
Table of Contents
- Understanding the Importance of Unit Testing in Java
- Implementing Effective Test Case Naming Conventions
- Strategies to Avoid Code Redundancy in Unit Tests
- Guidelines for Writing Specific and Simple Unit Tests
- Leveraging the Test-Driven Development (TDD) Approach in Java
- Ensuring Comprehensive Test Coverage: The 80% Rule and Beyond
- Balancing Expected vs Actual Outcomes in Unit Tests
- Using Appropriate Assertions for Reliable Test Results
Introduction
Unit testing is a crucial aspect of software development, allowing developers to examine their code in detail and ensure each component functions as intended. By following best practices and leveraging tools like JUnit, developers can significantly enhance the reliability and maintainability of their code, leading to high-quality software products. In this article, we will explore the importance of unit testing in Java and the benefits it brings to the software development process. We will also discuss strategies for writing effective unit tests, including test case naming conventions, avoiding code redundancy, ensuring comprehensive test coverage, and balancing expected versus actual outcomes in tests. By implementing these strategies, developers can improve the quality and stability of their Java applications.
Unit testing plays a vital role in the software development process, allowing developers to identify bugs early, streamline code refactoring, and simplify integration testing. It serves as a safety net during code maintenance and refactoring, preventing regression and facilitating smooth transitions in the codebase. Additionally, unit tests act as living documentation, providing current information about the software's expected behavior and promoting collaboration among developers, testers, and stakeholders. In this article, we will explore the importance of unit testing in Java and discuss best practices for writing effective unit tests. We will also examine specific strategies for avoiding code redundancy in tests, ensuring comprehensive test coverage, and balancing expected versus actual outcomes. By following these guidelines, developers can create reliable and maintainable software applications
1. Understanding the Importance of Unit Testing in Java
Unit testing in Java plays a crucial role in the software development process
Try Machinet to automate your unit tests and save time!
, providing developers with the tools to examine their code in fine detail, ensuring each software component operates as intended. This approach enables early detection and resolution of bugs, streamlines code refactoring, and simplifies integration testing. By following best practices for Java unit tests, developers can significantly boost the reliability and maintainability of their code, enhancing the final software product's overall quality.
Unit tests act as a vital safety net during code refactoring and maintenance, preventing regression and enabling smooth transitions in the codebase. They also serve as living documentation, providing current information about the software's expected behavior, facilitating collaboration among developers, testers, and stakeholders.
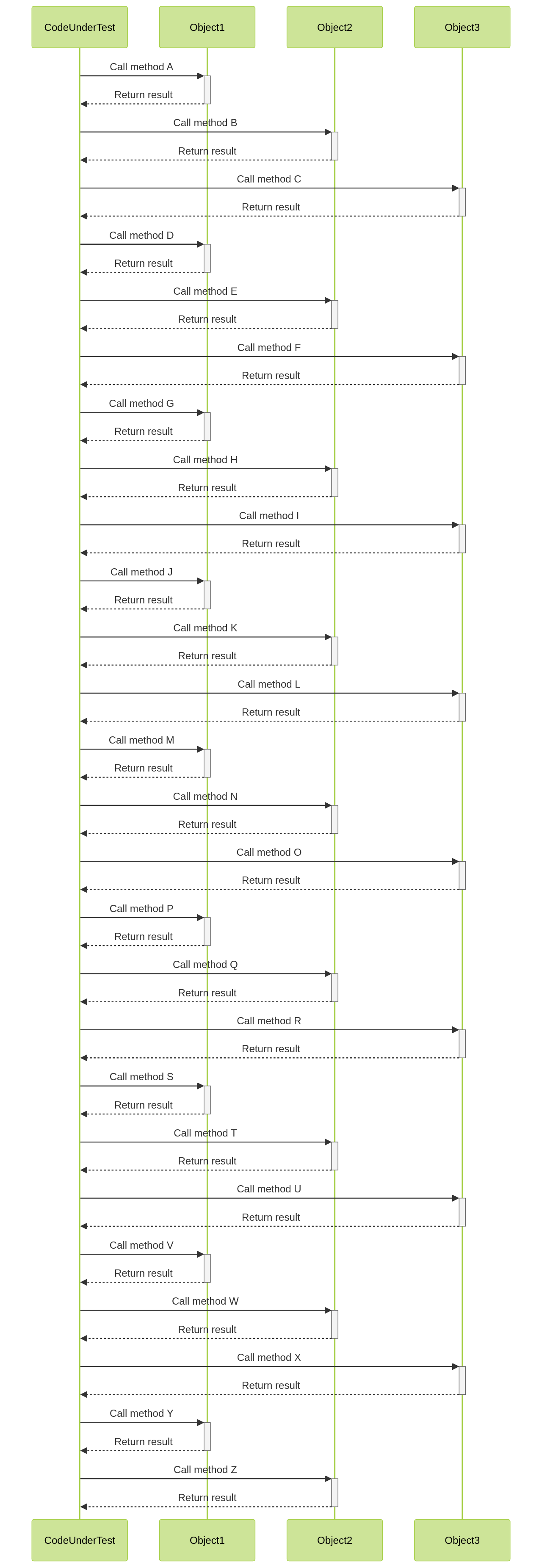
By adhering to a consistent methodology and breaking down test code into manageable parts, developers can create effective tests more rapidly. Utilizing a clear and easy-to-follow naming convention for test methods further enhances this process. For instance, a naming convention that includes the method name under test, given condition, and expected behavior can prove beneficial.
