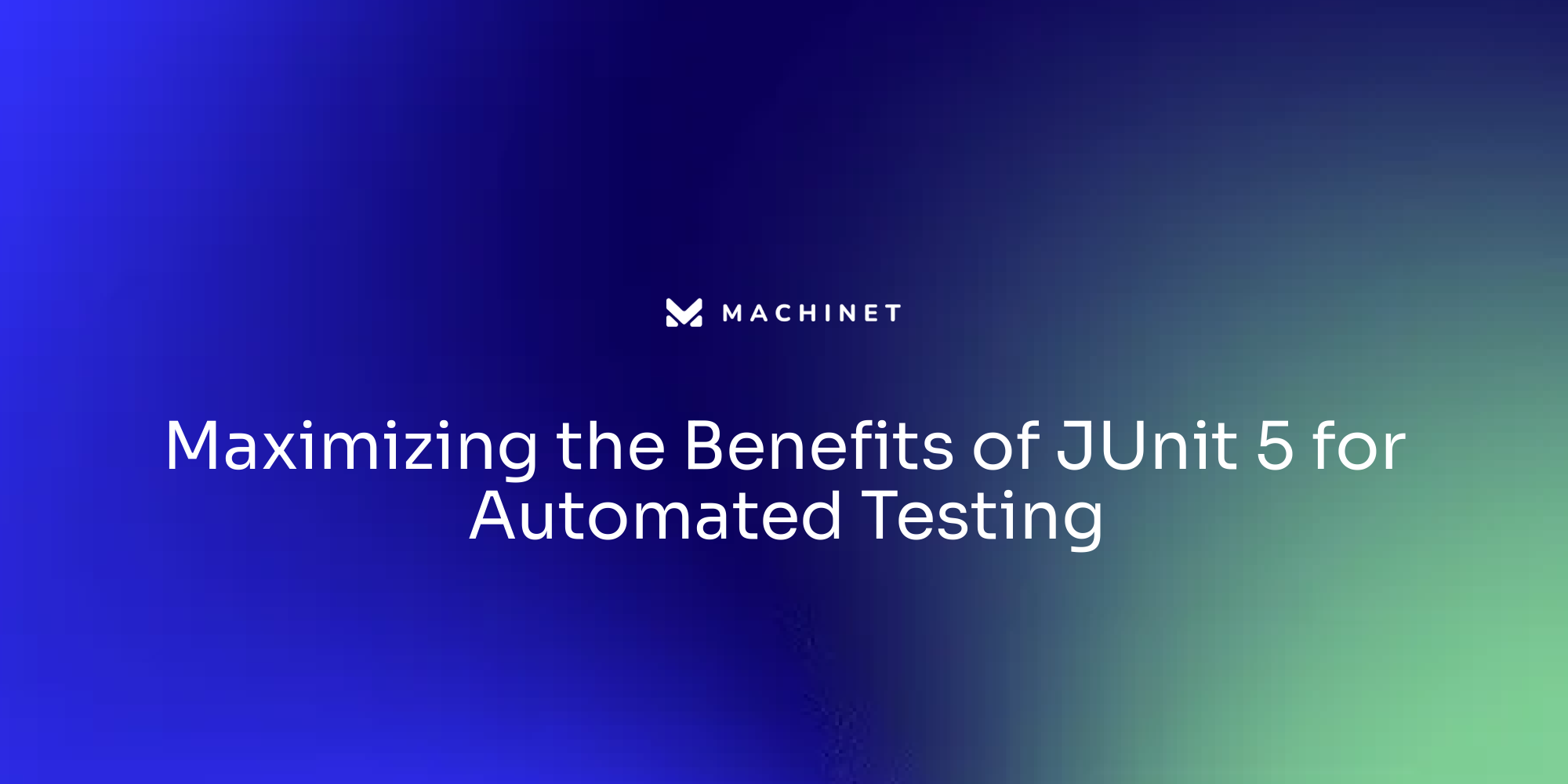
Table of Contents
- Understanding the Basics of JUnit 5
- Key Advantages of Using JUnit 5 for Automated Testing
- Migrating from JUnit 4 to JUnit 5: A Step-by-Step Guide
- Implementing Robust and Flexible Testing Frameworks with JUnit 5
- Strategies for Managing Workload and Balancing Deadlines in JUnit 5 Testing
- Addressing Common Challenges in Automated Testing with JUnit 5
- Case Study: Successful Implementation of JUnit 5 in a Real-World Project
Introduction
JUnit 5, the latest version of the popular Java testing library, brings a host of new features and enhancements that make it a powerful tool for automated testing. With its modular architecture and support for Java 8 and above, JUnit 5 offers developers increased flexibility, customization, and improved test readability. This article explores the basics of JUnit 5, its key advantages, migration strategies, implementation of robust testing frameworks, and strategies for managing workload and balancing deadlines. Whether you're new to JUnit or looking to upgrade from JUnit 4, this article provides valuable insights and guidance to help you harness the full potential of JUnit 5 for your testing needs
1. Understanding the Basics of JUnit 5
JUnit 5, a modern and innovative addition to the Java testing ecosystem, introduces a myriad of enhancements and novel features that differentiate it from its predecessor, JUnit 4. The design of JUnit 5 is built with power and flexibility in mind, with its modular architecture enabling increased customization and extensibility.
