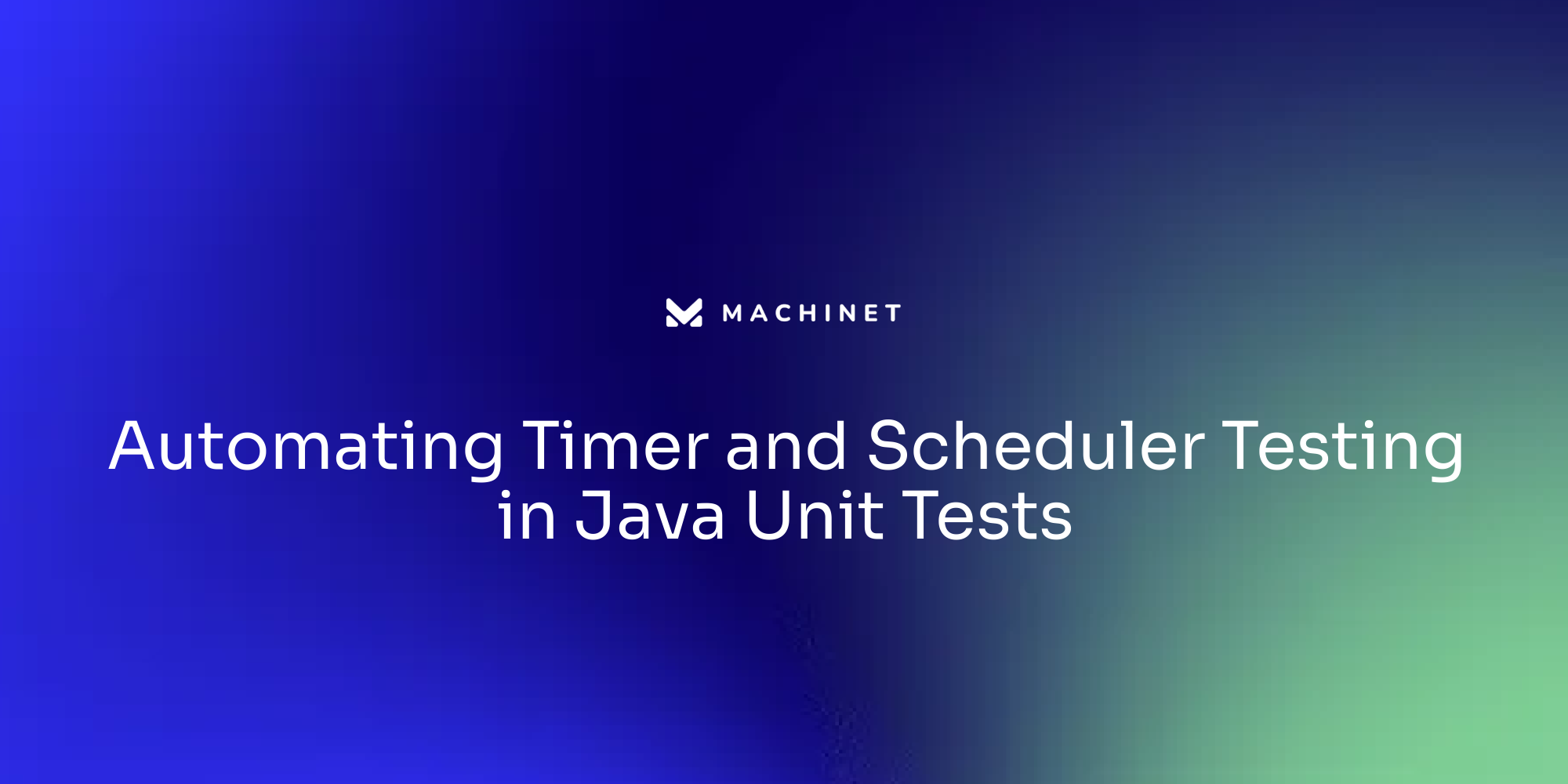
Table of Contents
- Understanding the Basics of Timer and Scheduler in Java
- The Role of Unit Testing in Validating Timer and Scheduler Functionality
- Implementing Java Timer in Unit Tests
- Scheduling Tasks for Execution: A How-To Guide for Unit Tests
- Techniques to Stop the Thread of the TimerTask Inside Run During Testing
- Automating Timer and Scheduler Testing: Tools and Frameworks
- Handling Changing Requirements in Timer and Scheduler Testing
- Best Practices for Managing Workload and Balancing Deadlines in Test Automation
Introduction
Understanding the basics of Timer and Scheduler in Java is essential for developers working with time-dependent code. Java provides built-in classes like Timer and TimerTask that allow scheduling tasks for future execution in a background thread. These tasks can be executed once or repeatedly at regular intervals. In this article, we will explore how to implement Timer and TimerTask in Java, schedule tasks for execution, and handle exceptions. We will also discuss the benefits of using the more modern alternative, ScheduledExecutorService and ScheduledFuture, for scheduling tasks. By mastering these concepts, developers can effectively manage time-dependent code and optimize their software applications.
