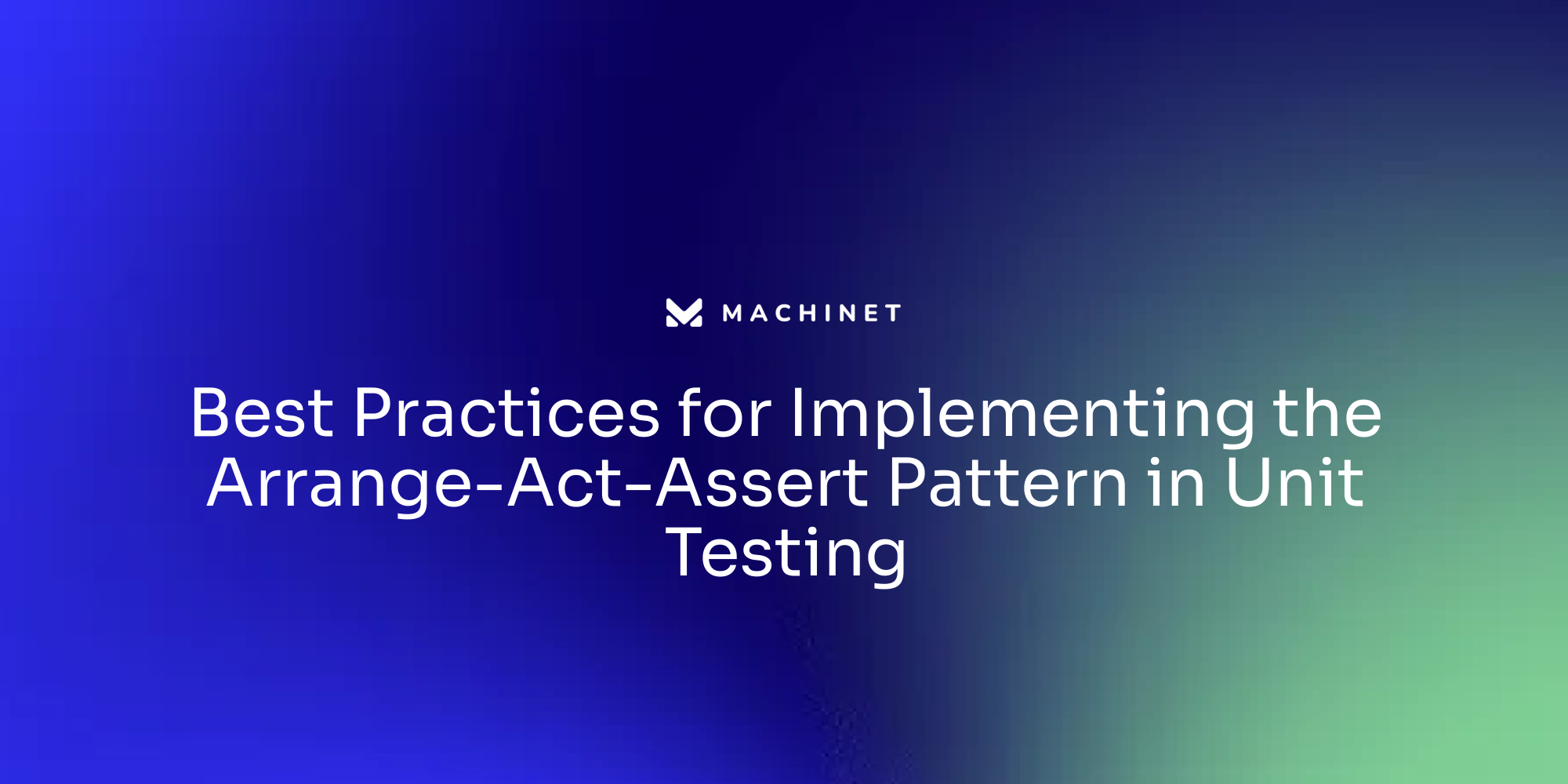
Table of Contents
- Understanding the Basics of Arrange-Act-Assert Pattern
- The Role of AAA Pattern in Unit Testing
- Implementation Steps for the AAA Pattern in Unit Testing
- Best Practices for Using Arrange-Act-Assert in Unit Testing
- Benefits and Challenges of Applying the AAA Pattern to Unit Testing
- Case Study: Successful Application of the AAA Pattern in a Real-world Scenario
- Adapting the Arrange-Act-Assert Pattern to Evolving Project Needs
Introduction
The Arrange-Act-Assert (AAA) pattern is a structured approach to unit testing that provides numerous benefits in terms of clarity, maintainability, and reliability. This pattern divides unit tests into three distinct phases: Arrange, Act, and Assert. In the Arrange phase, the necessary preconditions and inputs for the test are set up. The Act phase involves executing the specific functionality or method being tested. Finally, the Assert phase verifies the expected outcome of the test by comparing it with the actual result.