The architecture of JUnit 5 consists of three main components: the JUnit Platform, JUnit Jupiter, and JUnit Vintage.
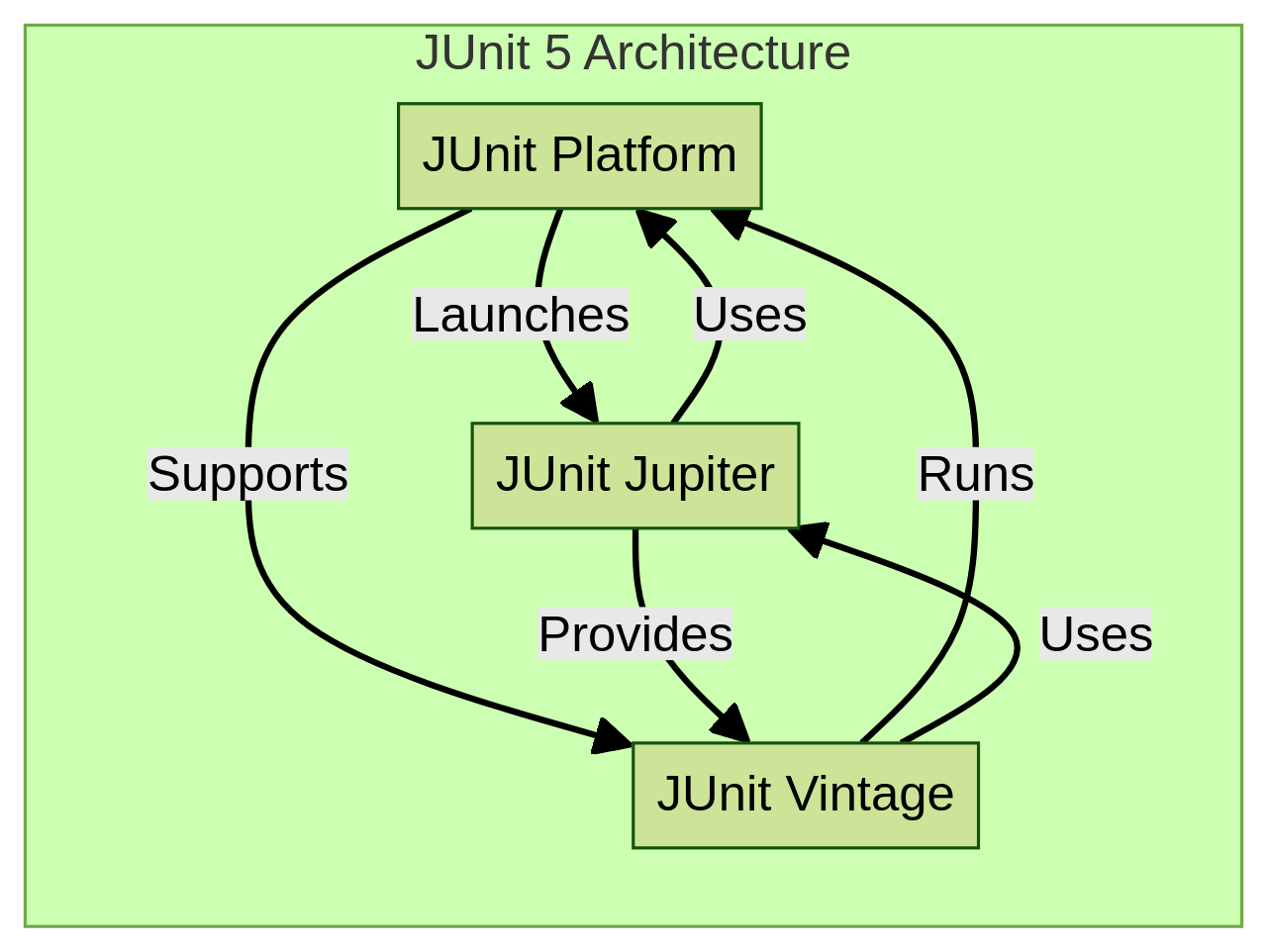
The JUnit Platform serves as the foundation for launching testing frameworks on the Java Virtual Machine (JVM). Jupiter introduces a new programming and extension model for creating tests, while Vintage ensures compatibility by facilitating the execution of tests written in JUnit 3 and 4.