In addition to understanding the basics of Timer and Scheduler, it is crucial to validate the functionality of these components through unit testing. In another article, we will explore the role of unit testing in validating Timer and Scheduler functionality. We will discuss best practices for unit testing time-dependent code, techniques for handling changing requirements in testing, and tools and frameworks available for automating timer and scheduler testing. By following these best practices and utilizing the right tools, developers can ensure the reliability and accuracy of their timer and scheduler implementations
1. Understanding the Basics of Timer and Scheduler in Java
Java offers a variety of tools and strategies for handling time-dependent code in unit tests.
Try Machinet to automate code generation and unit testing in Java!
One approach is to use the built-in Timer and TimerTask classes, which provide a mechanism for scheduling tasks for future execution in a background thread. The Timer class, which is thread-safe and can be shared among many threads, handles the task scheduling, while TimerTask, an abstract class, encapsulates the task to be executed at a specified time. These tasks can be scheduled for one-time execution or repeated execution at regular intervals.
To implement Timer and TimerTask, you would first import the necessary classes from the java.util package. Then, create instances of the Timer and TimerTask classes, with the TimerTask instance containing the logic you want to execute. Once your TimerTask instance is created, you can schedule it for execution using the schedule method of the Timer class and then start the Timer using the start method.
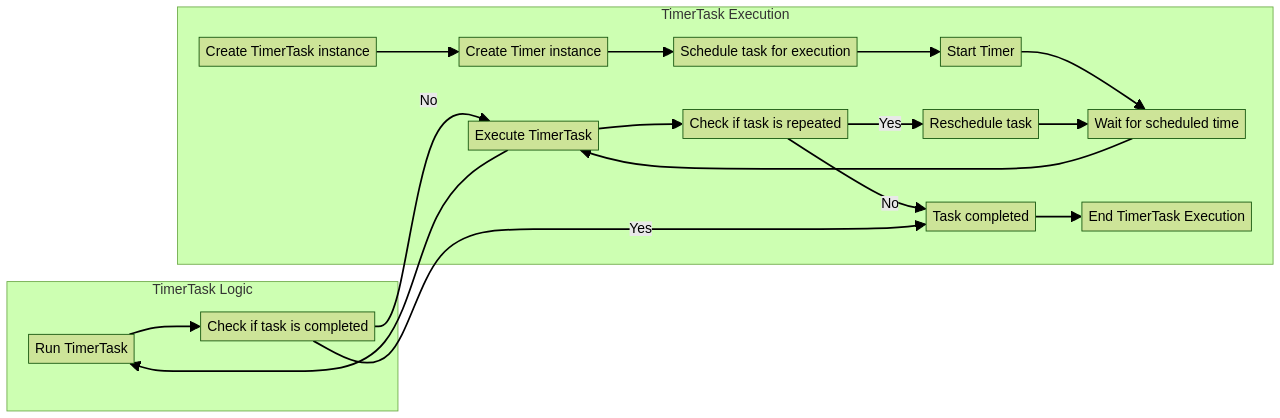
Here's an example:
```javaimport java.util.Timer;import java.util.TimerTask;
public class TimerExample { public static void main(String[] args) { Timer timer = new Timer();
TimerTask task = new TimerTask() {
@Override
public void run() {
// Insert your logic here
System.out.println("TimerTask executed!");
}
};
// Schedule the TimerTask to execute after a delay of 1 second
timer.schedule(task, 1000);
// Start the Timer
timer.start();
}
}```
In this example, the TimerTask will be executed after a delay of 1 second. You can modify the delay as per your requirements. Be sure to handle any exceptions that may be thrown by the Timer and TimerTask classes, such as IllegalArgumentException or IllegalStateException, based on your specific use case.
