
Table of Contents
- Understanding the Basics of Selenium
- Setting up Your Environment for Selenium Automation Testing
- First Steps with Selenium: Creating a Basic Test
- Advanced Techniques in Selenium Automation Testing
- Dealing with Challenges in Selenium Automation Testing
- Best Practices for Efficient and Effective Selenium Automation Testing
- Case Study: Implementing Selenium in a Real-World Project
Introduction
Selenium, a powerful open-source framework, has become a go-to choice for web browser automation in the world of software engineering. With its comprehensive suite of software components and support for multiple programming languages, Selenium offers a flexible and robust solution for web application testing. From controlling browsers to interacting with web pages, Selenium's capabilities empower developers to automate tasks such as web scraping, testing, and web application development.
In this article, we will delve into the basics of Selenium, exploring its various components such as Selenium IDE, Selenium RC, WebDriver, and Selenium Grid. We will also discuss the importance of using test frameworks in conjunction with WebDriver to enhance testing capabilities. Furthermore, we will highlight the challenges faced in Selenium automation testing and explore advanced techniques and best practices to overcome them. By leveraging Selenium's potential and implementing efficient strategies, software engineers can construct reliable and effective test suites that optimize their testing efforts
1. Understanding the Basics of Selenium
Selenium, a potent open-source framework, has earned its reputation in the world of web browser automation. It isn't just a single tool, but a comprehensive suite of software, each component catering to diverse testing needs. This suite is composed of the Selenium Integrated Development Environment (IDE), Selenium Remote Control (RC), WebDriver, and Selenium Grid.
One of Selenium's many strengths is its support for a variety of programming languages, including Java, C#, Python, Ruby, and others. This versatility, along with its compatibility with various operating systems and browsers, positions it as a favored choice for web application testing.
The WebDriver component is at the heart of Selenium's power, playing a crucial role in controlling the browser. Just like a human user, WebDriver interacts with the browser, performing actions like clicking, typing, and selecting from drop-down menus, effectively replicating user behavior. Communication with the browser happens via a driver, which could be local or remote, as per the setup. Selenium Server or Selenium Grid can facilitate this communication for remote scenarios.
However, WebDriver's primary focus is on browser interaction, and it does not inherently come with testing, assertion, or reporting capabilities. To address this, WebDriver can be used together with test frameworks such as JUnit, NUnit, Cucumber, and Robotium. These frameworks not only run WebDriver tests but also offer additional testing features. For instance, Cucumber, a natural language framework, can be integrated to create test cases that are more descriptive.
It's worth noting that Selenium is a project managed by the Software Freedom Conservancy and has the support of various sponsors. It provides detailed documentation covering various facets of the framework, including terminologies like API, library, driver, and framework. A deep comprehension of these elements and terminologies is vital for constructing effective test suites.
Additionally, Selenium WebDriver offers a wide range of methods and functions to simulate user actions like clicking buttons, filling out forms, and navigating through web pages. This allows developers to write scripts in popular programming languages to automate web browsers and perform tasks such as web scraping, testing, and web application development.
While Selenium remains a powerful tool for web application testing, alternatives like Cypress, Puppeteer, Playwright, and TestCafe are also worth considering. These tools provide similar functionality to Selenium and are often chosen for their user-friendly nature and robustness. Each tool comes with its unique features and advantages, making it essential to choose based on specific testing needs.
To wrap up, Selenium, with its suite of tools and comprehensive documentation, offers a robust and flexible framework for web application testing. By harnessing its capabilities, software engineers can construct effective and resilient test suites
2. Setting up Your Environment for Selenium Automation Testing
Establishing the right environment for Selenium automation testing is crucial for effective testing.
