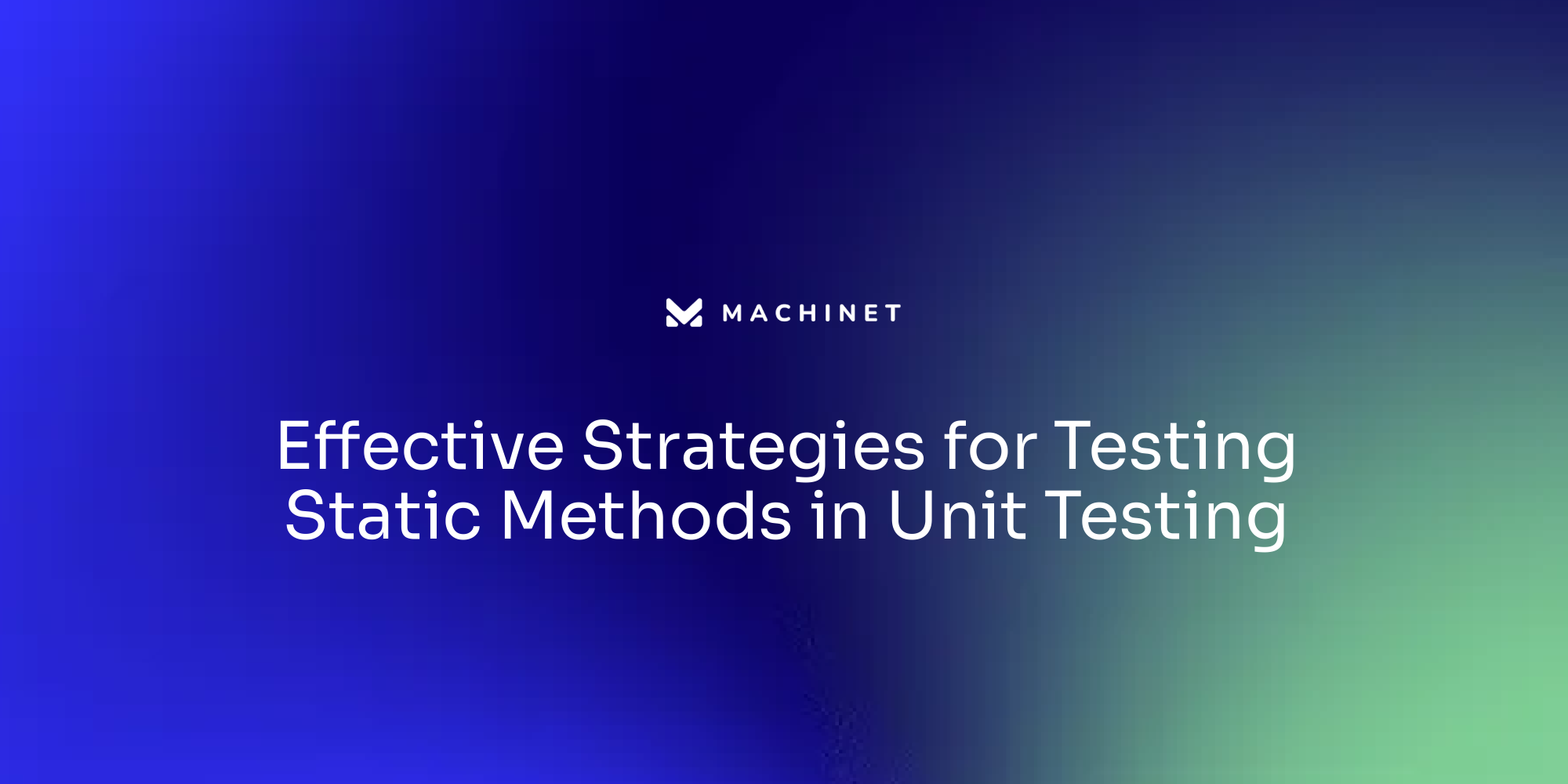
Table of Contents
- Understanding the Challenges of Testing Static Methods in Unit Testing
- Best Practices for Unit Testing Methods that Call a Static Method
- Implementing Robust and Flexible Testing Frameworks for Static Methods
- Strategies to Refactor and Improve Existing Test Suites with Static Methods
- Workload Management and Deadline Balancing in Unit Testing with Static Methods
- Case Study: Overcoming Challenges with Java's Static Methods in Unit Testing
- Future Trends: Evolving Project Needs and Adaptability of Unit Testing for Static Methods
Introduction
Unit testing static methods in Java can present unique challenges that require careful consideration and strategic approaches. These challenges include difficulties in mocking or stubbing static methods, managing state throughout the application lifecycle, and dealing with interactions with external systems. However, by employing the right tools and techniques, these challenges can be overcome effectively.
