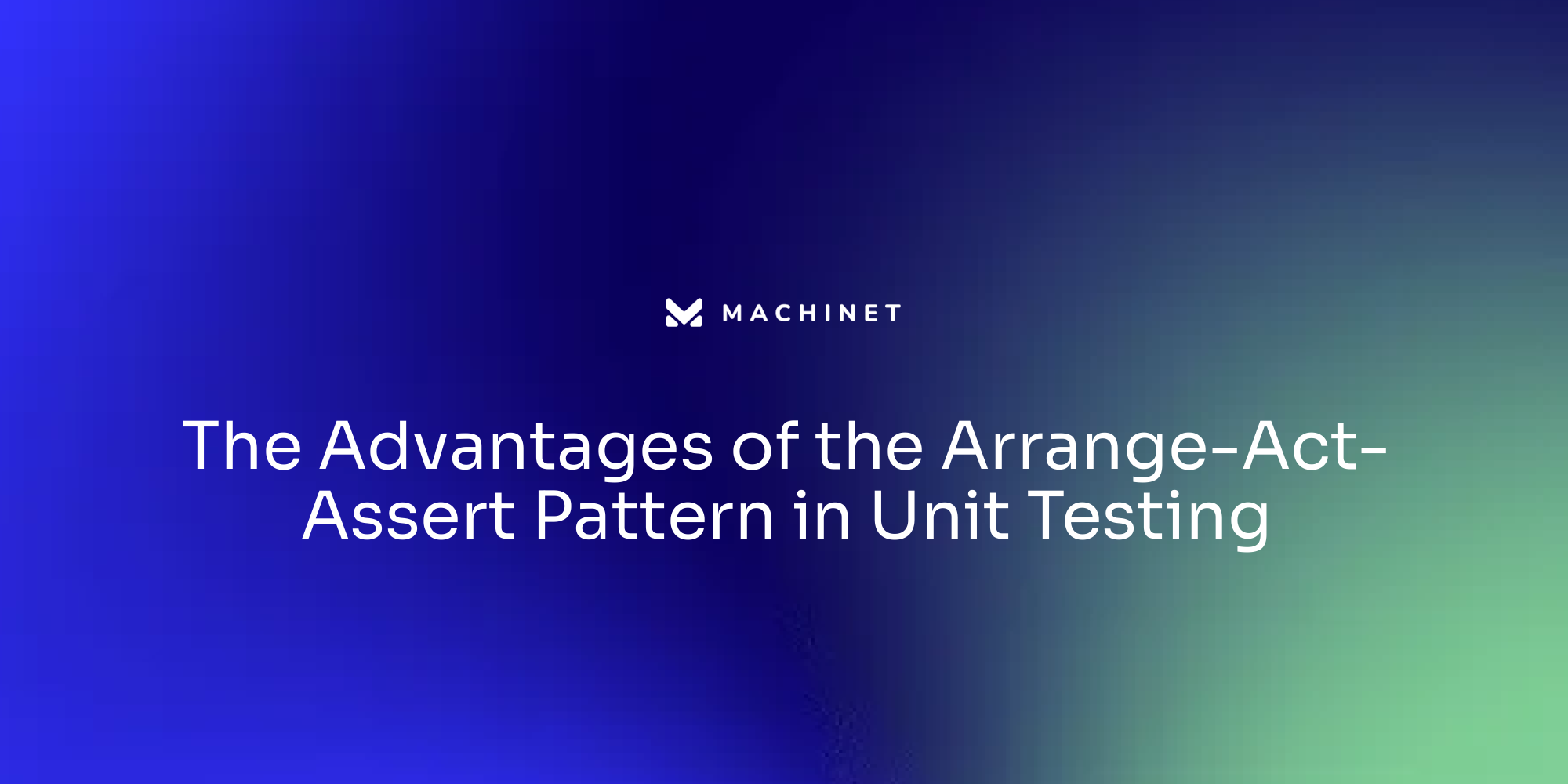
Table of Contents
- Understanding the Arrange-Act-Assert (AAA) Pattern
- How AAA Testing Improves Unit Testing Processes
- Key Components of the AAA Pattern: Arrange, Act, Assert
- Practical Application: A Unit Test Scenario Using AAA Pattern
- Refactoring Tests with the AAA Pattern for Better Readability and Maintenance
- Comparing AAA Testing to Other Testing Approaches: Why Choose AAA?
