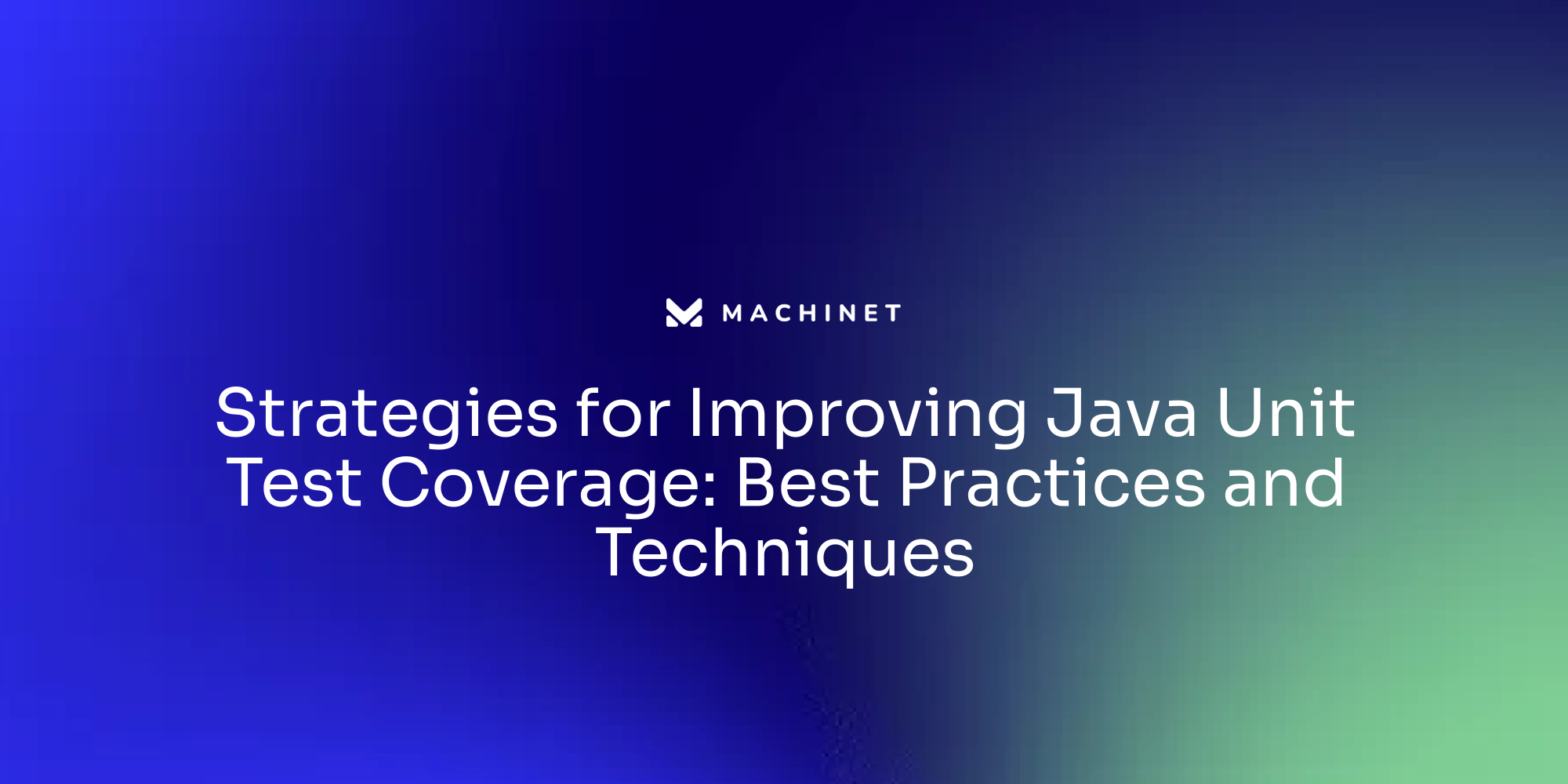
Table of contents
- Understanding the Importance of Unit Test Coverage in Java
- Exploring Techniques to Increase Java Unit Test Coverage
- The Role of Refactoring in Enhancing Test Coverage
- Utilizing JaCoCo for Viewing and Improving Unit Test Coverage
- Addressing Common Challenges in Achieving Optimal Code Coverage
- Best Practices for Managing Technical Debt and Legacy Code in Unit Testing
- Adapting to Changing Requirements: Strategies for Robust and Flexible Testing Frameworks
- Balancing Workload Management and Deadlines without Compromising on Quality
Introduction
Unit testing is a crucial aspect of software development, ensuring the reliability and quality of code. However, achieving optimal code coverage and managing technical debt and legacy code can present challenges. Additionally, adapting to changing requirements and balancing workload management without compromising on quality are key considerations in unit testing. In this article, we will explore various strategies and best practices for achieving high unit test coverage, managing technical debt and legacy code, adapting to changing requirements, and effectively managing workload and deadlines. By implementing these techniques, developers can enhance the quality and reliability of their software applications while maintaining productivity and meeting project deadlines
1. Understanding the Importance of Unit Test Coverage in Java
Unit testing is a cornerstone of robust software development in Java, assisting in the early detection of bugs and ensuring the reliability and quality of code. The percentage of the codebase that unit tests exercise is what we refer to as code coverage. The more extensive the code coverage, the higher the probability of catching bugs early on, thus enhancing the reliability of the software.
However, achieving complete code coverage can be challenging due to various conditions within methods that may not be executed during testing. Therefore, it's essential to continuously review and update unit tests as the codebase evolves, maintaining and improving coverage. This practice can be furthered by employing code coverage analysis tools to identify parts of the code with low coverage, and creating new test cases specifically for those areas.
Tools like JaCoCo, Cobertura, and Emma are invaluable in measuring code coverage, providing insights into areas that are not adequately tested. They can help you prioritize writing tests for parts of the code that are currently under-tested. Notably, some of these tools also measure branch coverage, which represents the coverage of different branches within conditional statements.
