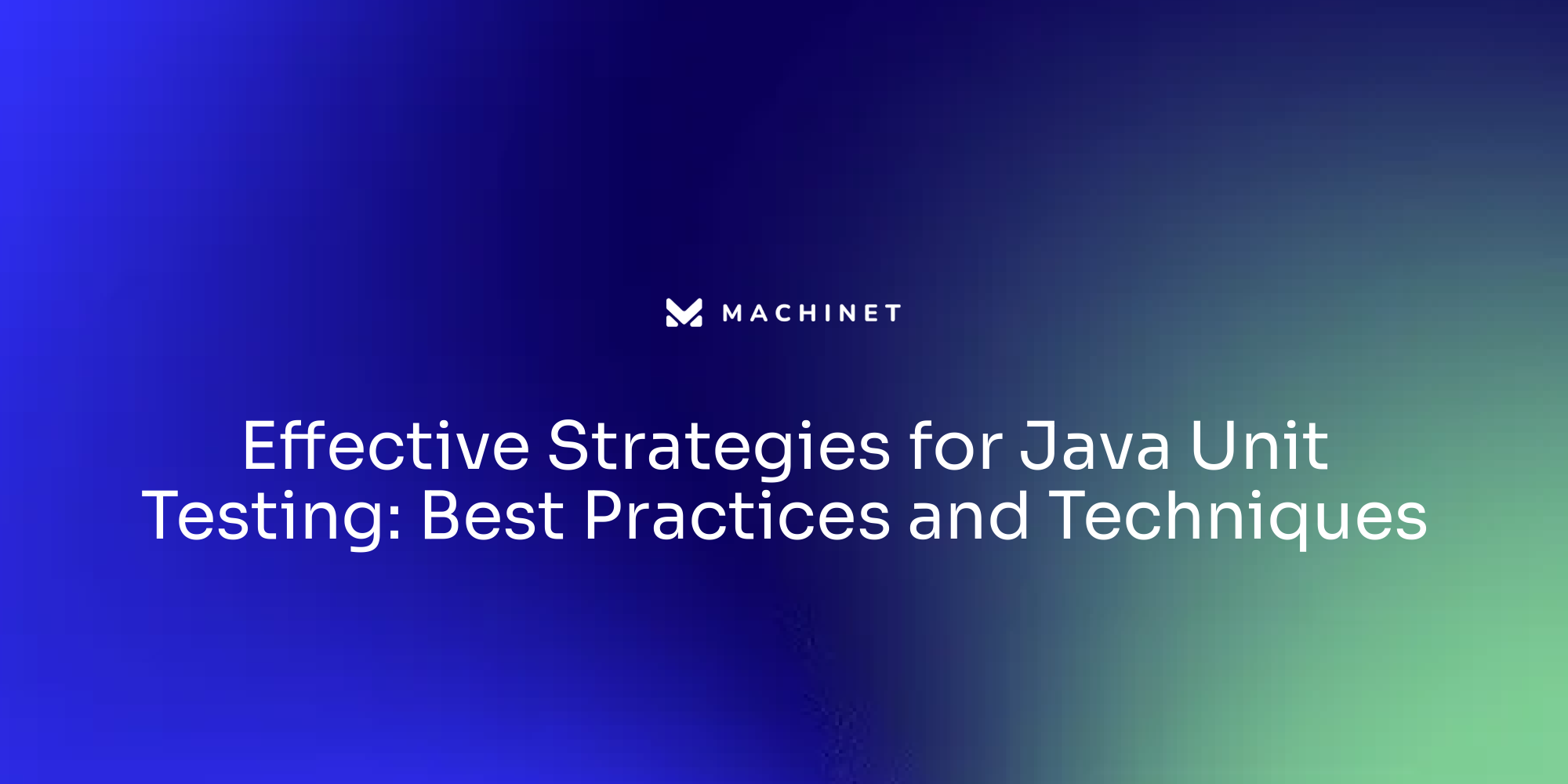
Table of contents
- Understanding Unit Testing in Java
- Writing a Basic Unit Test with JUnit
- Strategies for Writing Testable Code in Java
- Techniques for Testing RESTful APIs in Java
- Ensuring Comprehensive Test Coverage in Java Unit Testing
- Role of Mocking Frameworks and Handling Dependencies with Mocks in Java Unit Testing
- Effective Use of Integration with Build Tools for Streamlined Java Unit Testing
- Balancing Workload Management and Deadline in Java Unit Testing
Introduction
Unit testing is a fundamental aspect of Java development, ensuring the accuracy and reliability of individual code segments. By creating specific test cases for each function or method, developers can isolate program segments and verify their correct operation. Unit testing frameworks like JUnit and Mockito play a pivotal role in Java unit testing, providing tools for creating mock objects, simulating behavior, and verifying system behavior during testing. In this article, we will explore the importance of unit testing in Java, best practices for writing effective unit tests, the role of mocking frameworks like Mockito, and the integration of unit testing with build tools like Maven and Gradle. By following these strategies and techniques, developers can enhance the quality and stability of their Java code through comprehensive and efficient unit testing
1. Understanding Unit Testing in Java
Unit testing is a fundamental aspect of Java development, a methodical approach that verifies the accuracy of individual code segments.

The smallest testable units of a software application such as methods or classes are the usual focus. The main goal of unit testing is to isolate each program segment and ensure its correct operation. This is achieved by creating specific test cases for each function or method, designed to scrutinize the function independently. A unit testing framework like JUnit is commonly used in Java for this purpose.
Unit testing plays a pivotal role in bug detection and prevention, providing a safety net for code refactoring and maintenance.
Start unit testing your code with Machinet and save time and effort!
It serves as a living document of code behavior, promoting component isolation and modular design, thus speeding up the development process. Unit tests are key in continuous integration and deployment, contributing to code quality assurance and regulatory compliance standards. They facilitate collaboration and communication among developers, testers, and stakeholders, instilling confidence and reliability in the codebase.
