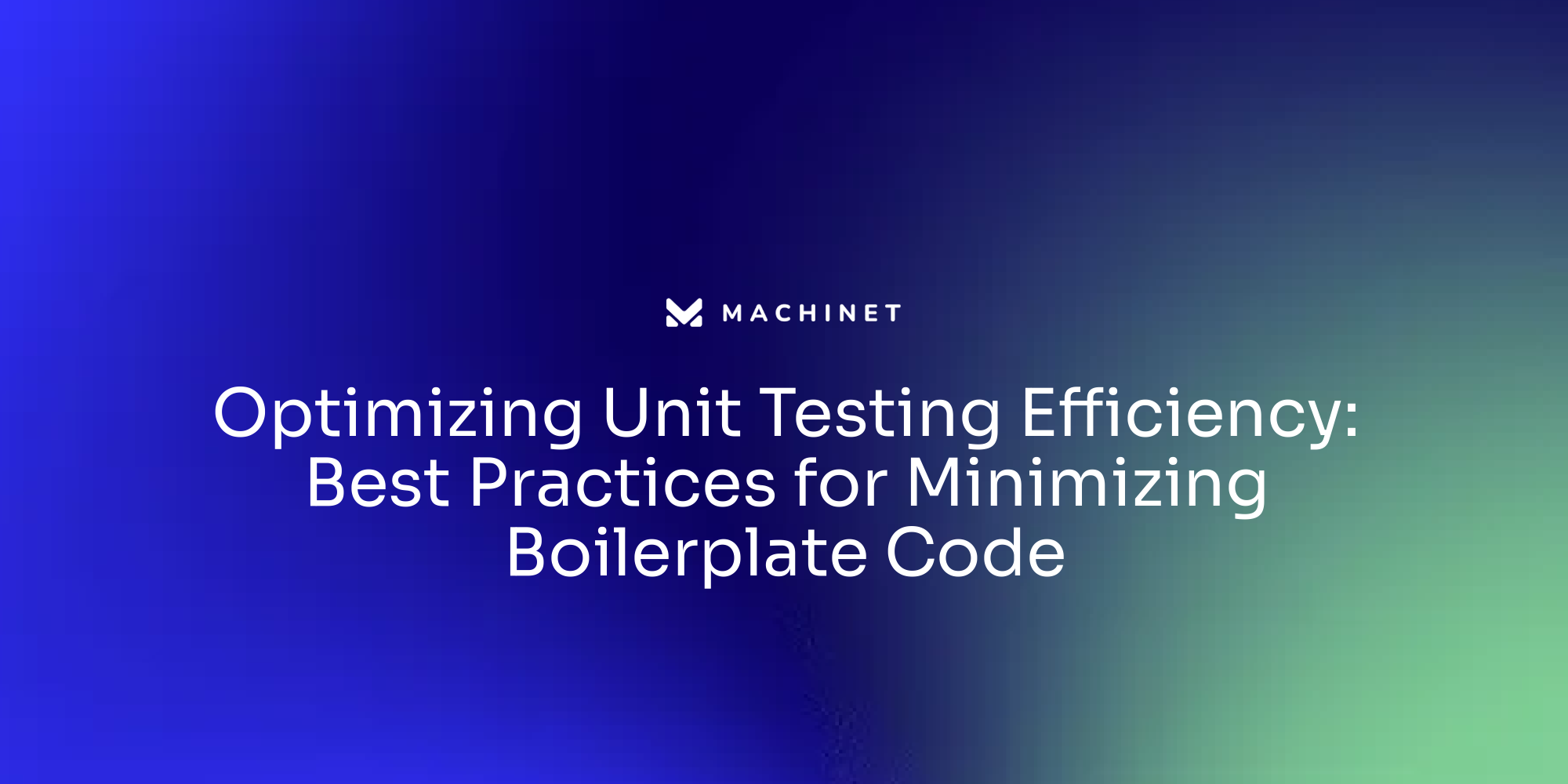
Table of Contents
- Understanding the Importance of Minimizing Boilerplate Code in Unit Testing
- Analyzing the Impact of Boilerplate Code on Unit Testing Efficiency
- Dependency Injection: A Key Strategy for Reducing Boilerplate Code
- The Debate: Is Duplicated Code More Tolerable in Unit Tests?