Java also provides a more modern and preferred alternative to Timer and TimerTask in the form of ScheduledExecutorService and ScheduledFuture.

The ScheduledExecutorService interface extends ExecutorService, supporting both delayed and periodic execution of tasks, as opposed to ExecutorService that only supports one-time task execution.
The ScheduledExecutorService introduces new methods to create tasks with delay and periodicity properties. It returns an instance of the ScheduledFuture interface for every method call. The ScheduledThreadPoolExecutor class, which extends ThreadPoolExecutor and implements ScheduledFuture, can run multiple tasks periodically in parallel, depending on the pool size. It uses an unbounded queue and acts as a fixed-sized pool using core pool size threads.
An example project, utilizing JDK 1.8 and Maven 3.04, demonstrates scenarios with single or multiple tasks submitted to a ScheduledThreadPoolExecutor instance. This project includes source code files and a pom.xml file.
To illustrate, consider an AlarmClock class that uses ScheduledExecutorService to run a simple task once every second, starting 3 seconds from now, and canceling the task after 20 seconds. Alternatively, using the older Timer and TimerTask classes, a task can be performed once a day at 4 am, starting the following morning.
Whether using the older Timer and TimerTask classes or the more modern ScheduledExecutorService and ScheduledFuture, Java provides robust support for scheduling tasks for future execution in a background thread. Always remember to cancel the Timer when you're done with it to release system resources. This allows you to schedule tasks to be executed at a specific time or after a specified delay using Timer and TimerTask in Java
2. The Role of Unit Testing in Validating Timer and Scheduler Functionality
Unit testing is a critical tool for validating the functionality of Java's Timer and Scheduler components.
Boost your Timer and Scheduler testing with Machinet's AI-powered unit testing capabilities!
It enables the assessment of the performance of the Timer and TimerTask classes under various conditions, which involves verifying the functionality of methods such as schedule(), cancel(), and purge(). This early verification process plays a crucial role in identifying potential issues during the development cycle, thereby saving time, effort, and resources that might be spent addressing these problems later on.
An obstacle that developers often encounter when performing unit tests is managing asynchronous code, particularly when using operators like interval, debounce, or timer. Marble testing, an innovative approach for testing asynchronous code, addresses this challenge by providing a readable and time-efficient testing mechanism. This technique is based on the TestScheduler concept in RxJS, which organizes activities in time and controls the order of event emissions, thus creating a more structured and manageable testing environment.
The ability to manipulate time is a crucial aspect of testing asynchronous code. Dart's Quiver package offers the FakeAsync class, which allows developers to control time within their code. This class is frequently used in Flutter's widget testing library to manage the passage of time, making the testing process more manageable and developer-friendly. The FakeAsync class includes methods like flushMicrotasks
and elapse
, which enable developers to control the timing of events in unit tests.
When writing unit tests for the schedule() method in the Timer class, various scenarios and edge cases should be considered. This could include verifying that the method correctly schedules a task to run at a specific time, ensuring that the scheduled task is executed as expected, and confirming that the method can handle exceptions or errors gracefully. Additionally, it would be beneficial to test the method's handling of recurring tasks and its ability to manage delays and intervals correctly, if applicable.
Despite the lack of specific context information on unit testing frameworks and tools for timer and scheduler in Java, these testing methodologies and tools, when effectively utilized, can significantly enhance the efficiency and accuracy of unit tests. This, in turn, ensures robust validation for Timer and Scheduler functionality in Java
3. Implementing Java Timer in Unit Tests
Incorporating a Java Timer into unit tests necessitates the creation of both a Timer and TimerTask instance.
Save time and effort in Timer and TimerTask testing with Machinet's automated code generation!
The latter is specifically engineered to override the run() method, thereby defining the task execution. The Timer instance's schedule() method is then called upon to arrange the execution of the TimerTask. This method accepts both the TimerTask instance and the delay, measured in milliseconds, as parameters. The expected and actual results are compared after the task execution.
