
Table of Contents
- What is Java Exception Handling?
- Understanding Try-Catch Blocks
- The Power of Try-Catch
- Multiple Catch Blocks
- The Finally Block
- Nested Try-Catch Blocks
- Checked and Unchecked Exceptions
- Best Practices for Exception Handling
Introduction
Java Exception Handling is a crucial aspect of the Java programming language. It offers a structured way to handle runtime errors, ensuring smooth application performance. Understanding and implementing proper exception handling is key to enhancing the reliability and fault-tolerance of Java applications. This article explores various aspects of Java Exception Handling, including the concept of exceptions, try-catch blocks, multiple catch blocks, the finally block, nested try-catch blocks, and best practices for exception handling. By mastering these concepts, developers can ensure their Java applications are resilient and capable of handling runtime errors effectively.
What is Java Exception Handling?
Java Exception Handling is not just a mechanism, it's a crucial aspect of Java, a language acclaimed for its robust handling of exceptions. It offers a structured way to combat runtime errors, thus promoting smooth application performance. An exception in Java is an event that interrupts the usual flow of program, and can lead to abrupt termination if not properly managed. There are two primary categories of exceptions in Java, and understanding them is key to implementing effective exception handling. When an exception arises, the Java runtime will halt the execution of the current method, and pass an exception object, which contains error details, to the nearest catch block capable of handling the exception.
Throwing exceptions efficiently is as important as catching and handling them. To indicate an exception, you need to throw one using the 'throw' keyword, which initiates the exception machinery in the Java Virtual Machine (JVM). This creates a new exception object that encapsulates information about the disruptive event, including the exception type and a detailed message about the event. By incorporating proper exception handling, you can enhance the reliability and fault-tolerance of your Java applications. This will not only help in dealing with runtime errors but also elevate the quality of your code, making you a more proficient Java developer.

Understanding Try-Catch Blocks
Java's exception handling mechanism, a crucial element for application robustness and fault tolerance, revolves around the concept of events that disrupt the normal flow of the program. These events, known as exceptions, are runtime objects that may be caught by catch blocks. If left unhandled, they can abruptly terminate your program. A fundamental structure in managing these exceptions is the Try-Catch block. Within the Try block, we place the code that could potentially throw an exception.
In the event of an exception, the Catch block intercepts it, providing an opportunity for the developer to write code that addresses the exception. try { // Code that may throw an exception} catch (ExceptionType e) { // Code to handle the exception}
Exception handling in Java extends beyond just catching exceptions. It's also important to know how to effectively throw them. When an exception occurs, the Java runtime halts the execution of the current method and passes an exception object to the nearest catch block capable of handling it. The exception object carries crucial information about the event, reflected in the exception type and other properties, like the exception message, which provides detailed insights into the event. By mastering these concepts and properly implementing exception handling, developers can ensure their Java applications are resilient, capable of handling runtime errors, and maintaining smooth execution.

The Power of Try-Catch
Java's Try-Catch blocks empower programmers to tackle exceptions smoothly, ensuring the program's continuity even in the face of potential errors. The encapsulation of code that could potentially trigger exceptions within a Try block ensures that the program's normal flow remains undisturbed, even if an exception arises. The Catch block then provides a controlled environment for handling these exceptions, which could include actions like logging the error, showcasing an error message, or executing corrective measures to deal with the exception. Consider the case of a project where a lock firmware platform, a server, and a homegrown TCP protocol were built. The system was riddled with issues, with the server hanging regularly and the locks frequently becoming unresponsive. The high incidence of exceptions made the code challenging to debug, but the Try-Catch mechanism was instrumental in handling these exceptions. Exception handling in Java is more than just dealing with errors at runtime.
