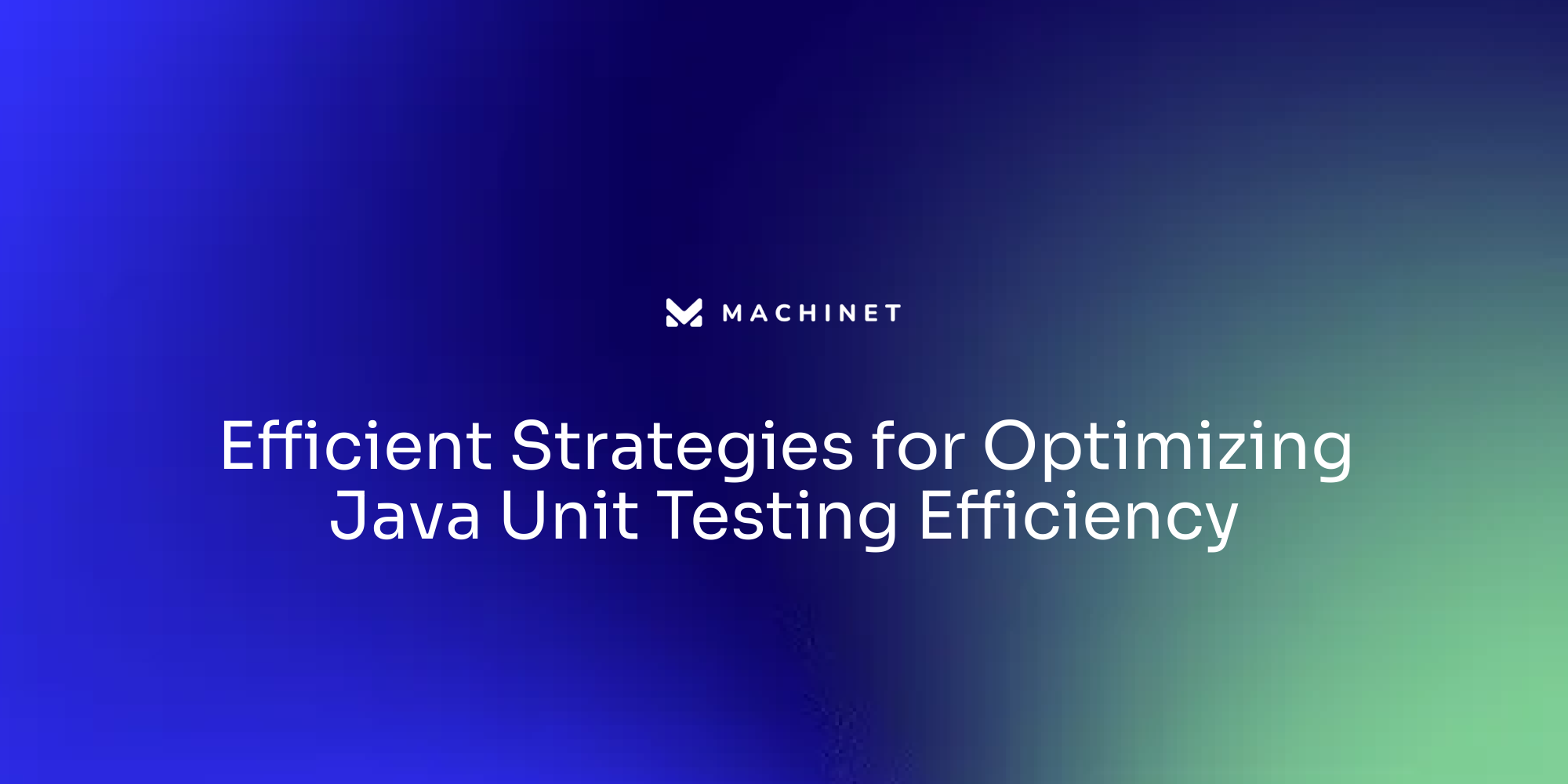
Table of Contents
- Understanding the Importance of Unit Testing in Java
- Exploring Different Frameworks for Java Unit Testing
- Techniques for Speeding Up Java Unit Tests
- Setting Up Efficient JUnit Projects: A Step-By-Step Guide
- Debugging Exceptions in Java Unit Tests: Best Practices
- How to Adapt Testing Frameworks to Changing Requirements
- Balancing Workload and Deadlines in Java Unit Testing Efforts
- Improving Code Quality through Effective Java Unit Testing
Introduction
Unit testing serves as a cornerstone within the software development landscape, particularly within the Java ecosystem. It equips developers with the ability to verify each code segment independently, ensuring that every unit functions as anticipated. This approach not only aids in the early identification and rectification of bugs but also aids in maintaining code quality and enhancing software reliability.
