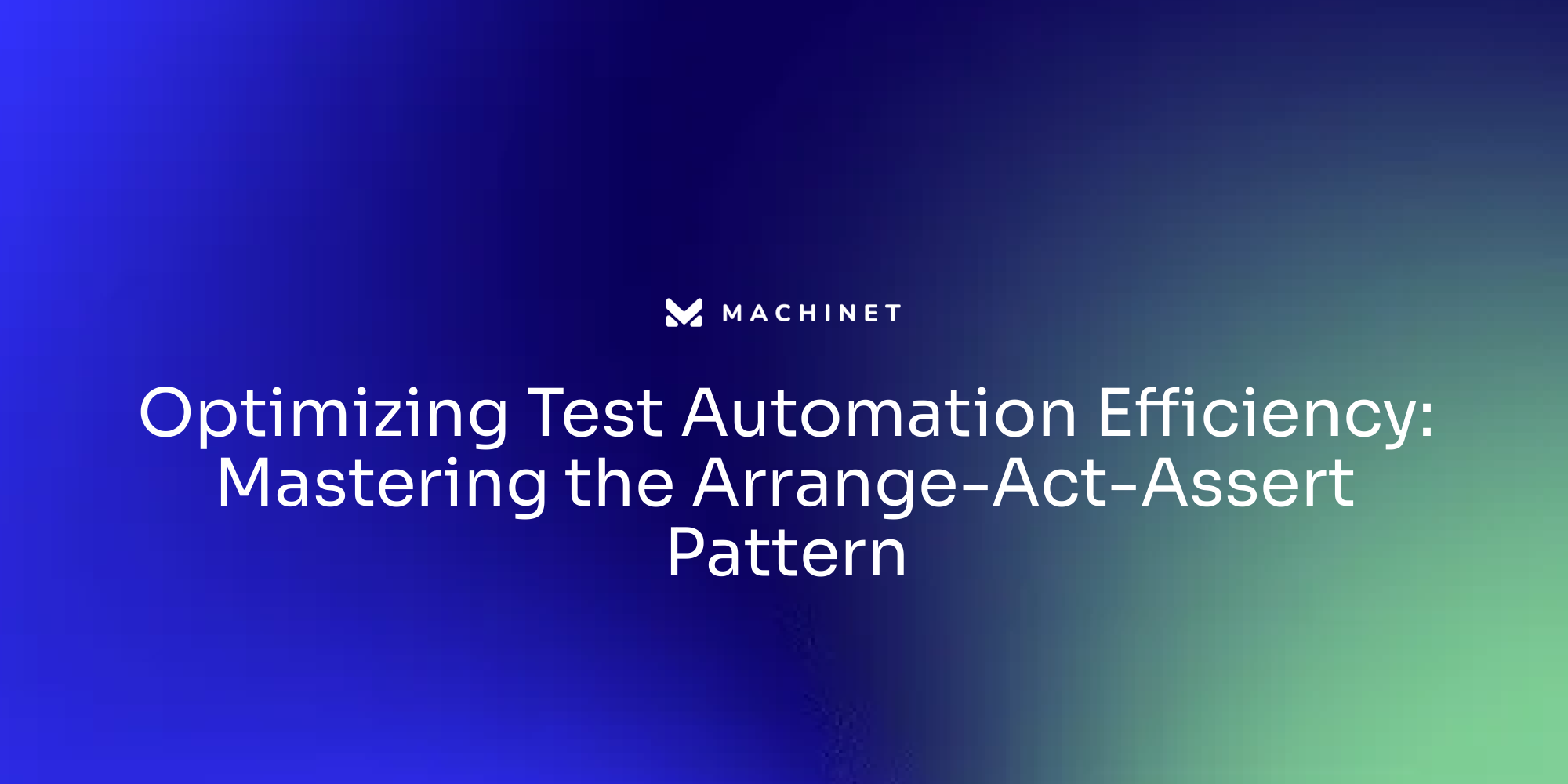
Table of Contents
- Understanding the Arrange-Act-Assert (AAA) Pattern
- Implementing the AAA Pattern in Unit Testing: A Comprehensive Guide
- Best Practices for Unit Testing with AAA Pattern: Ensuring Optimal Efficiency
- Dealing with Technical Debt and Legacy Code: Strategies for Effective Refactoring
- Utilizing Test Explorer for Efficient Unit Testing: An Overview
- Establishing Robust and Flexible Testing Frameworks to Handle Evolving Project Needs
- Workload Management and Deadline Balancing in Test Automation: Key Considerations
- Bridging the Gap between Development and Testing Teams: The Role of Communication in Test Automation Efficiency
Introduction
The Arrange-Act-Assert (AAA) pattern is a robust methodology employed for structuring unit tests. It provides a systematic lens through which software functionality can be validated. The pattern is broken down into three distinct phases: Arrange, Act, and Assert.