To ensure the dependability of software and maintain the quality of the code, it is crucial to write comprehensive test cases that cover different scenarios and edge cases. A good practice is to use mocking frameworks to simulate dependencies and isolate the code under test, allowing for more focused and targeted testing. Mockito, for instance, is a powerful framework that can be used to mock classes and stub methods for testing.
Writing testable code is another important aspect of improving unit test coverage. This involves writing small, focused methods, using dependency injection, and separating the concerns in your code by following the principles of encapsulation and modularity. By breaking your code into smaller, independent units or modules, you can test them in isolation.
Moreover, writing loosely coupled code, where the dependencies of a class are provided from outside rather than being created internally, allows for easier substitution of dependencies during testing. This makes the code easily mockable, which is beneficial for creating mock objects that simulate the behavior of real objects during testing.
In essence, unit testing is more than just a measure of code reliabilityβit also serves as a form of documentation, providing insights into the behavior and expected outcomes of different components of the codebase. As such, achieving high unit test coverage in Java contributes to more reliable, robust, and scalable software applications.
With these strategies and practices in place, you can significantly improve the quality and reliability of your Java unit tests, enhancing the overall quality and dependability of Java applications
2. Exploring Techniques to Increase Java Unit Test Coverage
Boosting Java unit test coverage can be achieved through a variety of strategies. One prevalent method is utilizing established testing frameworks, like JUnit and Mockito. For a more comprehensive understanding of these testing frameworks and their effective usage, you can refer to resources such as the blog posts on "machinet.net" which provides detailed insights.
Test coverage tools like JaCoCo offer significant benefits. They measure the extent of testing conducted on the program's source code by providing information about which parts of the code are executed during testing. This valuable data allows developers to identify untested areas of the code and improve overall test coverage. The JaCoCo tool offers metrics like line coverage, branch coverage, and cyclomatic complexity, which aid in assessing the quality and effectiveness of the tests.