- Addressing Common Challenges in Unit Testing with the AAA Pattern
- Maximizing Test Coverage with the AAA Pattern
Introduction
The Arrange-Act-Assert (AAA) pattern is a structured and effective approach to unit testing in software development. This pattern divides the testing process into three distinct phases: Arrange, Act, and Assert. In the Arrange phase, the test environment is set up by initializing variables, creating objects, and configuring the necessary conditions. The Act phase executes the specific functionality being tested, and the Assert phase verifies the expected outcome of the test.
The AAA pattern brings clarity and order to unit tests, making them more readable, maintainable, and easier to understand. It promotes a focused testing approach by testing one functionality at a time, avoiding complex test scenarios. By adhering to the AAA pattern, developers can enhance test coverage, identify gaps in testing, and ensure the delivery of high-quality software products. In this article, we will explore the benefits of the AAA pattern, its practical application in unit testing, and how it addresses common challenges in the testing process. We will also discuss real-world examples and success stories that highlight the effectiveness of the AAA pattern in maximizing test coverage
1. Understanding the Arrange-Act-Assert (AAA) Pattern
Unit testing is an integral part of software development, ensuring the final product is reliable and free from errors. The Arrange-Act-Assert (AAA) pattern is a highly effective strategy used during this process, divided into three distinct, interconnected phases.
The initial phase, 'Arrange', sets the foundation for the test. Tasks in this phase may include initializing variables, creating objects, or setting up mocks. The aim is to create the ideal conditions for the test to operate at its peak.
The second phase, 'Act', is when the functionality under examination is put into motion. The code or feature being tested is executed during this phase, bringing the test to life.
The concluding phase, 'Assert', evaluates the outcome of the 'Act' phase. This step verifies if the result matches the expected output.
Jay Cruz, a well-known figure in the software testing community, has said, "Testing is an essential part of building software," and "The AAA pattern provides simple yet effective steps for testing our code." Timothy Foster shares these sentiments, stating, "I love the AAA pattern. I use it often when teaching to devs unfamiliar with testing."
One could see the AAA pattern in action when testing a user model in JavaScript, for instance. The 'Arrange' step creates an instance of the user class. The 'Act' step calls the getfullname method, and the 'Assert' step verifies if the result is accurate.
This pattern is not just a recommendation, but a standard that all types of testing, including unit tests, should follow. As Rich Edwards, a software testing enthusiast, points out, "All testing needs to follow this pattern. It's alarming how often tests are written without any assertion/validation of results."
The AAA pattern, when used in conjunction with testing frameworks like Jasmine, ensures that the tests are well-structured and easy to read.
Try Machinet to automate your unit tests and improve code quality!
It eliminates ambiguity and encourages the use of concrete values in tests, as Gabirieli Lalasavan suggests, "In a real-world scenario, adding uncertainty if the test breaks is not ideal. The test should have concrete values."
Lou Cyx also emphasizes, "The internal state isn't relevant to unit testing, just the surface of contact with the external world." This underlines the importance of the AAA pattern in keeping unit tests focused on external interactions rather than internal states.
To write clear and concise "arrange-act-assert" sections in unit testing, a few practical tips should be followed:
- Keep the arrange section focused: Only include the necessary setup code in the arrange section to prepare the test environment. Avoid adding any unnecessary or redundant code.
- Use meaningful variable and method names: Opt for descriptive names for variables and methods in the arrange section. This makes it easier to comprehend the purpose of each step in the test.
- Keep the act section simple: The act section should only contain a single method or action that you are testing. Avoid adding multiple actions or complex logic in this section.
- Use clear and concise assertions: The assert section should clearly state the expected outcome of the test. Use precise assertions to check the results of the test and avoid adding unnecessary assertions.
By following these tips, unit tests following the "arrange-act-assert" pattern will be easier to understand and maintain.