In this article, we will explore various strategies and best practices for testing static methods in Java. We will discuss the use of mocking frameworks like Mockito and PowerMock to mock dependencies and control the behavior of static methods during testing. Additionally, we will examine the benefits of refactoring code to make static methods more testable and explore techniques for testing static methods that interact with external systems.
By understanding and implementing these strategies, developers can improve the quality and reliability of their code through effective unit testing of static methods in Java. Let's dive into the details and explore the world of unit testing static methods
1. Understanding the Challenges of Testing Static Methods in Unit Testing
Static methods in Java inherently carry unique complexities when it comes to unit testing. The fact that they are tied to the class rather than an object makes the task of mocking or stubbing them during testing a challenging one.
Furthermore, they tend to maintain state throughout the lifecycle of an application, which can lead to unforeseen side effects that complicate the testing process. This is especially noticeable when these methods interact with external systems such as databases or file systems, causing tests to rely on the state of these systems, which in turn reduces their dependability.
Overcoming these challenges requires a strategic approach and the use of appropriate tools. One such approach is to use a mocking framework like Mockito to mock dependencies of the static method. By gaining control over the behavior of these dependencies, it becomes possible to test the static method in isolation.
In some cases, it may be beneficial to refactor the code to make the static method more testable. This could involve extracting the logic within the static method and placing it in a separate non-static method. This non-static method can then be tested using traditional unit testing techniques.
In situations where static methods interact with external systems, testing can prove to be more complex and may require additional setup and configuration. Here, it's advisable to use a library like PowerMock that allows for mocking of static methods. PowerMock enables you to mock the behavior of external dependencies used by the static method and verify interactions with those dependencies.
Moreover, tools like PowerMock offer additional capabilities for testing static methods, including the ability to mock static method calls. This makes it a valuable tool for unit testing static methods in Java, ensuring the quality of the code.
However, the challenges of testing static methods extend beyond just Java. For instance, testing API-specific code on Android can be particularly difficult and is often dismissed as "too hard to test." The Kotlin compiler, designed for building optimized Android apps, can further complicate unit testing. Yet, with version checks and runtime reflection, it is possible to overcome these challenges. A tool like Dagger can play a significant role in simplifying the testing of project code and handling static properties, making testing API or build version-specific code less daunting.
In conclusion, although static methods in Java present unique challenges in unit testing, these can be effectively addressed with the right tools and techniques. By leveraging mocking frameworks and refactoring code where necessary, it is possible to ensure the quality of your code through effective testing
2. Best Practices for Unit Testing Methods that Call a Static Method
Unit testing methods involving static method calls demand a strategic approach. The primary objective is to isolate the method under test as much as possible. This can be achieved through the application of best practices and the use of frameworks like Mockito for creating mock objects for dependencies, thereby simulating the behavior of static method calls. This enables a more controlled and isolated testing environment, focusing on testing the specific methods under test.
Instead of relying on a static DateTime
class, which may lead to the need for manual datetime resetting and potential thread safety issues, it is advisable to use a static DateTime
class with precautions in place to mitigate potential errors and ensure thread safety. A technique that can be used to override the datetime is to call a method that returns an IDisposable
which restricts the scope of changes to the underlying datetime. An example of this preferred approach could be using DateTime2020-12-25
.
Frameworks like PowerMock, an extension of Mockito and EasyMock, can be used to mock static methods. PowerMock provides the ability to mock static methods, final classes, and other difficult-to-test scenarios. This allows for more comprehensive and reliable testing of your code. However, caution is advised as over-reliance can lead to brittle tests. The focus of the test should not be the static method itself but rather the behavior of the method under examination. This approach ensures that the test remains a true 'unit' test, examining only a single piece of functionality.