In Java unit testing, certain best practices ensure the effectiveness and reliability of the tests. These practices include writing independent tests, testing boundary conditions, using meaningful test names, using assertions, testing edge cases, keeping tests concise, and regularly refactoring tests. Each unit test should be independent of other tests. It is crucial to test both the normal and boundary conditions of the code being tested. Test names should clearly indicate what is being tested, making it easier to understand and identify any failures. Assertions should be used to check the expected output or behavior of the code being tested. Tests should be kept concise and focused on testing a specific unit of functionality. As the codebase evolves, it is important to refactor the tests to keep them aligned with the changes in the code.
JUnit, a widely-used testing framework, provides annotations and assertions for writing and executing unit tests. JUnit uses annotations to define test methods, test classes, and test suites. The @Test
annotation marks a method as a test method. In the test method, assertions using methods like assertEquals()
or assertTrue()
verify the expected behavior of your code. Other annotations like @Before
and @After
define setup and teardown methods that run before and after each test method. These methods can be used to initialize or clean up resources used by your tests. To execute your JUnit tests, you can use an IDE like Eclipse or IntelliJ, which provide built-in support for running JUnit tests. You can also use the JUnitCore
class provided by JUnit to run your tests programmatically.
Automated unit testing tools offer various advantages such as test frameworks and runners, code coverage analysis, integration with continuous integration, mocking and test doubles, parameterized testing, test execution reporting, test data management, test prioritization, integration with IDEs, and cross-browser and cross-platform testing. Manual unit testing involves selecting the unit to test, setting up the test environment, defining test cases, arranging, acting, and asserting, handling exceptions, repeating test cases, test clean-up, running the unit test, analyzing test results, and maintaining the test code as the codebase evolves.
Incorporating unit testing into the development process and using automated unit testing tools can streamline development, enhance collaboration, and foster a culture of continuous improvement. This ensures the correctness and robustness of the code, promotes bug detection and maintainability, and contributes to overall code quality and stability. This article provides a comprehensive tutorial on writing unit tests in Java by following best practices and using JUnit for unit testing
2. Writing a Basic Unit Test with JUnit
JUnit 5 stands as a formidable framework for unit testing in the realm of Java.
