable tests.
Lastly, automating the execution of unit tests using build tools like Maven or Gradle is recommended. This ensures that the tests are run regularly and consistently throughout the development process.
By following these best practices, developers can create robust and maintainable unit tests for their Java code. Awareness of common mistakes can also lead to more effective and reliable unit tests, ensuring that their unit tests are accurate and provide valuable insights into the performance and functionality of their code
2. Writing Testable Code: Key Principles and Techniques
Crafting testable code is a craft that mixes practice with understanding core principles. One of these principles is making sure each code unit has a single responsibility. This simplification makes testing and debugging easier. Furthermore, designing loosely coupled code units is vital as it promotes independence, ensuring changes in one unit don't impact others.
Loosely coupled code significantly enhances testability by reducing dependencies and isolating code units. When code is loosely coupled, each component or module can function independently without heavily relying on other components. This independence makes it easier to test individual code units in isolation, as they're not tightly bound to other system parts. Reducing dependencies means that changes or modifications to one component have minimal impact on other components, simplifying test case creation and maintenance. Improved testability also comes from the ease of creating test cases that cover specific functionalities in the codebase without having to consider the entire system's intricacies.
The code should also be deterministic, consistently producing the same output given identical inputs. Deterministic code is crucial in testing because it allows for consistent and repeatable test results. By ensuring that the code behaves predictably, deterministic code makes it easier to identify and fix bugs during the testing process. Additionally, deterministic code enables effective test case management and promotes reliable and accurate test coverage.
Writing testable code also involves implementing design patterns conducive to testing, such as Dependency Injection and Mocking. By following certain design patterns, developers can create code that is easier to test and maintain. Some common design patterns for writing testable code include the Dependency Injection pattern, the Strategy pattern, and the Mocking pattern. These patterns help decouple dependencies, allow for easy substitution of components, and enable the creation of test doubles for unit testing. By applying these design patterns, developers can ensure that their code is more modular, flexible, and testable.
Moreover, it's crucial not to mirror the structure of the tests to the production code. Instead, tests should be designed independently to reduce coupling with the production code. This can be achieved by extracting private methods from the original functions and imposing a polymorphic interface between the tests and the production code. As the production code is refactored and private methods are extracted, new classes can be discovered and grouped together. This allows the structure of the tests to vary independently from the structure of the production code, promoting more comprehensive testing and allowing for the detection of potential issues or bugs early on in the development process.
To conclude, developers should embrace principles like the Single Responsibility Principle and the Dependency Inversion Principle to improve code maintainability and reliability. Simplicity and inversion of control are valuable tools in achieving these goals. By adopting strategies like unit testing and test-driven development (TDD), developers can ensure that their code is thoroughly tested from the start. TDD involves writing tests before writing the actual code, which helps to clarify the expected behavior and requirements. Through these methods, developers can greatly improve the testability of code and lead to more robust and maintainable software
3. Setting Up Your Java Unit Tests: An Overview
Establishing a robust unit testing environment in Java entails careful deliberation and execution of several key steps. The journey begins with the selection of an appropriate testing framework. JUnit, with its ubiquity and versatility, emerges as a favored choice for Java unit testing. The framework's annotation-based approach for identifying tests and assertion capabilities for verifying outcomes makes it a powerful tool in the hands of Java developers.
The subsequent phase involves setting up the development environment to streamline test execution. This includes configuring the IDE or build tool to identify and execute your tests, ensuring a smooth and efficient testing process.
The final, yet often neglected, step is to structure the tests effectively. A well-structured test is independent, succinct, and tests a single aspect of the code. The test name should reflect its purpose clearly, simplifying identification and resolution of issues.
JUnit's latest version, JUnit 5, has brought along several enhancements and new features. It continues the annotation-based approach for marking tests, such as @Test, and encourages the use of Hamcrest matchers for more expressive assertions.
JUnit provides a variety of features like test runners, control over test execution order, exception testing, parameterized tests, and more. To help developers utilize these features, JUnit offers extensive documentation, FAQs, and usage guides.
Moreover, JUnit also supports a host of third-party extensions, such as custom runners and utility classes, to further amplify its capabilities. JUnit QuickCheck, an extension providing parameter suppliers for JUnit theories, is particularly noteworthy.
To configure JUnit for your Java unit testing, begin by importing the necessary JUnit libraries into your project. This can be accomplished by adding the JUnit dependency to your project's build file. Next, create a new Java class for your test cases, separate from your main application code. Utilize JUnit annotations to define your test methods. Commonly used annotations include @Test
for marking a method as a test case and @Before
and @After
for defining setup and teardown methods. After writing your test cases using JUnit assertions, run your tests. Most modern IDEs support running JUnit tests, allowing for easy execution and results view.