Another technique that can significantly enhance test coverage is the adoption of test-driven development (TDD). TDD promotes the practice of writing tests prior to the actual code, which ensures that all code written has corresponding tests, thereby improving test coverage. There are several best practices to consider when implementing TDD in Java. These include starting with small, incremental tests, writing tests before code, using meaningful test names, keeping tests independent and isolated, writing minimal code to pass tests, refactoring code after passing tests, and continuously running tests.
Refactoring code to improve its testability is another method that can contribute to enhanced test coverage. Several techniques can be used for refactoring code to improve testability in Java. These include extracting methods, dependency injection, decoupling dependencies, applying SOLID principles, and using design patterns. By making the code more modular and reducing its complexity, tests can be written more easily and efficiently, covering a larger portion of the codebase.
However, while striving to increase Java unit test coverage, developers should be wary of common pitfalls such as relying too heavily on a single type of test, writing tests that are too tightly coupled to the implementation details of the code, writing tests that are too complex or difficult to understand, and not regularly reviewing and updating the tests as the codebase evolves.
Ultimately, the combination of these techniques can significantly increase the unit test coverage in Java, leading to more robust and reliable software. It is also beneficial to study successful case studies to gain insights into effective strategies and techniques that have been proven to work in improving test coverage in Java projects
3. The Role of Refactoring in Enhancing Test Coverage
Refactoring holds a significant role in enhancing test coverage, a metric that denotes the proportion of the codebase verified by unit tests. This process involves modifying the code structure to make it more testable and readable, without affecting its primary functionality. The refined code is simpler to test thoroughly, leading to a stronger suite of unit tests.
Refactoring, albeit being a complex task, especially with convoluted code, can be managed efficiently with a strategic approach. One such approach is the breakdown of complex methods into simpler ones. This enhances code readability and isolates different functionalities, enabling individual testing.
Another prevalent refactoring strategy is the elimination of duplicate code. This strategy reduces the codebase size and eradicates the need for repeated testing. Moreover, it boosts the code's maintainability, making future modifications less susceptible to errors.
Design improvement of the code is a crucial aspect of refactoring. It focuses on enhancing the code structure to make it more logical, efficient, and coherent. This, in turn, increases the code's testability, allowing for more precise and reliable unit tests.
It's vital to remember that refactoring should not be a standalone process. Rigorous testing should accompany it to ensure the code's functionality remains intact. Temporary logs can be used during refactoring to strengthen tests and ensure correct data logging. Once the refactoring process is complete, these temporary logs should be replaced with proper assertions to maintain the integrity of the tests.
Refactoring also plays a pivotal role in managing fragile tests, a common issue in Test-Driven Development (TDD). Fragile tests are those that require significant changes when minor modifications are made to the production code. To mitigate this, the tests' structure should be contra variant with the production code structure, meaning the tests should not mirror the production code. Instead, they should have an independent structure to minimize coupling with the production code.
As the production code undergoes refactoring, the tests should also be adapted to maintain this contra variance. This adaptation could involve reorganizing private methods into separate classes and extracting them from the original class. It's worth noting that this extraction process does not necessitate new tests, as these extracted classes should already be covered by the existing tests.
By decoupling the structure and behavior of tests from the production code, the system becomes more resilient and adaptable to refactoring. Over time, as the development progresses, the behavior of the tests becomes more specific, while the behavior of the production code becomes more generic. This generalization of the production code is a form of decoupling, as it allows the code to cater to a wide range of unspecified behaviors.
In a nutshell, meticulous and strategic refactoring significantly contributes to enhancing Java unit test coverage. It improves the code's testability and the tests' robustness, leading to a more reliable and high-quality software product
4. Utilizing JaCoCo for Viewing and Improving Unit Test Coverage
JaCoCo is a widely recognized tool in the Java ecosystem, offering a valuable mechanism for enhancing unit test coverage. As a free and open-source code coverage library, it offers detailed insights into the execution of various parts of your code during unit testing. This invaluable tool supports diverse coverage metrics such as line coverage, branch coverage, and even cyclomatic complexity, providing a comprehensive understanding of your code's test coverage.