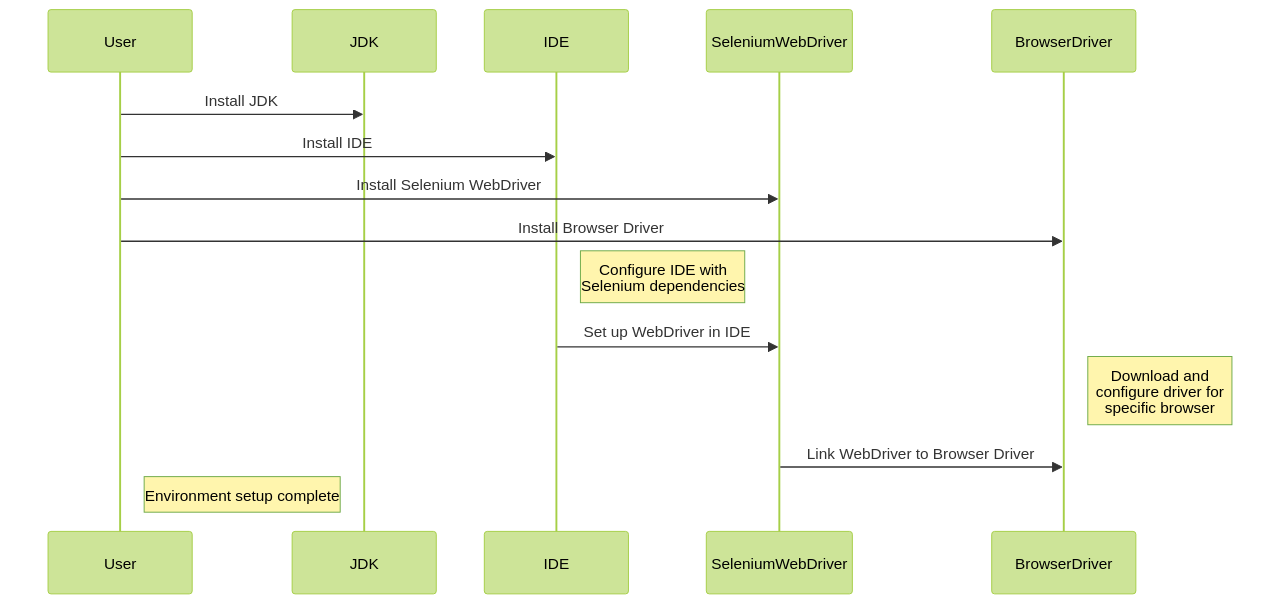
This process involves several steps, among which is the installation of the Java Development Kit (JDK). JDK is often the language of choice for writing Selenium tests. To install JDK, you'll need to download the JDK installation package from the official Oracle website. Follow the on-screen instructions to complete the installation. After the installation, you may need to set up the JAVA_HOME environment variable to point to the JDK installation directory. This will ensure that your Selenium tests can access the necessary Java libraries.
Next, you will need an Integrated Development Environment (IDE). Eclipse and IntelliJ IDEA are popular choices due to their extensive features and support for Java. To install Eclipse, download the installer from the official Eclipse website. After the installation, launch Eclipse and create a new workspace. Install the Selenium WebDriver plugin for Eclipse and configure it by specifying the location of the WebDriver executable for the browser you want to automate. You can then create a new Java project in Eclipse for your Selenium test automation and add the Selenium WebDriver library to your project's build path.
For IntelliJ IDEA, follow similar steps. Download the latest version of IntelliJ IDEA Community Edition from the official website and install it. After installation, open your project in IntelliJ IDEA. Configure your project to use Selenium by adding the necessary dependencies to your project's build file (like pom.xml for Maven or build.gradle for Gradle). You can then write your Selenium tests using IntelliJ IDEA's powerful code editing features.
The following step involves the Selenium WebDriver. To install it, navigate to the "Downloads" section on the official Selenium website and download the latest version. Once downloaded, extract its contents to a preferred location on your computer. Depending on your programming language, you may need to set up additional configurations or dependencies.
Lastly, you will need to install the browser driver specific to the browser you intend to test. For example, to install ChromeDriver for testing on Google Chrome, download the ChromeDriver executable file from the official Selenium website. Extract the downloaded file to a preferred directory and set the path to the ChromeDriver executable in your test script or configure it in your test framework. Ensure that the version of ChromeDriver you downloaded is compatible with the version of Chrome installed on your machine.
For Firefox, install the Firefox browser and download the geckodriver executable for your operating system. Add the location of the geckodriver executable to your system's PATH environment variable. In your Selenium test code, initialize the FirefoxDriver by creating an instance of it.