Upgrade your unit testing capabilities with Machinet's AI-powered plugin!
It's array of features streamline the testing process, making it a tool of choice for many developers. The heart of JUnit 5 revolves around the creation of a test class, typically named after the class it tests with a 'Test' suffix. Inside this class, individual test methods are defined, each representing a unique test case. These methods are then marked with the @Test
annotation.
JUnit 5 places a strong emphasis on effective naming conventions and organization. This is to ensure that each test's purpose is clear, enhancing readability and understanding of the test suite.
The framework also provides a multitude of assertion methods, including assertEquals()
. These methods are used to compare expected and actual results of a test. To further increase readability of test code, it's recommended to leverage static imports for JUnit assertions.
The advanced features JUnit 5 offers include dynamic and parameterized tests, testing for exceptions, assumptions, and defining timeouts for tests. It also allows for disabling tests when needed.
The concept of nested tests is another unique feature of JUnit 5. This facilitates a more structured approach to writing tests. Additionally, the @TempDir
annotation is introduced for creating temporary files and paths for tests.
The use of @BeforeEach
methods for test setup is supported by JUnit 5. This helps in reducing redundancy in test code. It also allows for grouped assertions via assertAll
and repeated test execution via the @RepeatedTest
annotation.
To manage and navigate large test suites more easily, tags and display names can be used. This improves organization and readability of tests.
Regarding project setup, JUnit 5 tests can be written and executed using popular build tools and IDEs such as Maven, Gradle, and Eclipse. The framework also has upcoming support for test suites, making it more versatile and robust.
JUnit 5 encourages a hands-on approach to learning by providing examples and exercises for writing unit tests for a data model and services. This gives developers practical experience with the framework, solidifying their understanding of unit testing in Java.
Lars Vogel once stated, "The following code defines a minimal test class with one minimal test method". This statement underlines the simplicity and elegance of writing tests with JUnit 5. He further noted, "The initialization of myclass happens in every test move the initialization to a beforeeach method", emphasizing the importance of using setup methods to avoid redundancy in test code.
In essence, JUnit 5 is a comprehensive and robust framework for unit testing in Java. It offers a plethora of features and functionalities that enable developers to write effective and organized tests. It emphasizes best practices such as proper test naming, organization, and the use of setup methods, and it supports advanced testing techniques such as dynamic and parameterized tests, nested tests, and more. Furthermore, with its support for popular build tools and IDEs, JUnit 5 is a versatile and flexible choice for any Java developer looking to enhance their unit testing skills.
To write a basic unit test in JUnit, the steps are simple.
Simplify your unit testing process with Machinet's context-aware AI chat!
First, import the necessary JUnit libraries into your project. Then, create a new test class for the class you want to test. This test class should be in the same package as the class under test. Add the @Test
annotation to the test method you want to write. This annotation indicates that the method is a test case. Write the test logic inside the test method, which can include calling methods from the class under test and asserting the expected results using JUnit's assertion methods. Finally, run the test class as a JUnit test to execute the test cases. By following these steps, you can write basic unit tests in JUnit to verify the behavior and correctness of your code
3. Strategies for Writing Testable Code in Java
Writing testable Java code is an intricate process that requires a strategic blend of principles, techniques, and careful construction.

It's about enhancing the testability, maintainability, and overall quality of your code.
It's vital to ensure that your Java code is written in a manner that allows for effective testing. One key technique is adhering to the Single Responsibility Principle (SRP), which advocates that each class should only have one reason to change. This approach simplifies testing and makes maintenance more manageable.
Another pivotal technique is Dependency Injection (DI), which can boost the flexibility and testability of code by decoupling class dependencies. It's paramount that classes only request the dependencies they genuinely require. For instance, if a vehicle class requires an engine, it should request the engine directly, not a factory to manufacture its own.