For instance, the LocatorServiceTest
class showcases how PowerMock can be used to verify that a static method was called, by intentionally invoking it twice with different distances. The verification process is twofold - it confirms that the static method was called a specific number of times and validates the exact parameters used.
To avoid brittle tests when mocking static methods in unit tests, it is recommended to use a mocking framework like Mockito. Mockito provides a way to mock static methods by using the PowerMock extension. PowerMock allows you to mock static methods and verify their behavior in unit tests. However, it is generally recommended to avoid relying heavily on static methods in your codebase. Instead, consider refactoring your code to use dependency injection and instance methods, which are easier to mock and test.
In summary, the key to unit testing methods with static method calls is to decouple the methods being tested from the static methods, so that their behavior can be easily controlled during unit testing. This can be achieved through the use of mocking frameworks, abstraction layers, and dependency injection. By focusing on the behavior of the code rather than the implementation details of static methods, unit tests can be more resilient to changes in the codebase
3. Implementing Robust and Flexible Testing Frameworks for Static Methods
Unit testing static methods might seem like a daunting task in the world of software development. However, it can be made manageable and efficient with careful planning and the right tools. One of the first steps towards this goal is to choose a testing framework capable of creating mocks or stubs for static methods. A combination of JUnit and PowerMock is a popular choice for this purpose.
To effectively use JUnit and PowerMock for testing static methods in your Java projects, you'll need to follow a few important steps. Firstly, you'll need to ensure your project has the necessary dependencies, including JUnit, PowerMock, and the PowerMock JUnit Runner. Your test class should be annotated with @RunWith(PowerMockRunner.class)
, instructing JUnit to use the PowerMock runner. The test method should be annotated with the @PrepareForTest
annotation, specifying the classes that contain the static methods you're testing. Then, the PowerMockito.mockStatic()
method can be used to mock the static class, allowing you to control the behavior of the static methods during testing. The behavior of the static methods can be defined using the PowerMockito.when()
method, allowing you to specify return values, throw exceptions, or perform other actions. Finally, you can invoke the static methods to be tested and use JUnit assertions to verify their expected behavior.
Designing tests to be as independent as possible is another crucial element. This means striving to avoid dependencies on the state of external systems or the sequence in which tests are executed. This can be achieved by avoiding the use of global state and designing static methods to be self-contained, relying only on their input parameters. Parameterized tests can be used to test different inputs and expected outputs, ensuring coverage of a wide range of scenarios and edge cases. Mocking frameworks can be used to create fake implementations of dependencies, allowing the control of their behavior during testing. It's also crucial to test all possible code paths and keep tests focused and independent.
Adaptability is a key characteristic of a robust testing framework. It is essential to write tests with a focus on behavior rather than implementation, which allows the tests to remain valid even when the codebase goes through changes. When the codebase changes, you should consider refactoring tests, updating them to reflect the changes made to the codebase. This includes modifying the inputs and expected outputs to align with the new functionality of the static methods. It's also important to verify if any other tests or dependencies are affected by the codebase changes to ensure the refactored tests do not introduce any unintended side effects or break other parts of the codebase.
A real-world example of these principles in action is the "Code Health: Now You're Thinking with Functions" blog post. The author emphasizes code health and the use of functions in programming, illustrating how to maintain code health by focusing on the behavior of functions in unit tests rather than their implementation.
In the embedded space, unit testing is often overlooked, with integration and hardware-in-the-loop testing typically taking precedence. However, unit testing is an essential component of software testing in this domain as well. For instance, the Notecard library provides functions for communicating with the Notecard, a small system-on-module that combines prepaid cellular connectivity and low-power design for secure off-internet communications. Unit tests can be written to verify the correctness of these functions, demonstrating the importance and applicability of unit testing in the embedded space.
In summary, constructing a robust and flexible testing framework for static methods involves selecting the appropriate testing framework, designing independent tests, and ensuring the adaptability of the tests to changes in the codebase. The importance of these principles is demonstrated in various real-world applications, including the programming practices advocated in the "Code Health: Now You're Thinking with Functions" blog post and the unit testing practices in the embedded space, such as those used in the Notecard library
4. Strategies to Refactor and Improve Existing Test Suites with Static Methods
Refining test suites that encapsulate static methods is a complex process, but there are several techniques to streamline it. An efficient approach is to gradually replace static methods with instance methods or interfaces, which are generally more straightforward to test. This approach can be incorporated into regular development activities or as a specific refactoring initiative.