It enhances the reliability of the application, making it more robust and fault-tolerant. For instance, NullPointerExceptions, which occur when an attempt is made to access or modify an object reference with a null value, can disrupt an application's flow, leading to crashes or unexpected behavior. Proper handling of such exceptions can significantly enhance the application's stability and reliability. However, it's important to remember that while Try-Catch blocks are a key feature of error handling, they aren't the only tool available. Over-reliance on this construct can sometimes lead to verbose and hard-to-maintain code. Alternative approaches can help make your code cleaner, more robust, and easier to reason about. For instance, the Antithesis platform uses a fuzzer to find interesting scenarios, injecting faults to explore branches of the code that were previously unexplored. In summary, while Try-Catch blocks are an essential part of Java's exception handling mechanism, they should be used judiciously and in conjunction with other strategies to ensure high-quality, robust, and maintainable code.
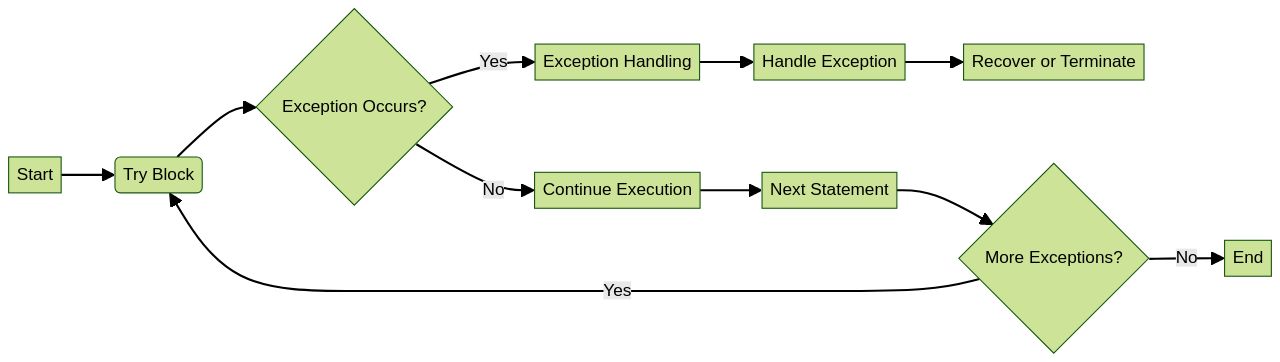
Multiple Catch Blocks
In the realm of Java, developers are equipped with the ability to implement multiple Catch blocks following a single Try block. This distinct feature enhances the developer's ability to manage a variety of exceptions separately, lending them the power to define specific actions for each exception type. It's like having a personalized toolkit, where each Catch block is designed to handle a unique exception type. This, in turn, gives developers a more nuanced and precise control over managing exceptions. The Catch blocks are then assessed in a sequential order, and the first Catch block that aligns with the exception is put into action.
It's like a relay race where each participant (Catch block) is ready to take the baton (exception) and run their course, but only the one that matches the exception type gets to run. This unique feature of Java not only makes the code more reliable but also enhances its fault tolerance. However, one must be mindful not to leave any Catch block empty and avoid catching overly broad exceptions. Moreover, with the introduction of virtual threads in Java 19, the performance of server applications has seen significant improvement. This, combined with the fact that Java has a release every six months, ensures that developers are always equipped with the latest tools and features to handle exceptions and other tasks efficiently. The robustness and networking focus of Java, as stated by James Gosling, the creator of Java, further reinforces its reliability and efficiency in handling exceptions.