When building objects for DI, factories can provide substantial assistance. These classes handle the creation and configuration of all necessary objects, streamlining the process. However, they should be used judiciously. For example, if one object needs to create another, it's recommended to pass an OtherObjectFactory
into OneObject
's constructor, allowing you to create a MockOtherObject
and MockOtherObjectFactory
for your tests.
The constructor itself should be crafted prudently, avoiding substantial work and being as lenient as possible to allow tests to pass in mocks and nulls more easily, thus enhancing testability.
Simplicity and clarity are also paramount in writing testable code. This includes using meaningful names for variables and methods, maintaining short and focused methods, and avoiding complex conditional logic.
Objects should be categorized as either 'injectables' or 'newables'. Injectables are objects that are injected into others, meaning objects depend on them. These objects must be created by a DI framework and therefore cannot require any custom values in their constructors. Conversely, newables are objects created by passing only custom values to their constructor. These objects are easy to construct and are generally low on behavior. They should be positioned at the end of your object graph for optimal testability.
Lastly, be cautious of elements that can make code brittle or testing challenging. These include global state, which can affect the outcome of tests, public static methods and properties, which can create a form of global state, and singletons. The latter, while enforcing a limit of one instance, can make code inflexible and less reusable.
To write testable code in Java, it's crucial to follow certain best practices like separating concerns by dividing your code into smaller, focused modules that handle specific tasks, making it easier to test each module independently. Limit the use of static methods and global state, as they can make code challenging to test. Program to interfaces and abstractions rather than concrete implementations, allowing you to easily substitute implementations with mock objects during testing. Develop comprehensive unit tests for each module or class, covering different scenarios and edge cases to ensure the code behaves as expected. Consider using Test-Driven Development (TDD), where tests are written before the actual implementation, ensuring that the code is written with testability in mind from the start. Utilize mocking frameworks like Mockito to easily create and manage mock objects during testing. Mock objects can simulate the behavior of dependencies and allow you to focus on testing specific units of code.
By following these techniques and best practices, you can write testable Java code that is easier to maintain, debug, and evolve over time
4. Techniques for Testing RESTful APIs in Java
In the realm of Java, there are an array of methodologies and tools available for testing RESTful APIs.

One common method is leveraging the Spring MVC Test framework, a robust suite of tools designed specifically for testing web applications without the need for a running server. It facilitates sending HTTP requests directly to the Controller method, thereby enabling assertions on the response.
An integral part of the Spring MVC Test framework is the MockMvc class. It is designed to simulate HTTP requests to Spring MVC controllers and allows for response verification. Initializing a MockMvc instance involves creating it using the standaloneSetup() method with your controller class as an argument. Once initialized, the perform() method of the MockMvc instance can be used to send HTTP requests, specifying the HTTP method, URL, and any request parameters or headers as needed.
javaMvcResult result = mockMvc.perform(get("/api/users")) .andExpect(status().isOk()) .andReturn();
In the example above, a GET request is sent to the "/api/users" endpoint, and the response status is asserted to be 200 OK. The andExpect() method, combined with various matchers provided by the Spring Test framework, can be used to assert the response. For instance, the content() matcher can be used to verify the response content:
javamockMvc.perform(get("/api/users")) .andExpect(content().json(expectedJson));
In this example, the response content is asserted to match the expected JSON. Utilizing MockMvc in Spring MVC tests provides a controlled and predictable way to send HTTP requests and assert responses, making it a potent tool for testing Spring MVC controllers.
Another technique that has been gaining traction is using Rest Assured. Pioneered by Johan Haleby, Rest Assured has been revolutionizing the way REST services are tested in Java. It brings the simplicity and flexibility of dynamic languages like Ruby and Groovy into the Java domain. Rest Assured is a Java library that simplifies the testing of RESTful APIs. It provides a fluent interface that allows you to specify the HTTP request, parameters, headers, and body for your API tests easily.
For instance, you can use Rest Assured's given-when-then syntax to set up the initial state of the test, perform the HTTP request, and assert the expected response. Moreover, Rest Assured offers additional features for testing RESTful APIs, such as authentication, handling cookies, and validating JSON or XML responses.