When structuring unit tests, follow the Arrange-Act-Assert (AAA) pattern. This pattern enhances test code readability and maintainability. Ensure test method names are descriptive and tests are grouped into meaningful test classes. Use annotations like @Before
and @After
for setup and teardown, and keep tests independent and isolated. Choose assertions that are relevant to the specific scenario being tested.
For achieving independent and concise unit tests, each test should focus on a specific functionality or behavior. Use test fixtures or setup methods for a consistent state for each test. Mock external dependencies for better control over the test environment and use assertions to verify expected outcomes.
Additional resources like online tutorials, documentation, and books can be beneficial for a more comprehensive understanding of Java unit testing with JUnit. Platforms like Java Tutorials, Baeldung, and Tutorials Point offer in-depth tutorials on JUnit and Java unit testing. The official JUnit documentation provides detailed information about the framework and its features
4. Running a Unit Test in Java: A Step-by-Step Guide
The endeavor of conducting a unit test in Java can be a smooth and straightforward journey given the proper tools and understanding. Let's delve into a methodical guide. It all begins with the creation of your test using a testing framework, such as the widely used JUnit. This step involves initializing a new test class and crafting test methods. It's crucial to keep in mind that each method should be tasked with testing an individual unit of code.
Once your test is crafted, the subsequent step is to run it. With the aid of an Integrated Development Environment (IDE), such as Eclipse or IntelliJ, executing the test becomes as simple as right-clicking the test class and selecting 'Run As > JUnit Test'. The results of your test will be displayed in a dedicated view.
The concluding step is to scrutinize the test results. If a test fails, the testing framework will yield comprehensive details about the failure. This information proves invaluable in pinpointing and rectifying the issue.
JUnit 5, a renowned unit testing framework in the Java landscape, offers a plethora of features that can be employed to enhance the process. For instance, JUnit 5 tests can be crafted using Maven or Gradle build systems and can also be authored using the Eclipse IDE. JUnit 5 supports dynamic tests, facilitating the creation of multiple tests at runtime, and parameterized tests, permitting running tests with varying input values. JUnit 5 provides annotations for disabling tests, as well as conditional enablement of tests. Moreover, JUnit 5 allows for dictating the execution order of tests using annotations.
Beyond these features, JUnit 5 offers various assert statements for testing conditions, including testing for exceptions. These assert statements can be authored using static imports to condense the code and enhance readability. JUnit 5 also supports nested tests, allowing for the grouping of tests and additional setup/teardown methods.
In order to make the testing process more user-friendly, JUnit 5 offers the @TempDir annotation for generating temporary files and paths in tests. It's also noteworthy that JUnit 5 permits the creation of test suites, although support for this feature is still under development.
By utilizing JUnit 5 and the Eclipse IDE, you can author tests swiftly and effectively. For instance, you can author a JUnit 5 test using Maven and Eclipse or Gradle and Eclipse in a mere 5 minutes.
By capitalizing on the extensive features of JUnit 5, you can engineer robust and flexible unit tests, ultimately enriching your Java application's dependability and performance. As per the provided Solution Context, there isn't a direct solution for enhancing unit test coverage in Java projects. The context does not offer any specific details or examples related to unit test coverage or Java projects. Hence, it isn't feasible to provide a solution based solely on the given context
5. Leveraging JUnit 5 for Effective Java Unit Testing
JUnit 5, an updated version of the esteemed testing framework, introduces many improvements that can significantly enhance Java Unit Testing. This framework is specifically designed for Java 8 and later versions, offering a robust foundation for tests initiated by developers and supporting various test styles.
Key enhancements in JUnit 5 include powerful assertions, instrumental in validating expected test results, and assumptions, which allow tests to be terminated under certain conditions. The framework also includes conditions, a feature that enables tests to be run only under specific circumstances.
In addition, JUnit 5 introduces parameterized tests, allowing the same test to be run multiple times with different inputs, eliminating the need for developers to write repetitive test cases. The framework also supports dynamic tests that can be generated at runtime, providing more flexibility in testing scenarios.
The development of JUnit 5 began with the JUnit Lambda project and its crowdfunding campaign on Indiegogo. The creators of JUnit 5 encourage users to follow their work, review it, and provide feedback, promoting a community-driven development approach.
There are also extensive resources available for both beginners and seasoned developers who are already familiar with previous versions of JUnit. The tutorial is divided into different sections, including getting started, basics, advanced topics, and a practical guide for using JUnit 5 test lifecycle annotations. The tutorial also covers advanced topics such as using Mockito with JUnit 5 and migrating from JUnit 4 to JUnit 5. The guide also provides examples of running JUnit 5 tests with Maven and Gradle, making it a comprehensive guide for building support and running JUnit 5 tests.