It's crucial to remember that timing can lead to non-determinism, a prevalent cause of unstable tests, which can undermine the code's reliability. Controlling the testing clock can help alleviate this problem. This is reflected in the advice given by software engineer Mark Justin Waks, who suggests controlling your clock and avoiding the use of instant.now for anything other than logging. He recommends using a dependency-injected clock mechanism for testing instead.
Testing for non-events or nothingness is essential and should not be overlooked. As Waks points out, waiting for a non-event is always non-deterministic. In this regard, it can be beneficial to add code to your system solely for the benefit of the test harness to signal 'I decided not to do anything' and listen for that in your test.
Race conditions can also lead to defects. Specific hooks can be added to test and manage threads during testing. Waks emphasizes that "You need to write your code specifically to make it testable."
Lastly, when dealing with tests involving service API clients, a mock server can be instrumental in simulating network latency and timeouts. For instance, testing the Spring WebClient with MockServer or configuring and testing timeouts with MockServer are practical real-world examples. However, it's worth noting that increasing timeouts is not a recommended solution for production code, but can be used for testing resilience mechanisms.
To implement a Java timer in unit tests, the Timer class provided in the Java standard library can be used. This class allows you to schedule tasks to run at specified intervals or after a delay. Here is an example of how a timer can be implemented in unit tests:
```javaimport java.util.Timer;import java.util.TimerTask;
public class TimerExample { public static void main(String[] args) { Timer timer = new Timer();
TimerTask task = new TimerTask() {
@Override
public void run() {
// Define the logic for the task to be executed
System.out.println("Task executed!");
}
};
// Schedule the task to run every 1 second
timer.scheduleAtFixedRate(task, 0, 1000);
// Sleep for some time to allow the timer tasks to execute
try {
Thread.sleep(5000);
} catch (InterruptedException e) {
e.printStackTrace();
}
// Cancel the timer
timer.cancel();
}
}```
In this example, the TimerTask's run() method is executed every 1 second. The timer is canceled after 5 seconds using the timer.cancel() method. You can tailor this example to suit your specific unit testing requirements
4. Scheduling Tasks for Execution: A How-To Guide for Unit Tests
Testing time-dependent code in unit tests, particularly those involving scheduled tasks, necessitates a comprehensive understanding of the available tools and methodologies. This can be a complex process, involving the creation of a Timer, defining a TimerTask, and applying the schedule() method. Testing asynchronous or time-dependent functionality can be facilitated by scheduling the execution of a particular code block or method at a specified time using the schedule()
method in a defined test case.
The scheduleAtFixedRate() method is a handy tool for executing tasks repeatedly, accepting the TimerTask, delay before the first execution, and period between successive executions as parameters. However, the context doesn't provide specifics on its use in unit tests. For verifying task execution times and intervals, understanding the specific task or functionality being tested is crucial. For instance, libraries like Mockito or PowerMock can be employed to control the timing of task execution, mock or stub time-related dependencies, and simulate time passage, ensuring tasks are executed at expected intervals.
Unit testing timer and timertask in Java can be achieved using various testing frameworks such as JUnit and Mockito. These frameworks offer the creation of mock objects and simulation of timer and timertask behaviors during unit tests, allowing for the verification of timer and timertask implementations in isolation.
The advent of Kotlin 1.6 brought significant changes to coroutine testing, including the TestDispatcher. A special CoroutineDispatcher variant, it allows more control over coroutine execution during testing. There are two main types of TestDispatchers: StandardTestDispatcher and UnconfinedTestDispatcher. The former ensures task execution order but requires explicit triggering, while the latter advances virtual time and executes tasks automatically, without guaranteeing execution order.
The TestCoroutineScheduler is responsible for scheduling and executing coroutines in the test environment, with methods like runCurrent, advanceTimeBy, and advanceUntilIdle available to control virtual time and task execution. However, endless coroutines can prevent test completion, making it essential to clean up any leaked coroutine jobs.
When testing time-dependent classes, traditional methods often result in slow, unreliable tests. However, packages such as nodatime and nodatimetesting can help alleviate these issues. For example, the nodatime package introduces the concept of time as a dependency, streamlining the testing process.