The detailed coverage reports generated by JaCoCo empower developers to identify areas of code lacking sufficient testing, thus necessitating additional tests. By using JaCoCo, you can ensure the effectiveness of your unit tests in testing all parts of your code. Notably, its compatibility with popular build tools like Maven and Gradle allows for a smooth integration into the development process.
To integrate JaCoCo with Maven for test coverage analysis, you'll need to incorporate the JaCoCo plugin into your Maven project's configuration. This plugin will then generate the required coverage reports during the build process. Furthermore, you can configure the plugin to define your preferred coverage metrics and output formats. Once you've configured the JaCoCo plugin, running your Maven build will generate the coverage report ready for analysis.
For projects using Gradle, the JaCoCo plugin needs to be added to your Gradle build script. This plugin provides tasks for generating code coverage reports by instrumenting your code during the testing phase. You can also configure the plugin to exclude certain classes or packages from the coverage report if needed. Once the plugin is configured, running the appropriate Gradle task will generate the coverage report.
Understanding code coverage, or the degree of your source code coverage by a test, is essential to building confidence in your test results. Tools like Codecov can transform code coverage reports into meaningful data, working in conjunction with your continuous integration (CI) system to analyze every commit and provide insights into test performance.
Integrating JaCoCo into your Android project allows for the generation of a code coverage report. This report can then be used to write better tests and build better apps. The process of implementing code coverage in your Android project involves writing unit tests, adding JaCoCo to your project, and automating the process using GitHub Actions.
You can generate code coverage reports in HTML format using the Gradle command "gradlew connectedcheck" or "gradlew testdebugunittest". These reports can then be viewed locally in Android Studio. To send a code coverage report to Codecov, the Codecov plugin must be installed in your GitHub repository. The Jacoco XML report format can be used to push a test report to Codecov. Automating the process of sending your code coverage report to Codecov can be achieved using a YAML file in your GitHub Actions workflow.
The powerful capabilities of JaCoCo and its compatibility with various programming languages and frameworks make it an essential tool in any developer's arsenal. By leveraging JaCoCo's features, you can ensure healthier code, improved software quality, and a more robust testing process
5. Addressing Common Challenges in Achieving Optimal Code Coverage
Achieving optimal code coverage is a critical component of software testing, although it comes with its set of challenges. The complexity of the codebase, limitations in time and resources, and the pressure to deliver comprehensive tests can often result in inadequate testing. Code coverage, as a metric, offers a visual depiction of which sections of the source code are executed during testing. It's a vital tool in mitigating bugs and syntax errors, providing developers with clear, measurable goals for improving code quality and enhancing testing proficiency.
Several factors can impact the ability to attain substantial code coverage. These range from the choice of programming languages and testing frameworks, to the level of professional maturity and experience of the development team. Certain industries, such as aviation, transportation, and electrical safety, may have legal obligations necessitating a minimum code coverage benchmark.
Quality tests should always take precedence over achieving a high code coverage percentage. Different tests like unit tests and end-to-end tests have unique objectives, levels of control, and practicality for testing various aspects of an application. Approaches to writing code, such as adhering to the rules of test-driven development (TDD), can influence the level of code coverage.
In light of these factors, it's crucial to aim for an appropriate level of code coverage rather than a universal metric. This level should be determined based on proper testing, regulatory requirements, team size and maturity, test types, and test quality. While achieving 100% code coverage may seem ideal, it's not always practical or cost-effective. An over-reliance on code coverage percentage can lead to a false sense of security if the tests do not adequately check for correct results. Google suggests that 60% coverage is "acceptable," 75% is "commendable," and 90% is "exemplary." Most repositories using Codecov find that their code coverage values decrease when they go beyond 80% coverage.