The AAA pattern thus provides a structured, effective, and universally applicable approach to unit testing, ensuring the delivery of reliable, high-quality software
2. How AAA Testing Improves Unit Testing Processes
The Arrange-Act-Assert (AAA) pattern is a formidable tool in the unit testing landscape. This pattern brings clarity and order to unit tests, improving their understandability and readability. Its benefits extend beyond the developers who draft initial tests, aiding other team members who may need to comprehend or modify these tests in the future.
The AAA pattern promotes the practice of testing individual functionalities in isolation, facilitating the creation of sturdy and reliable tests. This in turn enhances the software development process's overall quality. When a test encounters failure, the AAA pattern enables easy diagnostics, pinpointing the exact section of the test that faltered, offering a clear insight into the code's problematic area.
The AAA pattern's efficiency is apparent when testing expected exceptions. It ensures thorough testing of both the normal operation of a method (the happy path) and its failure conditions (the sad paths). This is especially significant when a method might throw custom domain exceptions or when guard clauses are employed to ensure input expectations are met.
The AAA pattern's functionality can be expanded by using actions, which are operations expected to throw exceptions. This suggestion, made by Shady Nagy, has been observed to make exception tests cleaner. It allows the operation that is the focus of the test to stand alone in the 'Act' section, simplifying the test's readability and follow-through.
FluentAssertions offers a more expressive method of writing assertions, in addition to built-in assertions. It provides a novel approach to testing exceptions, enhancing the clarity of tests.
The AAA pattern, when paired with custom test data builders and asserts, can be even more beneficial. Builder patterns for setting up test data can simplify the creation of complex objects, offering customization and reducing the potential for technical debt in unit test code. Likewise, custom asserts can simplify complex checks and provide more informative error messages in tests.
When used with these practices, the AAA pattern can offer a thorough and efficient approach to unit testing. It aids not only in identifying issues in the code under test but also contributes to the delivery of high-quality software products
3. Key Components of the AAA Pattern: Arrange, Act, Assert
The Arrange-Act-Assert (AAA) pattern, a cornerstone of well-structured unit tests, consists of three integral components. In the 'Arrange' phase, the groundwork for the test is laid by setting up the requisite objects and variables. The 'Act' phase then takes the lead, executing the function under scrutiny. Lastly, the 'Assert' phase validates the results, ensuring the output aligns with anticipated outcomes.
Distinct separation of these components is crucial for improving readability and clarity of the test code. This not only enhances the understanding of the test, but also aids in identifying the failing section if an issue arises.
The AAA pattern aligns perfectly with the principles of Test-Driven Development (TDD). TDD is a cycle that emphasizes defining the behavior of the code through tests before its implementation, thus reducing the likelihood of bugs and ensuring the code behaves as expected.
One popular framework for .NET applications, XUnit, supports the AAA testing and TDD principles. It offers various methods for making assertions in tests, such as type, null, equality, and exceptions. It also introduces the concept of theories that allow testing with multiple values.
The AAA pattern also enables testing collections using methods like assertEmpty and assertSingle. An attribute can be added to namespaces to test internal methods, thereby granting access to internal properties/methods from a different specified project.
In the initial phase of TDD, it's recommended to create the class and define its properties and methods, but not implement them. Instead, have the methods throw a NotImplementedException, leading to test failure. This approach aligns with the AAA testing method, where a failure in the initial phase (Arrange) can be an indication of a missing or incorrect setup.
The test methods' naming convention, reflecting the structure of the source code projects, further enhances the organization and readability of the tests. This systematic approach to testing, backed by the AAA pattern, ensures the delivery of robust, high-quality software products.
Let's take an example. Consider a scenario where an Azure client is used to find expiring secrets. The 'Arrange' phase would involve setting up the Azure client and defining the criteria for the secrets to be found. The 'Act' phase would then execute the function to find the expiring secrets. Finally, the 'Assert' phase would verify that the secrets returned by the function meet the defined criteria.