The given-when-then style is vital while writing test cases. The 'given' step establishes a certain condition or creates the objects required for the test. The 'when' step initiates the action being tested, and the 'then' step ensures that the application's state is as expected after the test action. Implementation of these guidelines can simplify the test writing process, reduce bugs, and facilitate code refactoring.
Unit testing also plays an essential role in continuous integration and deployment
Supercharge your CI/CD pipeline with Machinet's automated unit testing!
, contributing to code quality assurance and regulatory compliance. Automated unit testing tools offer benefits such as test frameworks and runners, code coverage analysis, integration with CI/CD, mocking, and test doubles, parameterized testing, test execution reporting, test data management, test prioritization, integration with IDEs, and cross-browser and cross-platform testing.
For instance, generating code coverage reports using tools like Jacoco can quantify progress in unit testing. These tools not only streamline the development process but also enhance code quality, provide rapid feedback loops, and foster a culture of continuous testing and improvement.
One of the commonly used tools for Java unit testing is the JUnit framework. It provides annotations and assertions that make unit testing in Java applications straightforward. Developers can write test cases to verify the correctness of individual units of code using JUnit. The framework offers a set of annotations such as @Test, @Before, and @After, which allow developers to define test methods and set up and tear down test fixtures. JUnit also provides a range of assertion methods such as assertEquals, assertTrue, and assertFalse, which enable developers to assert expected results and verify their code's behavior. Using the JUnit framework, developers can automate test execution and ensure the quality and reliability of their Java applications.
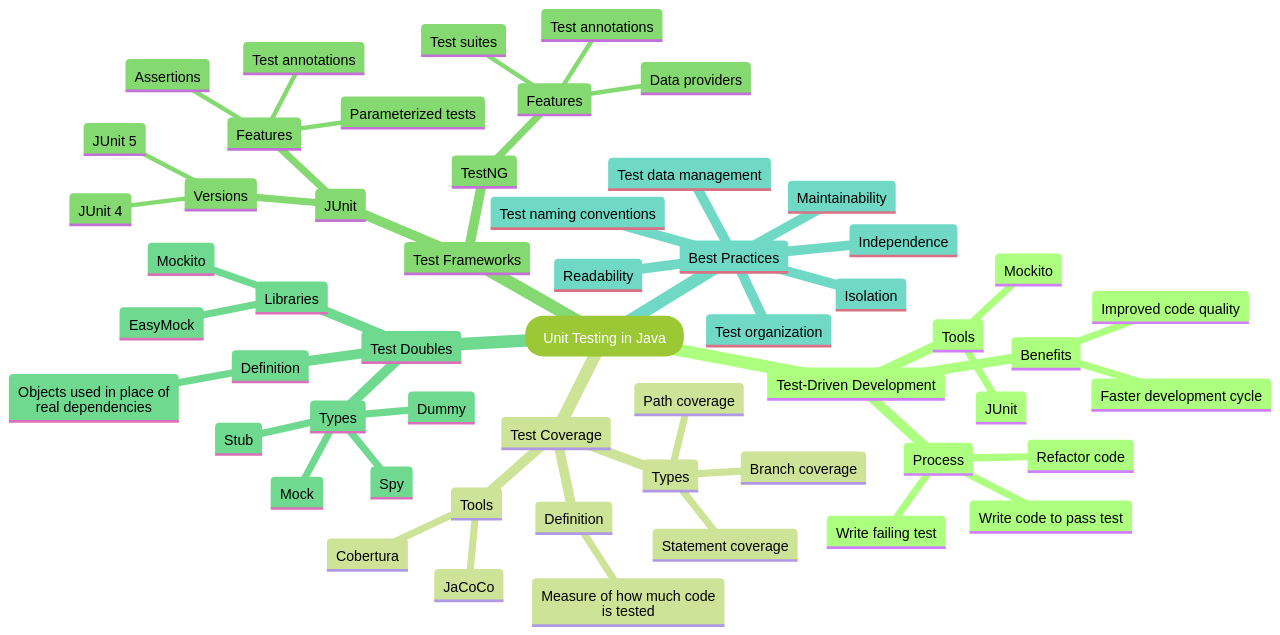
In essence, unit testing is a critical practice for delivering reliable, maintainable software applications of high quality. It's a valuable skill that significantly enhances any software development process
2. Implementing Effective Test Case Naming Conventions
Unit testing, a fundamental aspect of software development, greatly benefits from the implementation of clear and consistent test case naming conventions. These conventions serve as a living documentation, providing vital insights into the specific functionality being tested. In Java, a common strategy includes the usage of the 'test' prefix alongside descriptive method names. For instance, 'testCalculateInterest' effectively communicates the test case's purpose. This method simplifies the understanding of each test case's objective, thereby facilitating debugging and maintenance processes.
Adopting a standardized approach to crafting unit tests not only accelerates the procedure but also enhances its enjoyment. The secret lies in decomposing the test code into manageable, bite-sized tasks. Test methods should adhere to an uncomplicated naming convention and structure, which can be evaluated using tools such as JaCoCo to produce code coverage reports.