Prioritizing high-quality tests for critical parts of the codebase is more beneficial than striving for 100% code coverage. This focus nurtures a robust testing culture, resulting in fewer bugs in production. To navigate these challenges, it's essential to follow best practices like writing testable code, leveraging testing frameworks and coverage tools, and integrating testing into the development process.
One effective way to achieve optimal code coverage is by implementing effective unit testing. Unit testing involves writing test cases for individual units of code, such as functions or methods, to ensure that they produce the expected output. By writing comprehensive unit tests and covering as many code paths as possible, you can increase the code coverage.
Writing testable code is crucial to this process. Best practices include writing modular and loosely coupled code, applying the Single Responsibility Principle (SRP), using dependency injection, writing unit tests, and using mocking frameworks.
Incorporating testing into the development process is also critical. This involves creating a clear testing strategy and implementing it throughout the development lifecycle. By including testing activities such as unit testing, integration testing, and system testing, developers can identify and fix issues early on, ensuring the quality of the software being developed.
Machinet, a software development and testing platform, can be instrumental in automating the generation of unit tests, thereby saving valuable time and effort. The benefits of using Machinet for unit test generation may include improved code quality, increased test coverage, faster test creation, and reduced manual effort in writing unit tests
6. Best Practices for Managing Technical Debt and Legacy Code in Unit Testing
In the sphere of unit testing, managing technical debt and legacy code is a significant challenge. Technical debt, the additional work that surfaces from choosing an easier, short-term solution over a more sustainable one, can accumulate over time, complicating efforts to enhance the codebase. It often manifests as intricate, inadequately tested and documented code, impeding the attainment of high test coverage.
For startups, particularly those that are self-funded or bootstrapped, the accumulation of technical debt is a common phenomenon as they aim to rapidly market their products. This strategic decision, prioritizing customer acquisition and product growth over codebase perfection, can lead to long-term effects that are challenging to manage, such as a brittle and complex codebase.
To effectively manage technical debt, it's crucial to measure it, allocate time to address it, and consistently monitor and adjust the allocation. Various methods can be employed to measure technical debt, such as tracking tech debt-related tickets with an issue tracker or using DevOps Research and Assessment (DORA) metrics. Allocating time for tech debt work, typically around 10-20% of total development time, should be a long-term, ongoing initiative rather than a single sprint.
Legacy code, on the other hand, signifies outdated code that still impacts software functionality. It's often characterized by bugs, a lack of tests, and specific server dependencies, with limited documentation available. Gaining a deep understanding of the system, its original purpose, and the core problem it was designed to solve is crucial to effectively manage legacy code. Setting up a sandboxed environment for safety and ease of changes is also crucial.
Following the code paths and documenting them can shed light on the various functions within the codebase. Writing integration tests, rather than unit tests, can maximize test coverage and minimize the level of understanding required to work with the code.
Regularly reviewing and refactoring existing unit tests can help identify areas of the code that are difficult to test due to accumulated technical debt. Prioritizing unit testing for critical or frequently changing code can minimize the impact of legacy code on the testing process. Furthermore, establishing clear guidelines and standards for unit testing, including code coverage requirements and test case documentation, can ensure consistency and quality in the testing process.
In terms of refactoring code, it is recommended to break down the code into smaller, more manageable functions or methods to improve readability and maintainability. Applying the SOLID principles, such as Single Responsibility Principle (SRP) and Dependency Inversion Principle (DIP), can ensure each class or module has a clear and specific responsibility and dependencies are properly managed.
The use of appropriate design patterns, such as the Factory Method or Dependency Injection, can also contribute to code quality and testability. These patterns promote loose coupling and facilitate the creation of test doubles, like mocks or stubs, essential for writing effective unit tests.
In terms of managing legacy code, one approach is to prioritize the most critical functionality and create tests for that first. This can help ensure that the most important parts of the code are covered by tests. Additionally, it may be necessary to refactor the code to make it more testable. This could involve breaking dependencies, extracting interfaces, or introducing dependency injection.
