
Table of Contents
- Setting the Stage: Understanding Selenium and Its Importance in Unit Testing
- Getting Started with Selenium: Installation and Setup
- Diving Deeper: Exploring Selenium's Features and Capabilities
- Hands-On Tutorial: Validating Text in PDF Files using Selenium Automation
- Advanced Techniques: Reading Content from PDF File using Apache PDFBox and Integrating it with Selenium
- Best Practices for Verifying PDF File Content with Selenium
- Practical Tips to Assert PDF Text and Validate Contents of Downloaded PDFs
- Balancing Workload and Deadlines: Strategies for Optimizing Your Selenium Testing Efforts
Introduction
Selenium, a powerful open-source tool, plays a crucial role in software testing, particularly in unit testing. It provides a robust platform to authenticate the functionality of different components within a software application. With its automation capabilities, Selenium simplifies software testing and makes it more reliable by simulating user interactions with a web application. By automating tasks like button clicks, form submissions, and functionality checks, Selenium ensures that every website element operates as expected.
In this article, we will explore the importance of Selenium in unit testing and its automation capabilities. We will discuss the process of automating web browsers using the Selenium WebDriver library and how it can be integrated with different programming languages. Additionally, we will delve into practical tips and best practices for validating text in PDF files using Selenium automation and Apache PDFBox. By understanding these concepts, developers and testers can enhance their testing efforts and ensure the accuracy and reliability of their software applications
1. Setting the Stage: Understanding Selenium and Its Importance in Unit Testing
Selenium, a stalwart in the open-source software landscape, is pivotal in software testing, particularly in unit testing. It provides a robust platform to authenticate the functionality of different components within a software application. With its adeptness in simulating user interactions with a web application, Selenium has become a crucial tool in unit testing, ensuring a thorough analysis of a software's response to varied inputs and situations.
The unique selling point of Selenium lies in its automation capabilities, simplifying software testing and making it more reliable. Automating tasks like button clicks, form submissions, and functionality checks, Selenium ensures that every website element operates as expected.
Selenium functions by initiating a web browser, navigating to a website, clicking and typing, and verifying the presence of certain elements. This process not only conserves time and effort but also augments the reliability of tests by allowing for repeatability. Selenium conducts tests quicker than humans, maintains consistency, tests multiple website components concurrently, and integrates effortlessly with other software tools.