For Safari, ensure that you have the latest version of Safari installed on your machine. Open Safari and go to the Safari menu, click on "Preferences" and then go to the "Advanced" tab. Check the box that says "Show Develop menu in menu bar". Close the Preferences window and go to the Develop menu and click on "Allow Remote Automation". Now you can use safaridriver for Selenium test automation on Safari browser.
Once these components are successfully installed and configured, you can start writing Selenium tests. This setup is designed with a Windows PC in mind, but the process is similar across different operating systems. The goal is to create an environment that supports your Selenium automation testing efforts effectively, allowing you to write and execute tests with ease
3. First Steps with Selenium: Creating a Basic Test
As you venture into Selenium automation testing, the first critical step is creating your initial Selenium test.

This basic test involves a series of actions such as initiating a browser, navigating it to a specific web page, executing specific actions, and subsequently verifying the results. Here's a detailed guide to creating a basic Selenium test using Java.
To start, it's essential to import the necessary Selenium libraries into your Java project. This can be done by following these steps:
- Open your Java project in your preferred IDE (Integrated Development Environment).
- Right-click on your project in the IDE's project explorer and select "Properties" or "Build Path."
- Navigate to the "Libraries" or "Dependencies" tab in the properties or build path settings.
- Click on the "Add External JARs" or "Add JARs" button.
- Locate the Selenium libraries on your local machine. These are typically in JAR (Java Archive) format.
- Select the Selenium JAR files you want to import and click "Open" or "OK" to add them to your project's build path.
After importing the Selenium libraries, you need to generate a new instance of the WebDriver interface. This can be done by creating an instance of the WebDriver interface, which represents the browser you want to automate. For instance, you can use the ChromeDriver class to automate Google Chrome.
The next step is to direct the browser to the desired web page. This can be achieved by using the WebDriver's get() method. This method takes a URL as a parameter and directs the browser to that URL.
After navigating to the web page, you will need to perform actions on the page. WebDriver provides methods like click()
, sendKeys()
, and navigate().to()
that allow you to interact with elements on the web page. The click()
method can be used to click on buttons or links, the sendKeys()
method can be used to enter text into input fields or forms, and the navigate().to()
method can be used to navigate to a specific URL.
Once you have performed the necessary actions, you will need to verify the outcomes. This can be done using assertions provided by testing frameworks like JUnit or TestNG. Assertions are used to check whether the expected results match the actual results of the test.
Finally, it's important to close the browser once the test is complete. This can be done by calling the quit() function on the WebDriver object. This ensures that the browser sessions are properly cleaned up even if the tests fail.
This Selenium WebDriver course covers various topics including installing Selenium WebDriver and its dependencies, launching and interacting with websites, finding elements within a web application, interacting with elements, handling popups and alerts, uploading files, working with iframes, advanced interactions, integrating with a test assertion library, organizing test code using the page object model, using wait strategies to control timing, taking screenshots, listening for WebDriver events, customizing the browser used in test execution, running headless tests, navigating multiple open tabs, cookie management, and writing custom actions using JavaScript.
In addition, there are optional independent exercises included in the course for those who wish to practice and apply what they have learned. These exercises help to reinforce the concepts and techniques taught in the course, and provide an opportunity for learners to gain hands-on experience with Selenium WebDriver.
This course is an excellent resource for those who are interested in learning Selenium WebDriver with Java. It provides a solid foundation in Selenium automation testing and equips learners with the skills and knowledge needed to write effective and robust Selenium tests
4. Advanced Techniques in Selenium Automation Testing
The use of advanced methodologies in Selenium can significantly boost the robustness and efficiency of your tests.

One such effective technique is the use of explicit waits. By incorporating explicit waits, tests are made to pause until certain preconditions are met, mitigating the occurrence of flaky tests that might fail sporadically due to timing discrepancies.
To implement explicit waits in Selenium for robust tests, the WebDriverWait class provided by the Selenium WebDriver can be utilized. This class allows you to wait for a certain condition to be met before proceeding with the test execution. One common use case for explicit waits is waiting for an element to be visible, clickable, or have a specific value.
Another advanced methodology is the implementation of the Page Object Model (POM), a design pattern that promotes code reusability and enhances readability. POM organizes web pages into separate classes, where each class represents a specific page or a component on the page. These classes contain the locators and methods to interact with the elements on the page. By implementing POM, you can achieve code reusability by creating a separate class for each page or component.