- Techniques and Best Practices to Reduce Boilerplate Code in Java Unit Tests
- Case Study: Successful Implementation of Strategies to Minimize Boilerplate Code in Unit Testing
- Future Trends: How AI and Automation are Changing the Landscape of Unit Testing
Introduction
Minimizing boilerplate code in unit testing is a crucial aspect of software development. Boilerplate code, which often involves repetitive setup and teardown operations, can make tests more complex, harder to maintain, and prone to errors. In this article, we will explore the importance of reducing boilerplate code in unit testing and discuss various strategies and best practices to achieve this goal.
By utilizing techniques such as factory methods, Domain-Specific Languages (DSLs), extension methods, and test data builders, developers can simplify object construction, eliminate syntactic fluff, and reduce code duplication in unit tests. We will also examine the impact of boilerplate code on unit testing efficiency and discuss the debate surrounding the tolerability of duplicated code in tests. Additionally, we will explore real-world case studies and successful implementations of strategies to minimize boilerplate code in unit testing. Finally, we will look into future trends, such as the use of AI and automation, and how they are changing the landscape of unit testing. With these insights, developers can enhance the efficiency, readability, and maintainability of their unit tests, ultimately improving the quality of their software products
1. Understanding the Importance of Minimizing Boilerplate Code in Unit Testing
Boilerplate code, especially in unit testing, is an area that often demands the attention of software developers. This refers to pieces of code that see repetitive use in various areas with little to no modification. Although boilerplate code may seem like a necessary evil in unit testing due to the frequent repetition of setup and teardown operations, its overuse can lead to unforeseen challenges. It can make the code more complex, harder to maintain, and debug, and increase the risk of errors and bugs as developers may overlook crucial details in the midst of repetitive code.
The typical unit test can contain a significant amount of non-essential information in the constructor, making it tedious to both write and review. This can be mitigated by using factory methods to hide irrelevant details and establish sensible defaults. As stated by Brian Kihoon Lee, "Factory methods hide fluff." These methods can simplify unit tests where objects accumulate fields and subobjects, making object construction a long and tedious process.
Domain-Specific Languages (DSLs) serve as another powerful tool for eliminating syntactic fluff in unit tests. They can be used to parse various file types, including CSV, YAML, and dot files. As per Lee, "DSLs hide syntactic fluff." By investing in methods that streamline object constructors, writing comprehensive unit test suites becomes significantly easier. This simplification benefits not just the test-writing process but also other areas, such as creating tutorial notebooks and debugging sessions.
Functional tests, which involve several classes or interact with the infrastructure, testing most of the application's functionality from the outside in, are another crucial aspect. Any duplication in these tests can lead to code smells and antipatterns, making the tests harder to maintain. Helper methods can assist in keeping the tests clean and dry, but they are typically useful only within the test class where they reside.
Extension methods provide a way to add functionality to existing types and can be shared across multiple test projects. Developers can create a NuGet package to share these extension methods with the community. These methods offer a cleaner syntax, and more extensions, including building POST, PUT, and DELETE endpoints with built-in logging and automatic serialization/deserialization, are planned to be added to the package.
Reducing boilerplate code is a critical aspect of software development. While it may seem like a necessary evil, there are numerous strategies and tools that developers can use to tackle this issue effectively. From factory methods and DSLs to extension methods, developers have a plethora of options to ensure their code remains clean, maintainable, and efficient.
One way to reduce boilerplate code in unit testing is by using test frameworks and libraries that provide utility functions and methods to simplify common testing tasks.
Explore test frameworks and libraries to simplify your unit testing process.
These frameworks often include features such as test setup and teardown, assertion libraries, and mocking frameworks, which can help reduce the amount of repetitive code needed in unit tests. Additionally, using design patterns such as the factory pattern or the builder pattern can also help minimize boilerplate code by providing a more flexible and reusable way to create test objects or test data.