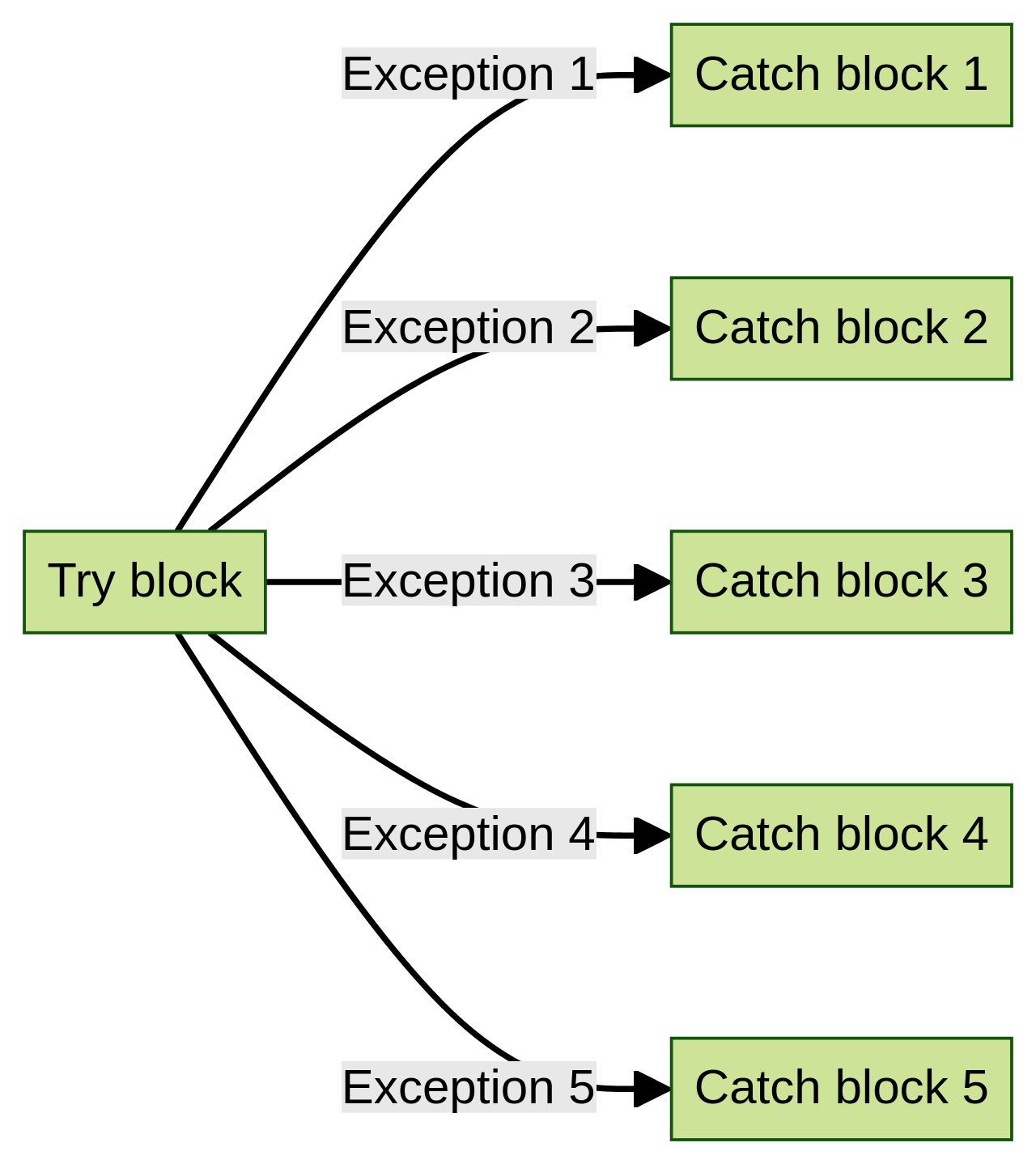
The Finally Block
Java's Try, Catch, and Finally blocks are pivotal tools for managing potential errors during runtime. Consider a scenario where a program reads data from a file. Several issues might arise, such as missing or unreadable files, or lack of user permissions. The Catch block is designed to anticipate and handle these exceptions, preventing abrupt program termination. However, Java also offers the Finally block, an optional yet significant feature.
The Finally block ensures the execution of crucial code segments, regardless of the outcome in the Try block. This is typically used for releasing resources or performing cleanup operations that need to be done regardless of whether an exception was thrown or not. Understanding and implementing these blocks can significantly enhance the robustness and fault-tolerance of Java applications. It's also worth noting that writing clean, understandable, and maintainable code is paramount in Java, one of the most widely used programming languages globally. Such principles and best practices not only make the code functionally correct but also easy to read, understand, and modify, ultimately leading to enhanced readability, reliability, and scalability of Java applications.