On the other hand, tools like Postman can be used for testing HTTP requests and validating responses. Postman supports various types of requests, including GET, POST, PUT, and DELETE, and can validate the response status, headers, and body. However, Rest Assured, with its Java-centric approach and continuous improvements, remains a compelling option for Java developers.
For deeper integration testing of REST APIs, JUnit offers a potent solution. It allows for the testing of the system from an external perspective, examining the HTTP response code, response headers, and payload (JSON/XML). It is also advisable to automate API tests to run them repeatedly and consistently, saving time and effort. Tools like Postman, RestAssured, or Newman can be used for this purpose.
Whether it's leveraging the power of the Spring MVC Test framework, exploring the capabilities of Rest Assured, or utilizing JUnit for integration testing, there are numerous avenues for effectively testing RESTful APIs in Java. Each tool and technique offers its unique strengths, and the choice ultimately hinges on the specific requirements and context of the testing scenario
5. Ensuring Comprehensive Test Coverage in Java Unit Testing
Java unit testing is not a mere box-ticking exercise.

It's a critical process that thoroughly examines each class, method, and line of code. The use of tools such as JaCoCo and Cobertura can aid this process by providing a detailed analysis of the percentage of code that has undergone testing. This data is crucial in identifying areas that need further testing. However, while striving for high code coverage is praiseworthy, it's equally important to formulate tests that corroborate the correctness of the code, rather than simply focusing on reaching high coverage statistics.
In the quest for comprehensive test coverage, the experiences of industry leaders like Amazon can provide valuable lessons. Over the past decade, Amazon's approach to unit tests and code coverage has seen a significant shift. The absence of defined metrics or gates for rigorous unit testing previously led to subpar code coverage. By 2021, code coverage had become an integral part of Amazon's development process. It's now a key component of the pre-submit build process, and code reviewers can easily identify which lines of code are covered by tests.
This shift can be, in part, attributed to the creation of a tool designed to identify and comprehend test gaps. The tool initially faced resistance and wasn't widely adopted. However, when data-driven reports highlighting test gaps and low code coverage were presented, the company began to put a new emphasis on improving test coverage. This change in focus led to the tool's widespread adoption by hundreds of teams, resulting in a significant improvement in code coverage statistics.
The Cyber Defence Lab of the Royal Military Academy in Belgium also offers insights into achieving comprehensive code coverage. They use Java and Maven for their projects, with JUnit tests used for testing. They emphasize the importance of code coverage in testing and explain how to measure it using the Jacoco Maven plugin. They provide guidance on generating a code coverage report in various formats (HTML, XML, CSV) using the Jacoco plugin and how to automatically generate a code coverage report during the Maven verify phase.
Achieving comprehensive test coverage in Java unit testing is a complex process that requires rigorous testing of all code elements, the use of code coverage tools, and the crafting of meaningful tests. The experiences of Amazon and the Cyber Defence Lab underscore the importance of adopting a disciplined approach to unit testing, using data to identify test gaps, and leveraging tools like the Jacoco Maven plugin to measure and enhance code coverage.
To achieve comprehensive test coverage in Java, it's crucial to follow best practices. This includes writing unit tests that cover all potential scenarios and edge cases, using test-driven development (TDD) to ensure tests are written before the actual code, and using code coverage tools to measure the effectiveness of the tests. In addition, it's important to use mocking frameworks to isolate dependencies and make tests more focused and efficient. As the codebase evolves, regular review and updating of test cases are also crucial to maintain comprehensive test coverage.
To verify code correctness in Java unit testing, various techniques and tools can be used. A common approach is to use assertions to check that the expected outputs match the actual outputs. By writing test cases that cover different scenarios and edge cases, the code can be ensured to behave as expected. Additionally, code coverage tools can be used to measure the extent to which the tests cover the code. This can aid in identifying areas that may need further testing. Another technique is to use mocking frameworks, such as Mockito, to simulate dependencies and isolate the code under test. This allows for specific units of code to be tested without worrying about the behavior of external dependencies.