In this article, we will explore the importance and benefits of implementing the AAA pattern in unit testing. We will delve into each phase of the pattern and discuss how it contributes to the effectiveness and efficiency of the testing process. Additionally, we will examine real-world examples and best practices for utilizing the AAA pattern in various testing scenarios. By understanding and implementing the principles of the AAA pattern, developers can enhance the quality and reliability of their software products
1. Understanding the Arrange-Act-Assert (AAA) Pattern
The Arrange-Act-Assert (AAA) pattern is a robust methodology employed for structuring unit tests. It provides a systematic lens through which software functionality can be validated. The pattern is broken down into three distinct phases: Arrange, Act, and Assert.
The 'Arrange' phase is the precursor to the test, setting the stage for what's to come. This phase encompasses the preparation of the test environment, including the creation of objects, defining data values, and setting up any prerequisites or inputs that the test may require. This preparatory stage ensures that all necessary conditions are in place, allowing the test to proceed without hitches.
Following the 'Arrange' phase, comes the 'Act' phase. The 'Act' phase is the execution stage, where the functionality under test is performed. This could be a method call, a process initiation, or any other action that is being tested. This phase is pivotal as it is where the operation of interest takes place.
The final phase is the 'Assert' phase, which is the verification stage. This is where we confirm if the operation performed during the 'Act' phase behaved as expected. This could involve checking the return value of a method, verifying the state of an object, or any other validation that confirms the operation has behaved as expected.
Adhering to these three cornerstones of effective unit testing ensures a systematic and comprehensive approach to validating software functionality. This methodology is not just a theoretical framework but a practical tool that has demonstrated its value in real-world software development scenarios.
However, it's essential to note that the AAA pattern, while powerful, has its nuances. For example, testing for exceptions – a vital aspect of comprehensive unit testing – can pose challenges to the AAA pattern. In such cases, strategies like employing the Assert.Throws
method provided by the xUnit framework or using FluentAssertions as an alternative to built-in assertions can be beneficial. These tools can streamline the testing of exceptions, making it easier to adhere to the AAA pattern even in these intricate scenarios.
Additionally, using local functions or actions to represent the operation expected to throw an exception can help keep your tests clean and aligned with the AAA pattern. These strategies can enhance the readability and maintainability of your tests, improving the overall effectiveness of your unit testing process.
One way to bolster test coverage with the AAA pattern is to ensure all relevant scenarios and edge cases are considered in your tests. This means identifying various input combinations and confirming the expected outputs are correctly asserted. Tools and frameworks providing code coverage metrics can also be utilized to pinpoint areas of your code that aren't adequately covered by tests. By leveraging these techniques, you can bolster your test coverage, ensuring your tests are comprehensive and effective.
In essence, the AAA pattern extends beyond a mere set of guidelines. It is a practical tool that can assist in writing effective unit tests, ensuring your software behaves as expected under a variety of conditions. By understanding and implementing the principles of the AAA pattern, you can augment the quality and reliability of your software products
2. Implementing the AAA Pattern in Unit Testing: A Comprehensive Guide
The AAA (Arrange-Act-Assert) pattern holds a prominent position in unit testing, offering a systematic and well-defined structure for verifying software functionality. The pattern is divided into three distinct phases: Arrange, Act, and Assert, each with its own unique role and purpose.
The Arrange phase is the initial step, serving as the foundation for the test. It involves setting up the test environment, which could include defining variables, initializing objects, or setting up any other necessary preconditions. This phase ensures that the stage is appropriately set for the test to be executed.
Following the Arrange phase comes the Act phase. This phase involves executing the method or function that is being tested. The execution could be a method call with specific parameters or triggering a specific event. The Act phase plays a critical role as it directly interacts with the system under test, leading to the production of results that will be assessed in the subsequent phase.