In conclusion, effectively scheduling tasks for execution in unit tests, especially time-dependent ones, requires a deep understanding of the tools and strategies available. Using the right tools and strategies can significantly boost the efficiency and reliability of your tests
5. Techniques to Stop the Thread of the TimerTask Inside Run During Testing
In the world of Java programming, the Timer class serves as an instrumental utility that facilitates the scheduling of a task to be executed at a future time. This class can be used to schedule a task for one-time execution or for repeated execution at regular intervals. The TimerTask, an abstract class that implements the Runnable interface, can only have one thread running at a time.
Consider a simple scenario where a Timer object is scheduled to execute a task every 10 seconds. The output of this program would print the start and finish times of the timer task. It's important to note that if a task is already executing, the Timer will wait until the task completes before initiating the next task.
A Timer object can be created to run associated tasks as a daemon thread. This is especially helpful when you want to halt the thread of a TimerTask within the run() method during testing. This can be accomplished by invoking the cancel() method of the TimerTask.
javaTimerTask task = new TimerTask() { @Override public void run() { // Your task logic here }};// To stop the task before it runs, call the cancel() method on the tasktask.cancel();
By using the cancel()
method on a TimerTask instance, you can prevent the TimerTask from scheduling further executions. However, the cancel()
method does not interrupt a task that is currently in execution.
To interrupt a task that is currently executing, the run() method should routinely check a boolean variable that indicates whether the task should continue executing. If this variable is set to false, the run() method should immediately return.
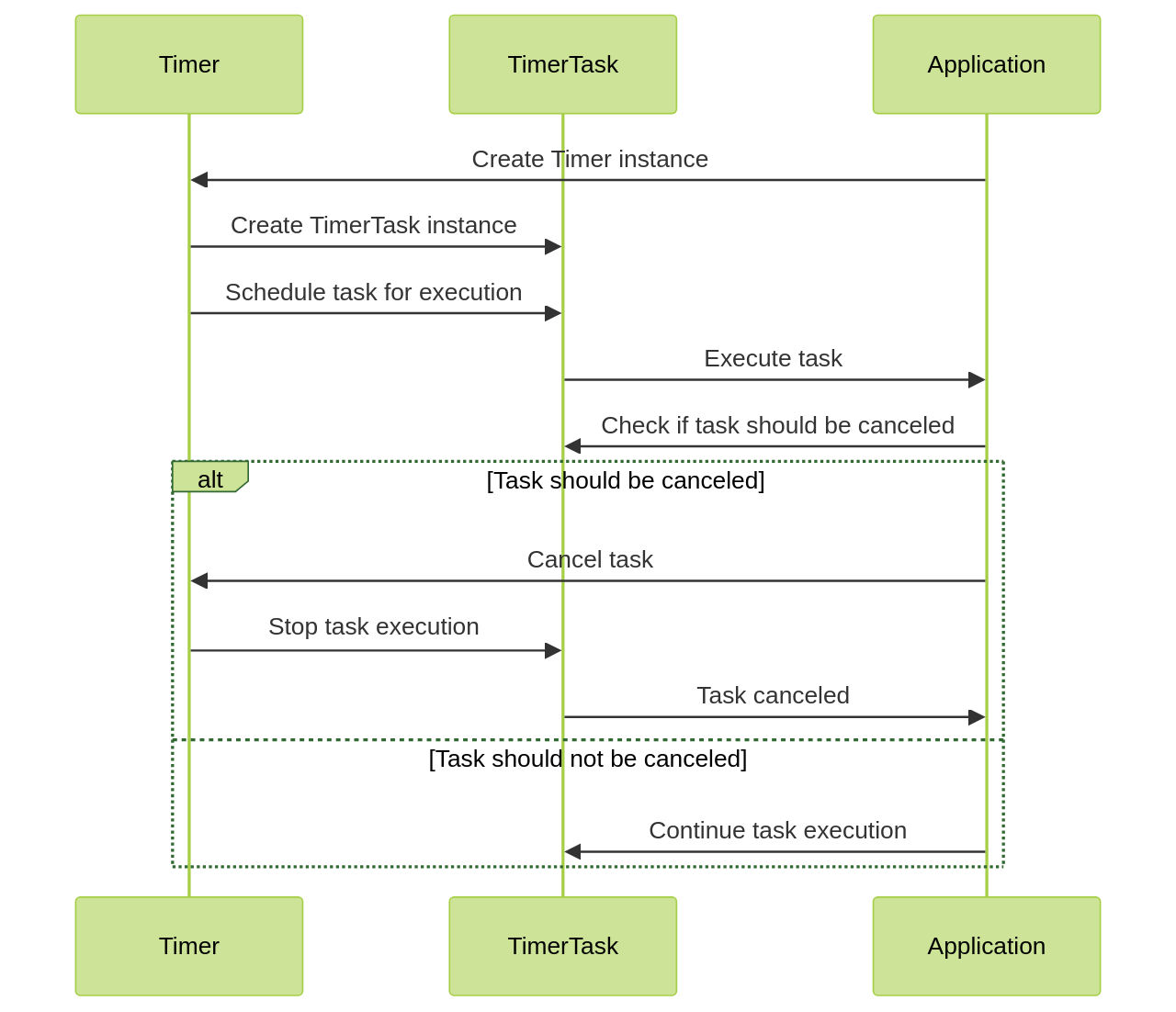
The cancel()
method of the Timer class terminates the Timer and discards any scheduled tasks.