To minimize boilerplate code in unit tests, it is important to follow best practices.
Learn and implement best practices for minimizing boilerplate code in your unit tests.
These practices include using test fixtures, using mocking and stubbing frameworks, and utilizing helper methods. By using test fixtures, you can set up common test data and configurations, reducing the amount of repetitive code in your tests. Mocking and stubbing frameworks allow you to simulate dependencies and isolate the code under test, eliminating the need for complex setup code. Additionally, creating helper methods for common test tasks can further reduce boilerplate code and improve test readability. By following these best practices, you can minimize boilerplate code in your unit tests and make them more efficient and maintainable
2. Analyzing the Impact of Boilerplate Code on Unit Testing Efficiency
While boilerplate code in unit testing may be a necessary evil, it can significantly hamper the efficiency of the process. It not only complicates tests making them harder to understand and maintain, but also increases debugging time, thus reducing time for feature development. Moreover, any changes made to the boilerplate code may affect multiple tests leading to a cascade of failures. This makes it difficult to isolate and address issues, thereby slowing down the development process.
Constructing objects for testing, also known as "fluffy constructors", is another common challenge in unit testing. Over time, these constructors may become bloated due to accumulation of fields and subobjects, making object construction an intimidating task. In some cases, hundreds of lines of code are required just to construct the arguments and expected output. This complexity can discourage comprehensive unit testing as the constructors are tedious to write, review, and scroll through.
However, several strategies can mitigate these issues. One such strategy is the use of factory methods, which can simplify object construction by hiding irrelevant details and setting sensible defaults. Not only do these methods reduce the amount of code needed, but they also facilitate manual verification of the tests.
Another strategy involves the use of Domain-Specific Languages (DSLs) to eliminate syntactic fluff in unit tests. DSLs can provide a more concise and readable syntax for writing tests, reducing visual noise and making tests easier to understand. Existing DSLs, such as those used for parsing CSV, YAML, and dot files, can be leveraged to avoid the need for manual object construction.
In the context of functional tests for API endpoints, boilerplate code can also introduce challenges. Functional tests, which involve several classes or interact with infrastructure, are intended to test most of an application's functionality from the outside in. However, code duplication in these tests can lead to code smells and antipatterns, such as shotgun surgery.
To avoid these issues, it is recommended to keep test classes small and focused, and tests neat and to the point. Helper methods can be used to keep tests clean and dry, but they are usually only useful within the test class where they reside.
Extension methods offer a way to add functionality to existing types and can be used to share code across multiple test projects. They can also be packaged into a NuGet package for easy sharing and reuse. For instance, an extension method for making HTTP requests and deserializing the response can be created and shared across multiple projects. As these methods are expanded and added to the NuGet package, they can be easily accessed and utilized by other developers.
On the other hand, strategies like test data builders and mocking frameworks can also be beneficial in reducing boilerplate code in unit tests. Test data builders are a design pattern that allow you to create test data objects with minimal code, reducing the amount of boilerplate code in your unit tests. Mocking frameworks allow you to create mock objects that simulate the behavior of real objects, reducing the need to write repetitive and boilerplate code for setting up test scenarios. By using these techniques, you can make your unit tests more concise and focused, while still ensuring comprehensive test coverage.
Moreover, using testing frameworks and libraries that provide useful utilities and abstractions for writing tests can also be an effective way to reduce boilerplate code. These frameworks often have built-in methods for setting up test fixtures, mocking dependencies, and asserting expected outcomes. By using builders or factories, you can easily create complex objects required for testing without writing repetitive code. Also, by decoupling components and injecting dependencies, you can focus on testing individual units of code without the need for setting up complex environments.
In conclusion, strategies such as factory methods, DSLs, and extension methods, along with techniques like test data builders, mocking frameworks and leveraging testing frameworks can be instrumental in reducing boilerplate code in unit tests. These strategies can enhance the efficiency of unit testing, making it easier to write, review, and maintain tests, and ultimately accelerating the software development process
3. Dependency Injection: A Key Strategy for Reducing Boilerplate Code
Dependency Injection (DI) is a vital tool in reducing boilerplate code in Java unit tests. However, its true power emerges when used in conjunction with other strategies like test data builders, the Object Mother pattern, and the Builder pattern.