In unit testing, meaningful tests are of great importance. They help ensure that the code being tested behaves as expected and produces the desired results. Meaningful tests provide a clear understanding of what the code is supposed to do and what the expected outcomes are. This makes it easier to identify and fix any issues or bugs in the code. Additionally, meaningful tests serve as documentation for the code, making it easier for other developers to understand and work with the code in the future. They also help in maintaining the codebase by providing a safety net when making changes or refactoring the code. Overall, meaningful tests play a crucial role in ensuring the quality and reliability of the software being developed
6. Role of Mocking Frameworks and Handling Dependencies with Mocks in Java Unit Testing
Mockito, a renowned open-source Java framework, is a key player in the sphere of unit testing. It primarily assists in handling dependencies by creating mock objects that simulate the behavior of actual objects. This becomes crucial when real objects cannot be feasibly included in the unit test, such as a database dependency.
Mockito makes it possible to create these mock objects and verify system behavior during testing. It's often used alongside JUnit, a reputable Java testing framework. If a class under test has dependencies, Mockito can create mock objects to simulate these dependencies, enabling isolated testing without the actual dependencies.
Moreover, Mockito is handy when testing methods with side effects, like writing to a database or making API calls. By mocking these external dependencies, Mockito can verify the correct interactions. It also offers features for verifying method invocations, stubbing method calls to return specific values, and handling exceptions. These functionalities grant more control over the mock objects' behavior during testing, which simplifies creating robust and maintainable test suites.
To ensure the effective use of Mockito, it's advisable to follow certain guidelines. For instance, Mockito's @Mock
annotation is recommended for creating mock objects without explicitly writing the creation code. Mockito's @InjectMocks
annotation is useful for injecting dependencies into the test class, simplifying the functionality testing of the class.
The verify()
method in Mockito is used to verify the behavior of mock objects, allowing developers to check if certain methods have been called on the mock objects with expected parameters. The when()
method is used to specify the behavior of mock objects, defining the return values or behaviors when certain methods are called.
After each test, it's important to clean up the mock objects using Mockito's @After
annotation or the MockitoJUnitRunner
class. This maintains the tests' integrity and prevents unwanted interactions between the tests. By adhering to these best practices, developers can effectively use Mockito in Java unit tests and ensure the reliability and accuracy of their tests.
In Mockito, the when
and thenReturn
methods are used to stub behavior. The when
method defines the method call to be stubbed, and thenReturn
specifies the value to be returned when that method is called. This helps control the behavior of the mocked object during testing. For instance, a mock object of SomeClass
can be created using Mockito.mock
. Then, the when
method can stub the behavior of the someMethod
method to return someValue
when called. Finally, the method is called, and assertions verify that the expected behavior is achieved.
```Java// Create a mock objectSomeClass someObject = Mockito.mock(SomeClass.class);
// Stub the behavior of a methodMockito.when(someObject.someMethod()).thenReturn(someValue);
// Call the method and verify the behaviorassertEquals(someValue, someObject.someMethod());```
In this example, necessary Mockito classes are imported (import org.mockito.Mockito;
), and the assertEquals
method from JUnit or a similar testing framework is used for assertions. By using Mockito to stub behavior in Java unit tests, different scenarios can be easily simulated to ensure that the code functions correctly.
However, it's important to note that the comparison of Mockito with other mocking frameworks in Java requires additional context information not provided here. Similarly, the Mockito documentation and user guide are not directly mentioned in the provided context.
In conclusion, Mockito's versatility in mocking final classes and static methods, and its ability to modify private fields and call private methods on objects using reflection, makes it an indispensable tool for any software engineer involved in Java unit testing
7. Effective Use of Integration with Build Tools for Streamlined Java Unit Testing
Harnessing the power of build tools, such as Maven and Gradle, in the Java unit testing workflow can greatly streamline and simplify the process. These tools can be configured to automatically run unit tests during the development cycle, proficiently managing dependencies, compiling the codebase, and packaging the application. Furthermore, they generate insightful reports that can help improve code quality. This integration not only conserves time but also guarantees a consistent testing environment. Moreover, the synergy between these build tools and Continuous Integration (CI) servers facilitates automatic test execution whenever changes are made to the code repository.