The Timer class provides several schedule methods for scheduling a task to run once at a specific date or after a specified delay. For more robust alternatives to the Timer class, the java.util.concurrent package provides the ScheduledThreadPoolExecutor, which allows for more flexible scheduling of tasks.
Remember, once a TimerTask is cancelled, it cannot be scheduled for execution again. If you need to run the task again, a new instance of the TimerTask will need to be created and scheduled separately
6. Automating Timer and Scheduler Testing: Tools and Frameworks
Java unit testing, specifically for time-dependent code, can be quite challenging. The utilization of certain testing frameworks and libraries, such as JUnit and Mockito, significantly enhances the automation of timer and scheduler testing. JUnit offers developers annotations and assertions, which facilitate the construction of structured and understandable tests. Mockito, on the other hand, simplifies the creation of mock objects and the verification of interactions, a feature that is particularly beneficial when testing Timer and TimerTask classes.
In particular, JUnit annotations serve to indicate the purpose and behavior of the test methods. For instance, the @Test annotation marks a method as a test method, which JUnit executes during the test execution. The @Before and @After annotations are used to denote methods that should be executed before and after each test method, respectively, often used to set up the test environment or initialize test data and to clean up resources or perform any necessary cleanup operations.
JUnit assertions verify the expected behavior of the code being tested. The assertEquals(expected, actual) assertion checks if the expected value is equal to the actual value, failing the test if the values are unequal. The assertTrue(condition) and assertFalse(condition) assertions check if a given condition is true or false, respectively, failing the test if the condition is not met.
For mocking objects in Java unit testing, Mockito is a highly recommended framework. It provides a way to create mock objects that mimic the behavior of real objects, allowing isolation of the code under test and control over the behavior of its dependencies. Mockito provides various methods to create mock objects, define their behavior, specify what methods should return, throw exceptions, or verify that certain methods are called.
To automate timer testing in Java, frameworks like Mockito or libraries like PowerMock or EasyMock can be used, which offer capabilities for simulating and testing time-based events. Mockito, in particular, allows the creation of a mock timer object and definition of its behavior, such as scheduling tasks and verifying if the tasks were executed correctly. JUnit, on the other hand, provides the Timeout
annotation, which allows specification of a maximum time limit for a test case to complete, failing the test case if it does not complete within the specified time limit.
The use of tools like Machinet can further streamline the testing process by generating unit tests automatically, thereby conserving both time and effort. Machinet analyzes the codebase and creates test cases automatically, examining the structure of the code and generating test inputs and assertions accordingly. This automation of the process of writing unit tests ensures better code coverage and improved software quality, proving especially advantageous when working with time-dependent code, where precision and accuracy are paramount.
Moreover, JUnit 5, a popular unit testing framework in the Java ecosystem, added many new features based on the Java 8 version of the language. It provides configuration options for using JUnit 5 in Maven and Gradle projects. JUnit 5 allows the definition of tests using the @Test annotation and supports assertions and assumptions for testing expected results and conditions. It also includes features for creating dynamic and parameterized tests, nested tests, test execution order, and test suites.