Test data builders are key in eliminating duplication and enhancing expressiveness in test data creation. They remove the need for redundant setup and teardown code, leading to cleaner and more maintainable tests. For instance, when testing business logic surrounding objects such as orders or customers, test data builders come in handy.
The Object Mother pattern provides factory methods for different use cases in tests, enhancing the readability of test code. It can be used to create various objects for testing purposes, such as when testing a constructor with a null or non-null parameter, or testing a method like getName.
Moreover, the Builder pattern simplifies object construction, especially for complex objects. For instance, a test data builder for an 'Order' class could be created with 'with' methods for each constructor parameter.
A good practice is setting safe default values in builders to hide irrelevant details in the test code. This can be achieved by passing builders as arguments, which simplifies the code and removes syntax noise. The use of factory methods can emphasize the domain and reduce noise in tests.
To reduce code duplication when creating similar objects, a builder with a joint state can be extracted to handle the differences separately. This strategy can be particularly useful when applying different shipping rates for foreign addresses, or applying a discount rate and coupon code simultaneously.
Lombok, a library that helps reduce boilerplate code, is another effective technique. Lombok annotations like @Data
and @Builder
can be used to automatically generate a builder class and eliminate the need for default constructors and getters. This not only simplifies the creation of builders but also enhances code readability.
Additionally, combining builders and object mothers can provide safe default values and further reduce boilerplate code. This combination can be particularly beneficial when dealing with fields that are not relevant to the test case.
These techniques, when combined, can significantly increase the readability, resilience to changes, and reduction of boilerplate code in unit tests. They can result in easier and faster test writing, fewer bugs, and easier code refactoring.
To implement DI in unit tests, frameworks like Mockito for Java can be used. Mockito allows you to create mock objects and inject them into your unit tests, enabling the unit to be tested in isolation and focusing on the specific functionality being tested. Mock objects can be created for any class or interface, allowing you to simulate the behavior of dependencies without actually using the real implementations. This makes it easier to write unit tests that are independent of external dependencies and more focused on the unit itself.
In the end, writing good tests is a marketable skill, and adopting these conventions can greatly enhance the quality of your tests, making them a valuable resource for your entire tech team
4. The Debate: Is Duplicated Code More Tolerable in Unit Tests?
The topic of code duplication in unit tests is a subject of much debate in the software development realm. Some developers argue that a certain degree of duplication can enhance readability and isolation in tests, while others counter that this practice can lead to complexities similar to those seen in production code, such as making tests harder to maintain and increasing the risk of elusive bugs. The appropriate approach often depends on the specific circumstances and requirements of the project.
A common pitfall in unit testing is the replication of logic in assertions, which should be avoided. Instead, use known hard-coded pre-calculated values. It's inadvisable to create private methods that duplicate the logic under test for use in assertions. Also, avoid exposing the internals of the tested logic by transforming private methods into public and static ones.
The focus should be on testing the observable behavior of the tested code, such as returned values, thrown exceptions, or external invocations. Known values should be used for assertions rather than duplicating the tested logic or exposing internals. To make the code more readable, constants can be created for known expected values. It's also advisable to avoid overcomplicating tests with excessive logic; tests should concentrate on assignments and method calls.
As software developers, we should remember that code duplication, even in our tests, can lead to significant issues down the line, including challenges with maintainability and hard-to-trace bugs. Therefore, it's vital to exercise caution when writing unit tests and to ensure they are as clean and efficient as possible.
The issue of code duplication in unit tests is not a straightforward one; it's a complex topic that requires careful thought. The key is to strike a balance that maintains the efficiency and readability of your tests without compromising their integrity. The specific approach will depend on the nature of your project and the challenges it presents.