By adhering to the AAA pattern, developers can enhance the comprehensibility of their tests, focus on testing a single behavior per test, and ensure that tests are independent and repeatable. This approach also facilitates easier debugging and problem-solving by isolating potential issues to specific areas of the code. Additionally, the AAA pattern can be adapted to evolving project needs, such as when dealing with complex systems, implementing advanced architectures, or refactoring legacy code. Overall, the AAA pattern is a valuable tool for developers to create efficient and reliable unit tests
1. Understanding the Basics of Arrange-Act-Assert Pattern
The Arrange-Act-Assert (AAA) methodology, a recognized strategy in unit testing, presents a systematic and logical approach to organizing test cases. Each segment of this approach plays a unique role in crafting a comprehensive test scenario.
The 'Arrange' phase configures the system under test, setting up an appropriate environment for the test case. This critical stage involves initializing variables, configuring dependencies, and employing test object builders for frequently used objects, ensuring the accurate setup of the objects involved in the test.
The 'Act' stage activates the system under test. This phase invokes the specific behavior or action under scrutiny. Each test ideally targets a single concept or behavior, ensuring the tests remain fast, independent, repeatable, and self-validating.
The 'Assert' phase validates the system's behavior under test. This stage compares the actual output from the 'Act' phase with the expected results, ensuring the code functions as expected. Tests should fail when they are supposed to, indicating an accurate functioning of the code.
Emphasizing testing edge cases and boundaries, not just the so-called 'happy path,' ensures that potential issues or errors are identified and handled effectively. Strive for good test coverage, prioritizing critical parts of the application before less crucial paths. Avoid dogmatism about achieving 100% coverage.
Tests should be developed concurrently with the production code and should be environment-independent and repeatable. Tests should validate themselves, not requiring human interpretation. Refrain from using snapshot tests, as they don't provide clear validation of correct output.
Consideration of failure conditions, often referred to as 'sad paths,' is as important as the expected working conditions, known as the 'happy path.' This consideration is especially crucial when a method may throw custom domain exceptions or when using guard clauses.
In essence, the AAA pattern offers a clear and concise manner to write tests, enhancing the understanding of each test's role and purpose. Adhering to these guidelines ensures well-written and maintained tests that bolster code reliability and confidence
2. The Role of AAA Pattern in Unit Testing
The Arrange-Act-Assert (AAA) pattern is an indispensable tool in the arsenal of unit testing, providing a clear and consistent schema for designing tests. This pattern enhances the comprehensibility of tests for developers by outlining the specific conditions and functions each test is designed to assess. The AAA pattern is fundamentally aligned with the principle of focusing on a single behavior in effective unit testing.
The AAA pattern encourages the development of efficient and maintainable tests, which are essential for building robust software.
Try Machinet to streamline your unit testing process and enhance test efficiency!
It provides a systematic methodology for unit testing, empowering developers to guarantee the functionality and maintainability of their code.
The Arrange phase of the AAA pattern involves setting up the necessary preconditions and inputs for the unit under test. This includes creating requisite objects, establishing the initial state, and configuring dependencies. The Act phase encompasses the invocation of the method or action being tested. This could be a method call or an event trigger. In the Assert phase, the test case checks the expected outcome or behavior of the unit under test. By comparing the actual result with the expected result, and asserting any other expected side effects or state changes, the effectiveness of the unit test can be determined.
In the context of best practices for implementing the AAA pattern, the Arrange phase should be kept minimal and focused on preparing the necessary objects and dependencies. The Act phase should be concise, including only the specific functionality or method being tested. Clear and descriptive assertions should be used in the Assert phase to make the test results more understandable. Avoiding unnecessary duplication of code by using setup and teardown methods or test fixtures is also recommended. By adhering to these best practices, the AAA pattern can be effectively implemented in unit testing, resulting in well-structured, maintainable tests that deliver accurate results.