JUnit 5 also supports the creation of test reports for Maven and Gradle projects and provides annotations for testing exceptions and conditional enablement. It allows the grouping of tests using nested tests and supports testing with multiple input parameters. JUnit 5 provides the @BeforeEach and @AfterEach annotations for setup and cleanup tasks, and allows the use of static imports for assert statements to make test code more readable. The @DisplayName annotation can be used for custom test names, and tags can be used to filter tests. Extensions can also be used to add additional functionality to tests.
By utilizing these tools and frameworks, developers can write more effective tests, identify bugs early in the development process, and ensure that software works as expected and meets quality standards
7. Handling Changing Requirements in Timer and Scheduler Testing
In the realm of timer and scheduler testing, the ability to effectively manage and adapt to evolving requirements is crucial. This can be achieved by devising tests that are flexible, maintainable, independent, repeatable, and self-validating. A key tool that aids in this process is JUnit, a testing framework that provides the capability to organize tests into logical groups, or suites.
This feature, known as Test Suites, allows multiple test classes to be run together as a single unit. This is especially beneficial when dealing with a large number of test classes that need to be executed simultaneously. To create a test suite in JUnit, the @RunWith
and @Suite
annotations are used. This enables the execution of a specific subset of tests when requirements change, enhancing the adaptability of the testing process.
A fundamental strategy in managing changing requirements is the creation and maintenance of a repository of reusable code and assets. This approach can drastically minimize the need to write new test code from scratch, thus saving time and boosting confidence in existing resources. For instance, the team at Philips successfully managed to reduce test development effort and schedule by 80% through the standardization and reuse of software modules.
Adopting an agile test approach is another critical aspect. This involves the use of modular instrumentation and software to remotely update and manage test stations, reducing deployment time and improving operational metrics. A case in point is a leading appliance manufacturer that managed to cut down deployment time from 30 minutes per system to just three minutes by adopting this approach.
The impact of machine learning and data analytics in test engineering cannot be overlooked. These technologies can be harnessed to automate test program generation and optimize designs, thereby making the testing process more efficient and adaptable to changing requirements. The future of test programs could potentially involve automatically generated tests through intelligent, data-driven systems.
Moreover, utilizing context-aware AI tools, such as Machinet's chat, can be instrumental in generating code based on the updated project description, further aiding in the adaptation of tests to new requirements efficiently and effectively
8. Best Practices for Managing Workload and Balancing Deadlines in Test Automation
In the realm of software development, the challenge of maintaining a manageable workload and meeting deadlines is often met head-on through the implementation of test automation. A key strategy to navigate these challenges is by establishing a sustainable pace for the team. Agile software development practices, such as test-driven development and continuous integration, are significant contributors to maintaining this pace.
However, it's not uncommon for teams to face obstacles in preserving a sustainable pace, such as unrealistic deadlines and pressure from stakeholders. These challenges can lead to negative outcomes, such as technical debt and burnout, if teams are forced to work long hours and sacrifice good practices.
To mitigate these challenges, a potential solution is to prioritize tests. Prioritization allows the team to focus on critical tests first, thereby ensuring that the most important functionalities are verified. Techniques like risk-based testing, exploratory testing, smoke testing, and checklists can aid in prioritizing and covering the most important test cases.
Another strategy to optimize the testing process is to parallelize test execution. This approach reduces the total test execution time by running multiple tests simultaneously, thereby making the process more efficient. Tools like Machinet can be instrumental in this regard, as they provide capabilities to parallelize your test execution, significantly reducing overall execution time[^1^].
Moreover, the time-consuming aspect of testing, test data creation, can also be automated to ensure that tests have the necessary data to run. Automating this process eliminates the need for manual data entry, thus freeing up more time for other important tasks.
It's important to remember that not all test cases need to be run for every software release. Test teams should not hesitate to inform management if they are unable to complete a full regression for a software release, especially if time constraints are limiting.
While the initial investment of time may seem daunting, automating regression tests can offer significant time savings in the long run. This approach allows for the repetitive execution of test cases, further reducing the testing time and helping to meet deadlines without compromising on quality.