For instance, when testing a straightforward customer class, three potential test candidates might be identified: testing the constructor with a null parameter, testing the constructor with a non-null parameter, and testing the getName
method. To maintain clarity and readability, each test should be limited to a few lines of code and verify only a single functionality.
A beneficial practice is to adhere to the given-when-then style in tests. The 'given' step establishes a specific condition, the 'when' step initiates the action under test, and the 'then' step asserts the anticipated behavior. This sequence of steps creates a logical, comprehensible, and consistent flow.
By adhering to these conventions, we create a succinct, easy-to-read living documentation for the project. This practice facilitates test writing, accelerates the process, and makes it more enjoyable, leading to fewer bugs and facilitating code refactoring. It's also worth noting that the ability to write effective tests is a highly marketable skill.
Maintaining consistency is crucial in test organization. It fosters efficiency and confidence in system maintenance. Without consistency, test suites can become confusing and challenging to maintain over time. Tests should be organized to enable developers to easily locate and comprehend them. They should be situated near the production code and grouped based on technical characteristics or domain functionality.
The test class structure should be uniform and consistent, with clear indications of external dependencies, setup, teardown, and helper functions. Test naming is vital for understanding their purpose and functionality. Good names reflect the domain language and provide information about what is being tested. Test names can be structured based on scenarios, functionality, or specifications. The aim is to have expressive and unique names that are still readable.
Failure messages in tests should provide sufficient information to identify the problem. They should be clear and concise, indicating which aspect of the test failed. In essence, organizing and naming tests require attention and consistency to facilitate code handling and ensure maintainability.
When naming unit tests, it is valuable to use descriptive method names. These names clearly express the purpose and behavior of the test case, making it easier for other developers to comprehend the test and identify the causes of failures or issues. A few guidelines to follow when naming your methods include:
- Use clear and concise names: The method name should precisely describe the behavior being tested. Generic names like "testMethod" or "testCase" should be avoided. Instead, aim for specificity and descriptiveness, such as "testCalculateTotalWithDiscount" or "testSortByDateAscending".
- Use action verbs: Begin the method name with an action verb that describes the test's action. For example, "verify", "test", "check", "validate", etc. This helps to clearly indicate that the method is a test case.
- Be specific about the expected outcome: Include the expected outcome or behavior in the method name. This helps to clearly define the purpose of the test. For example, "testAdditionWithPositiveNumbers" or "testSaveUserWithValidData".