Enhancing the design of your codebase to optimize its testability is another effective technique. This could involve integrating dependency injection to decouple the code, or restructuring classes to adhere to the Single Responsibility Principle. These modifications can simplify the codebase, reducing the complexity of testing.
Incorporating regular reviews and updates of tests is also essential to ensure their ongoing effectiveness as the codebase evolves. Detailed tests serve as documentation, and including calculations in tests helps avoid hard-coded test data smell. This also simplifies the process of identifying the issue when a test fails.
Refactoring the code to extract calculations from loops can simplify tests, while introducing mockable abstractions reduces the number of paths through the code, further streamlining the tests. Testing conditional branches and values in isolation facilitates the testing of specific branches and calculations.
Employing the blackboard pattern can break dependencies within complex calculations, making tests more concise and understandable. This amalgamation of refactoring strategies and testing techniques can enhance both the readability and maintainability of the tests, leading to more effective and efficient unit testing processes.
To improve the maintainability and readability of your code, you can refactor test suites with static methods. Identify the static methods used in your test suite, which may include utility methods or helper methods used across multiple test cases. Extract these static methods into separate classes or utility classes to make them more modular and reusable. If the static methods are specific to a certain test suite, create a separate class for each test suite and move the static methods into that class. Replace the calls to the static methods in your test cases with the new class methods to make your test cases more readable and easier to understand. Repeat this process for all the static methods in your test suite.
To test code with interfaces instead of static methods, use dependency injection. Define an interface that represents the functionality of the static method, create a class that implements that interface, and inject an instance of the class that implements the interface into your code instead of directly calling the static method. This allows you to easily mock or stub the behavior of the interface during testing, making it simpler to write unit tests for your code.
To improve the testability of your codebase, design code that follows the principles of modularity and encapsulation. Break down the code into smaller, independent modules that can be tested in isolation. Minimize dependencies between modules, making it easier to write unit tests for each module. Use dependency injection for easier mocking and stubbing of dependencies during testing. Write code with clear separation of concerns to improve testability. Adopt a test-driven development (TDD) approach to improve the testability of your codebase.
To maintain effective tests as your codebase evolves, follow certain best practices. Regularly review and update tests as the codebase evolves. Use version control systems, such as Git, to manage changes to the codebase. Follow the principles of test-driven development (TDD) to ensure that tests are always up to date. Automate tests using frameworks like JUnit or Selenium to ensure that tests are executed consistently and efficiently. Implement continuous integration and continuous delivery (CI/CD) pipelines to automate the process of building, testing, and deploying code changes
5. Workload Management and Deadline Balancing in Unit Testing with Static Methods
The process of unit testing, particularly in relation to static methods, often grapples with the complexity of managing workloads and adhering to deadlines. One effective way to mitigate this complexity is by employing risk-based prioritization of tests. This approach focuses on testing areas of the codebase that carry a high risk or are subject to frequent modifications first. However, to make this strategy more effective, several techniques can be implemented.
Identifying the critical functionalities or features of the system and prioritizing their testing is crucial. This early detection ensures that potential issues or bugs in these critical areas are identified early on. Another strategy lies in prioritizing tests based on the likelihood and impact of a failure. Tests covering high-risk areas or functionalities critical to the overall system performance should be given higher priority. Additionally, considering the dependencies between different components or modules can be helpful in determining the order of testing. Tests for components with a higher number of dependencies should be prioritized to ensure that issues in these components do not affect the functionality of other components.
In terms of balancing deadlines, it is essential to prioritize and plan effectively. One approach is to start by identifying the critical functionalities or components that need to be tested first. This strategy ensures that the most important parts of the system are thoroughly tested before the deadline. Additionally, breaking down the testing process into smaller, manageable tasks can be helpful. By setting achievable goals and milestones, it becomes easier to track progress and ensure that testing is completed within the given timeframe.
Integrating testing into the development process, rather than deferring it to the end, can also aid in deadline management. This integration ensures that testing gets its due consideration in project timelines, thereby mitigating the risk of overshooting deadlines. Adopting a continuous integration and continuous testing approach allows developers to regularly run automated tests on their code changes, catching potential issues before they are merged into the main codebase. This not only reduces the testing workload but also improves the overall quality of the software being developed.