Designed with the modern era in mind, JUnit 5 emphasizes Java 8 and subsequent versions. This focus allows it to support various testing styles, thereby offering a comprehensive platform for developer-side testing. Interestingly, the development of JUnit 5 was made possible through a successful crowdfunding campaign on Indiegogo.
The JUnit team encourages users to follow their ongoing work, review it, and provide feedback. They provide a user guide, Javadoc, GitHub repository, and issue tracker as resources. To facilitate their work on JUnit with greater focus and efficiency, they request support from users in the form of donations. The work of JUnit is also supported by numerous sponsors, including IntelliJ IDEA, Micromata, Quo Card, Premium Minds, Testmo, Codefortynine, Info Support, Stiltsoft, and Code Intelligence.
The JUnit team utilizes GitHub for version control, project management, and continuous integration, and they depend on Develocity to analyze and speed up their builds. The latest JUnit artifacts can be downloaded from Maven Central. For updates and news, users can follow the JUnit team on social platforms like Mastodon and Twitter.
Embodying the spirit of the modern era, JUnit 5.4, the most recent version of the popular testing library, offers several improvements over its predecessor, version 5.3.2. It incorporates features such as lambda support, test method parameter injection, and more. The process of including the library has been streamlined in JUnit 5.4, which requires only the single junit-jupiter dependency.
JUnit 5.4 also introduces the use of temporary directories for testing, providing an extension to create and clean up temporary files. Parameterized tests now support null and empty values as input. Another significant feature is the ability to generate the display name of test methods dynamically based on the nested class or method name.
Moreover, JUnit 5.4 introduces the ability to order test methods using the @Order
annotation in conjunction with the @TestMethodOrder
annotation. The comprehensive documentation for JUnit 5 provides detailed explanations and code examples. Given the multitude of improvements and features it offers, migrating to JUnit 5 is highly recommended.
JUnit 5 also offers customization and extensibility features that enable developers to customize their testing experience to meet their specific requirements.
Customize your testing experience with JUnit 5 and improve the efficiency of your testing process!
These features include the ability to create custom annotations and assertions, as well as the capacity to extend the functionality of JUnit 5 through custom extensions. This allows developers to create more powerful and adaptable unit tests in Java.
To write tests in JUnit Jupiter, developers can use annotations such as @Test
, @BeforeEach
, and @AfterEach
to define test methods and setup and teardown methods. Assertions like assertEquals()
and assertTrue()
can be used to verify the expected behavior of the code. Additionally, JUnit Jupiter provides features like parameterized tests and test interfaces to enhance test flexibility and reusability
2. Key Advantages of Using JUnit 5 for Automated Testing
JUnit 5 represents the most recent generation of the renowned Java testing library, offering a multitude of advantages for automated testing through a slew of new features and improvements.
Upgrade to JUnit 5 and experience the benefits of modern automated testing!
This potent tool was born out of the JUnit Lambda project, shaped by contributions from an Indiegogo crowdfunding campaign.
One of the most notable benefits of JUnit 5 lies in its modular architecture, which fosters a higher degree of flexibility and customization. This new architecture simplifies dependency management, allowing developers to use only the components they need, thus reducing unnecessary overhead. For example, you can adopt JUnit 5.4 simply by including the junit-jupiter dependency, an aggregating artifact that brings together the necessary components.