Techniques such as custom test data builders and asserts can significantly enhance the setup of test data, improving the readability and efficiency of unit tests. The use of object mother and builder patterns can aid in the creation of test objects in the correct state, mitigating code repetition. Custom assert classes can encapsulate complex checks, offering meaningful error messages in unit tests.
The application of principles and best practices, such as Domain-Driven Design (DDD), can result in clean and readable test code that aligns with the project's ubiquitous language. Builders and custom asserts add an additional layer of abstraction, which has advantages in terms of test logic reusability and code clarity but also requires additional code to be written and maintained.
Data security and privacy are significant considerations in software development, and the AAA pattern can help address these concerns. By ensuring that each unit test is focused and effective, developers can catch potential security vulnerabilities early in the development process, making it easier to build secure and reliable software
3. Implementation Steps for the AAA Pattern in Unit Testing
Unit testing, a vital tool in the software development process, can be streamlined and improved by the AAA (Arrange-Act-Assert) pattern. This pattern provides a structured approach to writing tests, ensuring they are organized, easy to understand, and reliable.
The first phase of the AAA pattern is the Arrange phase, where the test environment is prepared. This involves setting up the necessary preconditions for the test, including creating objects, initializing variables, and setting up any required dependencies. By ensuring the system is in a known state before proceeding, the Arrange phase lays a solid foundation for the subsequent phases.
Following the Arrange phase, the Act phase is where the specific functionality or method being tested is executed. This usually involves invoking a method or performing an action. The Act phase allows for the observation of the expected behavior and the validation of the test results, providing crucial insights into how the code behaves when activated.
The final phase is Assert, where the expected outcome of the test is verified by comparing it with the actual result. This phase uses assertions to check if the test passed or failed, confirming whether the code has performed as expected. The Assert phase is of vital importance as it provides the final validation of the code's behavior.
Implementing the AAA pattern in unit testing not only makes the test cases more readable and maintainable but also helps in quickly identifying the cause of failures.
This pattern, when used in conjunction with Test-Driven Development (TDD), can help ensure code quality and reduce the likelihood of bugs.
The AAA pattern is also widely used in practical applications such as XUnit, a popular testing framework for .NET applications. XUnit provides various methods in the Assert class for making assertions in tests, including checking types, null values, and equality. It also allows for testing with multiple different values by passing arguments to test methods and for testing exceptions using the Assert.Throws method.
Organizing test projects to mimic the structure of source code projects is a recommended best practice when using the AAA pattern. This practice facilitates better organization and contributes to the readability, maintainability, and effectiveness of unit tests. Understanding and implementing the AAA pattern can thus lead to the production of higher-quality software products and contribute to the successful delivery of software projects
4. Best Practices for Using Arrange-Act-Assert in Unit Testing
Unit testing, when performed using the Arrange-Act-Assert (AAA) pattern, can be made significantly more effective by adhering to certain best practices. A primary practice is to focus on testing a single behavior per test, which enhances the comprehensibility of what the test is intended to achieve. The AAA pattern plays a crucial role in enforcing this focus. The 'Arrange' phase sets up the necessary preconditions and inputs for the behavior to be tested. This may involve creating objects, initializing variables, or mocking dependencies. The 'Act' phase then invokes the method or behavior that is being tested, triggering the specific behavior. Finally, the 'Assert' phase verifies that the behavior produces the expected outcome by checking the output, state changes, or interactions with dependencies.
Keeping the Arrange and Act sections free from assertions is another recommended practice. The primary role of these sections is to establish the conditions for the test and carry out the actions that lead to the outcome to be verified. Assertions, which are designed to verify the system's behavior under test, should be confined to the Assert section.
Maintaining the independence of the tests is another crucial practice. Tests should not depend on the state of other tests, and each test should be executable in isolation and in any sequence. This is achievable by setting up the test environment properly in the Arrange phase, allowing each test to run independently without being affected by the state of other tests. It's crucial to clean up any resources or state changes made during the test in the Assert phase to leave the environment in a clean and predictable state for subsequent tests.