One of the tools that can be leveraged to generate dynamic tests is Machinet AI Chat. To use this tool for code generation, visit the base URL: https://machinet.net, find the chat functionality provided by Machinet AI, and start a conversation with the AI chatbot. Specify that you want to generate code, provide any additional details or requirements for the code generation, and follow the prompts and instructions given by the AI chatbot to generate the desired code.
In summary, utilizing the power of JUnit 5 can lead to the development of more robust and flexible unit tests, ultimately improving the overall quality of the software being developed
6. Dealing with Changing Requirements: Implementing Robust and Flexible Testing Frameworks
In the dynamic world of software development, the constant evolution of requirements can pose a formidable hurdle. This hurdle, however, can be skillfully navigated by implementing robust and adaptable testing frameworks. A resilient testing framework is designed to withstand changes without succumbing to failure, while a flexible framework can be readily adjusted to accommodate new requirements.
Critical to this process is the design of tests that are independent, repeatable, and self-verifying. Additionally, the use of testing techniques inherently capable of accommodating changes, such as parameterized tests and mock objects, can further bolster the robustness and adaptability of the framework.
The notion of "fragile tests" in Test-Driven Development (TDD) is a significant consideration in this context. Fragile tests are those that necessitate substantial changes in response to minor amendments in the production code. To minimize this, the structure of the tests should not simply reflect the structure of the production code. This approach reduces coupling with the production code, thereby decreasing the fragility of the tests.
One effective strategy to achieve this involves decoupling the structure of the tests from the production code. For instance, private methods can be extracted into separate classes, thereby allowing the tests to be independent of the specific structure of the production code. However, it is crucial to ensure that the behavior of the tests and the production code remains coupled, despite the structural decoupling.
As development progresses, the behavior of the tests becomes more specific, while the behavior of the production code becomes more generic. This act of generalizing the production code is essentially an act of decoupling, as it allows the code to cater to a wide range of unspecified behaviors.
The ultimate goal is to enhance the generality of the production code to such an extent that no new failing tests can be written. This ensures that the unit tests remain effective and reliable, irrespective of changes in the software requirements.
Several robust testing frameworks are available in the industry that can help in achieving these goals. These include JUnit and TestNG for Java applications, NUnit for .NET applications, and PyTest for Python applications. These frameworks offer various features such as test case management, test automation, and parameterized testing.
By utilizing these robust testing frameworks, developers can create a structured approach to testing, identify potential issues early in the development process, automate test cases, and ensure that the software meets the desired functionality and quality standards. This helps in identifying and fixing bugs before the software is released, reducing the chances of errors and enhancing the overall reliability of the software.
In comparing different testing techniques for handling changing requirements, it is important to consider factors such as the flexibility of the testing approach, the ability to easily modify test cases, and the level of automation provided. By considering these factors, organizations can determine the most suitable testing technique for their specific needs and requirements.
Thus, with a robust and adaptable testing framework, you can ensure the consistent effectiveness of your unit tests, even in the face of changing requirements
7. Balancing Workload and Deadlines in Unit Testing Efforts
In the realm of proficient Java unit testing, the equilibrium of workload and deadlines is a significant aspect. This balance is attained through proficient resource management and efficient time utilization, ensuring comprehensive testing of every unit within the prescribed timeline. The prioritization of tests based on the complexity and significance of each unit is a crucial step in this process.
Moreover, the automation of tests is a key strategy that helps save time and effort while enhancing efficiency. For instance, the Mockito framework, a popular tool in Java, allows the creation of mock objects and stub methods, isolating the code being tested. Mockito's automation capabilities facilitate the simulation of diverse scenarios and behaviors without manual intervention, ensuring consistent and reliable test results. Additionally, Mockito's verification and argument matching features further enhance the automation of Java unit tests.
In the context of managing a large IT ecosystem, where the smooth operation and constant availability of Java-based web application services are critical, testing becomes paramount. One instance of this involves conducting a performance test on a basic Java microservice using a load test tool like SlapperX. This test allows us to understand the impact of blocking actions on web application requests and response times. It also enables us to observe how Java microservices behave under high load conditions and identify the threshold at which the service becomes unresponsive.
To manage these high load conditions, incorporating circuit breakers to prevent overload on backend systems is crucial. These can handle various error states, ensuring the system's smooth function. To manage blocking IO operations, we could explore the concept of reactive programming. The upcoming feature of virtual threads in Java 21 also shows promise in addressing the limitations of Java microservices under high load conditions.
Shadow testing, a technique that involves deploying a new or modified system alongside the existing production system, can be employed. This method allows developers to analyze the performance of the new system under real-world conditions. This helps in optimizing and fine-tuning its behavior before widespread deployment.