JUnit 5 also introduces new annotations and assertions that enhance the readability of tests and streamline their creation. The @DisplayName
annotation is a prime example of this, allowing for more descriptive test names, promoting clarity and comprehensibility. This feature is even more potent in JUnit 5.4, where display names can be dynamically generated based on the nested class or method name. Additionally, the assertAll
assertion enables grouped assertions, simplifying the process of validating multiple conditions.
Another significant advantage is JUnit 5's support for Java 8 and above, empowering developers to utilize the latest features of the Java language. This emphasis on modern Java versions is evident in features such as lambda support and test method parameter injection, which are integral to contemporary Java development practices.
The robustness and flexibility of test suites are enhanced in JUnit 5 through improved support for parameterized tests and conditional test execution. For instance, JUnit 5.4 introduces support for temporary directories in tests, which simplifies the handling of file creation and cleanup. It also allows null and empty input values in parameterized tests, offering greater flexibility in test data. Moreover, the new orderannotation
and order
annotations in JUnit 5.4 enable the specification of the execution order of test methods, giving precise control over the test flow.
The myriad improvements in JUnit 5, particularly in the latest version, JUnit 5.4, make it a highly recommended upgrade for automated testing. Comprehensive resources are provided by the JUnit team, including a user guide, Javadoc, and a GitHub repository with source code and an issue tracker, to assist users in fully exploiting the capabilities of JUnit 5. The team's commitment to continual improvement, supported by sponsors such as IntelliJ IDEA, Micromata, and Code Intelligence, ensures that JUnit 5 remains a leading Java testing framework
3. Migrating from JUnit 4 to JUnit 5: A Step-by-Step Guide
The process of migrating from JUnit 4 to JUnit 5, although a systematic task, can be approached with a step-by-step guide. The first stage involves updating project dependencies to include the JUnit 5 libraries, equipping your project with the tools necessary to support the functionalities that JUnit 5 offers. It's important to note that specific instructions on how to update these project dependencies can be found in the official JUnit documentation or relevant online resources.
The next step involves replacing JUnit 4 annotations with their JUnit 5 counterparts. For example, JUnit 4's @Before
annotation transitions to @BeforeEach
in JUnit 5, and @After
in JUnit 4 becomes @AfterEach
in JUnit 5. The specifics of replacing these annotations are beyond the scope of this context, but can be readily found in the JUnit documentation or related resources.
After the annotation substitution, it's essential to modify the assertions and assumptions to be compatible with the new JUnit 5 APIs. This involves using the Assertions
and Assumptions
classes in JUnit 5, which provide a suite of static methods for performing various checks and validations in your unit tests. For instance, you can utilize the assertEquals
method to verify if two values are equal, or the assertTrue
method to validate if a condition is true. Similarly, the Assumptions
class can be used to make assumptions before executing a test. If the assumption fails, the test will be skipped.
The concluding stage of the migration process requires replacing the test runner with the JUnit Platform. This platform can execute both JUnit 4 and JUnit 5 tests, ensuring a smooth transition between the two frameworks. The JUnit Platform also provides enhanced test execution and reporting capabilities, and allows for the integration of other testing frameworks.
While JUnit 5 offers backwards compatibility with JUnit 4, allowing existing JUnit 4 tests to run unaltered, the specifics of this compatibility are not within the scope of this context. This backwards compatibility can be particularly useful during the transition period.
The migration from JUnit 4 to JUnit 5 isn't merely a transition to a new version. It also offers an opportunity to leverage JUnit 5's enhanced features, such as improved test grouping and parallel test execution. These features significantly optimize the testing process, making it more efficient and effective. However, migrating from JUnit 4 to JUnit 5 can present challenges, such as the need to understand the new APIs and potentially rewrite existing assertions. To overcome these challenges, understanding the changes introduced by JUnit 5 and gradually updating your test code can be beneficial. Through a proper understanding and gradual migration, the challenges of transitioning to JUnit 5 can be effectively managed
4. Implementing Robust and Flexible Testing Frameworks with JUnit 5
JUnit 5 introduces numerous capabilities that enhance the creation of robust and flexible testing frameworks.
Build robust and flexible testing frameworks with JUnit 5 and take your testing to the next level!
One of the key features is the extension model, allowing developers to create custom extensions that modify test execution. This is vital in implementing features like test lifecycle callbacks, parameter resolution, and conditional test execution.