Moreover, unit testing plays a key role in managing technical debt as it facilitates refactoring without the fear of disrupting existing functionality. This is possible because unit tests act as a safety net, identifying any errors introduced during the refactoring process.
The advent of tools like Machinet further underscores the significance of unit testing. Machinet introduces automation into the unit testing process, improving efficiency by reducing manual effort and increasing accuracy by eliminating human error. The end result is a reliable, high-quality software product that aligns with the expectations and needs of its users.
In this article, we will explore the importance of unit testing in Java and its impact on code quality. We will delve into best practices for writing effective unit tests, including techniques for isolating units, organizing test cases, and leveraging automation tools. Additionally, we will discuss how Machinet can enhance the unit testing process by providing automation capabilities and guidelines for writing robust unit tests. By implementing these practices and utilizing tools like Machinet, developers can ensure the reliability and maintainability of their Java code
1. Understanding the Importance of Unit Testing in Java
Unit testing serves as a cornerstone within the software development landscape, particularly within the Java ecosystem. It equips developers with the ability to verify each code segment independently, ensuring that every unit functions as anticipated. This approach not only aids in the early identification and rectification of bugs but also aids in maintaining code quality and enhancing software reliability.
Moreover, unit testing plays a key role in managing technical debt as it facilitates refactoring without the fear of disrupting existing functionality. This is possible because unit tests act as a safety net, identifying any errors introduced during the refactoring process.
The advent of tools like Machinet further underscores the significance of unit testing. Machinet introduces automation into the unit testing process, improving efficiency by reducing manual effort and increasing accuracy by eliminating human error. The end result is a reliable, high-quality software product that aligns with the expectations and needs of its users.
For Java unit testing examples, an exploration of the blog.machinet.net website could prove beneficial.
Explore Java unit testing examples on blog.machinet.net
Blog posts on this platform cover a range of topics related to Java unit testing, including best practices and understanding Mockito for Java unit testing. A visit to these blog posts could provide specific examples and techniques related to Java unit testing.
To automate unit testing with Machinet, a series of steps can be followed. Firstly, a test suite that includes all the unit tests intended for automation should be set up. Then, Machinet's testing framework, designed for defining and running unit tests, should be used. Individual test cases for each unit of code to be tested should be written, ensuring coverage of all possible scenarios and edge cases. Machinet's testing framework can then be used to run the unit tests automatically, saving time and effort compared to manually running the tests. After the tests are run, the results should be analyzed to identify any failures or errors, aiding in the identification and rectification of any issues in the code. To further automate the unit testing process, Machinet can be integrated with a continuous integration (CI) system, allowing for automatic running of tests whenever changes are made to the codebase.

In terms of best practices for unit testing in Java, writing isolated and independent tests, using appropriate assertions, and incorporating test-driven development (TDD) principles are commonly recommended. Mocking frameworks like Mockito are often suggested for creating test doubles for dependencies and ensuring that tests are fast, reliable, and maintainable.