To implement the Page Object Model (POM) in Selenium, a separate class for each web page or component in your application is created. The web elements and actions on each page are defined as instance variables and methods in the respective class. The PageFactory pattern or the @FindBy annotation is used to initialize the web elements. The actions and interactions with the web elements are encapsulated within methods, providing descriptive names for each method.
In addition to these, handling of pop-ups and alerts, management of dropdowns, and the capturing of screenshots for debugging purposes are included in the sophisticated methodologies. To handle pop-ups and alerts in Selenium, the Alert class provided by Selenium WebDriver can be used. This class allows you to interact with pop-up windows, JavaScript alerts, and confirmation dialogs.
Taking screenshots in Selenium for debugging purposes can be done using the built-in screenshot functionality provided by Selenium WebDriver. This allows you to capture the current state of the webpage during test execution. The WebDriver's getScreenshotAs method can be used to capture the screenshot and save it to a desired location on your machine. This can be done at any point in your test script where you want to capture the webpage state.
In essence, the implementation of advanced techniques in Selenium automation testing, such as explicit waits and the use of the Page Object Model, can greatly enhance the robustness and efficiency of your tests. By gaining a deeper understanding of these techniques, you can confidently tackle more complex testing scenarios and ensure the reliability of your software applications
5. Dealing with Challenges in Selenium Automation Testing
When it comes to automation testing, tools like Selenium are often the go-to choice for software engineers due to their robust capabilities.
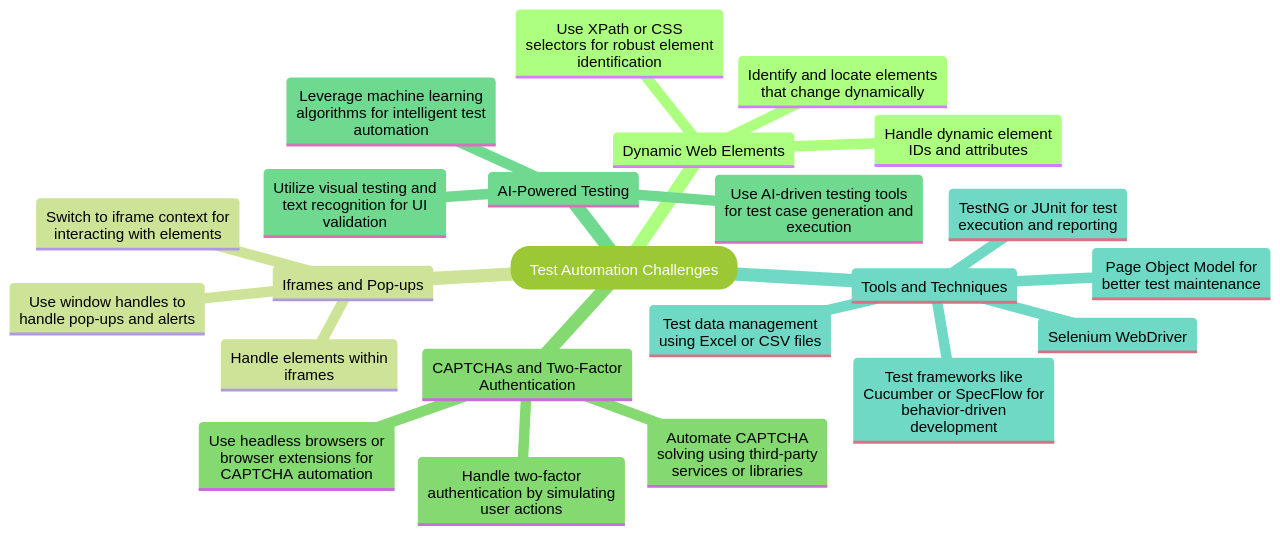
Yet, the process isn't without its challenges. Dynamic web elements, for instance, can cause headaches due to their propensity to alter attributes with each page load. To navigate this, developers often utilize dynamic XPath or CSS selectors, which can identify elements based on their varying attributes.