Several strategies can help reduce code duplication in unit tests, thus improving maintainability and reducing the risk of errors:
- Extract Reusable Helper Methods: Identify common operations or setups that are repeated across multiple tests and extract them into reusable helper methods. This allows you to write the code once and reuse it in multiple tests.
- Use Test Data Builders: Instead of manually creating test data for each test case, consider using test data builders. These are objects or methods that help in creating test data in a concise and readable way. They can be reused across multiple tests, reducing code duplication.
- Utilize Test Fixtures: Test fixtures are a set of common objects or setup steps that are shared across multiple tests. By using test fixtures, you can avoid repeating the same setup code in each test and instead define it once in the fixture.
- Apply the DRY Principle: The DRY (Don't Repeat Yourself) principle encourages developers to avoid duplicating code. Apply this principle to your unit tests by identifying common patterns or logic that can be abstracted into reusable components.
- Use Inheritance or Composition: Inheritance and composition are object-oriented programming techniques that can be used to reduce code duplication. In the context of unit tests, you can create base test classes that contain common test setup or helper methods, and then have specific test classes inherit from or compose those base classes.
By applying these strategies, you can effectively reduce code duplication in your unit tests and improve the maintainability of your test suite.
Implement strategies to reduce code duplication and improve the maintainability of your unit tests.
Code duplication in unit tests can have various effects on the overall quality and maintainability of the codebase. By avoiding code duplication, developers can ensure that unit tests are concise, easier to understand, and less prone to errors. This can lead to more efficient testing processes and improved code coverage. Additionally, reducing code duplication in unit tests can also help in identifying and fixing bugs more effectively, as changes made in one place will automatically reflect in all the relevant tests. Case studies have shown that minimizing code duplication in unit tests can lead to better test coverage, improved code quality, and enhanced maintainability of the codebase.
A trade-off analysis is important when it comes to code duplication in unit tests. It's necessary to carefully consider the benefits and drawbacks of reducing code duplication. One benefit of reducing code duplication in unit tests is improved maintainability. By eliminating redundant code, it becomes easier to make changes or updates to the test suite. This can save time and effort in the long run. On the other hand, reducing code duplication in unit tests may come with some drawbacks. It can lead to increased complexity, as the test code may become more abstract or require additional setup. This can make the tests harder to understand and maintain.
Ultimately, the decision to reduce code duplication in unit tests should be based on the specific needs and constraints of the project. It may be beneficial to prioritize maintainability and readability, especially in larger test suites. However, in some cases, a certain level of code duplication may be acceptable if it simplifies the test implementation or improves test coverage. Overall, it is important to weigh the trade-offs and make an informed decision based on the specific context of the project
5. Techniques and Best Practices to Reduce Boilerplate Code in Java Unit Tests
In the world of Java unit testing, there's a myriad of strategies and practices that can significantly pare down boilerplate code, thus enhancing the readability and efficiency of the tests. A key player in this process is the JUnit testing framework, as it offers several features specifically engineered to minimize boilerplate. This includes annotations such as @Before
, @After
, @BeforeEach
, and @AfterEach
, which alleviate the need for manual setup and teardown code.
Additionally, JUnit's parameterized tests feature allows for a single test method to be executed multiple times with different sets of input data. This, along with the @ParameterizedTest
and @ValueSource
annotations, eliminates the need for duplicating test logic. Furthermore, JUnit's assertions library simplifies test assertions with a comprehensive set of methods like assertEquals()
, assertTrue()
, and assertThrows()
.
Moreover, JUnit's test fixture inheritance feature can be leveraged to reduce code duplication in your test classes. By creating a base test class that contains common setup and utility methods, you can reuse the common code in your individual test classes.
Mocking frameworks such as Mockito and EasyMock can further streamline the process. These frameworks assist in creating mock objects and defining their behavior, which reduces the need for extensive setup code.
To encapsulate common setup and teardown operations, utility methods and test helper classes can be implemented, making the tests more readable and cleaner. For instance, utility methods can be created for setting up test data or test objects, such as creating mock objects or initializing test fixtures. Similarly, a reusable method for asserting the expected outcome of a test can be created, making it easier to validate the correctness of their code without having to write the same assertions multiple times.