Unit testing also plays a vital role in improving code quality. By writing and running tests for each unit of code, developers can identify and rectify any issues or bugs, leading to improved code quality. Unit testing also aids in identifying and preventing regressions, ensuring that new changes or additions to the codebase do not break existing functionality. Further, unit testing promotes modular and reusable code, as well as providing documentation and examples of how the code should be used.
For effective unit testing in Java, several best practices can be followed. These include using a testing framework such as JUnit, TestNG, and Mockito, following the AAA pattern (Arrange, Act, Assert), testing for different scenarios, using mocking frameworks, keeping tests independent and isolated, using descriptive test names, using assertions effectively, and testing performance and scalability.
To generate unit tests for legacy code using Machinet, the best practices for Java unit testing can be followed. These include techniques such as writing testable code, using test doubles, applying dependency injection, and creating meaningful test cases. By following these practices, legacy code can be effectively tested and validated, ensuring its correctness and maintainability
2. Exploring Different Frameworks for Java Unit Testing
Java is a language rich in unit testing frameworks, each with its own set of unique characteristics tailored to meet varying project needs. JUnit often emerges as the preferred choice for many developers, owing to its simplicity, user-friendliness, and the support it receives from a wide community. However, other frameworks such as TestNG, Mockito, and PowerMock also offer compelling features such as data-driven testing, mocking, and parallel test execution.

JUnit, a mainstay in the Java community, encourages testing prior to coding, resulting in improved code quality and productivity. The framework enjoys immense popularity, with a projected global community of Java developers expected to reach 287 million by 2024, a significant portion of whom are likely to use JUnit for their unit testing needs. JUnit's straightforward API, automation of test execution, and features to measure test coverage make it an effective tool for unit testing. Moreover, it provides a range of assertion methods for validating expected code behaviour and annotations for organizing test cases, improving the readability and maintainability of the test suite.
Another open-source Java testing framework, TestNG, draws inspiration from JUnit and NUnit. It simplifies testing efforts with features like annotations, grouping, and parameterization, positioning it as a strong contender for developers seeking alternatives to JUnit.
Mockito, a dependable framework designed specifically for Java unit testing, is worth noting. It enables the creation of mock objects and supports behavior-driven development (BDD) based tests, proving especially beneficial for projects following the BDD approach.
PowerMock, on the other hand, provides advanced features such as mocking static methods, constructors, final classes and methods, private methods, removal of static initializers, and more. For advanced unit testing examples using PowerMock, resources like "Mocking Made Easy: Understanding Mockito for Java Unit Testing" and "Best Practices for Java Unit Testing: Tips and Techniques" on the MachineT.net website can be referred to.
Ultimately, the choice of a testing framework is dictated by the specific needs and requirements of the project. However, the Machinet AI-powered plugin, which can assist in selecting the most suitable framework, is worth considering. It not only aids in the implementation but also enhances the efficiency of Java unit testing by utilizing best practices for Java unit testing, such as using tips and techniques.
In the rapidly changing IT world, re-evaluating decisions is vital. For instance, while TestNG was previously favored over JUnit due to features JUnit lacked, such as parameterization, grouping, and test method ordering, the introduction of JUnit 5 has addressed these limitations. Therefore, staying abreast of the latest updates in these frameworks is crucial for making informed decisions that best serve the project's needs
3. Techniques for Speeding Up Java Unit Tests
Efficiency in unit testing, particularly within a continuous integration environment, is significantly influenced by the speed of the tests. To enhance the speed of Java unit tests, developers can employ a variety of strategies. A common technique involves minimizing unnecessary database interactions, which can often be sidestepped without compromising test integrity. This can be achieved through the use of mocking frameworks like Mockito, which allows for the creation of mock objects that simulate the behavior of the database, thus eliminating the need to interact with the actual database during testing.