To implement test lifecycle callbacks in JUnit 5, the @BeforeEach
and @AfterEach
annotations are used.
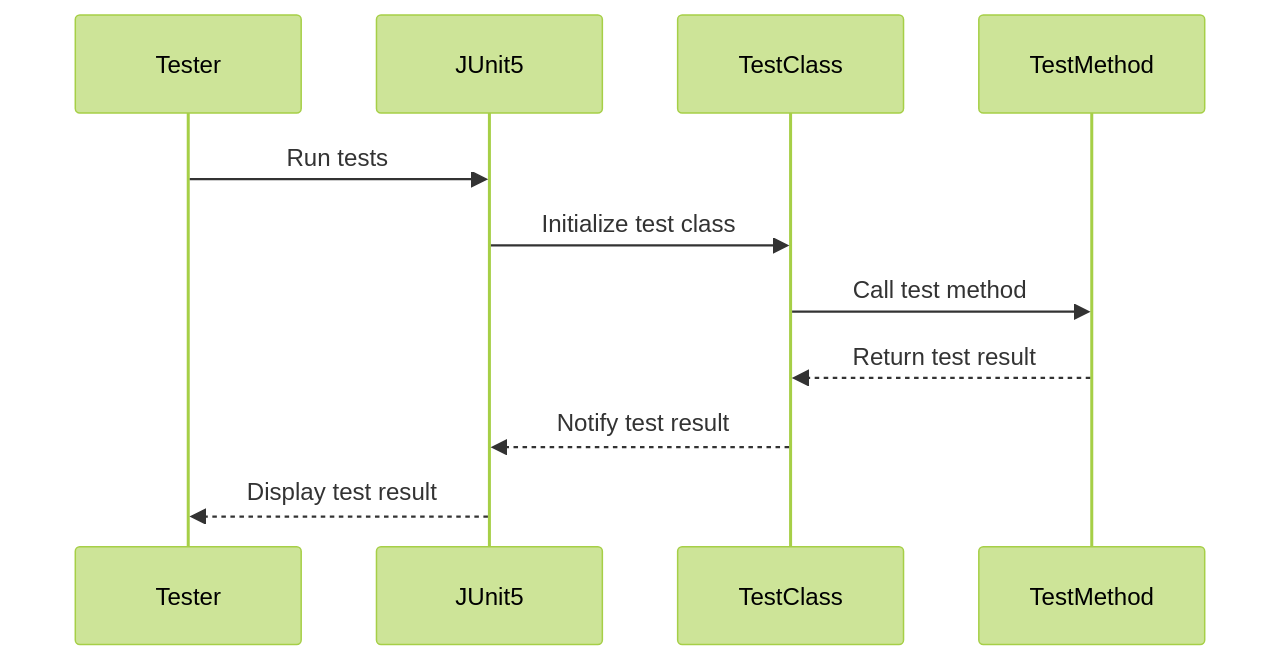
The @BeforeEach
annotation denotes a method that should be executed before each test, while the @AfterEach
annotation is used for a method that should be executed after each test. These annotations help maintain a clean and consistent state for each test method.
JUnit 5 also enhances its parameter resolution through custom extensions. By implementing the ParameterResolver
interface, developers can define custom logic for resolving parameters in test methods. To use a custom parameter resolver, the test class or test method is annotated with @ExtendWith
, specifying the custom extension class. The custom extension class should then implement the ParameterResolver
interface and override the supportsParameter()
and resolveParameter()
methods.
JUnit 5 also supports parameterized and dynamic tests, enhancing test coverage. Parameterized tests allow the execution of the same test with different input values, useful when testing a function against multiple data sets without writing separate test cases for each data set. However, the context does not provide direct examples of parameterized tests.
Dynamic tests are generated at runtime based on specific conditions, invaluable when testing functionalities that require varying setup or have a wide range of input conditions. To generate dynamic tests in JUnit 5, the @TestFactory
annotation is used to define a factory method that dynamically generates and returns a collection of test cases. However, the context does not provide specific examples of JUnit 5 dynamic tests based on conditions.
The tutorial on JUnit 5 is divided into 18 insightful chapters, covering a broad range of topics, including setup, writing tests, test lifecycle methods, test run order, assumptions, disabling tests, repeating tests, tags, assertions, running tests, and more. It also delves into advanced concepts such as the use of the Hamcrest library for assertions and migrating from JUnit 4 to JUnit 5.