While the AAA pattern provides a robust structure for writing tests, it's the disciplined application of this structure that leads to effective and reliable unit tests. Troubleshooting failed 'arrange', 'act', or 'assert' steps in unit testing requires thorough examination of the test environment, test data, and the code being tested. It may be helpful to use debugging tools or techniques to inspect the execution and identify any potential issues. By following this "arrange-act-assert" pattern in unit tests, the effectiveness of the testing process can be greatly enhanced, leading to improved quality of the code
4. Practical Application: A Unit Test Scenario Using AAA Pattern
The AAA pattern, a well-structured approach for creating robust and effective unit tests, involves three key steps: Arrange, Act, and Assert. This pattern can be leveraged to test a variety of scenarios, from simple functions to more complex cases involving custom domain exceptions or guard clauses.
Let's illustrate this with an example of a function calculating the area of a rectangle. In the Arrange step, critical parameters such as the rectangle's length and breadth are defined, laying the groundwork for the action to follow.
The Act stage is where we execute the function with the parameters defined in the Arrange step. This is the core operation of the test, where the function under scrutiny is invoked with the prearranged parameters.
The Assert stage is where the output of the function is compared with the expected result. This step verifies that the function is behaving as expected, serving as a crucial checkpoint.
When dealing with more intricate scenarios, such as methods that may throw custom domain exceptions or use guard clauses to ensure method inputs comply with expectations, Xunit's Assert.Throws<T>
method can be utilized to test for expected exceptions.
In cases where the Assert.Throws<T>
line might become overly long, an action representing the operation expected to throw an exception can be beneficial for readability.
FluentAssertions is an alternative assertion library that can be used in place of built-in assertions of the testing library. In certain circumstances, using a local function instead of an action can further simplify the test code.
Unit tests are essential for verifying the behavior of methods that throw exceptions under specific circumstances. When properly applied, the AAA pattern can significantly enhance the clarity and effectiveness of these tests. This pattern not only aids in structuring the unit tests but also enhances the readability and maintainability of the test code
5. Refactoring Tests with the AAA Pattern for Better Readability and Maintenance
Refactoring tests to adhere to the Arrange-Act-Assert (AAA) pattern significantly improves their clarity and maintainability. This pattern, which partitions tests into 'Arrange', 'Act', and 'Assert' segments, enables developers to quickly comprehend the operations and purpose of each test. This comprehension is particularly beneficial in large codebases where understanding each test's role and functionality can be complex. Furthermore, the AAA pattern makes maintaining and modifying tests more straightforward, as the structure offers a clear guide for any necessary changes.
In automated testing, the AAA pattern is a key principle for creating maintainable code. It acts as a guiding checkpoint throughout the development process, providing reliable feedback on code functionality. Adhering to this pattern enhances test effectiveness and reliability.
Maintainability in automated tests is crucial as it allows software solutions to be easily customized and adapted to meet new requirements or fix existing issues. The AAA pattern emphasizes test readability, a key attribute of high-quality automated tests. Code that is easy to read is also easier to understand and maintain, reducing the potential for bug introduction. It's worth noting that comments should not replace readable code.
Furthermore, API usability, the ease of understanding and using a software library's methods, is another key attribute of high-quality automated tests. The AAA pattern also promotes extensibility, allowing a shared library to be customized to fit different contexts and needs, and it simplifies the learning curve, making it easier for new team members to learn and use a test automation framework.
The AAA pattern is a prescribed solution to software challenges that groups and reuses logic to improve maintainability. It is a key concept in the book "Design Patterns for High Quality Automated Tests", which focuses on optimizing and stabilizing flaky tests, and improving test readability, maintainability, reusability, and extensibility through design patterns, principles, and best practices. By employing the AAA pattern, developers can enhance the readability, maintainability, and effectiveness of their unit tests, ultimately leading to higher-quality software products.
To improve test readability, it is crucial to follow patterns and best practices such as the AAA pattern. The Arrange phase involves setting up the necessary preconditions for the test, including initializing objects and setting up test data. The Act phase is where the actual test action is performed, such as calling a method or executing a specific behavior being tested. The Assert phase is used to verify the expected outcome of the test, typically involving a comparison of the actual result with the expected result using assertions or assertion libraries.
To update tests easily with the AAA pattern, follow these steps:
- Arrange: Set up the necessary preconditions for the test, including initializing variables, creating objects, and configuring the environment.