- Use camel case: Follow the camel case convention for method names. Start with a lowercase letter and capitalize the first letter of each subsequent word. For example, "testCalculateTotal" or "testGetUserById".
By using descriptive method names in unit testing, the readability, maintainability, and understandability of your tests can be improved. This will make it easier for you and other developers to work with the codebase and identify any issues or failures
3. Strategies to Avoid Code Redundancy in Unit Tests
Unit testing is a crucial aspect of software development, offering a multitude of advantages. These include preventing regression, serving as executable documentation, and fostering good design. The speed and efficiency of unit tests are superior to functional tests, as they can be executed at a button press and do not require a comprehensive understanding of the system.
In the sphere of unit testing, code redundancy can lead to unnecessary complexity and a heightened maintenance burden. However, this can be mitigated through certain techniques, including the creation of test setup methods, parameterized tests, and helper functions.
Test setup methods, identified with @Before in JUnit, allow for shared common setup code across multiple test cases. This results in a more efficient testing process and minimises code redundancy.
Parameterized tests are another effective strategy to combat code duplication. These enable the same test case to be executed with different input values, thereby enhancing the testing process's efficiency. Developers can also leverage reusable test fixtures or test data across multiple unit tests, further reducing code duplication and simplifying test maintenance.
Helper functions are instrumental in encapsulating complex operations or calculations. This not only encourages code reuse but also improves the code's readability, making it simpler for others to comprehend and maintain.
One prevalent error in unit testing, especially among novice unit test writers, is the duplication of logic in asserts. This can potentially introduce bugs and should be avoided. Instead, developers should use known hard-coded values for assertions. This shifts the test's focus to the code's observable behaviour, such as returned values or thrown exceptions.
Testing public methods rather than private ones and avoiding multiple acts in a test are also suggested best practices for writing unit tests. It is crucial to keep tests centred on assignments and method calls, rather than incorporating excessive logic.
Mocking frameworks, such as Mockito, are another powerful tool in unit testing. They allow developers to create mock objects that mimic the behaviour of dependencies in a unit test. This enables developers to isolate the code being tested and concentrate on specific units of functionality.
In conclusion, unit tests are a vital component of software development, providing numerous benefits when properly implemented. Adhering to best practices and avoiding common errors can help ensure the effectiveness and efficiency of unit testing. Moreover, code reuse techniques in unit testing can assist developers in writing more efficient and maintainable tests, ultimately enhancing their code's quality
4. Guidelines for Writing Specific and Simple Unit Tests
Unit testing is a critical component of software development that enables early detection of errors and ensures that the code works as intended. A well-crafted unit test targets a single functionality and is simple, minimizing reliance on external systems or components. This simplicity enhances the test's readability and maintainability.
In the development of unit tests, the AAA (Arrange, Act, Assert) model is commonly utilized. This model involves structuring a test into three distinct phases: setting up the test data, executing the action or method, and verifying the results. This approach, combined with clear and concise test names and descriptions, enhances the communication of the test's purpose and scope.
One of the key attributes of an effective unit test is its speed. Fast unit tests provide immediate feedback, facilitating quick identification and resolution of issues. It's also essential for tests to be deterministic, ensuring that they yield the same output every time they are executed. This reliability and repeatability make the tests more dependable.
In order to isolate the code being tested and create deterministic tests, developers often employ mocking frameworks like Mockito. Mocks imitate the behavior of external dependencies, allowing the code under test to run independently. This isolation simplifies the writing of deterministic tests.
In addition to successful execution, comprehensive unit tests also assess potential failures. This thoroughness ensures that the code can handle both expected and unexpected scenarios. It's also beneficial to integrate unit tests into the development pipeline to prevent issues in production environments.
The practice of Test-Driven Development (TDD) involves writing tests before or during the development process to ensure code testability and coverage. This technique helps to shape the code and align it with the expected outcomes, resulting in robust applications.
As Fotis Adamakis, a leading software development expert, puts it, "Treating unit tests as first-class citizens means giving them the same level of importance as production code." This mindset underscores the significance of unit tests in delivering high-quality software applications
5. Leveraging the Test-Driven Development (TDD) Approach in Java
Test-Driven Development (TDD) is a software development technique that mandates the formulation of tests preceding the actual code development. This methodology obliges developers to contemplate the anticipated functionality of their code beforehand, resulting in meticulously planned and robust software. The TDD process begins with the creation of a test case that initially fails, succeeded by the creation of minimal code to pass the test, and finally, refactoring the code to achieve quality standards. This cycle is continuously repeated for each new feature or functionality, ensuring comprehensive test coverage and superior code quality.