Additionally, test data builders can be used to not only eliminate code duplication but also enhance the expressiveness of test data construction. The Builder pattern, for instance, can be used for a more flexible solution to object creation problems. Setting safe default values in test data builders can also be beneficial as it hides irrelevant details, simplifying the code.
Programs like Lombok can be used to reduce boilerplate code in test data builders. Moreover, builders and object mothers can be combined to tackle the absence of safe default values.
For instance, testing a simple customer class can be done by testing the constructor with a null parameter, testing the constructor with a non-null parameter, and testing the getName
method. Each test should verify one piece of functionality and be a few lines of code.
Adopting the given-when-then style, where 'given' sets up a certain condition, 'when' triggers the action being tested, and 'then' asserts the expected outcome, can create concise and easy-to-read living documentation for the project. By following these conventions, writing tests becomes easier, faster, and more enjoyable, resulting in fewer bugs. This practice also makes future code refactoring significantly easier since the code is now backed by consistent, self-documenting tests
6. Case Study: Successful Implementation of Strategies to Minimize Boilerplate Code in Unit Testing
Airbnb's Android development process offers a powerful demonstration of how strategies focused on minimizing boilerplate code can be effective. They've centered their approach around a unit testing framework for viewmodel logic which has substantially streamlined manual testing. The framework is built on the idea that each viewmodel function should be independently testable, with its behavior determined by the state of the viewmodel and the parameters passed to it.
In practical terms, each unit test within this framework calls a single viewmodel function with the initial state and parameters, then asserts the expected changes in the state or expected calls to dependencies. The framework is designed to accommodate a wide range of viewmodel patterns, providing utilities for testing common patterns such as functions that update a single property on the state. Furthermore, the system is extensible, allowing the integration of third-party extension functions to add custom assertions and statements.
The framework also supports testing for the initialization behavior of viewmodels, including the execution of network requests during instantiation. To accommodate a multi-module environment, it includes tools for generating test scaffolding to establish a unit test environment for each new module.
The main goal of this unit testing framework is to alleviate the friction in testing viewmodel logic. It achieves this by offering a simple yet flexible API that can address all use cases of a viewmodel. The framework is open-source, which allows teams to add their own assertions to the DSL.
Airbnb is moving towards the automatic generation of tests as the ultimate goal. This approach has produced tests that are easier to read and maintain, more reliable, and faster to run. This has played a crucial role in Airbnb's ability to deliver high-quality software products more efficiently, highlighting the real-world benefits of minimizing boilerplate code in unit testing.
Airbnb's experiences also show that the use of mocks and spies can lead to the creation of reliable and fast isolated interaction-based tests. They also highlight the importance of separating logic from infrastructure using methods like hexagonal architecture and functional core imperative shell.
The "Testing without Mocks" pattern language offers an alternative method that avoids issues associated with broad tests and mocks. This pattern language emphasizes the use of narrow tests, state-based tests, and overlapping sociable tests, along with infrastructure techniques like nullables. This allows for isolated testing of code that is dependent on external systems or state.
However, the success of strategies to minimize boilerplate code ultimately rests on the use of automated tests, the separation of logic from infrastructure, and the adoption of innovative testing patterns. Airbnb's experiences provide valuable insights into how these strategies can be applied to deliver high-quality software products more efficiently.
In line with this, minimizing boilerplate code in unit testing can bring several benefits. By reducing the amount of repetitive code, it becomes easier to write and maintain unit tests. This can save developers time and effort. Furthermore, minimizing boilerplate code can improve test code's readability and clarity, making it easier for other developers to understand and work with it.
In a similar vein, Machinet has implemented dependency injection and testing frameworks to minimize boilerplate code. They've adopted dependency injection, a design pattern that allows the separation of object creation and usage. This helps to minimize boilerplate code by providing a way to manage dependencies and easily switch out implementations.