The adoption of automated testing tools can further streamline workload management and deadline balancing by curtailing the time and effort invested in executing tests. Automated testing tools can improve the efficiency and effectiveness of the testing process by automating repetitive tasks, saving time and effort. These tools also provide consistent and reliable test results, eliminating human errors and ensuring the same tests are executed in the same way every time.
However, testing time-dependent classes introduces a new set of challenges as traditional methods often lead to slow and flaky tests. The nodatime and nodatimetesting packages can be instrumental in addressing these issues. The iclock interface is employed to inject the concept of time as a dependency. The fakeclock class from nodatimetesting can modify the current time for testing, supporting the advancement of the clock by a specific duration. Nodatime can enhance test consistency by specifying the duration between two commands, making it a valuable tool for datetime-related projects.
Finally, maintaining a sustainable pace in agile software development is of paramount importance. Teams often face challenges in sustaining this pace due to the pressure from company leaders to meet unrealistic deadlines. Overworking and compromising on good practices can have detrimental consequences. It's crucial for teams to resist unrealistic demands, prioritize their well-being, and adopt strategies to achieve a predictable and consistent cadence. Nurturing a learning culture and investing time in continuous learning can be beneficial. Small experiments and incremental steps can help achieve a more sustainable approach. Failure to maintain a sustainable pace can lead to negative outcomes, including burnout and turnover
6. Case Study: Overcoming Challenges with Java's Static Methods in Unit Testing
As a development team embarks on the journey of unit testing Java's static methods within a vast codebase, complexities and challenges are bound to arise. However, with the right strategies, these challenges can be effectively managed. Initially, the team faced a convoluted sync logic spread across multiple services and numerous static methods. To tackle this, the team took on a project to refactor the legacy code, centering around the mechanism of data synchronization between the application and server.
The team employed a finite state machine (FSM) approach, encapsulating the sync algorithm and its interdependencies within a class dubbed as SyncController. This strategy improved the quality and stability of the codebase, making it more comprehensible and maintainable. It also provided a clearer understanding of the application's behavior, as the states and transitions were explicitly declared.
To validate the FSM implementation's correct behavior, the team covered the SyncController class with 71 unit tests. These tests played a critical role in preventing regression bugs, thereby ensuring the stability of the codebase. Rather than using traditional mocking frameworks for unit testing, the team opted for manual test doubles that allowed for superior control and precision in the tests.
When the team was confronted with long build times due to the extensive codebase, they turned to modularization. By segregating the SyncController and its associated tests into a separate module, they managed to improve the build times and streamline the testing process. The application of interfaces permitted the SyncController to interact with the code in the main module sans direct dependencies, adhering to the Dependency Inversion Principle.
In addition, the team also embraced other SOLID principles in their implementation, such as the Single Responsibility Principle and the Interface Segregation Principle. These principles guided the team in developing a codebase that was more maintainable and scalable.
Despite the project's complexity and the extensive amount of affected code, the team was able to complete it on time, demonstrating the efficacy of the "clean" approach in software engineering. They also significantly enhanced their test coverage and reliability by effectively managing their workload and meeting project deadlines, vital in the ever-changing realm of software development. This journey serves as a testament to the power of best practices in software engineering, not only in delivering new features but also in enhancing code quality.
In the face of such challenges, the team turned to PowerMock, a popular testing framework that allows static methods in Java to be mocked. PowerMock provides a set of APIs that facilitate the mocking of static methods, final classes, constructors, and more. This approach enabled the team to effectively test static methods in Java and ensure the reliability and correctness of the code.
Another technique employed was refactoring the static methods into non-static methods within a class and then testing the non-static methods using traditional unit testing techniques. This was achieved by creating an instance of the class and invoking the non-static methods on that instance.
By integrating unit testing into the development process, the team ensured the quality and reliability of software applications. Regular execution of unit tests as part of the development process allowed the team to catch issues and regressions early, enabling timely resolutions and reducing the risk of new bugs.
The team also implemented dependency injection for unit testing, identifying the dependencies that needed to be injected and modifying the code to accept these dependencies as constructor parameters or setter methods, rather than creating the dependencies internally. This practice improved the modularity and testability of the code, as well as facilitated the substitution of dependencies during unit testing.