Lastly, JUnit 5 heavily relies on annotations, indicating how methods, classes, and variables should be treated by the JUnit testing environment. This makes the framework highly intuitive and easy to use, even for developers new to automated testing. With the thorough guidance provided in the tutorial, developers can quickly get up to speed with JUnit 5's features and start building more robust and flexible testing frameworks
5. Strategies for Managing Workload and Balancing Deadlines in JUnit 5 Testing
JUnit 5 testing can become an efficient process with strategic test planning and prioritization. This involves identifying the critical segments of the application that need to be tested first. The functionalities of parameterized and dynamic tests in JUnit 5 can be utilized to ensure a broad test coverage with less effort. Additionally, the support for test interfaces and default methods in JUnit 5 can be tapped into to promote code reuse and minimize duplication.
Incorporating Machinet library into your project's dependencies can further streamline the process. With Machinet, workload management becomes easier through the use of annotations such as @Test
, @BeforeAll
, @BeforeEach
, @AfterEach
, and @AfterAll
in your test methods. Moreover, Machinet's assertions can be used to validate the expected behavior of your code. These assertions can be added within your test methods using methods like assertEquals
, assertTrue
, and others provided by JUnit 5.
Another significant aspect of Machinet is its support for mocking and stubbing dependencies using libraries like Mockito. This feature is particularly helpful for isolating the code under test and creating controlled test scenarios.
JUnit 5.4, the latest version of this widely used testing library for Java, brings forth a plethora of new features. It supports lambda functions and test method parameter injection, making it a more modern alternative to JUnit 4. The inclusion of dependencies has been simplified in JUnit 5.4, requiring only the single JUnit Jupiter dependency. It also introduces support for temporary directories in tests, thus easing the creation and cleanup of temporary files. Furthermore, parameterized tests now accept null and empty values as input. The display name of tests can be generated dynamically based on the nested class or method name, and a new test method order annotation has been introduced that facilitates the specification of the test method execution order.
Parallel test execution is another noteworthy feature of JUnit 5 that can save significant time by running tests concurrently. To enable this feature, dependencies need to be added and the project configured appropriately. A file named "junit-platform.properties" should be created in the test resources folder, and a line enabling parallel test execution should be added to it. Test classes should be annotated with the "@ParallelTest" annotation to indicate that they should be run in parallel. While it does not solve all time-consuming problems, parallel test execution is a step in the right direction, especially for larger projects with extensive test suites.
The use of the Machinet AI plugin can be a game-changer in the automation of the testing process. It integrates with the Machinet platform and offers features such as automated test case generation, test execution, and result analysis. By using the Machinet AI plugin, the manual effort required for testing can be significantly reduced, thereby improving the efficiency and accuracy of the testing process.
The documentation for JUnit 5 is comprehensive and offers illustrative code examples, making it easier for developers to understand and implement the features. Given the myriad of new features it offers, upgrading to JUnit 5 is highly recommended. And, to get the maximum out of JUnit 5 testing, the use of Machinet library and its AI plugin can be a valuable addition to your testing toolkit
6. Addressing Common Challenges in Automated Testing with JUnit 5
JUnit 5.4, the latest iteration of the renowned Java testing library, is packed with features tailored to solve common problems in automated testing. It's been updated to include support for lambda expressions and test method parameter injection, making the testing process more streamlined.
A key strength of JUnit 5.4 is its capability for conditional test execution. It lets tests be enabled or disabled depending on specific conditions like operating system, JVM version, or environment variables. This proves particularly beneficial when dealing with tests that apply only in certain scenarios.
JUnit 5.4 simplifies dependency management by including just the single junit-jupiter dependency. This eradicates the need for multiple dependencies, which makes the setup process quicker and more efficient.
Another substantial feature in JUnit 5.4 is the support for temporary directories during testing. This feature facilitates the creation and cleanup of temporary files, enabling better resource management during test execution.
JUnit 5.4 also introduces support for parameterized tests with null and empty values. This addition offers more flexibility in crafting comprehensive tests, ensuring that software can deal with various input scenarios.
The enhanced exception handling in JUnit 5.4 provides more accurate control over anticipated exceptions, thereby improving the ability to test error conditions. Notably, the assertThrows
method provided by the framework can be used to assert that a particular exception is thrown by a piece of code under test. By specifying the expected exception type in the assertThrows
method, tests will pass only if the expected exception is thrown, giving precise control over handling and validating expected exceptions.