- Act: Perform the action or method that you want to test. This could be calling a function, invoking a method, or interacting with the system under test.
- Assert: Verify the expected outcome of the action. Check if the result matches the expected behavior or if the system is in the desired state.
By adhering to the AAA pattern, you can separate the different stages of the test, making it easier to understand and maintain. This pattern promotes clear organization and readability, allowing for quick updates to tests when necessary
6. Comparing AAA Testing to Other Testing Approaches: Why Choose AAA?
The Arrange-Act-Assert (AAA) pattern is a key player in the world of unit testing due to its simplicity and effectiveness. It eradicates the need for intricate setup or teardown procedures that are characteristic of other methods. Designed to be uncomplicated and easy to implement, the AAA pattern is a fundamental tool for any development team.
In unit testing, it's not enough to test merely the expected working condition, often dubbed the 'happy path'. It's also vital to assess various failure conditions, or 'sad paths'. This includes testing for exceptions, which becomes notably crucial when dealing with methods that may throw custom domain exceptions or when employing guard clauses to ensure method inputs are up to par.
Renowned testing frameworks like NUnit and xUnit offer methods for testing anticipated exceptions, with xUnit's Assert.Throws being a popular choice. The AAA testing pattern is a conventional approach in these frameworks, where tests are structured as public classes with a Fact method. However, it's not rare to see the Act step also include an assertion. This can make the line excessively long and more challenging to read.
To stick to the AAA pattern and maintain uncluttered test code, developers can use an action to represent the operation that is expected to throw an exception. For a more expressive syntax for testing exceptions, FluentAssertions, an alternative to built-in assertions, can be employed. Another strategy is to use a local function as opposed to an action, which can further streamline the test code. The xUnit test framework is acclaimed for its robust support for testing exceptions, and employing actions can contribute to cleaner tests.
Examples and case studies have delineated how to effectively leverage the AAA pattern. For instance, xUnit offers an Assert.Throws method to test for expected exceptions in unit tests. Similarly, NUnit 3 has a comparable method for testing expected exceptions. These examples illustrate how actions can be used to represent the operation that is expected to throw an exception, leading to cleaner and more digestible tests.
The AAA pattern, with its distinct separation of setup, action, and verification, simplifies test maintenance.
It boosts productivity and code quality, making it a valuable asset for any development team. By using the AAA pattern, developers can create comprehensive and dependable unit tests, ultimately facilitating the delivery of high-quality software products
7. Addressing Common Challenges in Unit Testing with the AAA Pattern
The Arrange-Act-Assert (AAA) pattern stands as a lighthouse in the vast ocean of unit testing, providing a clear path to navigate the common testing challenges. One of these challenges is the lack of a clear structure in tests, making them difficult to maintain and understand. The AAA pattern, with its distinct phases of arrangement, action, and verification, brings order to this chaos. Tests that follow this pattern are more readable and manageable, thus making maintenance considerably easier.
The AAA pattern also advocates for a focused testing approach, emphasizing the testing of a single functionality per test. This aspect is vital as it helps avoid test complexity. Often, tests become intricate and difficult to debug when they attempt to test multiple functionalities at once. By emphasizing testing one functionality at a time, the AAA pattern ensures that tests remain clear and straightforward, making the debugging process less intimidating.
In the world of software development, automated tests play a crucial role in maintaining code and identifying bugs. They act as reliable checkpoints, consistently providing feedback on the code's functionality. Writing effective tests can be challenging, but it is essential for ensuring code quality. In this scenario, the AAA pattern emerges as a recommended structure for tests, enhancing their readability and making debugging easier.
Moreover, the practice of extracting setup code into separate functions promotes code reuse and leads to cleaner test code. Tests should be simple, focusing on the user perspective, and avoid testing implementation details to ensure robustness and survival of code refactors. Proper naming of tests, including the application state, actions, and expected outcomes, enhances clarity and comprehensibility.