To effectively perform Java unit testing with Maven, the following steps can be adopted:
- Incorporate the necessary dependencies for unit testing in the Maven project's pom.xml file, such as JUnit and Mockito.
- Develop unit tests using JUnit, a popular testing framework for Java.
- Employ Mockito, a mocking framework for Java, to create mock objects and simulate behavior for dependencies.
- Execute unit tests using the Maven command "mvn test" or by running the test phase in your preferred IDE.
Maintaining swift build speeds is an essential facet of software development. Slow builds can impede team productivity, particularly when multiple commits and pull requests are happening concurrently. Some overhead is inevitable, but it's valuable to invest time in optimizing build performance. This optimization can involve running tests concurrently, differentiating between unit and integration tests, and keeping build tools updated. Avoiding sleep calls in tests and fixing flaky tests also contribute to improved build speeds. Utilizing faster CI machines tailored to the team's preferences and needs can be beneficial.
Automating unit tests with build tools significantly enhances the efficiency and reliability of the software development process. Tools like Jenkins or Travis CI can be used to trigger the execution of unit tests automatically whenever changes are made to the codebase, ensuring that new code additions or modifications do not introduce regressions or break existing functionality. These tools also generate reports or notifications to alert developers of any test failures, enabling them to swiftly identify and resolve issues.
Integration tests play a pivotal role, especially in specific tech stacks like a Solr plugin project, to ensure the plugin's functionality across different Solr versions. The Solr test framework offers tools for writing both JUnit and integration tests. The procedure of integration testing involves launching a Solr server, loading a product catalog, and verifying if the plugin correctly handles query rewrites. Tools such as Maven, Docker, and Testcontainers are typically employed in this process. Maven functions as the build tool, Docker runs Solr containers, and Testcontainers, an extension to JUnit, spins up Docker containers.
Integration tests are distinguished from unit tests using JUnit categories. The Maven Surefire Plugin is configured to exclude integration tests during unit testing and include them during integration testing. Testcontainers is used to launch a Solr container from JUnit tests, and the SolrContainer class is extended to bind mount artifacts and test configsets into the container. Maven build properties are injected into the JUnit test to locate the built plugin jar. The parameterized extension of JUnit is used to run the same test case for multiple versions of Solr. The Solr versions to test against are injected via a system property. This process of adding integration tests to Solr plugins underscores the benefits of integrating build tools into the Java unit testing workflow
8. Balancing Workload Management and Deadline in Java Unit Testing
The intricacies of managing workload and meeting deadlines in Java unit testing can be daunting. To navigate this labyrinth effectively, a strategic approach is paramount. Identifying high-risk areas in the code serves as the starting point. These areas, if compromised by a defect, can lead to significant damage. Concentrating our efforts on these regions first allows us to address the most substantial risks upfront.
The complexity of the code is a crucial consideration. Over 200 different complexity measures can signal potential trouble spots in the software. By pinpointing these areas, we can focus our testing efforts where they will yield the most value.
After the high-risk and complex areas are identified, a risk-based approach helps determine the priority of the tests. This strategy considers factors such as visibility, frequency of use, and potential cost of failure. Assigning weights to these factors and computing a weighted sum for each system area lets us discern which areas warrant the most attention.
Adopting a stepwise release strategy is beneficial. This strategy involves testing the most critical functions and features first, while deferring others. This gradual release of the software aids in workload management and ensures the most vital aspects of the software are tested first.
Defect-prone areas warrant special attention. Historical data shows that defects tend to cluster in certain areas of the code. By zeroing in on these areas and generating more tests targeting the types of previously detected defects, we can enhance the effectiveness of our testing process.