To automate web browsers using Selenium, the Selenium WebDriver library is a powerful tool. It provides a way to interact with web browsers programmatically, automating various tasks such as navigating to URLs, filling out forms, clicking buttons, and extracting data from web pages.

It supports multiple programming languages, including Java, Python, C#, and JavaScript. By using the appropriate WebDriver bindings for your chosen programming language, you can write scripts to automate web browsers and perform tasks efficiently.
To simulate user interactions with Selenium, the WebDriver API provided by Selenium is used. This API automates various user actions, such as clicking on elements, typing into input fields, and navigating through web pages.
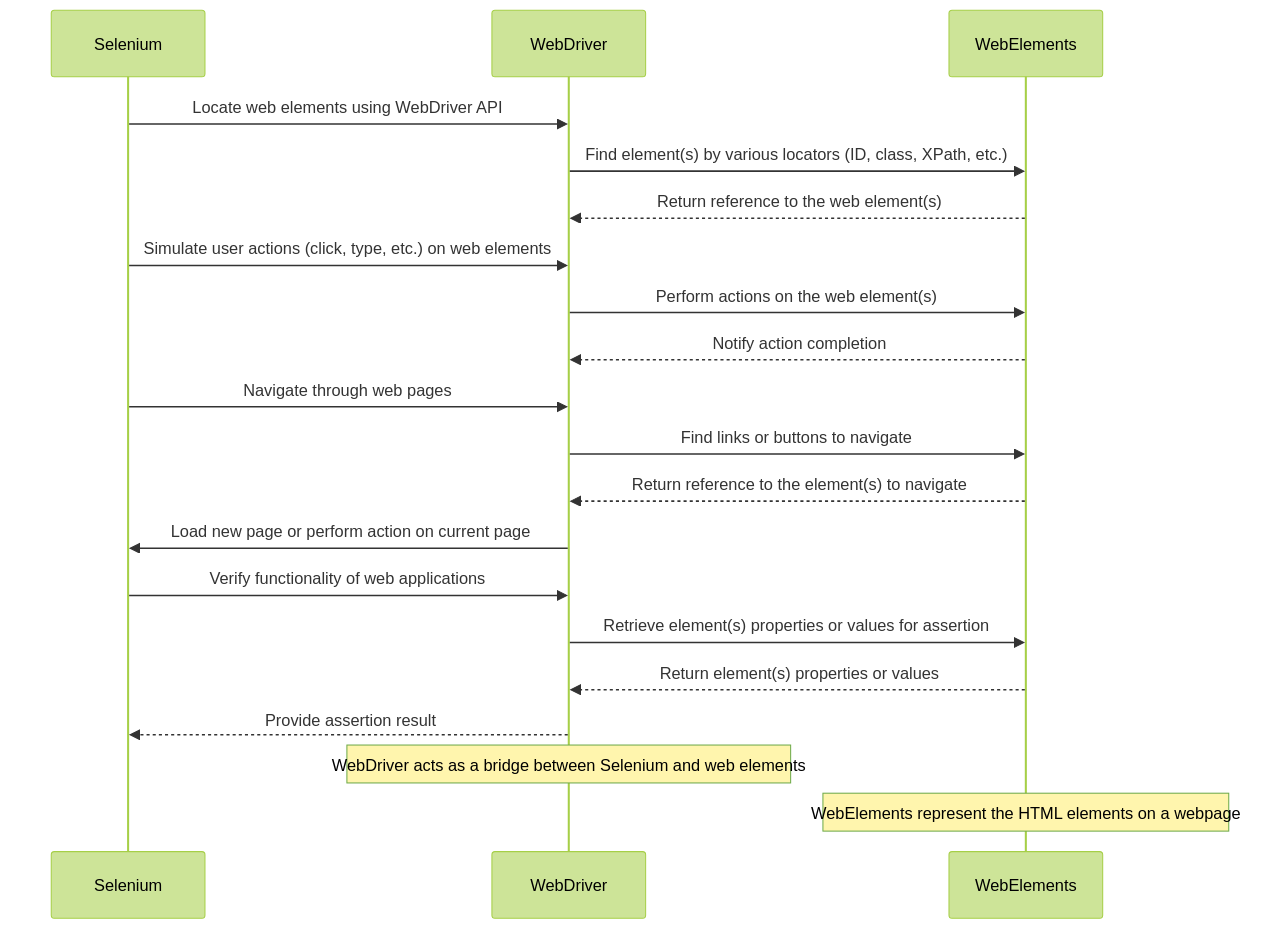
For example, to simulate a click on an element, the click()
method provided by the WebDriver API is used. This method simulates a mouse click on the specified element. To type into an input field, the send_keys()
method provided by the WebDriver API is used. This method sends text to the specified input field. To navigate through web pages, methods such as get()
to open a specific URL, back()
to navigate back to the previous page, and forward()
to navigate forward to the next page are used. By using these methods and combining them in your test scripts, you can simulate various user interactions with Selenium, automating the testing of user interfaces and ensuring that your web application behaves correctly under different scenarios.
To integrate Selenium with Java for unit testing, you can follow certain best practices. One of the common practices is to use the JUnit framework, which provides annotations and assertions specifically designed for Java unit testing.
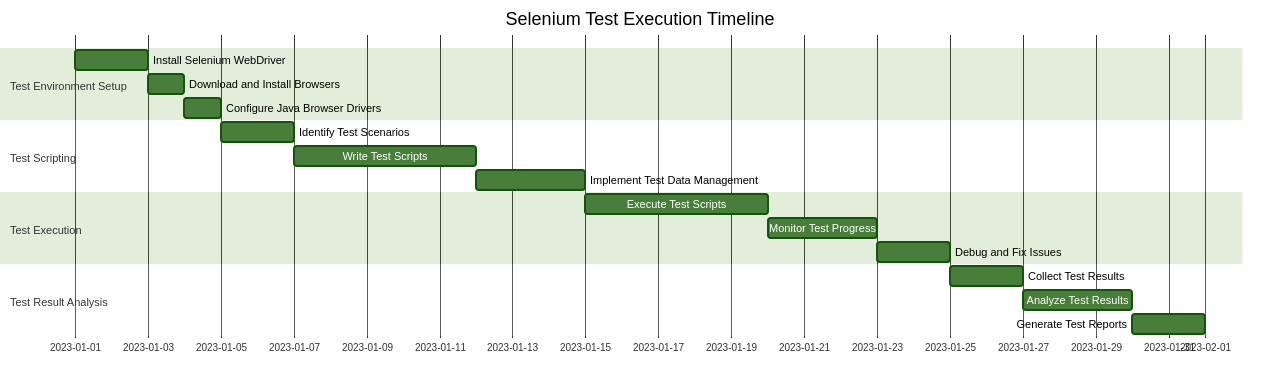
By leveraging these features, you can write effective and efficient unit tests for your Selenium-based Java applications. JUnit provides annotations such as @Before
, @After
, @BeforeClass
, and @AfterClass
that allow you to set up preconditions and clean up resources before and after each test case. These annotations help in managing the test environment and ensuring the tests are executed in a consistent state.
In summary, Selenium is instrumental in software testing, detecting issues early and ensuring that websites and applications function correctly. It acts as a computer aide, dutifully following instructions to test websites and applications. For those seeking to guarantee the functionality of their website or application, Selenium is a highly recommended tool. It is compatible with a broad spectrum of programming languages, including Java, making it a versatile choice for both developers and testers
2. Getting Started with Selenium: Installation and Setup
Starting your journey into automated unit testing with Selenium involves a straightforward installation and setup process. Selenium is a powerful tool for automating browsers and requires fundamental prerequisites like Node.js, npm, and a good understanding of JavaScript. An Integrated Development Environment (IDE), such as Visual Studio Code, is also necessary.