One approach to handle these dynamic web elements in Selenium involves using unique identifiers, such as IDs or CSS selectors, to locate and interact with these elements. It's also advisable to implement explicit waits using the WebDriverWait class in Selenium to ensure the dynamic element is present before interacting with it. In instances where an element's attributes are changing dynamically, XPath with dynamic values can be a useful tool. Itβs worth noting, however, that XPath may become unstable if the page structure changes, and therefore itβs important to handle potential stale element references which can occur when a dynamic element is updated or removed from the DOM.
Another hurdle to tackle during Selenium automation testing is the handling of iframes and pop-ups. As these elements require a shift in the WebDriver's context, developers can use the switchTo method to transition between contexts smoothly. This includes switching to an iframe or handling pop-ups, which involves using the switchTo().alert() method to accept or dismiss the pop-up. It's crucial to remember to switch back to the default content using the switchTo().defaultContent() method once you are finished working with iframes or pop-ups.
Moreover, some aspects of automation testing, such as CAPTCHAs and two-factor authentication, often necessitate manual intervention or the utilization of a third-party service. To circumvent CAPTCHAs, third-party services that provide captcha-solving capabilities can be used, while the Selenium WebDriver's capabilities can be leveraged to interact with the authentication page for handling two-factor authentication.
Despite these challenges, Selenium's potential in automating web application testing should not be underplayed. With the right strategies, these obstacles can be managed effectively. Moreover, advancements in AI-powered testing are paving the way for more efficient and effective testing processes. Companies like Qualitest are leveraging AI-powered quality engineering services to enhance their software testing processes, while Functionize, an autonomous testing platform, uses AI and machine learning to bolster test creation and maintenance. Functionize's integration with platforms like Salesforce, Workday, SAP, Oracle, and others provides a comprehensive solution for avoiding common pitfalls in Selenium tests.
In essence, while Selenium presents certain challenges in automation testing, the right strategies and tools, coupled with advancements in AI-powered testing, enable developers to effectively navigate these challenges and maximize their software testing efforts
6. Best Practices for Efficient and Effective Selenium Automation Testing
To fully capitalize on the capabilities of Selenium, it's vital to adhere to several key principles. One central tenet is to keep your tests succinct and focused, with each test validating a singular functionality or behavior. This approach eases the maintenance and debugging of tests. It's equally important to assign descriptive names to your tests and test data, enhancing the understandability of each test's function and expectations.
Assertions are crucial as they verify the outcomes of your actions, serving as a bug detector and enhancing the reliability of your tests. One common method of assertion is the assertEquals method, which compares two values and throws an assertion error if they are unequal. This can be used to verify that a certain web page element has a specific value or text. Another useful assertion method is the assertTrue method, which throws an assertion error if the given condition is not true, verifying that certain elements or conditions exist on a web page.
Lastly, post-test cleanups are essential, which includes closing the browser and eradicating any test data. This involves removing files, databases, or any other resources that were used for testing purposes. Implementing these strategies can augment the efficiency and effectiveness of your Selenium tests.
To optimize your tests further, consider implementing additional techniques. Blocking unnecessary requests can expedite tests, while reusing test code with functions can create cleaner, more organized tests. APIs can be leveraged to shortcut lengthy workflows, thereby improving efficiency. Employing a headless mode can lead to faster test execution, and writing tests as though they will be running in parallel can help avoid flaky tests.
When creating resources in tests, use unique identifiers. Be sure to handle network throttling and rate limiting for tests involving third-party APIs. Prioritize user-facing attributes and use locators with auto waiting, chain and filter locators to narrow down search, and avoid using DOM-dependent locators that may change.
Playwright's test generator can be a valuable tool for generating tests and picking locators. Use web-first assertions for more reliable tests, and keep Playwright up to date for testing on the latest browser versions. Integrate Playwright tests with CI/CD for frequent testing and utilize Playwright's tooling, such as the VS Code extension and trace viewer. TypeScript support can improve IDE integrations.
Several techniques can be used to expedite UI automated tests. These include using the API and database layers of the application, visual regression, parallel execution, distributed execution, headless browsers, efficient test setup and teardown, using cookies, navigation shortcuts, and creating atomic non-repeating tests. The API layer of an application executes commands faster than the UI layer and can be used for test data creation, cleanup, and verification. Visual regression ensures the GUI of an application maintains the defined look.