Moreover, smarter display name generation for test methods helps keep tests organized and easily identifiable.
Upgrading to JUnit 5.4 is strongly advised due to the array of enhancements it brings. The documentation for JUnit 5 is thorough and includes illustrative code examples, proving invaluable for understanding and implementing the new features.
Lastly, JUnit 5.4 introduces a new feature for test method order, allowing for the execution of test methods in a specific sequence. This feature can be crucial in managing complex testing scenarios where the order of test execution is significant.
In essence, JUnit 5.4 is a major upgrade that provides a multitude of features designed to simplify and enhance the automated testing process
7. Case Study: Successful Implementation of JUnit 5 in a Real-World Project
JUnit 5's successful application in a project managed by Machinet, a renowned tech company, serves as a practical illustration of its extensive capabilities. The project, characterized by intricate complexities and ever-changing requirements, was an ideal candidate for JUnit 5's implementation.
The transition from JUnit 4 to JUnit 5 was the initial step in the project's evolution. This migration was not merely a transfer of tests but an opportunity to leverage the advanced features that JUnit 5 offers. The complexity of the migration process was eased by following a structured approach:
- Updating the JUnit dependency in the project's build file to the latest JUnit 5 version
- Replacing the JUnit 4 test methods and lifecycle methods annotations with the equivalent JUnit 5 annotations. For instance,
@Test
was replaced with@org.junit.jupiter.api.Test
- Updating the assertions to the new JUnit Jupiter API. For instance,
assertEquals()
was replaced withAssertions.assertEquals()
- Updating the test runner configuration to use the JUnit Platform test engine for executing JUnit 5 tests
- Running the tests to ensure they are functioning correctly with JUnit 5 and addressing any compilation errors or failures.
In Machinet's project, the team made extensive use of JUnit 5's parameterized tests, enabling them to significantly increase their test coverage. This feature allowed them to execute a single test method multiple times with varying data sets, effectively broadening the scope of their testing process.
Moreover, JUnit 5's support for custom extensions proved invaluable for this project. These extensions catered to specific testing requirements unique to the project, thereby streamlining the testing process. Custom extensions were either created by implementing the Extension interface provided by JUnit 5 or by utilizing existing extensions provided by JUnit 5 or third-party libraries.
Another noteworthy feature utilized was the conditional test execution. This feature empowered the team to manage tests based on the project's fluctuating requirements, enhancing their control over the testing process and addressing the project's dynamic nature effectively.
The result of implementing these steps was an improved testing process. The team was able to increase test coverage, reduce testing time, and ultimately, deliver a high-quality application on schedule. This successful implementation stands as a testament to JUnit 5's capabilities in managing complex and demanding projects.
For developers wishing to implement JUnit 5, the JUnit 5 repository on GitHub serves as a comprehensive resource, providing a user guide, Javadoc, source code, and different versions and releases of the framework. The repository's significant number of stars, forks, and contributors underscore the popularity and community support for this framework, as evinced by the successful implementation of JUnit 5 in the Machinet project
Conclusion
In conclusion, JUnit 5 brings a wealth of new features and enhancements that make it a powerful tool for automated testing in Java. With its modular architecture and support for Java 8 and above, JUnit 5 offers developers increased flexibility, customization, and improved test readability. The migration from JUnit 4 to JUnit 5 is highly recommended due to the multitude of improvements it offers, such as lambda support, test method parameter injection, and the ability to generate dynamic test display names. Implementing robust and flexible testing frameworks is made easier with JUnit 5's extension model, which allows for custom extensions that modify test execution. The comprehensive documentation and resources provided by the JUnit team further assist developers in harnessing the full potential of JUnit 5 for their testing needs.
In broader terms, the advantages of using JUnit 5 extend beyond individual projects. The emphasis on modern Java versions and support for customization through custom annotations and assertions enable developers to create more powerful and adaptable unit tests. Additionally, by streamlining the testing process with strategic planning, prioritization, and the use of tools like Machinet's AI-assisted coding and automated unit test generation, developers can boost their productivity and improve the efficiency and accuracy of their testing efforts. Embracing JUnit 5's advancements not only enhances individual projects but also contributes to the overall improvement of software quality in the industry.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.