The Assert phase brings the test to a conclusion by validating the results obtained from the Act phase. The validation process could involve checking a method's return value, verifying the state of an object, or confirming the occurrence of an exception under certain conditions. Essentially, the Assert phase forms the crux of the test. It is where the system's behavior is evaluated against the expected outcome.
An integral part of the AAA pattern is the clear demarcation of each phase. This separation lends a well-defined structure to the test, enhancing its readability and maintainability. For instance, it is advisable to test for a variety of failure conditions, often referred to as "sad paths," alongside the expected successful condition, or the "happy path." This comprehensive approach ensures that your method is robust and can gracefully handle a wide array of inputs and scenarios.
Testing for exceptions forms a crucial part of the Assert phase. This is particularly important if the method can throw custom domain exceptions or if guard clauses are used to authenticate inputs. Libraries such as xUnit and NUnit offer methods like Assert.Throws<T>
to test for anticipated exceptions, aligning well with the principles of the AAA pattern.
FluentAssertions serves as an alternative to built-in assertions, providing a more fluid syntax for making assertions. It can be used in combination with actions to make the tests for exceptions more readable and clear.
In conclusion, the AAA pattern offers a powerful framework for structuring unit tests, ensuring each test is comprehensive, readable, and maintainable. By incorporating practices such as testing for exceptions and utilizing tools like FluentAssertions, developers can create cleaner and more effective unit tests
3. Best Practices for Unit Testing with AAA Pattern: Ensuring Optimal Efficiency
Unit testing, especially when using the AAA (Arrange-Act-Assert) pattern, is a critical part of software development, ensuring the robustness and reliability of intricate systems. To achieve optimal efficiency, it requires a strategic approach. Here are some guiding principles and best practices for this process.
A cardinal rule is to ensure each test's independence to prevent unexpected interferences that could emerge from dependencies between tests, guaranteeing accurate results.
Keeping the tests concise and focused on a single concept is another vital practice. It enhances the tests' readability and maintainability, simplifying the process of identifying and resolving any emerging issues.
Adherence to naming conventions is also crucial in unit testing. Tests should bear meaningful names that lucidly convey the test's purpose and what it aims to check. A common naming convention includes the format "test_[MethodName][Scenario]" or "[MethodName][Scenario]should[ExpectedOutcome]". Such a clear and descriptive naming system can significantly simplify the process of identifying and addressing issues.
Another best practice is to steer clear of logic in your tests. Logic can introduce a layer of complexity, making the tests harder to comprehend and maintain. An effective approach to avoid including logic in unit tests is to use test doubles, such as mocks or stubs, to isolate the code under test. These test doubles can simulate the behavior of external dependencies or other components, enabling the unit test to focus on the specific functionality under test.
Additionally, considering edge cases and invalid inputs while crafting your tests is important. This ensures that your code can handle all possible scenarios, enhancing its resilience and reliability.
Custom test data builders and asserts can further streamline the unit testing process. For instance, custom test data builders can set up interconnected objects in tests in a loan application evaluation service, while custom asserts can encapsulate complex checks and provide meaningful error messages.
Unit tests should be treated with the same level of care and attention as production code. This approach aids in managing technical debt and maintaining the quality of the software over time.
Lastly, unit testing should not only focus on testing the expected outcomes (happy path) but also on testing the failure conditions (sad paths). This includes testing for expected exceptions, particularly when a method may throw custom domain exceptions. Libraries like FluentAssertions can be used for assertions in testing, improving the readability of the test code.
In essence, adhering to these best practices and principles can significantly enhance your unit testing process's efficiency, leading to the production of higher-quality software products
4. Dealing with Technical Debt and Legacy Code: Strategies for Effective Refactoring
Technical debt, the metaphorical representation of the extra effort needed to restore the code back to a maintainable state, is a widespread concern in the software development industry. It's not confined to the codebase alone but also extends to the whole system and its architecture. This issue, often a result of quick and sub-optimal decisions, can be aggravated by external code in the form of libraries and frameworks.
Over time, our understanding of technical debt has evolved. It now encompasses various factors such as time constraints, complexity, inadequate testing, and postponed cleanups and upgrades. For long-term projects, the cost of 'paying off' the debt through code refactoring can be significant. Nonetheless, managing technical debt is critical. Failure to do so can lead to project standstill, challenges in implementing necessary changes, difficulties in upgrading dependencies, and problems with scaling and deployment.