Nested Try-Catch Blocks
Java supports hierarchical exception handling through the nesting of Try-Catch blocks. This feature allows a Try block to encapsulate another Try-Catch block, offering a tiered approach to managing exceptions during code execution. This becomes particularly useful in scenarios requiring different levels of exception handling or specific actions based on the outcome of inner Try-Catch blocks. In the world of Java, the use of Try-Catch blocks is a cornerstone of error handling, contributing to the creation of resilient software. This approach allows for the graceful management of both checked and unchecked exceptions, preventing unexpected crashes.
This includes the graceful closure of resources such as files, streams, and database connections through the use of try...catch...finally and try...with...resources constructs. However, it's crucial to remember that empty catch blocks should be avoided, exceptions should be logged at minimum, and overly broad exceptions should not be caught. While nesting Try-Catch blocks can provide robust error handling, it's important to note the performance implications. As the number of nested loops increases, the time complexity of your code can escalate, impacting your program's performance, especially if the iterations are large. In the recent years, Java has introduced features like auto-compile and the var keyword, making the platform more accessible for beginners and veterans alike. These features, along with the robust exception handling mechanisms, contribute to the ongoing evolution of Java, making it a powerful tool in the hands of developers.
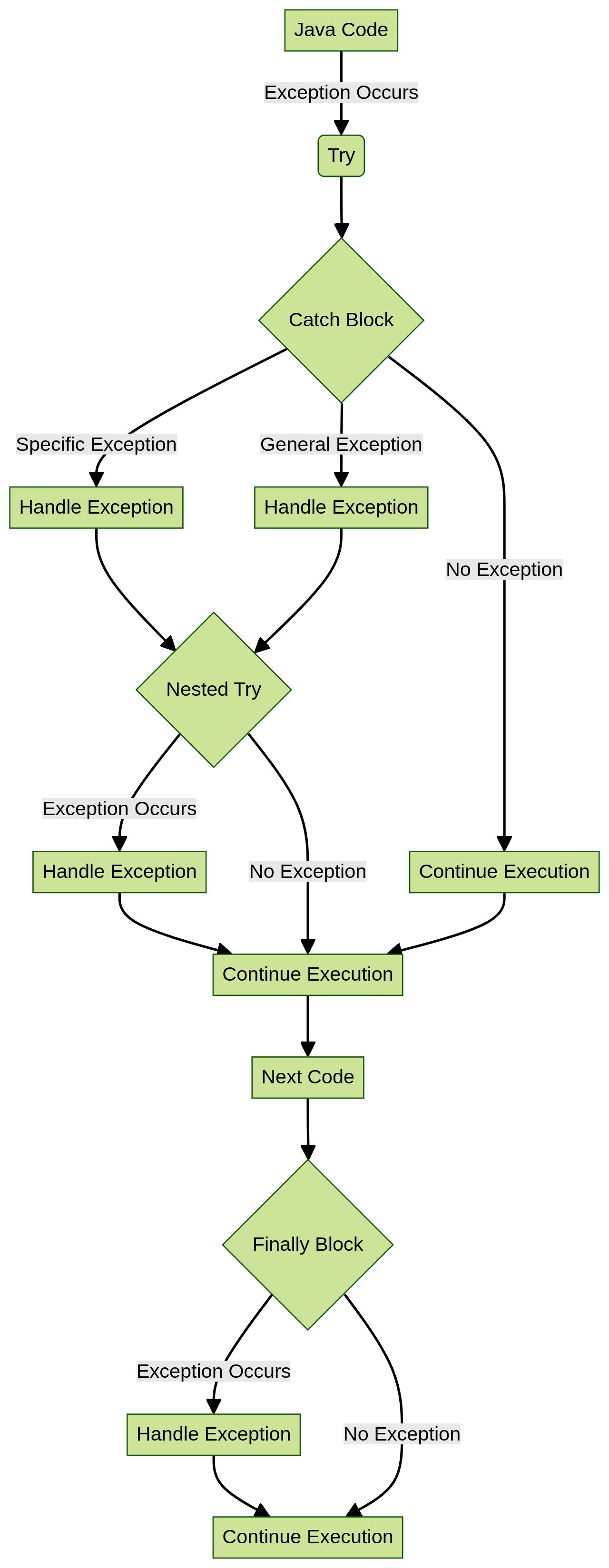
Checked and Unchecked Exceptions
Java, uniquely among mainstream programming languages, implements the concept of checked exceptions, a once innovative but now controversial feature. These exceptions must be explicitly declared or caught, with IOException and SQLException serving as prime examples. However, unchecked exceptions, such as NullPointerException or ArrayIndexOutOfBoundsException, do not require this explicit handling. They mainly represent programming errors that disrupt the typical flow of instructions. When such an exception occurs, the Java runtime halts the current method execution and passes an exception object detailing the error to the nearest capable catch block.