Lastly, the tests should be written in a manner that prioritizes readability and comprehension. This can be achieved by using descriptive names for tests and variables, and by keeping the tests concise and straightforward. For instance, a test that checks the behavior of a method calculating the average of a list of numbers could be named "CalculateAverage_WhenGivenListOfNumbers_ReturnsCorrectResult". Similarly, variables can be named to clearly describe their purpose, such as "listOfNumbers" for the input list and "expectedResult" for the expected average value. This practice not only simplifies the understanding of tests but also facilitates their maintenance and reduces the likelihood of errors
5. Benefits and Challenges of Applying the AAA Pattern to Unit Testing
The Arrange-Act-Assert (AAA) pattern in unit testing introduces a multitude of advantages. It enhances clarity and consistency in test structures, thereby improving readability and comprehensibility. A distinct advantage is that it focuses each test on a single behavior, heightening the effectiveness of the tests.
The AAA pattern also streamlines the maintenance and updating of tests due to a uniform and predictable structure. However, it's important to understand that the AAA pattern may not be universally applicable. Certain types of tests, especially those requiring complex setup or teardown, may not be amenable to this pattern. Ensuring that each test is wholly independent and does not rely on the state of other tests can also present a challenge.
It's also critical to remember that while the AAA pattern offers a clear structure for tests, this structure should not mirror the production code's structure. This is to avoid coupling and fragility, as highlighted by Robert C. Martin in his blog "The Clean Code Blog". Martin discusses the importance of minimizing the coupling between tests and production code to avoid the fragile test problem.
He rightly emphasizes, "If a small change in one module of a system causes large changes in many other modules of the system, the system has a design problem. The coupling between the tests and the production code must be minimized. The structure of the tests must not reflect the structure of the production code because that much coupling makes the system fragile and obstructs refactoring." He further adds, "The act of generalizing is the act of decoupling. We decouple by generalizing."
In the context of unit testing, the AAA pattern should be designed to evolve towards greater specificity as development progresses, while the production code should move towards greater generalization. This approach reduces coupling and allows the production code to satisfy a broad range of unspecified behaviors.
At times, unit testing may require access to private members to verify a class's functionality. In such instances, implementing the attorney pattern can be beneficial. This pattern creates a limited forwarding proxy around the class instance and selectively exposes some implementation details. This controlled access to private members during unit testing not only enforces explicit access but also mitigates the risk of tests becoming overly dependent on low-level implementation details. It's less error-prone than directly accessing private members and avoids inconsistencies that might arise due to future type or signature changes.
To elaborate, the AAA pattern divides the unit test into three distinct sections. The Arrange phase is where you set up the necessary preconditions for the test, including the creation of objects or initialization of any variables required for the test. The Act phase involves performing the actual action or operation that you want to test. The Assert phase is where you make assertions about the expected outcome of the test, comparing the actual result of the action with the expected result to check if they match.
By adhering to the AAA pattern, unit tests become more structured and easier to understand. It also helps in isolating different parts of the test, making it easier to identify and fix issues when they arise.
Boost your unit testing with Machinet's powerful AI plugin and streamline your testing process.
The AAA pattern promotes good testing practices and helps ensure the reliability and correctness of your unit tests. Each test is self-contained and does not depend on the state or results of any other test, helping to prevent interference between tests and ensuring that they can be run independently
6. Case Study: Successful Application of the AAA Pattern in a Real-world Scenario
An engaging example of the Arrange-Act-Assert (AAA) pattern's practical effectiveness can be seen in a project executed by MJC Engineering. They were assigned with the manufacturing of a metal spinning machine for a Japanese firm. A significant hurdle they encountered was the extensive delay in receiving parts from their supplier, which considerably prolonged their machine build times.
In an attempt to mitigate these challenges, MJC Engineering sought the support of Fanuc America. This move resulted in a significant acceleration in the delivery of vital components such as controls, motors, and drives. Fanuc America's prompt response ensured that MJC Engineering received these parts within weeks, a stark contrast to the year-long waits they had previously endured.
The ready availability of domestic parts considerably improved lead times, and the expert advice from Fanuc America's application engineers made the transition to the new control platform seamless for MJC Engineering's team. The outcome was a reliable and accurate machine, delivered on schedule.
The machine crafted by MJC Engineering is pivotal in shaping aluminum liners for high-pressure hydrogen cylinders, a crucial element in Japan's decarbonization strategy. The project's success highlights the value of Fanuc products, such as their CNCs, motors, and drives, in boosting efficiency and guaranteeing dependable performance.