Refactoring, a key strategy for managing technical debt, involves cleaning the code, enhancing its readability, and breaking it down into smaller parts. This process is often gradual and unfolds over time. In conjunction with this, applying the Arrange-Act-Assert (AAA) pattern in testing can be extremely useful in pinpointing parts of the code that need improvement. However, it's worth noting that refactoring requires time and, in some cases, the cost needs to be justified to stakeholders.
Legacy code management is another vital aspect of handling technical debt. Legacy code refers to code used for a particular software that has accrued substantial technical debt. Although working with legacy code can be intimidating for developers, continuous learning and training can help surmount this obstacle. It's crucial to prioritize parts of the code most prone to errors or most critical to the application's functionality.
Incorporating tasks to manage technical debt into the development process can help curb its growth. Product owners can also contribute to reducing technical debt by focusing on a maximum viable product, eliminating unnecessary features, and promoting clean code and testing.
To improve code quality in legacy applications, it's important to conduct thorough code reviews and identify possible code smells or anti-patterns. Automated testing is crucial for ensuring code quality. By creating unit tests and integration tests, developers can verify the correctness and stability of the existing codebase. The SOLID principles and other design patterns can greatly enhance the maintainability and extensibility of legacy applications by breaking down monolithic code into smaller, more modular components. Documenting the codebase and maintaining proper documentation can aid in understanding the existing code and facilitate future modifications. Continuous refactoring of the codebase and addressing technical debt is vital for improving code quality in legacy applications.
In essence, managing technical debt is a multifaceted process requiring a proactive and comprehensive approach. By employing strategies such as incremental refactoring, applying the AAA pattern in testing, and fostering a culture of continuous learning, the impact of technical debt and legacy code on software development projects can be mitigated
5. Utilizing Test Explorer for Efficient Unit Testing: An Overview
The Test Explorer remains a fundamental element in effective unit testing, providing an accessible platform for executing tests and interpreting their outcomes. It is compatible with a wide variety of testing frameworks, and its capabilities such as test categorization, filtering, and sorting simplify the management and organization of tests.
The true potential of the Test Explorer is brought to light when it is used in conjunction with the Arrange-Act-Assert (AAA) pattern. This combination results in a markedly more streamlined unit testing process.
Further complexity and efficiency can be introduced through the integration of exploratory testing, also known as XT. This testing technique, first presented by Microsoft in Microsoft Test Manager with Visual Studio 2010, improves the unit testing process by enabling the identification of bugs through an exploration of the application's functionality, as opposed to adhering strictly to predefined test cases.
Over time, exploratory testing has undergone numerous enhancements and is now incorporated into Visual Studio as the Test Feedback Extension, available for Visual Studio Team Services (VSTS) or on-premises Visual Studio Team Foundation Server (TFS). The extension simplifies the initiation of an exploratory session, the documentation of actions, the inclusion of comments and notes, and the generation of work items such as test cases, tasks, or bugs.
Additionally, the Test Feedback Extension provides features such as screen recording, the capture of screenshots, and the export of HTML reports. These features, along with the extension's compatibility with both Chrome and Mozilla Firefox browsers, make it a crucial component of the unit testing process.
The practical application of these tools and methodologies is evidenced in a variety of case studies. For example, exploratory testing in Visual Studio has demonstrated its effectiveness in uncovering bugs in applications, with business analysts who have crafted the application's requirements frequently being the best candidates to conduct this type of testing. The Test Feedback Extension, which can be installed as an extension in Visual Studio, offers capabilities like screen recording, the addition of screenshots, and the creation of bugs, while also producing an HTML report with comments, screenshots, and bugs generated during the testing.
In conclusion, the integration of the Test Explorer, the AAA pattern, and exploratory testing can significantly boost the efficiency of the unit testing process. By utilizing these tools and methodologies, software engineers can facilitate the delivery of superior quality software products, effectively manage technical debt and legacy code, and adapt to ever-evolving requirements
6. Establishing Robust and Flexible Testing Frameworks to Handle Evolving Project Needs
Developing a robust and adaptable testing framework is a pivotal step in handling the dynamic demands of a software project. To achieve this, a framework must support a wide variety of tests, be easy to maintain and adapt, and have the flexibility to accommodate changes in project requirements. The Arrange-Act-Assert (AAA) pattern contributes significantly to this goal, offering a consistent structure that simplifies the understanding and usage of the framework.