Parallel execution allows running multiple automated tests at the same time, reducing the overall test suite's runtime. Distributed execution involves executing tests across multiple virtual machines or computers in the cloud, improving test speed and eliminating the need for server and browser version management. Headless browsers operate without a graphical user interface, making tests faster and reducing memory usage compared to GUI browsers.
Efficient test setup and teardown using APIs, databases, and other techniques can speed up UI tests. Cookies can be used to store information and preload test scenarios, improving test speed. Navigation shortcuts can be used to directly navigate to specific pages, reducing unnecessary steps. Creating atomic non-repeating tests helps keep tests focused and reduces runtime.
In conclusion, testing is a critical part of software development. Using the right tools and techniques, you can streamline your testing workflow and ensure reliable and bug-free web applications
7. Case Study: Implementing Selenium in a Real-World Project
The Georgia Lottery Corporation (GLC), a state-run entity, utilized SmartBear's QACcomplete and TestComplete Web solutions to automate application requirements, tests, and defects in their lottery system. These tools proved instrumental in enabling GLC to transition from a waterfall to an agile development environment, thereby increasing the speed and frequency of new features and applications rollouts. The result? An impressive increase in software releases per quarter.
In addition to SmartBear solutions, Katalon, a comprehensive quality management platform, has also made significant strides in the field of software testing. The platform is versatile, offering solutions for test authoring, execution, management, reporting analytics, and AI-powered testing. Its scalability allows teams of varying programming skills to adopt the tool, streamlining their testing processes and enhancing their products and services.
However, when it comes to web application testing, Selenium WebDriver is a standout tool. A powerful solution for automating interactions with web browsers, Selenium WebDriver supports various programming languages including Java, Python, C#, and JavaScript. This makes it an excellent choice for automating testing for complex web applications, allowing you to write test scripts that simulate user interactions and verify your web application's behavior.
While setting up a Selenium environment for web application testing, best practices include installing the required software, configuring the browser, managing dependencies, preparing test data, implementing test automation frameworks, maintaining a stable test environment, version control, and continuous integration. These steps can help ensure a reliable and effective testing environment.
To handle application complexity and improve test automation with Selenium, it's recommended to use explicit waits and the Page Object Model (POM) design pattern. Explicit waits ensure that certain conditions are met before proceeding with the test execution. This is particularly useful when dealing with dynamic elements or slow-loading components. Meanwhile, the POM design pattern aids in creating a maintainable test automation framework by creating separate classes for each page of the application. This results in better code reusability, readability, and maintainability.
Selenium can also effectively handle dynamic elements and iframes. This is achieved by using dynamic locators, such as XPath or CSS selectors, and leveraging Selenium's iframe handling capabilities. Consequently, you can effectively test dynamic elements and iframes in your automation scripts.
In the realm of e-commerce, Selenium has proven to be a successful tool for ensuring the functionality and reliability of websites. By automating the testing process, it allows companies to test their websites across different browsers and platforms, saving time and effort. Selenium's ability to perform end-to-end testing, run tests in parallel, and provide robust reporting and logging capabilities contribute to its success in the e-commerce sector.
In conclusion, automation tools like QACcomplete, TestComplete Web, Katalon, and especially Selenium WebDriver, have revolutionized the field of software testing.
They offer solutions that streamline the testing process, enhance product quality, and improve the efficiency of software releases
Conclusion
In conclusion, Selenium is a powerful open-source framework that offers a comprehensive suite of software components for web browser automation and testing. With support for multiple programming languages and compatibility with various operating systems and browsers, Selenium provides a flexible and robust solution for automating tasks such as web scraping, testing, and web application development. The WebDriver component, in particular, plays a crucial role in controlling browsers and interacting with web pages. By using test frameworks in conjunction with WebDriver, developers can enhance their testing capabilities and create reliable test suites. Despite the challenges faced in Selenium automation testing, such as handling dynamic elements and iframes, implementing advanced techniques like explicit waits and the Page Object Model can help overcome these obstacles. Overall, by leveraging Selenium's potential and implementing efficient strategies, software engineers can optimize their testing efforts and achieve reliable and effective results.
To boost your productivity with Machinet, experience the power of AI-assisted coding and automated unit test generation. Boost your productivity with Machinet. Experience the power of AI-assisted coding and automated unit test generation.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.