The principles of the AAA pattern were key in structuring the unit tests during this project. The clarity, brevity, and focus on singular behaviors in the tests simplified the process of identifying and resolving bugs, leading to a top-notch product. However, it is important to note that the context does not explicitly detail how Machinet.net's development team applied the AAA pattern for unit testing. Without this specific information, we cannot definitively establish their particular experience with this pattern.
The successful implementation of the AAA pattern in this project serves as a powerful testament to its potential to streamline processes and enhance product reliability in practical situations. However, the context provided does not directly indicate Machinet.net's use of the AAA pattern in their development and testing. Therefore, while we can analyze their approach to development and testing, any direct statements about Machinet.net's successful use of the AAA pattern must be made with caution
7. Adapting the Arrange-Act-Assert Pattern to Evolving Project Needs
The AAA (Arrange-Act-Assert) pattern serves as a reliable structure for unit tests, yet it's not a one-size-fits-all solution. As the project's requirements and objectives evolve, it becomes necessary to adapt the AAA pattern to better cater to these needs. For instance, if a test necessitates a complex setup or teardown process, dividing the test into smaller, more focused tests could prove more efficient.
In line with this, if a test becomes excessively complicated or challenging to comprehend, it's likely that the test is attempting to validate too many behaviors simultaneously. In such scenarios, segmenting the test into multiple tests, each focusing on a single behavior, could be beneficial. This approach aligns with the Single Responsibility Principle in unit testing, which recommends testing one specific behavior or functionality at a time. This not only enhances the clarity and comprehension of the test but also isolates potential issues or bugs to a specific area of the code, simplifying debugging and problem-solving.
Flexibility and adaptability are crucial traits for developers to ensure that their tests remain effective and manageable, even as project requirements shift. This adaptability becomes particularly significant when dealing with complex systems or when implementing advanced architectures, such as the hexagonal architecture. This architectural pattern, also known as the ports and adapters architecture, encapsulates domain logic and decouples it from other implementation details, promoting loose coupling and strong encapsulation.
If a codebase is becoming unwieldy due to legacy code, refactoring can be a valuable tool for developers. Refactoring involves modifying the existing codebase to enhance performance, cater to new requirements, or simply make it more readable and maintainable. It aligns with the principles of the Agile Manifesto, which emphasizes delivering working software frequently and making small changes over time.
When refactoring, working in a separate dedicated branch is important to avoid conflicts with other changes. It's also crucial to have good test coverage to ensure that the new implementation works as expected and doesn't introduce bugs. The use of available attributes, deprecated attributes, or annotations can help to indicate that certain code should not be used and is slated for removal.
Techniques such as branch by abstraction can be employed. This technique involves introducing an abstraction around the legacy code, allowing it to be gradually replaced without breaking the application. Throughout the refactoring process, maintaining clear communication with the team and updating documentation are essential steps to ensure that everyone adopts the new implementation correctly.
Both automated and manual integration testing should be performed to ensure the new implementation works well with the rest of the codebase. Once the new implementation has proven itself, the legacy code can be safely removed. By breaking down the refactor into smaller steps and using dedicated pull requests, the team can avoid being overwhelmed by large changes.
In summary, the AAA pattern, while a powerful tool for structuring unit tests, is not a rigid framework. It can and should be adapted to suit the evolving needs of a project. Whether it's implementing new architectural patterns, refactoring legacy code, or simply breaking down complex tests into more manageable units, flexibility and adaptability are key to maintaining an effective and maintainable testing strategy
Conclusion
In conclusion, the Arrange-Act-Assert (AAA) pattern is a valuable approach to unit testing that provides clear structure and organization to test scenarios. By dividing tests into the Arrange, Act, and Assert phases, developers can enhance the comprehensibility of their tests and focus on testing a single behavior per test. This pattern promotes independent and repeatable tests, making them easier to debug and ensuring code reliability. The AAA pattern can also be adapted to evolving project needs, such as dealing with complex systems or refactoring legacy code.
The broader significance of the AAA pattern lies in its ability to improve the efficiency and reliability of unit tests. By adhering to this pattern, developers can create well-structured tests that are easier to understand and maintain. This leads to higher-quality software products with fewer bugs. The AAA pattern also encourages good testing practices, such as focusing on edge cases and considering failure conditions. By adopting the AAA pattern in unit testing, developers can boost their productivity and create more efficient and reliable software.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.