Various tools are available for managing technical debt and legacy code in unit testing, such as SonarQube, which provides comprehensive code quality analysis, and JUnit, a popular unit testing framework for Java applications. Tools like Mockito and PowerMock can be used to mock dependencies and simulate different scenarios during unit testing.
By following these practices, you can improve the quality and testability of your code, leading to higher test coverage and better software products
7. Adapting to Changing Requirements: Strategies for Robust and Flexible Testing Frameworks
Unit tests, like software requirements, are fluid in nature and require constant adaptation to stay relevant. This fluidity can be effectively managed with robust and adaptable testing frameworks that facilitate easy test alterations. For instance, flexible testing frameworks allow for easy test customization and modification, enhancing the efficiency and effectiveness of the testing process. Such frameworks, with a wide range of functionalities and integrations, can be seamlessly incorporated into existing testing workflows.
One approach to adapting to changing requirements is the use of parameterized tests. These tests define different test cases using different input parameters, allowing for easy modification of test cases without rewriting the entire test suite. In addition, mock objects can be employed to isolate the unit under test from its dependencies, making it easier to modify the behavior of these dependencies as per the changing requirements.
Test-driven development (TDD) and behavior-driven development (BDD) are methodologies that can aid in the adaptation of unit tests. TDD emphasizes writing tests before writing the actual code, ensuring that the code meets the expected requirements. This approach enables continuous testing and refining of code, accommodating changes or updates in the requirements and enhancing adaptability and flexibility in the software development lifecycle.
In the context of BDD, a testing framework, like Cucumber, that supports BDD principles can be used. With Cucumber, tests are written in a human-readable format using Given-When-Then statements, expressing the behavior of the system under test. This bridges the gap between business requirements and technical implementation, making tests more understandable and maintainable. Furthermore, Cucumber allows for collaboration between stakeholders and developers, contributing to test creation in a common language.
A practical example of adapting to changing requirements can be found in non-destructive schema changes in a database. This incremental process, aimed at maintaining application stability while avoiding an inadequate schema, entails adding to the schema without removing any columns or tables, creating an abstraction layer, and using feature flags to conditionally write to the new schema. The new schema is then populated with data from the old one, verified to be in sync with the old schema, and updated to return data from the new schema. The finished product is cleaned up to ensure the application still works, no longer writes to/reads from the old schema, and deletes feature flags and references to the old schema.
Approval testing is another technique that mitigates the risk of code changes by managing changes to system behavior. Unlike traditional assertion-based testing, approval testing captures and stores the behavior of the system and allows for behavior changes to be approved, rejected, or ignored. This human-centered approach replaces upfront effort with iterative adjustment and has a built-in definition of done. It is particularly useful for testing legacy code.
To conclude, adapting to changing requirements in unit testing can be achieved through a combination of robust testing frameworks, parameterized tests, mock objects, test-driven development, behavior-driven development, non-destructive schema changes, and approval testing. These techniques ensure easy modification of tests, minimize the risk of code changes, and ensure tests are in line with the current software requirements. Regularly updating tests further ensures that they cover all necessary scenarios and edge cases, providing comprehensive test coverage, and improving overall testability
8. Balancing Workload Management and Deadlines without Compromising on Quality
Unit testing is a critical aspect of software development, but it often presents a challenging task of balancing workload, meeting deadlines, and ensuring the delivery of high-quality software. Managing workload effectively plays a central role in addressing these challenges. It involves strategic planning, assigning tasks, and ensuring balanced distribution of work among team members to avoid burnout and maintain productivity.
Workload imbalance can often lead to productivity decline and dissatisfaction within the team. Therefore, fostering a collaborative environment and proper task delegation can enhance workplace satisfaction and productivity, benefiting the team as a whole and positively impacting the company's overall performance.
