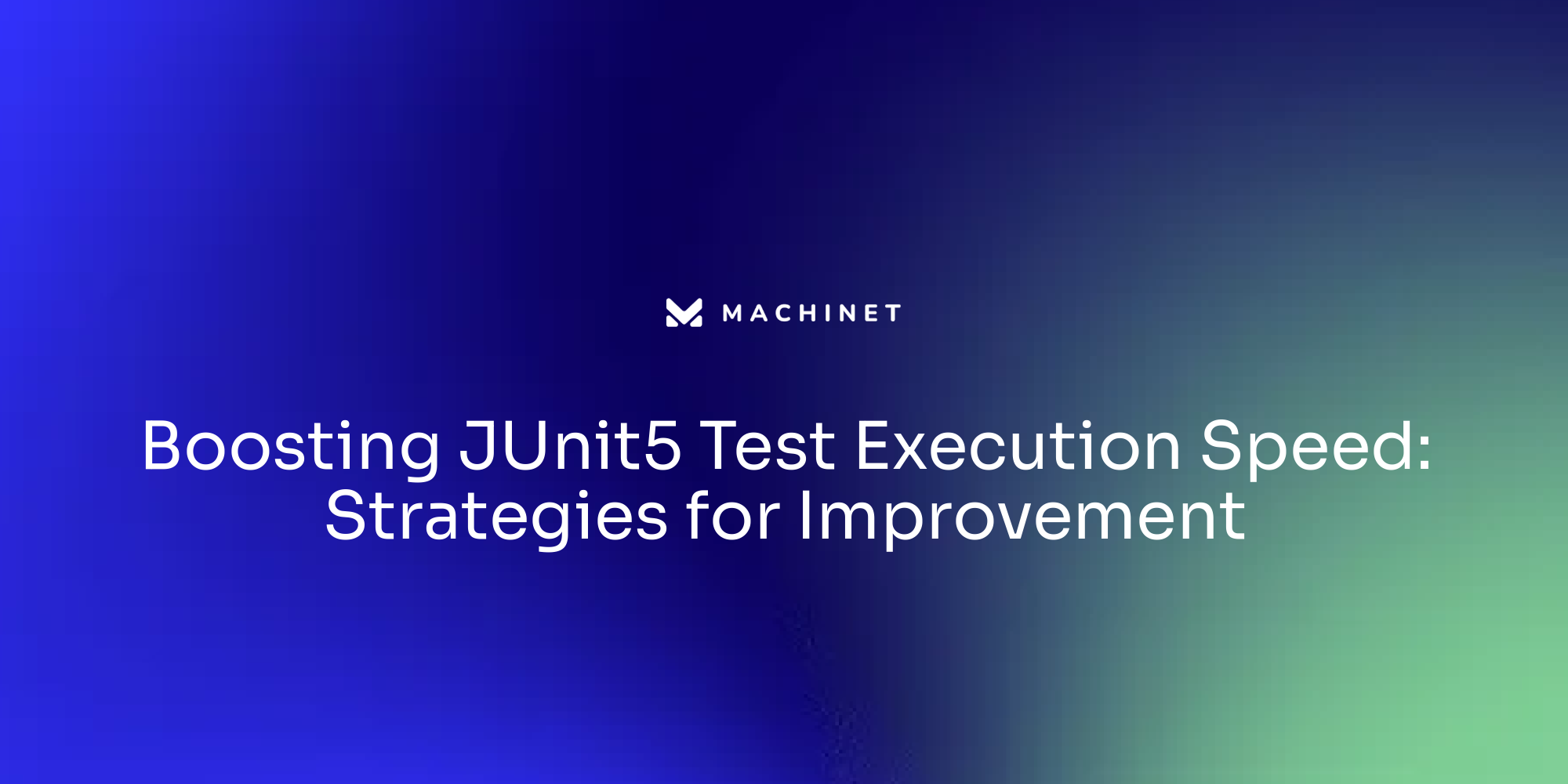
Table of Contents
- Understanding JUnit5: An Overview
- The Need for Speed in Unit Testing
- Key Factors Influencing JUnit5 Test Execution Speed
- Strategies for Boosting JUnit5 Test Execution Speed
- Implementing Optimizations in JUnit5: Practical Examples
- Evaluating the Impact of Optimizations on Test Execution Speed
- Managing Trade-offs Between Speed and Coverage in Unit Testing
Introduction
JUnit5, the latest version of the widely-used JUnit testing framework, brings a range of updates and new features that make it a preferred choice for Java developers seeking efficient unit testing. With its modular architecture and superior support for Java 8 and above, JUnit5 offers enhanced flexibility and improved testing capabilities. The release of JUnit 5.4 introduces streamlined dependency incorporation, simplified creation and cleanup of temporary files, and increased control over test execution order. These advancements, along with comprehensive documentation and practical examples, make the transition to JUnit5 a valuable move for Java developers looking to optimize their testing processes.