Automated testing is an essential element of workload management in Java unit testing. Automation tools execute tests swiftly and consistently, enhancing the efficiency of the testing process. Companies that have adopted automation have found it to be more cost-effective and efficient than manual testing.
Finally, sufficient time allocation for testing in the project schedule is key to managing the testing workload and meeting deadlines. This includes time for writing tests, running them, and addressing any issues they uncover. A test management tool can be a valuable asset in tracking the testing progress and managing the workload.
To balance workload management and deadlines in Java unit testing, a combination of strategies is necessary. These include prioritizing high-risk and complex areas, adopting a risk-based approach to testing, implementing a stepwise release strategy, focusing on defect-prone areas, utilizing automated testing, and allocating sufficient time for testing. By adopting these strategies, we can enhance the efficiency and effectiveness of our testing process, leading to higher software quality.
When it comes to Java unit testing, it is important to adhere to several best practices. Proper code organization is vital, as it makes the test code easier to understand and maintain. High test coverage is another critical practice, which involves ensuring that unit tests cover as much of the code as possible, including all possible branches and edge cases. Test design is another crucial aspect of effective unit testing, with tests designed to be independent of each other for easier debugging and maintenance. Utilizing mocking frameworks, such as Mockito, helps isolate the code being tested from its dependencies, creating more focused and reliable tests. Lastly, automating the execution of unit tests using build tools like Maven or Gradle ensures that the tests are run regularly and consistently throughout the development process. By adhering to these best practices, developers can create robust and maintainable unit tests for their Java code.
Automated testing offers several benefits in workload management. By automating the testing process, organizations can save time and resources by reducing the need for manual testing, which allows for faster and more efficient testing of workloads. This helps identify and fix issues earlier in the development process. Automated testing also provides more accurate and consistent results, eliminating the potential for human error, leading to improved overall workload management and better quality assurance.
Effective time allocation for testing in a project schedule is crucial for ensuring the quality and reliability of the software being developed. It is important to allocate sufficient time for planning, designing, executing, and evaluating test cases. This includes time for preparing test environments, executing both manual and automated tests, analyzing test results, and addressing any issues that arise during testing. Proper time allocation for testing helps to identify defects early in the development cycle, reducing the cost and effort required for fixing them later. It also allows for sufficient time to retest and validate fixes before the final release. Adequate testing time should be considered as an integral part of the overall project schedule to ensure the successful delivery of a high-quality software product.
To improve efficiency in Java unit testing, there are several best practices and techniques that can be implemented. These include writing focused and concise test cases that cover specific functionality, using mock objects to simulate dependencies and isolate the unit being tested, employing test data setup and teardown methods to ensure a clean and consistent test environment, using test suites to group and run related test cases together, implementing parallel test execution to leverage multi-core processors and reduce overall test execution time, applying code coverage analysis to identify areas of code that are not adequately tested, and using tools like JUnit and Mockito to simplify test case creation and verification. By following these practices, developers can improve the efficiency and effectiveness of their Java unit testing
Conclusion
In conclusion, unit testing is a crucial aspect of Java development that ensures the accuracy and reliability of individual code segments. By creating specific test cases for each function or method, developers can isolate program segments and verify their correct operation. Unit testing frameworks like JUnit and Mockito play a pivotal role in Java unit testing, providing tools for creating mock objects, simulating behavior, and verifying system behavior during testing. The use of these frameworks, along with best practices such as writing independent tests, testing boundary conditions, using meaningful test names, and keeping tests concise, can enhance the quality and stability of Java code through comprehensive and efficient unit testing.
The importance of unit testing goes beyond just bug detection and prevention. It promotes component isolation, modular design, and continuous integration and deployment. Unit tests serve as a living document of code behavior and facilitate collaboration among developers, testers, and stakeholders. By incorporating unit testing into the development process and leveraging automated unit testing tools integrated with build tools like Maven and Gradle, developers can streamline development, enhance collaboration, foster a culture of continuous improvement, ensure the correctness and robustness of the codebase, promote bug detection and maintainability, and contribute to overall code quality and stability.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.