Take the example of "MySocialConnect", a video conferencing application accessible on various platforms. The challenge here is to ensure tests are defined and business logic is executed only once, irrespective of the platform. This calls for a testing framework that can simulate multi-user scenarios and support testing across numerous modern browsers and devices.
Teswiz, an open-source automation framework, is a strong example of such a framework. It integrates tools like Cucumber JVM, Appium, Selenium WebDriver, and ReportPortal.io. Teswiz also uses Applitools Visual AI for visual testing, strengthening the framework's robustness.
Test generation is another key aspect of a robust testing framework. Tools like Machinet can greatly assist in generating comprehensive unit tests. Moreover, Teswiz supports parallel and distributed execution of tests for quicker feedback and adheres to the WCAG 2.0/2.1 guidelines for accessibility, making it a reliable choice for a testing framework.
Providing detailed reports for test execution is another essential feature of a robust testing framework. ReportPortal.io is a recommended tool for this purpose. Teswiz can be operated on local machines or in a CI environment, and it supports running the entire suite or a specific subset of tests on demand without any code changes.
To create robust and flexible testing frameworks, certain best practices can be followed. Using a unit testing framework that supports test isolation, mocking, and assertions can lead to the creation of modular and independent tests that are easy to maintain and extend. Implementing a continuous integration and delivery pipeline can automate the testing process, ensuring regular and consistent test execution. Adopting a test-driven development (TDD) approach, where tests are written before the code, can result in more testable and maintainable code. Regular review and refactoring of the test code can further improve the quality and flexibility of the testing framework.
In essence, creating a robust and adaptable testing framework is crucial to effectively managing the evolving needs of a project. The AAA pattern plays a significant part in this process, and the integration of tools like Machinet and frameworks like Teswiz can greatly enhance the overall robustness and flexibility of the testing framework
7. Workload Management and Deadline Balancing in Test Automation: Key Considerations
In the world of test automation, managing workload and aligning with project deadlines is a critical skill. The key task lies in prioritizing tests based on the importance of the functionality they inspect. This equilibrium between thorough testing and adhering to project timelines is an essential factor of the process. Automated testing tools are crucial in workload management, as they perform tests simultaneously or according to a pre-set timetable, thereby freeing developers to concentrate on other vital tasks. The Arrange-Act-Assert (AAA) pattern proves to be a powerful tool in managing workload, as it streamlines the process of creating and maintaining tests, therefore reducing the time and effort invested in testing.
Consider the story of Pedro, a seasoned tester, which exemplifies the challenges of workload management. Even with his technical expertise, Pedro often finds himself buried in administrative tasks and compliance-related activities, which take up a considerable portion of his productive time. Setting up and troubleshooting test environments is a time-consuming process, which distracts him from the actual testing work. The task of reproducing bugs and documenting them further eats into his testing time. Misunderstandings among Pedro's managers about his time allocation for testing lead to unrealistic expectations, adding to his stress. A graphical representation of Pedro's time allocation underscores the imbalance between administrative tasks and actual testing work. By reducing the time spent on administrative tasks and improving the bug investigation and reporting processes, Pedro can significantly increase his testing productivity.
This situation highlights the 'lumping problem', a frequent misconception that all testing-related activities are grouped into a single category, leading to unrealistic expectations from testers. To counteract this, testers should aim to improve the transparency of their work and time allocation to their managers to dispel misconceptions and foster understanding.
To decrease testing time and effort in test automation, it's crucial to adopt the Arrange-Act-Assert pattern. This pattern aids in organizing the test code and enhancing test maintainability. In the Arrange phase, necessary preconditions for the test are established, such as initializing objects, setting up test data, and configuring the test environment. This phase ensures the system is in the desired state before the test begins. In the Act phase, the action or behavior being tested is performed. This could involve invoking methods, interacting with user interfaces, or simulating user interactions. This phase triggers the functionality that needs to be tested. In the Assert phase, the expected outcomes of the test are verified. This involves comparing the actual results with the expected results and asserting whether the test passed or failed. This phase helps in ensuring that the system behaves as expected. By adhering to the AAA pattern in test automation, testing time and effort can be reduced, providing a structured approach to writing tests. This makes it easier to understand the test's purpose and flow and aids in identifying and rectifying issues swiftly.