Unit testing is a critical part of the software development lifecycle, and executing tests quickly is essential for identifying and resolving issues promptly. This article explores strategies for boosting JUnit5 test execution speed, evaluating the impact of optimizations on test execution, implementing practical examples of optimizations in JUnit5, and managing trade-offs between speed and coverage in unit testing. By understanding these key factors and employing effective strategies, developers can enhance their testing processes, improve efficiency, and ensure high-quality software delivery
1. Understanding JUnit5: An Overview
JUnit5, the most recent iteration of the well-established JUnit testing framework, introduces a suite of updates and new features, making it a preferred choice for Java developers seeking efficient unit testing. JUnit5's architecture is notably modular, which enhances flexibility and provides superior support for Java 8 and later versions.

JUnit5 is essentially composed of three primary modules: JUnit Platform, JUnit Jupiter, and JUnit Vintage. The JUnit Platform lays the groundwork for launching testing frameworks, while JUnit Jupiter offers new programming and extension models. JUnit Vintage ensures compatibility with older JUnit versions.
JUnit 5.4, a newer version of this renowned testing library, streamlines dependency incorporation by enabling the use of a single junit-jupiter dependency. This version also introduces a TempDirectory extension, simplifying the creation and cleanup of temporary files during testing.
In addition to these updates, JUnit 5.4 expands the capabilities of parameterized tests, including support for null and empty values. It also permits dynamic generation of test display names based on nested classes or method names, leading to more intelligible test display names. Moreover, JUnit 5.4 introduces the OrderAnnotation and TestMethodOrder annotations, allowing for the execution of test methods in a specific sequence, thus offering enhanced control over the test execution process.
These advancements, alongside numerous other features available in the JUnit 5 release, make the transition to JUnit 5 a beneficial move for Java developers. The documentation for JUnit 5 is extensive and includes instructive code examples, which simplifies the understanding and application of the new features and improvements for developers. Practical examples and case studies underscore the utility of these features, further emphasizing the advantages of transitioning to JUnit 5.
The process of creating a Gradle project that can compile and run unit tests using JUnit 5 has been simplified, courtesy of the native support for JUnit 5 in Gradle 4.6 and newer versions.
This comprehensive guide covers everything from acquiring the necessary dependencies with Gradle, including the JUnit Jupiter version 5.8.2, to writing a basic test class using JUnit 5. It also provides instructions on how to run unit tests with Gradle using the "gradle clean test" command.
In conclusion, JUnit5, with its modular structure, enhanced flexibility, and improved support for Java 8 and above, brings significant improvements to unit testing in Java.
Upgrade to JUnit 5 for better unit testing
Its three main components, JUnit Platform, JUnit Jupiter, and JUnit Vintage, collaborate to provide a robust and flexible testing framework. With the release of JUnit 5.4, developers can enjoy a more streamlined process of including dependencies, the ability to create and clean up temporary files during testing, more intelligible test display names, and increased control over test execution order. The comprehensive documentation and practical examples facilitate the understanding and application of the new features, further emphasizing the benefits of transitioning to JUnit 5
2. The Need for Speed in Unit Testing
Unit testing, a crucial part of the software development lifecycle, requires a fast execution pace for effectiveness. Swift test executions result in quicker feedback loops, aiding software engineers in swiftly identifying and rectifying issues. This process not only strengthens the code but also enhances the productivity of the development team. However, achieving optimal test execution speed can be a daunting task, particularly when dealing with complex and expansive codebases.
Powerful software project management tools like Maven can help. Maven simplifies the build process, offers a standardized build system, and provides quality project information. It enforces best-practice development guidelines and facilitates a smooth transition to new features. However, Maven isn't without its flaws, such as dependency conflicts, cache resolution problems, and slow build times.