Kent Beck, a renowned figure in the software development field, is credited with the inception of TDD. The core of TDD, often referred to as Classic TDD, is the Red-Green-Refactor cycle. This iterative process commences with the creation of a failing test (red), followed by the development of the minimum code to pass the test (green), and lastly, the refactoring of the code for improved design. This cycle assures that tests not only validate the code but also guide its development, enhancing software stability and robustness, and preventing regressions.
Outside-in TDD, also known as the London School of TDD, is another variant of TDD. This approach commences from the user's perspective and gradually moves inward, focusing on both functionality and user experience. It involves crafting high-level acceptance tests that mimic user interactions and expectations, thereby aiding developers in understanding how the software should function concerning user experience.
Another approach, Acceptance-driven TDD, prioritizes defining acceptance criteria for user stories before code implementation. It involves writing tests based on user stories and implementing code that satisfies these tests, resulting in software functionality directly aligned with user expectations.
TDD is a strategic and practical tool that establishes a solid foundation for security by necessitating comprehensive testing, anticipating edge cases, and fostering a security-conscious development culture. It contributes to code reliability, functionality, and user satisfaction, all fortified by proactive security measures, and helps uncover vulnerabilities before they pose a threat.
The Equifax data breach of 2017, which exposed the personal and financial data of millions due to a failure in patch management, underscores the importance of secure coding practices and regular patch management, principles integral to the TDD methodology.
In essence, TDD is not just a methodology but a culture that aligns the development process with the goal of creating secure and robust software from the outset. It aids in averting regressions, enhancing software stability and robustness, and aligns with agile development methodologies. By incorporating TDD, developers can ensure that they are creating software that not only meets functional requirements but also resonates with user expectations
6. Ensuring Comprehensive Test Coverage: The 80% Rule and Beyond
Testing is a cornerstone of robust software development, with test coverage serving as a key metric. The industry benchmark is often cited as 80%, suggesting that at least 80% of your code should be subjected to testing. However, this doesn't mean that test coverage is the sole determinant of test quality. It's also essential to ensure the comprehensive nature of your tests, covering all primary paths and edge cases in your code.
Take the Go programming language, for example. In its recent version, Go 1.20, support for code coverage at the package level has been introduced using the cover flag of the "go test" command. This is a significant advancement, particularly for larger Go applications that include integration tests. Until now, gathering a coverage profile for these tests was not straightforward. With Go 1.20, developers can now build coverage instrumented programs using "go build -cover", and use these binaries in integration tests to collect coverage profiles.
Consider the "mdtool" markdown processing tool as an illustration. A wrapper script is provided that builds the tool for coverage, then runs the integration test, and finally processes the resulting coverage profiles. These profiles can be converted to text format using "go tool covdata textfmt", and interpreted or visualized using "go tool cover func" or "go tool cover html". Coverage profiles can even be merged using "go tool covdata merge", amalgamating data sets from different integration test runs. This also allows for the selection of specific packages or sets of packages if necessary. These new features in Go 1.20 provide developers with a better understanding of how well their larger and more complex tests are functioning and which parts of their source code are being exercised.
Shifting focus to the Node.js ecosystem, tests are crucial for ensuring robustness and documenting functionality. They create a feedback loop for introducing new code, breaking tests, fixing them, and ensuring all tests pass. As projects grow in complexity, manually tracking how all code works becomes overwhelming, necessitating comprehensive and well-structured tests. In this context, achieving 100% test coverage can significantly reduce time spent on debugging and fixing bugs in production, thereby enhancing the overall efficiency of the software development process.
In the Java ecosystem, there are several strategies and techniques for achieving high test coverage in unit testing. Code coverage tools analyze the code and determine which parts are executed during testing. This information is used to assess the effectiveness of the test suite and identify areas that need more testing. These tools generate reports showing the percentage of code that is covered by the tests. Developers can use these reports to track test coverage progress over time and make informed decisions about where to focus their testing efforts.