A comprehensive approach to workload management involves identifying all tasks that need completion, assessing the team's capacity, prioritizing and trimming tasks, communicating frequently within the team, and helping team members improve their time management skills. Additionally, discouraging extended work hours, reassigning tasks, setting achievable deadlines, and leveraging tools like Machinet for automating unit test generation can streamline the process.
Indicators of workload management issues may include team members expressing dissatisfaction with the volume of work, missed deadlines, communication problems, signs of burnout, and lack of interest in the tasks at hand. Promptly addressing these issues can enhance productivity and morale.
Studies show that the average employee has about three hours of productive work per day. Overworking led to over 745,000 deaths in a single year, according to the World Health Organization. Thus, effective workload management is crucial to prevent burnout and improve productivity.
In the sphere of unit testing, integrating testing into the development process, utilizing automated testing tools, and prioritizing tests based on the complexity and criticality of the code can significantly enhance test coverage. This not only ensures high-quality tests but also saves time, thereby improving the overall productivity and efficiency of the team.
Here are some techniques for managing workload and deadlines in unit testing:
- Prioritize your testing tasks based on their importance and impact on the overall system. This can help you allocate your time and resources effectively.
- Break down your testing tasks into smaller, manageable chunks to make them easier to complete within the given deadlines.
- Utilize automation tools and frameworks to streamline your testing process and save time. These tools can help you automate repetitive tasks and execute tests more efficiently.
- Regularly update the team on the progress of testing, identify and resolve any bottlenecks or dependencies, and seek assistance when needed to ensure that testing tasks are completed on time.
Incorporating testing into the development process can greatly improve workload management. By implementing unit testing practices, developers can identify and fix issues early on, reducing the time spent on debugging later in the development cycle. This helps to ensure that the workload remains manageable and that the development process runs smoothly. Additionally, following best practices for unit testing in Java can further enhance testing efforts and streamline the workload management process.
Automated testing tools, such as JUnit, NUnit, TestNG, and Selenium, are available to streamline and optimize the unit testing process. These tools provide features like test case management, test execution scheduling, and reporting, automating repetitive tasks and offering insights into test coverage and results.
When prioritizing tests based on code complexity and criticality in unit testing, it is crucial to consider various factors. Start by identifying the critical parts of the codebase and prioritize testing for those areas. This can be done by analyzing the requirements and understanding which parts of the code are essential for the overall functionality of the system. Areas with more complex logic or dependencies may require more thorough testing. By prioritizing tests based on code complexity and criticality, developers can ensure that the most important parts of the code are thoroughly tested, and any potential issues are identified early in the development process.
Machinet is a platform that provides automation capabilities for generating unit tests. It is capable of automating the generation of unit tests for various programming languages, including Java. By leveraging Machinet, developers can streamline the process of creating unit tests, saving time and effort. The platform offers best practices and techniques for unit testing, helping developers ensure the quality and reliability of their code. With Machinet, developers can automate the generation of unit tests and improve the overall efficiency of their software development process
Conclusion
In conclusion, achieving high unit test coverage and effectively managing technical debt and legacy code are crucial for ensuring the reliability and quality of software applications. By implementing strategies such as using code coverage analysis tools like JaCoCo, writing comprehensive test cases, and practicing test-driven development, developers can enhance their unit test coverage. Additionally, writing testable code, utilizing mocking frameworks like Mockito, and refactoring code to improve testability contribute to improving unit test coverage. These practices not only enhance the quality and dependability of software but also serve as a form of documentation for different components of the codebase.
The ideas discussed in this article have broader significance in the field of software development. Achieving high unit test coverage is essential for building robust and reliable software applications. It helps in early bug detection, improves code quality, and enhances the overall maintainability of the codebase. By effectively managing technical debt and legacy code, developers can minimize issues caused by outdated or complex code structures. This leads to a more efficient testing process and allows for easier adaptation to changing requirements. Overall, implementing these best practices enables developers to deliver high-quality software while balancing workload management and meeting project deadlines.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.