Several strategies can alleviate slow Maven builds. Developers can utilize parallel builds by examining the project's dependency graph and enabling concurrent building when possible. Running Maven tests in parallel can significantly improve build speed. Building only necessary modules instead of the entire project can save time and computational resources. Offline mode can be activated to restrict internet access, preventing Maven from connecting to remote repositories, which often slows down the build process.
Other build tools like Gradle, known for its superior optimizations and the ability to bypass the build phase of a Java project, can also be explored. JRebel is another tool that stands out as it reloads code changes instantly, eliminating the need for building the project, thereby speeding up the development cycle.
To speed up unit test executions, several strategies can be employed.
Optimize your unit tests with Machinet
Reducing unnecessary dependencies and isolating unit tests from external systems or resources using mocking frameworks or stubs can help. The test suite can be optimized by running tests in parallel or grouping them based on their characteristics. Avoiding unnecessary setup and teardown operations in the unit tests can also contribute to faster execution times. The use of test doubles, such as test data builders or test fixtures, can provide pre-configured test objects, contributing to faster test execution.
For large and complex codebases, test parallelization can be effective. By running tests in parallel, the available resources can be leveraged, reducing the overall testing time. Careful test case selection and prioritization can help focus on testing only the critical functionality, avoiding unnecessary tests. Mocking frameworks can be used to isolate dependencies and reduce the overhead of setting up complex test environments. Tools and frameworks specifically designed for testing, such as test runners or continuous integration systems, can help streamline the testing process and improve efficiency.
Finally, optimizing the environment can also contribute to speeding up Maven. This could be achieved by using faster hardware or network connections. The end goal remains the same - to accelerate unit testing and, in turn, the overall development process, thereby ensuring high-quality, efficient software delivery
3. Key Factors Influencing JUnit5 Test Execution Speed
The performance of JUnit5 test execution can be influenced by a variety of factors such as the complexity of the codebase, the number and nature of tests, the efficiency of the testing code, and the performance of the testing environment. For instance, a large and intricate codebase with a comprehensive suite of tests will naturally take more time to execute than a smaller, less complex one. Similarly, if the testing code is not optimized or the testing environment is not up to par, it could result in a significant slowdown in test execution.
In the realm of software development, maintaining swift build speeds is crucial. Slow builds, particularly those that exceed a few minutes in continuous integration (CI), can notably hinder team productivity. This issue becomes especially problematic with multiple pull requests and commits happening simultaneously. While some overhead in build times may be unavoidable due to performance issues with certain tools, such as the Kotlin compiler, or the use of Docker in integration tests, there are several strategies that can be employed to counter these issues and enhance build speeds.
One effective strategy is to run tests concurrently, utilizing more threads than CPUs. This simple yet potent strategy can significantly boost execution speed. Another suggestion is to avoid costly setup and cleanup within tests. Instead, these operations should be performed once to save time and resources.
Tests should also be properly categorized into unit tests and scenario-driven integration tests. While unit tests focus on individual components of the code, integration tests cover code functionality and edge cases through a more comprehensive lens. It's vital to understand the difference between these types of tests and use them effectively to ensure thorough coverage.
Flaky tests, which yield inconsistent results, should be identified and fixed promptly. This process involves understanding the root cause of the flakiness and addressing any underlying technical debt. Moreover, unit and integration tests should be separated to allow builds to fail fast, enabling quicker identification and resolution of issues.
Sleep calls within tests should be avoided as they often indicate flaky tests or naive strategies for testing asynchronous code. Instead, consider using polling, which is a more reliable and efficient approach. Moreover, running tests with more threads than the system can handle can help identify interesting failures under load, which can then be addressed accordingly.
To speed up the execution of JUnit5 tests, you can reduce the number of dependencies and unnecessary code in your tests. This can help to minimize the time it takes to load and initialize the test environment. Additionally, you can use parallel test execution, which allows multiple tests to run simultaneously, improving overall execution time. Another tip is to use test fixtures and test data that are optimized for performance. By using smaller and more focused test cases, you can reduce the time it takes to execute each test.
To improve testing environment performance for faster JUnit5 test execution, optimize the test code, parallelize test execution, and use appropriate test data management techniques. These strategies can significantly reduce the time it takes to execute JUnit5 tests and improve overall testing efficiency.