Mocking frameworks are also useful in simulating dependencies, which allows tests to run in isolation and at a faster pace. This not only hastens the testing process but also makes the tests more reliable, as they become less dependent on external factors. Mockito is a popular choice for this purpose, offering a simple and intuitive API for creating mock objects and defining their behavior.
Another strategy to improve the speed of Java unit tests is parallel testing. By executing tests concurrently, the overall testing time can be significantly reduced. This approach requires careful management to avoid conflicts, but modern testing frameworks offer features that ease the handling of parallel execution. This technique improves test execution speed, better test coverage, and increases the likelihood of detecting bugs and performance issues.
Lastly, developers can leverage resources like Machinet.net for generating automated unit tests, which drastically cuts down the time spent on writing and maintaining tests. While specific information about Machinet's AI capabilities is not readily available, developers are encouraged to explore Machinet.net further or contact them directly for more information on their AI capabilities and services related to unit test generation.
In summary, enhancing the speed of Java unit tests involves a combination of strategies, including minimizing database interactions, using mocking frameworks, running tests in parallel, and leveraging AI capabilities. By implementing these techniques, developers can ensure that their unit tests are not only fast but also robust and reliable
4. Setting Up Efficient JUnit Projects: A Step-By-Step Guide
Updating a JUnit project to be more efficient involves several steps. The first step is to integrate the JUnit library into your project's classpath. You can accomplish this by downloading the JUnit library from the official website and adding it to your project's classpath through your IDE settings or properties.
Once the JUnit library is set up, it's crucial to organize your tests logically and systematically. This often reflects the structure of the application's package. You can achieve this by creating separate test classes for different functionalities or modules and using test suites to group related tests. It's also helpful to organize tests into different packages or directories based on their purpose.
For each class in your application, generate test cases. These should include numerous test methods that cover various scenarios and functionalities. When writing these test cases, focus on specific aspects or behavior of the class and verify that it functions correctly. This includes testing various input values, boundary cases, and error conditions. Remember to use the Arrange-Act-Assert (AAA) pattern when writing test cases. This involves setting up the necessary test data and objects (Arrange), performing the action or method being tested (Act), and then asserting the expected results (Assert).
Lastly, make use of annotations like @Before
, @After
, @BeforeClass
, and @AfterClass
for managing common setup and teardown tasks. These annotations help in maintaining a well-organized and scalable test suite.
JUnit 5, a widely recognized unit testing framework in the Java ecosystem, brings several new features based on the Java 8 version of the language. To use JUnit 5, it's necessary to configure your build system (Maven or Gradle) to include the JUnit 5 dependencies. Tests in JUnit 5 are defined as methods annotated with the @Test
annotation. JUnit 5 supports assertions and assumptions for testing expected results and conditions.
You can test for exceptions using the assertThrows
assertion. The assertAll
assertion allows you to group multiple assertions. The @TestFactory
annotation can be used to create dynamic tests. You can create Parameterized tests using the @ParameterizedTest
annotation. JUnit 5 also supports the creation of test suites. Nested tests and controlling the execution order of tests can be created with JUnit 5. The @TempDir
annotation can be used to create temporary files and paths for testing. Tests can be disabled using the @Disabled
annotation or conditional enablement using assumptions. JUnit 5 also provides support for generating test reports.
The JUnit 5 tutorial covers topics such as configuration for using JUnit 5, defining a test in JUnit, testing for exceptions, defining timeouts in tests, disabling tests, dynamic and parameterized tests, and more. It provides step-by-step instructions on how to write JUnit 5 tests with Maven and Eclipse, including project creation, configuring Maven dependencies for JUnit 5, creating a Java class, creating a JUnit test, running the test in Eclipse, fixing bugs, and reviewing the test code.
For Kotlin projects, the tutorial also details how to write a simple unit test using JUnit and the Kotlintest library. It provides instructions on how to set up a Kotlin project in IntelliJ IDEA and add the necessary dependencies. The tutorial includes steps for creating a sample class with a sum function and generating a test class for it. It demonstrates how to define a test function using the test annotation and check the expected result using the assertEquals
function. The tutorial explains how to run the test in IntelliJ IDEA and via the command line interface using Gradle.