Additionally, Machinet has implemented testing frameworks, tools that help automate the testing process. These frameworks provide a set of functions and utilities that simplify writing and executing tests. By using testing frameworks, Machinet can automate the testing process, reduce the amount of manual testing, and therefore minimize boilerplate code.
Also, Machinet uses utility methods to reduce boilerplate code in unit tests. They have seen improvements in their unit testing process by reducing repetition and increasing efficiency. By implementing techniques such as creating reusable test utilities and using frameworks that provide built-in testing functionalities, Machinet has streamlined their unit testing process. This has resulted in faster test creation, easier test maintenance, and improved overall code quality.
In conclusion, the successful implementation of strategies to minimize boilerplate code hinges on the use of automated tests, the separation of logic from infrastructure, and the adoption of innovative testing patterns. The experiences of Airbnb and Machinet provide valuable insights into how these strategies can be applied to deliver high-quality software products more efficiently
7. Future Trends: How AI and Automation are Changing the Landscape of Unit Testing
Unit testing is undergoing a significant transformation with the advent of AI and automation. The introduction of AI-powered unit testing tools such as SapientAI and CodiumAI is reshaping the field. These tools are designed to reduce the need for boilerplate code in unit tests, thereby increasing productivity and code quality.
SapientAI, an AI-driven unit test generator, stands out with its ability to understand code context and generate relevant tests. It provides comprehensive coverage for every exit point of methods. Supporting a variety of well-known programming languages, this tool can be seamlessly integrated with existing AI stacks. Its unique focus on unit testing sets it apart in the market.
SapientAI's ability to swiftly generate unit tests for an entire codebase is a key benefit. Simply point it to a directory, and it gets to work, saving developers considerable time and effort. Furthermore, it serves as an early warning system, flagging areas in the code that might need refactoring. This feature is particularly useful in managing technical debt, a common issue in software development. For Java developers, SapientAI is readily available on the IntelliJ Marketplace.
Another tool contributing to the transformation of unit testing is CodiumAI. It focuses on code integrity and generating meaningful tests. CodiumAI offers an IDE plugin and a Git plugin, allowing for easy integration into coding workflows. It provides code suggestions and test generation right inside the IDE, aiding developers in writing reliable code. CodiumAI supports major programming languages and Git code hosting services, making it a versatile tool for developers.
The benefits of using AI-powered unit testing tools are manifold. They not only accelerate development productivity but also enhance code coverage and scalability. Developers can automate the execution of test cases, generate test data, and analyze the test results, reducing the time and effort required for manual testing. These tools can also identify patterns and anomalies in test data, making it easier to detect and fix bugs.
Another way to reduce boilerplate code is to leverage AI-powered plugins, such as Machinet's AI plugin, which can automatically generate code snippets or templates based on specific requirements. By using AI algorithms, these plugins can analyze the context of the code and suggest optimized solutions, resulting in less boilerplate code and improved efficiency in development.
As AI and automation technologies continue to evolve, unit testing is set to become even more efficient and streamlined. The reduction in boilerplate code will undoubtedly lead to more concise and focused unit tests, thereby enhancing the overall quality of software applications. The future of unit testing with AI and automation is promising, and we can expect to see more advanced tools and techniques in the years to come
Conclusion
In conclusion, minimizing boilerplate code in unit testing is crucial for software development. It can lead to more efficient, maintainable, and reliable tests. Strategies such as using factory methods, Domain-Specific Languages (DSLs), extension methods, test data builders, and mocking frameworks can significantly reduce code duplication and simplify object construction. These techniques improve the efficiency and readability of unit tests while ensuring comprehensive test coverage. Additionally, the use of AI-driven tools and automation is changing the landscape of unit testing, offering opportunities to further streamline the process and enhance productivity.
The broader significance of minimizing boilerplate code in unit testing lies in improving the overall quality of software products. By reducing complexity and eliminating redundancies in tests, developers can focus on writing concise and effective tests that accurately verify the behavior of their code. This leads to faster bug detection and easier maintenance, ultimately resulting in higher-quality software. To boost your productivity with Machinet, experience the power of AI-assisted coding and automated unit test generation here
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.