Shadow testing also enables data integrity verification by comparing the results of the new system with the existing one. This ensures accurate data migration and transformation processes. Various testing methodologies, such as functional testing, performance testing, and security testing can be applied in the shadow environment, providing a comprehensive assessment of the new system.
Implementing shadow testing requires the use of various tools like Docker, Kubernetes, VMware, Chef, Puppet, Jenkins, Travis CI, Prometheus, Apache JMeter, DBUnit, Cisco VIRL, OWASP Zap, New Relic, Dynatrace, Slack, ELK Stack, etc. These tools facilitate the creation of parallel environments, monitoring, and analysis of the new or modified system.
Lastly, constant evaluation and adjustment of testing methods are instrumental in keeping the testing process aligned with the set objectives. Following best practices and utilizing appropriate tools and techniques, such as leveraging frameworks like JUnit and using annotations and assertions effectively, is crucial. A test management tool or a test automation tool can help streamline the testing process and provide insights into the progress of the testing efforts. Regularly reviewing and analyzing test results can also help identify areas that require more attention or where adjustments need to be made.
In essence, balancing workload and deadlines in unit testing efforts is a complex but essential process. It requires effective strategies, the right tools, and a proactive approach. With these in place, we can ensure that our Java unit testing is efficient, effective, and up to the mark
8. Refactoring and Improving Existing Test Suites: Strategies and Best Practices
Refinement and enhancement of existing test suites are critical elements in maintaining the effectiveness of unit tests. This process involves a regular review of your tests to identify potential areas for improvement. The goal here is to enhance the readability and maintainability of your tests, and to ensure they stay up-to-date with any changes in code or requirements.
The DRY (Don't Repeat Yourself) principle should be maintained during this process to prevent redundancy. Meaningful names should be designated to your tests, and they should be organized effectively. The continual improvement of your test suites is crucial in maintaining their efficiency and effectiveness.
In the realm of software development, outdated or low-value tests can lead to "cruft" - unnecessary or redundant elements that can hinder team productivity. Regular assessment of tests aids in identifying this cruft, and provides the opportunity to retire or rewrite it. Factors such as the time taken to set up and run tests, recent bugs found, human effort saved, features exercised, and the maintenance burden can be used to evaluate tests.
To improve maintainability, refactoring your unit tests is recommended. Refactoring is the process of altering the code structure without impacting its functionality. By refactoring unit tests, you can enhance their readability, organization, and reusability. This can be achieved by breaking down complex tests into smaller, more focused ones, removing duplicated code, and ensuring that the tests are easy to understand and modify. Furthermore, the use of appropriate naming conventions and comments can aid in the maintainability of unit tests.
To maintain DRY unit tests and adhere to best practices, techniques such as test data builders for creating reusable test data, mocking frameworks for isolating dependencies, and parameterized tests for running the same test with different inputs can be utilized. Additionally, annotations like @Before and @After can be used to set up and tear down test fixtures, helping to maintain clean and organized test code.
Improving the readability of unit tests involves organizing them in a structured manner. This can be achieved by using a consistent naming convention for test methods and test classes, grouping related tests together, providing descriptive and meaningful comments within the test code, and using test fixtures or setup methods to reduce duplication and improve maintainability.
To enhance the maintainability of a test suite, it is recommended to follow certain best practices. These include organizing test cases into logical groups or categories based on functionality or test objectives, writing clear and concise test case names, minimizing test case dependencies, implementing a reliable and efficient test data management strategy, regularly reviewing and updating test cases, implementing a version control system, using automation tools and frameworks, establishing a process for reporting and tracking test failures and defects, encouraging collaboration between testers and developers, and providing proper documentation and guidelines for testers.
Lastly, it's crucial to remember that the process of refining and improving existing test suites is not a one-time task but a continuous process. It requires a systematic approach and the utilization of appropriate tools and techniques to ensure that your tests remain effective and efficient
Conclusion
In conclusion, unit testing in Java is a crucial aspect of software development that ensures the reliability and robustness of code. By thoroughly examining individual code components and comparing expected outcomes with actual results, developers can detect bugs early in the development process and enhance the quality of their code. Frameworks like JUnit and Mockito streamline the testing process by providing tools for setting up tests, mocking external dependencies, and asserting test outcomes. Best practices such as organizing tests effectively, achieving high test coverage, and using mocking frameworks contribute to more efficient and reliable unit testing efforts.
The ideas discussed in this article have broader significance for software engineers. By mastering the art of unit testing in Java, developers can optimize their coding practices and improve the functionality and reliability of their code. Unit testing helps catch bugs early on, reducing the time and effort spent on debugging in later stages. It also promotes better code organization and maintainability by encouraging modular design principles. Incorporating effective unit testing strategies ultimately leads to higher-quality software that meets the desired functionality standards.
Boost your productivity with Machinet. Experience the power of AI-assisted coding and automated unit test generation.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.