By using PowerMock, the team was able to increase their test coverage by mocking difficult-to-test aspects, enabling them to write more comprehensive unit tests. Furthermore, PowerMock improved the reliability of the tests by allowing the team to simulate different scenarios and edge cases that are challenging to reproduce in real-world situations.
In conclusion, the team's journey stands as an example of how best practices in software engineering can overcome challenges, deliver new features, and enhance code quality
7. Future Trends: Evolving Project Needs and Adaptability of Unit Testing for Static Methods
Unit testing in the dynamic landscape of software development is a subject of continual transformation. With the advent of microservices and containerization, codebases are becoming more compartmentalized, easing unit testing to some extent, but also introducing new challenges such as boundary testing of services.
For Java developers, changes in Java 11's default date and currency formats necessitate code adjustments to align with these changes. Unit tests act as a safeguard here, ensuring that the code operates as intended and detecting bugs early on. For instance, a function parsing date strings may need to accommodate the change in Java 11's default date format pattern, and a function formatting numbers as currency would have to adjust to the change in the non-breaking space character for currency formatting. Unit tests validate these changes and ensure the expected behaviour of these functions.
In response to these evolving trends and challenges, testing frameworks and tools are continually being refined and updated. For example, Typemock, a unit testing vendor, has developed tools for .NET and C#, which facilitate code mocking without the need for code modification. They leverage machine learning and fuzzy logic to generate unit tests for code with no existing tests, thereby helping to tackle the challenges of testing legacy code. Their latest release, Isolator version 9.1, supports both .NET Core and .NET Framework, and integrates with Microsoft's Visual Studio 2022, providing detailed insights into code coverage.
To navigate the complexities of unit testing in microservices and containerization, best practices recommend testing each microservice in isolation. Each microservice should be accompanied by its own set of unit tests focusing on its specific functionality and behaviour. Mocking and stubbing techniques are used to isolate dependencies and simulate certain behaviours during testing, allowing for more controlled and predictable testing environments. For containerization, it is beneficial to create lightweight, disposable test containers for quick spin-up and tear-down.
Testing across service boundaries presents challenges such as handling different communication protocols and message formats between services and dependency on external services. To overcome these, clear communication channels and collaboration between teams is essential. Regular meetings, documentation, and shared test environments are helpful, as are tools and frameworks that support testing across service boundaries such as service virtualization or contract testing tools. Automated testing practices can greatly facilitate testing across service boundaries, enabling faster feedback and identification of issues.
Communities like the Dev Community, where developers share experiences and stay updated, also enrich the unit testing domain. For example, discussions about the use of flags as a parameter anti-pattern in Java, or the sharing of templates to quickly answer FAQs and store reusable code snippets, can provide invaluable insights for those navigating unit testing challenges.
Developers can improve unit testing for evolving software development practices by writing comprehensive test cases covering different scenarios, using mocking frameworks to isolate dependencies, practicing test-driven development, and regularly reviewing and refactoring test code. Staying updated with the latest trends and advancements in unit testing can contribute to continuous improvement in this area.
In conclusion, as software engineers, staying abreast of these trends and tools is paramount to being prepared for the unit testing challenges of the future. Whether it's adapting to changes in the JDK, dealing with the complexities of testing across service boundaries, or leveraging new tools and frameworks, staying informed and adaptable is key to mastering unit testing static methods in an ever-evolving landscape
Conclusion
The main points discussed in this article include the challenges faced when unit testing static methods in Java and the strategies and best practices for overcoming these challenges. The challenges highlighted include difficulties in mocking or stubbing static methods, managing state throughout the application lifecycle, and dealing with interactions with external systems. However, by employing the right tools and techniques, such as mocking frameworks like Mockito and PowerMock, refactoring code to make static methods more testable, and using dependency injection, these challenges can be effectively addressed.
The broader significance of these ideas is that by implementing effective unit testing strategies for static methods, developers can improve the quality and reliability of their code. Unit testing helps catch bugs early on, ensures that code behaves as intended, and allows for easier maintenance and refactoring. By addressing the challenges specific to testing static methods, developers can have greater confidence in the functionality and performance of their code.
To boost your productivity with Machinet. Experience the power of AI-assisted coding and automated unit test generation.](https://machinet.net/)
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.