To refactor tests using the AAA pattern, a structured approach is necessary. The 'Arrange' phase involves setting up the test environment by initializing objects, setting up dependencies, and preparing the necessary data. The 'Act' phase involves invoking the method or performing the action that is to be tested. This step represents the actual behavior that is to be verified. The 'Assert' phase involves verifying the outcome or the state of the system under test, checking if the expected results match the actual results.
By following the AAA pattern, tests become more readable, maintainable, and easier to debug. It helps separate the different concerns of a test and makes it clear what is being arranged, what action is being taken, and what assertion is being made. Remember to keep tests focused and independent. Each test should test a specific behavior or scenario, and avoid unnecessary dependencies or interactions with external resources.
Embracing the AAA pattern leads to more readable and manageable tests, addressing common unit testing challenges. It promotes simplicity and clarity, which in turn, assists in maintaining code quality and ensuring the successful delivery of high-quality software products
8. Maximizing Test Coverage with the AAA Pattern
The Arrange-Act-Assert (AAA) pattern is a critical tool in the software engineer's arsenal to maximize test coverage. This pattern underscores the importance of testing individual functionalities within each test, fostering a meticulous scrutiny of every function or method. The result is a broader and more comprehensive test coverage, ensuring that every part of the code undergoes appropriate testing.
The AAA pattern's systematic structure also simplifies the identification of any existing gaps in the test coverage. This, in turn, facilitates continuous enhancement of the testing process, making it more efficient and effective. Such a process is pivotal in software development, as it guarantees that each code piece is tested and functional, thereby minimizing errors or bugs in the final product.
The potency of the AAA pattern is evident in its application across various large-scale projects. For instance, Avant, an online lending platform, successfully streamlined their testing process using the AAA pattern. They run about 25 million tests daily, and have significantly cut down their end-to-end build times, courtesy of the AAA pattern. This structured approach has allowed them to optimize their build times, enhance productivity, and reduce infrastructure costs.
Similarly, Pattern, a company offering a wide range of services including brand protection and e-commerce consulting, has reaped the benefits of the AAA pattern. The implementation of the AAA pattern led to a substantial increase in revenue within the initial year. Moreover, they received positive feedback from clients who commended their meticulous approach and commitment, further highlighting the advantages of the AAA pattern.
To truly capitalize on the benefits of the AAA pattern, it is crucial to adhere to the following steps:
- Arrange: Establish the necessary preconditions for the test by initializing objects, setting values, and configuring any required dependencies.
- Act: Execute the specific action or method being tested. This could involve invoking a method, executing a certain behavior, or interacting with the system in some manner.
- Assert: Validate the expected outcome of the test by comparing the actual results with the expected ones. This could involve checking return values, validating state changes, or asserting certain conditions.
By adhering to the AAA pattern, you can ensure each test is well-structured, clear, and comprehensive. This pattern aids in organizing test cases, making them easier to understand, maintain, and debug. Thus, the AAA pattern's structured approach not only fosters thorough testing but also aids in identifying gaps in test coverage. This leads to ongoing enhancements in the testing process, guaranteeing the delivery of high-quality software applications. The success stories of companies like Avant and Pattern underscore the effectiveness and efficiency of the AAA pattern in maximizing test coverage
Conclusion
The Arrange-Act-Assert (AAA) pattern is a structured and effective approach to unit testing in software development. This pattern divides the testing process into three distinct phases: Arrange, Act, and Assert. The AAA pattern brings clarity and order to unit tests, making them more readable, maintainable, and easier to understand. It promotes a focused testing approach by testing one functionality at a time, avoiding complex test scenarios. By adhering to the AAA pattern, developers can enhance test coverage, identify gaps in testing, and ensure the delivery of high-quality software products.
The benefits of the AAA pattern extend beyond the developers who write initial tests. It aids other team members who may need to understand or modify these tests in the future. The AAA pattern simplifies test maintenance by providing a clear structure that helps identify failing sections of tests and facilitates easy diagnostics. It also promotes the practice of testing individual functionalities in isolation, ensuring sturdy and reliable tests. The AAA pattern is widely applicable and can be used with various testing frameworks like Jasmine for JavaScript or NUnit for .NET applications.
To boost your productivity with Machinet, experience the power of AI-assisted coding and automated unit test generation.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.