This object contains key information about the event, including the exception type and a message describing the incident in greater detail. It's crucial to handle exceptions gracefully, but equally important is knowing how to effectively throw them. This involves using the 'throw' keyword to trigger the Java Virtual Machine's exception handling mechanism and create a new exception object. Errors, another category, are exceptional conditions occurring at runtime, typically out of the programmer's control. They present serious issues that often can't be handled by the program, and attempting to recover might lead to unpredictable behavior.

Best Practices for Exception Handling
Java, a widely accepted programming language, is applauded for its robust exception handling capabilities. Exception handling is a crucial Java concept that lets developers manage runtime errors, ensuring the application runs smoothly. An exception, an event disrupting the program's regular flow, is an object thrown at runtime, and caught by catch blocks. If not adequately managed, it can abruptly terminate your program. There are two primary categories of exceptions in Java, and understanding and implementing proper exception handling makes Java applications more robust and fault-tolerant. When an exception occurs, Java's runtime automatically ceases the current method's execution. It then passes an exception object, carrying information about the error, to the nearest catch block capable of managing the exception. Here are some best practices for effective and efficient error management in Java:
- Catch specific exceptions instead of generic Exception types.
- Manage exceptions at a suitable code execution level. - Give meaningful error messages for easier debugging. - Use Finally blocks to release resources. - Refrain from using empty Catch blocks as they can conceal potential issues. - Log exceptions for future reference and troubleshooting. Throwing Java exceptions, including different exception types and creating custom exceptions, is as vital as catching and managing them gracefully. Throwing an exception in Java involves invoking the exception machinery in Java Virtual Machine (JVM). When you throw an exception, you create a new exception object that contains information about the occurred event, reflected by the exception type and several other properties, including the exception message describing what happened in more detail.
Conclusion
Java Exception Handling is a crucial aspect of the Java programming language. It offers a structured way to handle runtime errors, ensuring smooth application performance. Understanding and implementing proper exception handling is key to enhancing the reliability and fault-tolerance of Java applications. This article explores various aspects of Java Exception Handling, including the concept of exceptions, try-catch blocks, multiple catch blocks, the finally block, nested try-catch blocks, and best practices for exception handling. By mastering these concepts, developers can ensure their Java applications are resilient and capable of handling runtime errors effectively. The article emphasizes that Java Exception Handling plays a vital role in managing runtime errors and promoting smooth application performance.
It highlights the importance of both catching and throwing exceptions efficiently to enhance the reliability and fault-tolerance of Java applications. The use of try-catch blocks provides developers with a controlled environment to handle exceptions and prevent abrupt program termination. Additionally, the article discusses best practices such as catching specific exceptions, giving meaningful error messages, using finally blocks to release resources, and logging exceptions for future reference. To become proficient in Java Exception Handling, developers should thoroughly understand the concepts discussed in this article and implement them in their codebase. By doing so, they can ensure their applications are robust and capable of gracefully handling runtime errors. Start now to enhance your Java programming skills by mastering exception handling techniques. <"Start now">
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.