Finally, remember to keep your build tools up to date, as they are continuously being improved for better performance. Investing in faster CI machines can also help speed up build speeds and eliminate any performance discrepancies between local and CI builds. By understanding what makes builds slow and implementing these strategies, you can significantly improve build speeds and overall productivity
4. Strategies for Boosting JUnit5 Test Execution Speed
Boosting the speed of JUnit5 test execution is feasible through a myriad of strategies, each offering its unique advantages. One such tactic is the refinement of the test code, commonly referred to as code optimization. This process entails streamlining the code to enhance its efficiency, which could involve eliminating unnecessary operations or improving the data structures used.
Simultaneous test execution, or parallel testing, is another strategy that exploits the capabilities of contemporary multi-core processors. It allows multiple tests to run concurrently, thereby reducing the overall execution time. This strategy mirrors the use of parallel builds in Maven, a widely used Java build tool, which also taps into hardware capabilities to speed up build processes.
Selective test execution is another method that proves particularly beneficial when working with large codebases. It involves running only tests directly related to the modifications made, thereby bypassing the need to run irrelevant tests. This strategy mirrors Maven's approach of building only necessary modules, which saves time by avoiding the build of the entire project.
It's crucial to note that these strategies can be effectively combined. For example, parallel test execution can be coupled with selective test execution for a more efficient testing process. Similarly, code optimization can be incorporated with these strategies to prevent the testing code from becoming a bottleneck.
Ultimately, the aim is to reduce testing time without sacrificing the quality of the tests. Just as slow build times in Maven can impede productivity, so can slow test execution in JUnit5. Therefore, the effective implementation of these strategies can lead to more efficient testing processes, allowing software engineers to focus more on development and less on waiting for tests to complete.
Delving deeper into the concept of selective test execution, JUnit 5 offers a robust solution through the use of tags and tag expressions. Tags allow you to group related tests together and run them as a subset, providing a more streamlined approach to executing tests relevant to specific changes.
In JUnit 5, tags can be assigned to tests using the @Tag
annotation. For instance, your tests can be annotated with @Tag("smoke")
or @Tag("regression")
to categorize them.
To run tests based on tags, the --include-tag
or --exclude-tag
command line options can be used. For instance, --include-tag=smoke
will only run tests with the "smoke" tag, whereas --exclude-tag=regression
will exclude tests with the "regression" tag.
Tag expressions further enhance test selection by allowing more complex conditions to be defined. They enable the combination of tags using logical operators such as &&
(AND), ||
(OR), and !
(NOT). For example, --include-tag=smoke && regression
will only run tests that have both the "smoke" and "regression" tags.
The use of tags and tag expressions offers greater control over test execution based on specific criteria or changes. This proves invaluable in scenarios where a subset of tests relevant to a particular change or feature needs to be run, thereby contributing to a more efficient and targeted testing process
5. Implementing Optimizations in JUnit5: Practical Examples
JUnit5, with its myriad of features and strategies, significantly enhances the efficiency of test execution. One such strategy involves the use of the @TestInstance
annotation. This annotation is essential in managing the lifecycle of test instances and aids in reducing execution overhead. By default, JUnit5 creates a new instance of the test class for each test method. However, this behavior can be altered by specifying the @TestInstance
annotation at the class level with the Lifecycle.PER_CLASS
argument, creating a single instance of the test class for all test methods in the class. Developers can also use the @BeforeEach
and @AfterEach
annotations to perform setup and cleanup tasks before and after each test method, irrespective of the test instance lifecycle.
In some scenarios, the order of executing tests is critical. With JUnit5, developers can control this order using the @TestMethodOrder
annotation. This annotation allows you to specify the order in which the test methods should be executed. JUnit5 offers different execution modes for test methods, such as MethodOrderer.OrderAnnotation
, which executes the test methods based on the order specified by the @Order
annotation. Another mode is MethodOrderer.Random
, which randomly orders the test methods for each test class. Additionally, developers can implement their own custom MethodOrderer
by implementing the Orderer
interface.
JUnit5 also introduces the @Disabled
annotation, a valuable tool to bypass tests that are not currently needed, thus expediting the testing process.
Furthermore, JUnit5 provides a versatile tool in the form of the junit-platform.properties
file, that allows you to configure various aspects of JUnit5. To configure JUnit5 using this file, create a file named junit-platform.properties
in the classpath of your project, open the file and add the desired configuration properties, and save the file. This enables customization of various aspects of the JUnit platform, such as enabling or disabling certain test engines, configuring test execution behavior, and setting up custom test listeners.