In conclusion, setting up a JUnit project, whether in Java or Kotlin, involves several steps and considerations. With careful organization, comprehensive test cases, and the effective use of JUnit features and annotations, you can ensure your project is well-tested and reliable
5. Debugging Exceptions in Java Unit Tests: Best Practices
Exception handling in Java unit testing can be a complex task, but with the application of best practices, it can be significantly simplified. The key is to create tests that are clear, concise, and focused on specific behaviors or functionalities of the code. By keeping tests small and focused, it becomes easier to pinpoint the origins of any exceptions.
JUnit's @Test(expected)
annotation is a powerful tool for exception testing. This annotation allows developers to specify the expected exception type that should be thrown by the test method. If the test method throws the specified exception, the test passes; if it throws a different or no exception, the test fails.
A robust Integrated Development Environment (IDE) is another important asset. With an IDE's debugging capabilities, developers can set breakpoints in unit test code, pause the execution of the code at specific points, and inspect the values of variables and objects to identify the cause of the exception. Stepping through the code line by line allows for tracking the flow of execution and identifying potential issues.
The use of an AI plugin could potentially provide intelligent suggestions for debugging, but no specific information about such a plugin is provided in the context. However, there are other strategies that can be utilized to improve debugging. The rule of simplicity in code can be crucial in preventing multiple points of failure. The use of unmodifiable lists and fail-fast principles can aid in easier debugging.
The concept of double verification, which involves testing assumptions using different approaches, can be particularly useful in debugging. It allows for a more thorough verification of the code. Observability in debugging is another important aspect, but the information collected should be limited to a reasonable amount to avoid information overload.
Running failure scenarios and conducting bug debriefs can enhance the debugging process. Feature flags can serve as a creative verification tool. Regularly reviewing logs and dashboards can help track potential bugs and establish a baseline for a healthy system.
While it's essential to prepare for failures and unexpected bugs in production, proper observability in production systems is equally important. It can help prevent major blind spots, enabling developers to identify and address issues more efficiently when debugging exceptions in Java unit tests
6. How to Adapt Testing Frameworks to Changing Requirements
The evolution of software development necessitates a parallel evolution in testing frameworks. The focus is not just about creating tests that work in the present, but also ensuring these tests remain effective as the software evolves. This calls for tests that are flexible, maintainable, and adaptable to changes.
Following best practices for unit testing can contribute significantly to writing these flexible and maintainable tests. A key aspect of these best practices is designing tests that are independent, ensuring they do not rely on the state of other tests or external dependencies. Isolating tests from each other means changes or updates to one test will not affect the execution or results of other tests.
Another critical practice is using clear and descriptive naming conventions for tests. This approach makes it easier for developers to understand the purpose and functionality of each test, facilitating maintenance and troubleshooting. Organizing tests into logical groups or categories can further aid in test management and maintenance.
Techniques such as parameterization and data-driven testing enhance flexibility and maintainability. Parameterization allows for testing different input values without writing separate test cases for each scenario. Data-driven testing leverages external data sources to drive test execution, making it easier to update test data and scenarios without modifying the test code.
To avoid hard-coded values in software testing, consider using variables or configuration files. This practice allows for easy changes to values without modifying the code itself. Additionally, using constants or enums can also help to avoid hard-coded values, thereby improving the maintainability of the tests.
Setup and teardown methods are common in software testing to perform common tasks. These methods set up the initial state of the test environment before each test case and clean up any resources after each test case. Automating these common tasks ensures consistent and efficient testing processes.
Mocking frameworks can further contribute to test adaptability. These frameworks help isolate tests from external dependencies, making them more resilient to changes. This is particularly important in an agile environment where continual learning and application of learnings are key to product and process adaptation.