The case of 2i Technology, a notable software quality assurance testing company, demonstrates how automation can be used to bypass human error, operational delays, and inconsistent testing. The company has distinguished itself by offering test data generation services and specializing in non-functional testing to ensure digital systems adhere to high standards. The company's accolades, including prestigious awards such as Leader of the Year at the European Software Testing Awards in 2023 and SME Employer of the Year at the Scottish Women in Tech Awards in 2023, bear witness to its expertise in the field. By following the best practices of successful companies like 2i Technology, testers can enhance their testing productivity and effectively manage their workload
8. Bridging the Gap between Development and Testing Teams: The Role of Communication in Test Automation Efficiency
Harmonizing the dynamics between development and testing teams is crucial in the software production lifecycle. However, the shift from conventional engineering methods to agile methodologies can create conflict due to the divergent nature of their work. Redefining roles around automation and testing, promoting shared objectives and accountability, and ensuring equitable distribution of tasks are key to resolving these issues.
A recurring source of friction is the delayed delivery of code to the Quality Assurance (QA) team, often at the sprint's end. This gives them a limited window to identify and rectify issues before software deployment. To counter this, firms adopting agile methodologies should avoid creating mini waterfalls bi-weekly, which contradicts true agile practices.
Automation can be a game-changer in this scenario. Developers can speed up the testing process and foster trust and understanding with the QA team by creating end-to-end tests that undergo a review process.
Adopting a 'shift-left' testing approach in the release cycle is another way to enhance collaboration. This method involves incorporating QA within each feature team, eliminating the need for a separate QA team. This practice promotes transparency, understanding of acceptance criteria, and early planning stage input, thereby increasing accountability.
Moreover, segmenting larger projects into smaller, manageable code chunks can accelerate the shipping process. Proper documentation can also significantly reduce inefficiencies and potential conflicts between developers and testers.
Communication is the linchpin holding these strategies together. Regular discussions about feature alterations and building relationships within teams are crucial. Platforms like the Bugsnag's Apps Coffee Connection community's monthly meetups provide a platform for these conversations, promoting alignment between developers and testers.
The AAA pattern can also be a potent tool in facilitating communication. It provides a clear and consistent structure for tests, making them easier to comprehend and discuss. This minimizes misunderstandings and ensures everyone is on the same wavelength.
Taking these strategic steps and committing to effective communication can bridge the gap between development and testing teams. One such tool that can aid this process is Machinet, a platform designed specifically for collaborative coding. It offers features such as real-time code editing, version control, and collaborative debugging. It can also automate the generation of test cases, providing a clear and standardized set of instructions for the testing team. This leads to better communication between the teams, as it helps track the status and progress of testing activities, enabling more efficient collaboration.
By leveraging such tools and strategies, development and testing teams can work together more seamlessly, leading to the production of superior software products and a smoother production process
Conclusion
In conclusion, the Arrange-Act-Assert (AAA) pattern is a powerful methodology for structuring unit tests in software development. It provides a systematic approach to validate software functionality by breaking down the testing process into three distinct phases: Arrange, Act, and Assert. The Arrange phase sets up the test environment, the Act phase executes the functionality under test, and the Assert phase verifies the expected outcomes. By adhering to the AAA pattern, developers can create comprehensive and maintainable unit tests that enhance the quality and reliability of their software products.
The importance of implementing the AAA pattern in unit testing cannot be overstated. It offers numerous benefits such as improved test organization, readability, and maintainability. The clear separation of phases allows for better understanding and collaboration among team members. Furthermore, adhering to this pattern enables developers to identify edge cases and failure conditions that may otherwise be overlooked. By leveraging tools like xUnit's Assert.Throws
method or FluentAssertions, developers can effectively handle exceptions within the AAA pattern.
To boost productivity and efficiency in unit testing, developers should embrace best practices such as considering various input combinations, utilizing code coverage metrics, and using local functions or actions for cleaner tests. Additionally, integrating automation tools like Machinet can further streamline the testing process by automating test case generation.
Incorporating the principles of the AAA pattern in unit testing is essential for ensuring robust and reliable software products. By following these best practices and leveraging automation tools, developers can enhance their productivity and deliver high-quality code that meets customer expectations.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.