Beyond these features, JUnit5 comes with a robust extension model that allows developers to extend the behavior of test classes and methods by implementing interfaces such as TestInstancePostProcessor
, ExecutionCondition
, ParameterResolver
, or TestExecutionExceptionHandler
. These extensions can be used to add additional information to test methods, resolve parameters, manage exceptions, and more. Developers can use the @ExtendWith
annotation to leverage these custom extensions, or register the extension via a configuration file, making it available for all tests.
Moreover, JUnit5 supports parameterized tests, which allow tests to retrieve strings as parameters using a ParameterResolver
extension. This is particularly beneficial when dealing with a large number of input combinations.
JUnit5 also offers lifecycle extensions that provide valuable insights into the start and end time of each unit test, an essential factor when evaluating test execution speed.
Additionally, JUnit5 has the capability to handle exceptions within tests, such as ignoring IOExceptions, which can be achieved by creating a TestExecutionExceptionHandler
extension. This helps in maintaining a smooth testing process, even when unexpected exceptions occur.
In essence, the vast array of features and optimizations that JUnit5 offers provide developers with a flexible and efficient testing environment, making it a popular choice among seasoned software engineers
6. Evaluating the Impact of Optimizations on Test Execution Speed
Profiling and optimizing test execution speed is a vital part of improving software testing efficiency. By comparing the execution times before and after performance enhancements, you can assess the effectiveness of your modifications. JUnit5 provides several tools to aid in this evaluation, including TestExecutionSummary, which offers a comprehensive overview of test execution, including the total execution time. Additionally, you can employ external tools like JUnit Benchmark or JMH (Java Microbenchmark Harness) to measure your tests' performance.
To illustrate the practical application of these tools, let's consider the experience of a developer who was tasked with enhancing the speed of system tests for a sizeable Ruby on Rails application. This application had a significant codebase, with 38 million lines of code and a decade-long history. The developer encountered a bug within the test environment causing notable delays when serving a GraphQL request.
Despite identifying the issue using Datadog profiles, the developer lacked the necessary details to fully understand the problem. They replicated the issue on a local machine and found that the GraphQL queries were taking an unusually long time to execute. The developer hypothesized that the issue was related to threading and concurrency, with multiple requests being processed by a single worker process with multiple threads, leading to slow response times.
However, running the system tests with a single-threaded process did not resolve the problem. Further investigation revealed that the file update checker was taking a significant amount of time. The developer discovered that two different file update checkers were being used in the development and test environments. After benchmarking the default file update checker, it was found to be slower than the evented file update checker. Following this discovery, the developer changed the file watcher in the test environment, which made most of the system tests 40% faster to run locally.
This example underscores the importance of profiling, following leads, and benchmarking to improve performance. Furthermore, it illustrates how tools like JMH can be utilized to accurately measure the execution time and other metrics of your tests, helping you optimize your code for better performance.
Another example involves a developer at Tag1 Consulting experimenting with the Goose load testing tool. The developer sought to further optimize the performance of Goose and experimented with various compile-time optimization techniques. The results showed that adding link-time optimization (LTO) with the release flag increased network traffic and generated more requests.
However, some techniques did not show significant improvement or even resulted in slower load tests. The developer concluded that the release flag provided the largest optimization with minimal additional testing. Other optimizations, such as profile-guided optimization (PGO) and specific memory allocators, may not be worth the effort for most load tests.
These examples highlight the importance of evaluating the impact of optimizations on test execution speed and demonstrate how tools like JUnit5 and JMH can be used to measure performance improvements. The process involves benchmarking, identifying bottlenecks, and implementing changes to enhance performance, all of which are critical in ensuring efficient and effective software testing.
To further improve test execution speed with JUnit5 optimizations, consider the following approaches:
- Parallel Test Execution: JUnit5 supports running tests in parallel. By utilizing parallel execution, you can distribute the workload across multiple threads or processes, leading to faster execution times.
- Test Instance Lifecycle: JUnit5 introduces different lifecycle modes for test instances, such as per-class and per-method. By selecting the appropriate lifecycle mode, you can optimize the creation and destruction of test instances, reducing overhead and improving execution speed.
- Conditional Test Execution: JUnit5 allows you to conditionally execute tests based on specific conditions. By leveraging conditional execution, you can skip tests that are not relevant to the current scenario, resulting in faster test execution.