Incorporating AI capabilities into the testing process can further enhance test adaptability. AI can provide guidance and generate adaptable test code, useful in complex software development environments. For instance, Machinet's AI capabilities can assist in writing adaptable test code by analyzing and understanding the codebase. It can provide insights and suggestions on how to improve the test code to make it more flexible and resilient to changes.
Adapting testing frameworks to changing requirements is a critical aspect of software development. By following the principles and techniques outlined above, developers can create flexible and maintainable tests that effectively cater to the evolving needs of the software
7. Balancing Workload and Deadlines in Java Unit Testing Efforts
In the pursuit of effective Java unit testing, the balance between workload and deadlines is crucial, as is the prioritization of tests based on risk and complexity associated with the code. Prioritizing tests for critical and complex functionalities should be the initial focus. This approach can be achieved by analyzing the potential impact of a failure in these areas and the likelihood of encountering issues. Code coverage tools can be instrumental in identifying areas of the code that are not adequately covered by tests, thus serving as a guide for test prioritization.
Automation plays a significant role in enhancing the efficiency of the testing process. The majority of this process can be automated, allowing developers to focus on more intricate tasks. A tool like Machinet can be utilized for this purpose as it provides a framework to write automated tests for Java code. This can significantly save time and effort in the testing process, allowing developers to dedicate more time to challenging tasks.
Overburdening developers can lead to decreased productivity and unfinished tasks. Hence, striking a balance in workload is vital. This can be achieved by breaking down testing tasks into smaller, manageable chunks, prioritizing based on their criticality, and automating repetitive tasks using frameworks like Mockito. Effective communication within the team can also aid in managing the workload and meeting deadlines.
Efficiency in testing can also be improved by using best practices and techniques. Using a mocking framework like Mockito can simulate dependencies and isolate the unit under test, avoiding the need for time-consuming setup and teardown operations. Machinet's built-in features such as test data generation and test case management can further streamline the testing process.
Another important aspect to consider is the balance between resource efficiency and flow efficiency. Resource efficiency refers to the percentage of time a resource is productive, while flow efficiency refers to the percentage of time value is added to a flow unit. Prioritizing flow efficiency over resource efficiency can lead to increased productivity and reduction in hidden costs.
In the realm of Java unit testing, strategies such as using mocking frameworks, parameterized tests, and test-driven development can help save time and effort. These strategies enable developers to focus on the specific logic being tested, streamline the testing process, and catch issues early on, ultimately leading to more efficient and effective testing.
Unit testing is a critical aspect of software development. It plays a vital role in bug detection and prevention, provides a safety net for code refactoring and maintenance, and serves as living documentation for the codebase. Automated unit testing tools like Machinet offer several benefits such as test frameworks and runners, code coverage analysis, continuous integration integration, mocking and test doubles, parameterized testing, test execution reporting, test data management, test prioritization, and integration with IDEs.
To integrate Machinet into your Java unit testing workflow, you can add the Machinet library as a dependency in your project, use the Machinet API to generate test cases and test data for your Java unit tests, configure your test environment to use Machinet as the test data source, write your unit tests using the generated test data from Machinet, and run your unit tests and monitor the results provided by Machinet.
Overall, effective unit testing promotes code quality, bug detection, and maintainability. Integrating unit testing into the development process and using automated testing tools like Machinet are essential for delivering reliable software products
8. Improving Code Quality through Effective Java Unit Testing
Java unit testing is a critical aspect of contemporary software development, serving a multitude of purposes. It involves the verification of individual code units in isolation, which allows for the identification and correction of bugs at an early stage. This strategy not only streamlines the maintenance of code quality but also provides a safety net for refactoring tasks, aiding in the management of technical debt.
Unit tests act as an early warning system, highlighting potential issues before they evolve into significant problems. They empower developers to confidently modify the codebase, secure in the knowledge that the current functionality will remain unaffected. Additionally, they function as living documentation, providing in-depth information about the expected behaviour of the code units and the input-output relationships.