Tools like Machinet not only expedite the test creation process but also offer guidance, tutorials, articles, or blog posts on topics such as unit testing basics and benefits, understanding annotations and assertions for Java unit testing, and demystifying the unit testing process in general[^1^]. While hiring more people might seem like a plausible solution to address time constraints, it's often considered as a last resort due to the expense involved.
To integrate Machinet with test automation tools, you can follow a series of steps, such as identifying the test automation tool you want to integrate with Machinet, leveraging Machinet's REST API, and validating the integration by running your test automation scripts[^4^].
Maintaining a sustainable pace requires a proactive approach from management. They play a crucial role in advocating for a sustainable pace and educating stakeholders about the impact of technical debt. Fostering a learning culture and investing time in continuous learning and improvement can also help the team adapt to changing requirements and deliver high-quality software products.
Open communication and experimentation are key in finding a more sustainable approach. Each organization may face different challenges, but staying positive and proactive in finding ways to improve can make a significant difference. Failure to maintain a sustainable pace can lead to burnout and high turnover rates in organizations, further emphasizing the importance of these strategies.
Therefore, managing workload and balancing deadlines in test automation is not just about finding faster ways to complete tasks. It's about creating a sustainable working environment where good practices are maintained, important tasks are prioritized, and the team's well-being is considered.[^1^]: "To improve efficiency in test automation with Machinet, you can follow some best practices. First, make sure to write clear and concise test cases that cover all the necessary scenarios. This will help in reducing the time spent on debugging and re-running failed tests. Additionally, you can leverage the capabilities of Machinet to parallelize your test execution, which can significantly reduce the overall execution time. It is also important to regularly review and update your test suite, removing any redundant or obsolete test cases. Finally, consider implementing continuous integration and continuous delivery practices, which can automate the test execution process and provide faster feedback on the quality of your software."[^4^]: "To integrate Machinet with test automation tools, you can follow the steps below:nn1. Identify the test automation tool you want to integrate with Machinet. This could be a popular tool such as Selenium, Appium, or JUnit.nn2. Check if Machinet provides any specific plugins or libraries for the test automation tool you are using. These plugins or libraries can simplify the integration process and provide additional functionality.nn3. If there are no specific plugins or libraries available, you can still integrate Machinet by leveraging its REST API. Machinet likely provides APIs to interact with its features and functionalities programmatically. You can use these APIs to create, manage, and execute test cases from your test automation tool.nn4. Ensure that you have the necessary credentials (API keys, access tokens, etc.) to authenticate and authorize the integration between your test automation tool and Machinet.nn5. Write code or scripts in your test automation tool to interact with Machinet's API endpoints. This could involve creating test cases, executing them, retrieving test results, and analyzing test data.nn6. Test and validate the integration by running your test automation scripts and verifying that they successfully interact with Machinet and perform the desired actions.nnRemember, the specific steps and details of integrating Machinet with test automation tools may vary based on the test automation tool you are using and the specific features provided by Machinet. It is recommended to refer to the documentation or support resources provided by both Machinet and your chosen test automation tool for more detailed instructions
Conclusion
In conclusion, understanding the basics of Timer and Scheduler in Java is essential for developers working with time-dependent code. The Timer and TimerTask classes provide a built-in mechanism for scheduling tasks to be executed in the future, either once or repeatedly at regular intervals. However, the more modern alternative, ScheduledExecutorService and ScheduledFuture, offers additional features and flexibility for scheduling tasks. Unit testing plays a crucial role in validating the functionality of Timer and Scheduler components, ensuring that they perform as expected. Techniques such as marble testing and using tools like FakeAsync or TestScheduler can help effectively test time-dependent code. By following best practices and utilizing the right tools, developers can optimize their timer and scheduler implementations.
The broader significance of these ideas lies in the ability to effectively manage time-dependent code and optimize software applications. By mastering Timer and Scheduler concepts, developers can ensure reliable execution of tasks at specific times or after specified delays. This is particularly important when dealing with recurring tasks or managing delays and intervals correctly. Additionally, unit testing these components is essential for identifying potential issues early on in the development cycle, saving time, effort, and resources. Incorporating automation tools like Machinet can further enhance productivity by generating unit tests automatically and improving code coverage. Boost your productivity with Machinet. Experience the power of AI-assisted coding and automated unit test generation.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.