- Test Method Ordering: JUnit5 provides annotations like
@TestMethodOrder
and@Order
to define the order of test method execution. By optimizing the execution order, you can prioritize critical tests and reduce overall execution time. - Test Data Generation: JUnit5 offers support for parameterized tests and dynamic tests. By generating test data dynamically or using parameterized tests, you can execute a larger number of test cases with minimal duplication, leading to faster test execution.
Remember, the specific optimizations you choose will depend on the nature of your tests and the performance bottlenecks you encounter. Experimenting with different approaches and measuring the impact on execution speed can help you identify the most effective optimizations for your scenario
7. Managing Trade-offs Between Speed and Coverage in Unit Testing
Unit testing plays a pivotal role in the development of dependable software. A key metric of its efficacy is 'test coverage,' which refers to the extent of your codebase examined by your tests. Nevertheless, it's equally vital for tests to be executed rapidly. Striking a balance between comprehensive test coverage and speed optimization is the crux of the issue.
The mnemonic 'FIRST' encapsulates the principles of effective unit testing. 'F' signifies 'Fast.' The pace of conducting tests is crucial as frequent testing helps promptly identify issues, reducing the chances of errors infiltrating the codebase.
'I' stands for 'Isolated.' Each test should be self-contained with a singular reason to fail. Independence from external factors and other tests ensures their validity and reliability.
'R' represents 'Repeatable.' Tests should consistently yield the same results, irrespective of when or where they are run. This consistency assures the reliability of tests and that any failures genuinely indicate issues within the codebase.
'S' denotes 'Self-verifying.' Tests should conclusively pass or fail, leaving no room for interpretation or ambiguity. The results should clearly indicate the code's correctness.
Lastly, 'T' stands for 'Timely.' The most effective tests are those written concurrently with or before the production code. This method helps define the desired behavior and ensures that the code is testable from the start.
While speed is a crucial aspect of testing, it shouldn't compromise coverage. A fast test suite might offer quick feedback, but if it's not comprehensive, it could overlook significant issues. Therefore, it's essential to balance speed and coverage. In essence, we need as many fast tests as possible for rapid feedback, complemented by enough slow, comprehensive tests to ensure full coverage of the desired behavior.
Maintaining a swift test suite requires discipline, consistent refactoring of test code, and setting a limit on test suite duration. If a test suite begins to slow down, it's important to address this immediately by identifying and fixing the slowest tests, separating quick tests from slow ones, and keeping a strict limit on test suite duration.
To maximize coverage and efficiency, several factors should be taken into account while prioritizing test cases. Firstly, it is important to identify the critical and high-risk areas of the software or system being tested and give them higher priority in terms of test case coverage. Test cases that have no dependencies on others should also be prioritized as they can be executed independently, providing quick feedback. Additionally, prioritize test cases that cover frequently used functionality or those of high importance to end-users. Considering execution time and resources required for each test case can also significantly improve efficiency.
Automated approaches can help increase test coverage while maintaining fast execution. These include prioritizing test cases, using code coverage tools, employing test generation tools, integrating automated tests into your CI/CD pipeline, and managing test data. By combining these approaches, you can automate the testing process, focus on the critical areas of the application, and maintain overall test coverage.
To improve the effectiveness of your unit tests, focus on testing the core functionality and critical aspects of the code, use meaningful test cases, maintain test isolation, prioritize test execution, automate tests, mock external dependencies, and continuously refactor tests. By adhering to these strategies, you can write efficient unit tests that offer high coverage, ensuring the stability and reliability of your code
Conclusion
In conclusion, JUnit5 brings significant improvements to unit testing in Java with its modular structure, enhanced flexibility, and improved support for Java 8 and above. The release of JUnit 5.4 introduces streamlined dependency incorporation, simplified creation and cleanup of temporary files, and increased control over test execution order. These advancements, along with comprehensive documentation and practical examples, make the transition to JUnit5 a valuable move for Java developers looking to optimize their testing processes. With its robust extension model, support for parallel test execution, selective test execution, and customizable test instance lifecycle, JUnit5 offers developers a flexible and efficient testing environment.
The need for speed in unit testing is crucial for identifying and resolving issues promptly. Slow test execution can hinder productivity and delay the software development process. Strategies such as parallel test execution, selective test execution, code optimization, and proper test categorization can significantly boost test execution speed. By reducing unnecessary dependencies, isolating tests from external systems or resources, optimizing the test suite structure, and utilizing various testing tools and frameworks designed for performance improvement, developers can enhance their testing processes and ensure high-quality software delivery.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.