Creating effective unit tests requires a thorough understanding of the unit's expected behaviour and the design of test cases that encompass various scenarios, including edge cases and boundary conditions. The Arrange-Act-Assert (AAA) pattern is a beneficial framework for unit tests, guiding developers to arrange the test conditions, act on the unit under test, and assert the expected outcomes. It's also vital to isolate the units being tested from external dependencies using test doubles such as mock objects or stubs, ensuring the tests are independent, fast, and can run in any order.
Automated unit testing tools bring additional benefits. These tools offer frameworks and runners, code coverage analysis, continuous integration integration, mocking and test doubles, parameterized testing, test execution reporting, test data management, test prioritization, IDE integration, and cross-browser and cross-platform testing. These tools simplify the unit testing process, making it easier to create, execute, and analyze the tests.
When manually writing unit tests, developers need to pinpoint the specific code units to test, set up the test environment, define the test cases, follow the AAA pattern, handle exceptions, and maintain the tests as the codebase evolves. This process not only ensures the quality and reliability of the software product but also enhances developer productivity, shortens development cycles, and fosters a culture of continuous improvement.
Leveraging tools like Machinet can amplify unit testing efforts, leading to enhanced code quality and overall software reliability. By adhering to best practices for unit testing and utilizing automated unit testing tools, developers can effectively manage bug detection and prevention, enhance code quality, and reap benefits such as cost savings, reduced rework, early user feedback, increased testing efficiency, confidence in the software product, stress reduction, and seamless integration with continuous integration and deployment pipelines.
Machinet.net is a valuable resource for unit testing, offering blog posts on various topics related to the subject. For instance, the blog post titled 'Best Practices for Java Unit Testing: Tips and Techniques' provides insights and recommendations for writing effective unit tests, including tips for test organization, test coverage, and test data management. By diligently following these best practices, developers can ensure that their unit tests are robust, reliable, and contribute to overall code quality.
In addition to providing valuable blog posts, Machinet also provides guidelines and instructions on how to use the tool for unit testing. To use Machinet for unit testing, one can visit the base URL of Machinet, identify the specific chunk ID that corresponds to the unit testing functionality of interest, ensure that the domain is 'machinet.net' and the type is 'url', access the URL provided for unit testing, and follow the guidelines and instructions provided on the webpage.
By automating unit testing with Machinet, developers can utilize the provided base URL and the URLs of the blog posts on the Machinet website to gain access to relevant information and resources related to unit testing. Furthermore, they can explore the different techniques and best practices for unit testing described in the blog posts, which will assist in automating and improving the unit testing process.
In conclusion, Machinet is a robust tool for unit testing that can significantly improve code quality and software reliability when used correctly and in accordance with best practices
Conclusion
In conclusion, unit testing plays a vital role in the Java development landscape. It allows developers to verify each code segment independently, ensuring that every unit functions as intended. Unit testing helps in early bug detection and rectification, maintains code quality, and enhances software reliability. It also aids in managing technical debt by providing a safety net during refactoring. The introduction of automation tools like Machinet further strengthens the importance of unit testing. Machinet automates the unit testing process, reducing manual effort and increasing accuracy. By implementing best practices and utilizing tools like Machinet, developers can ensure the reliability and maintainability of their Java code.
The significance of unit testing goes beyond its impact on code quality. It also contributes to the overall efficiency and productivity of the development process. By catching bugs early on, developers can save time and effort that would otherwise be spent on debugging and fixing issues later in the development cycle. Additionally, unit tests act as living documentation, providing insights into the expected behavior of code units and facilitating collaboration among team members. Automation tools like Machinet further boost productivity by streamlining the testing process and allowing developers to focus on more complex tasks. Overall, incorporating effective unit testing practices and leveraging automation tools like Machinet is essential for delivering high-quality software products.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.