Balancing test coverage and test execution time is crucial. One approach is to focus on testing critical and high-risk areas of the software first, ensuring these functionalities are thoroughly tested. Another strategy is to use code coverage analysis to identify areas of the code that are not adequately covered by tests. By increasing test coverage in these areas, the overall quality of tests can be improved without significantly increasing execution time. Techniques such as test automation and parallel execution can also be considered to optimize test execution time.
In essence, while test coverage is not the sole measure of test quality, it is a crucial aspect of software testing. Striving for high test coverage and ensuring that your tests are thorough and cover all critical paths and edge cases in your code is essential. The recent advancements in languages like Go highlight the evolving nature of testing methodologies and the increasing emphasis on comprehensive test coverage in the software industry
7. Balancing Expected vs Actual Outcomes in Unit Tests
The crux of unit testing lies in the art of writing concise and precise assertions. The assertions form the backbone of any test suite, they compare the actual outcome against the expected outcome. However, there is a fine line between ambiguity and precision, crossing which can lead to unreliable test outcomes.
Overgeneralized assertions can result in false positives, where tests pass despite the presence of bugs. This can lead to these bugs being overlooked and incorporated into the final product. Conversely, overly specific assertions can lead to false negatives, where tests fail even when the code is functioning correctly.
Both false negatives and positives have their own set of problems. False negatives can lead to unnecessary debugging sessions, which result in wastage of resources and time. It can also decrease the team's confidence in the testing suite, leading to a decreased emphasis on testing. Thus, it's crucial to maintain a balance while crafting assertions. The assertions should be specific enough to effectively catch bugs but not so much that they fail to accommodate legitimate variations in code behavior.
Achieving this balance is a real-world challenge. It demands a thorough understanding of the codebase and an awareness of different scenarios that might arise. The Code Whisperer's quote, "Unstated assumptions and limiting beliefs interfere with our performance," is particularly relevant here. Developers must challenge their assumptions and broaden their perspective to craft meaningful assertions.
The Code Whisperer suggests using different simulators for different tests. An in-memory database may be ideal for happy paths, while a test double library or the crash test dummy pattern can simulate failure paths. This approach ensures that your assertions are both robust and flexible, capable of handling a wide range of scenarios.
Remember, understanding the context is vital. What works for one test might not work for another, and the choice of test doubles should be based on the specific requirements of the test and the context in which it is being conducted. The Code Whisperer also emphasizes, "Knowing where to find experienced people who can answer your questions doesn't only help you get answers to your questions but more importantly it frees you to focus on the details." By leveraging the expertise of others and staying focused on the details, you can craft powerful and flexible assertions, leading to a more reliable and effective testing suite.
While crafting unit test assertions, it is vital to ensure they accurately represent the expected behavior of the code under test. Assertions should be meaningful, clearly communicating the expected behavior, and accurate, verifying the code's correct functionality. To achieve this, consider the following:
- Define the expected behavior clearly: Before writing the assertion, a clear understanding of what the code should do is crucial. This aids in defining the expected outcome and writing a relevant assertion.
- Use descriptive messages: Adding descriptive messages to assertions provides additional context and makes understanding the purpose of the assertion easier.
- Test specific behaviors: Write assertions that test specific functionalities or behaviors of the code. This helps isolate issues and makes identifying the cause of failures easier.
- Choose appropriate assertion methods: Select suitable assertion methods provided by your testing framework to check the expected results. Use methods specific to the type of data being tested, such as assertEquals for comparing values or assertTrue/assertFalse for testing conditions.
- Consider edge cases: Test the code with different input values, including boundary and edge cases, to ensure it handles all scenarios correctly.
By following these guidelines, you can write meaningful and accurate unit test assertions that effectively verify the behavior and correctness of your code. To prevent false negatives, it's crucial to cover all possible scenarios and edge cases. Using appropriate assertions that accurately validate the expected behavior of the code being tested is also important.
In the context of Java unit testing, some examples of meaningful and accurate unit test assertions could be:
- Asserting that a specific value is equal to the expected value:
java assertEquals(expected, actual);
- Asserting that a condition is true:
java assertTrue(condition);
- Asserting that a condition is false:
java assertFalse(condition);
- Asserting that two objects are the same instance:
java assertSame(expected, actual);
- Asserting that an object is not null:
java assertNotNull(object);
- Asserting that an object is null:
java assertNull(object);
These examples illustrate how meaningful and accurate unit test assertions can ensure the correctness and reliability of software components. The specific assertions used will depend on the requirements and behavior of the component being tested.
To ensure meaningful comparisons in unit test assertions, it is important to carefully choose the values being compared and consider the expected outcomes. By selecting appropriate values for comparison and defining the expected results, unit tests can effectively validate the behavior and functionality of the code being tested
8. Using Appropriate Assertions for Reliable Test Results
Unit tests in Java hinge on a cornerstone concept: assertions. These are statements in your code that test the assumptions you've made during development. Assertions form the backbone of the three main sections of a unit test: "arrange", "act", and "assert". When implemented correctly, they serve as a powerful tool to validate the expected software behavior.
The JUnit framework in Java offers a plethora of assertion methods, such as assertEquals
, assertTrue
, and assertNull
, each tailored for a specific testing condition. Grasping these methods and their appropriate uses is pivotal for engineers aiming to craft robust and meaningful tests.
For instance, the assertEquals
method is employed when you want to verify that two values are equal. This method takes two parameters: the expected value and the actual value. If these two values match, the test passes; if they don't, the test fails. This is commonly used when you want to compare the actual result of a method or operation with the expected outcome.
On the other hand, the assertNull
method is used to validate if an object is null. It accepts a single argument, which is the object you wish to check. Should the object be null, the test passes, but if the object is not null, the test fails. This method is typically used when your code is expected to return null values under certain conditions.
But the art of assertion doesn't only lie in choosing the right methodsβit's also about crafting better assertions. Optimal test assertions are those that check the correct conditions and accurately express the requirements of the code being tested. It's vital to be precise yet avoid being overly exact or too general as this can lead to maintainability issues or overlooked regressions.
A general guideline is to focus on one assertion per condition, steering clear of bundling multiple checks in one assertion. The emphasis of these assertions should be on evaluating requirements, not implementation details. For example, if you're testing a Java method named "getEmployees" that retrieves a list of employees based on a given predicate, your assertions might check that the function retrieves all employees older than 25, and inspect specific conditions of the result list, such as the presence of certain employees and their ages.
To aid in precision and provide enhanced debugging information, consider utilizing assertion matchers like those offered by the Hamcrest library. These matchers simplify the expression of precise conditions in your assertions. Additionally, tools like Diffblue Cover can autonomously generate Java tests with assertions based on the current behavior of the code, thereby boosting the efficacy of your unit tests.
When testing a unit of code for expected behavior, it's critical to ensure that tests are both accurate and precise. For instance, when testing sorting algorithms, it's important to consider the specific behavior expected, such as sorting in ascending order and handling duplicate values. The length of the sorted sequence should be the same as the original sequence in some programming languages.
Testing against existing implementations can serve as a test oracle, ensuring that the new code produces the same results as the old implementation. The test code should be comprehensible and simple, with concrete examples extracted from the domain to help illustrate general cases. These concrete examples make tests more accessible and unambiguous, contributing to the simplification and clarification of the test code.
In the grand scheme of unit tests, the importance of assertions cannot be overstated. They are the backbone of unit tests, checking that the software performs as it should. When written well, assertions can significantly contribute to the value of your tests. The key lies in understanding the assertion methods, writing good assertions, and using tools and libraries to assist in the process
Conclusion
In conclusion, unit testing is a crucial aspect of software development in Java. It allows developers to examine their code in detail and ensure that each component functions as intended. By following best practices and leveraging tools like JUnit, developers can significantly enhance the reliability and maintainability of their code, leading to high-quality software products. Unit tests act as a safety net during code maintenance and refactoring, preventing regression and facilitating smooth transitions in the codebase. They also serve as living documentation, providing current information about the software's expected behavior and promoting collaboration among developers, testers, and stakeholders. By implementing effective test case naming conventions, avoiding code redundancy, ensuring comprehensive test coverage, and balancing expected versus actual outcomes in tests, developers can improve the quality and stability of their Java applications.
The broader significance of unit testing lies in its ability to identify bugs early, streamline code refactoring, simplify integration testing, and serve as living documentation. It plays a vital role in continuous integration and deployment, contributing to code quality assurance and regulatory compliance. Unit testing also helps foster a culture of continuous testing and improvement by providing rapid feedback loops. By adhering to best practices for writing unit tests and utilizing tools like JUnit, developers can create reliable and maintainable software applications. Embracing unit testing as an integral part of the software development process is essential for delivering high-quality software products.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.