To start your Selenium journey, you need to initialize an npm project in your project directory using the command npm init
. Following this, it's crucial to install necessary npm packages like selenium-webdriver
and chromedriver
. While Chrome is the chosen browser for automation in this context, Selenium supports all major browsers. Therefore, you can opt for any browser by downloading the corresponding driver.
Once the initial setup is done, create a test directory and a test.js
file within it. This file is where you'll write your first Selenium test and should import the necessary packages and classes from selenium-webdriver.
The primary interface to the browser in Selenium is the WebDriver instance. You can instantiate a new web driver and open a Chrome browser using the builder class. The driver.get()
method allows you to navigate to a specific website, like Google. With the driver.findElement()
method, you can locate a Document Object Model (DOM) element on the page, and the sendKeys()
method allows you to input a search term.
Simulating the Enter key press is possible with the key.return
method. After inputting the search term, the script waits for the page to load and then closes the browser using the driver.quit()
method. The final test script should incorporate error handling and wait for the page to load before terminating the browser.
As you progress, remember that Selenium is more than just a tool. It's a comprehensive framework that ties together different browser backends, thus enabling cross-browser and cross-platform automation. It offers Selenium IDE, a low-code tool for recording and playback, and Selenium Grid for scaling up tests.
For further exploration and understanding, you can refer to the Selenium official website and the npm packages used in this setup. With Selenium, you're equipped to automate repetitive web-based tasks, create quick bug reproduction scripts, and build robust browser-based regression automation suites
3. Diving Deeper: Exploring Selenium's Features and Capabilities
Selenium WebDriver, an open-source tool revered for its robustness and versatility, plays a crucial role in the development of solid web applications by automating web browsers. Its compatibility with various browsers such as Chrome, Firefox, and Safari is a testament to its comprehensive testing capabilities across different platforms.
The WebDriver's flexibility is evident in its support for multiple programming languages, including Java, Python, and C, empowering developers to code in their preferred language. This flexibility, coupled with Selenium WebDriver's ability to interact with web elements on a page and create automated tests, enhances developers' productivity and efficiency.
The architecture of the Selenium WebDriver encompasses four major components: the Selenium client library, the JSON Wire Protocol over HTTP, browser drivers, and web browsers. The client library offers an interface for controlling web browsers, while the JSON Wire Protocol facilitates communication between the client library and the browser drivers. On the other hand, browser drivers serve as intermediaries, translating commands into actions understandable by the browsers.
Setting up the WebDriver demands the developers to download and install the appropriate Selenium library for their programming language, set up the relevant web driver for the browser of choice, and initialize the browser driver. This setup paves the way for creating test scripts that are converted into HTTP requests using the JSON Wire Protocol, with the browser executing actions based on these requests.
Selenium WebDriver's cross-platform compatibility, easier debugging with built-in tools, and automation support for tasks like data entry and form submission provide efficient testing, improved user experience, and cost savings compared to manual testing. However, it has its limitations like lack of support for non-browser applications, high maintenance costs, limited reporting capabilities, cross-browser compatibility issues, and difficulty debugging certain types of code.
To overcome these limitations and boost Selenium WebDriver automation, developers can harness the capabilities of Machinet. Although the exact details of a specific "Machinet AI plugin for unit testing" are not directly mentioned, Machinet's platform offers advanced debugging and monitoring features, real user experience simulation, browser and platform coverage, and integration with popular testing frameworks.
Explore Machinet's platform features and enhance your Selenium testing efforts.
This boosts the potential of Selenium WebDriver for web application testing.
Machinet's platform can also provide more in-depth articles on advanced unit testing techniques and best practices, including test-driven development, mocking and stubbing, code coverage analysis, and continuous integration and deployment for unit tests. Additionally, Machinet might offer tutorials and examples using different programming languages and frameworks to cater to a wider audience. Machinet's interactive coding exercises or quizzes can help readers practice and solidify their understanding of unit testing concepts.
Moreover, Machinet's built-in functions and libraries enable precise interaction with web elements, such as clicking buttons, filling out forms, and extracting data. Its features also include handling alerts and pop-ups, and executing JavaScript on the website. The blog posts on the Machinet website provide insights into unit testing basics, tips, techniques, and best practices for Java unit testing, serving as a resourceful guide to effectively utilizing Machinet for unit testing purposes
4. Hands-On Tutorial: Validating Text in PDF Files using Selenium Automation
The practice of automating the validation of text within PDF files during unit testing can be significantly optimized with the application of advanced tools such as Applitools' ImageTester. As a Java command-line utility, ImageTester is purpose-built to perform visual assertions on PDF files, ensuring their accuracy.
To get started with ImageTester, you'll need to download it from the Applitools website and have Java installed on your machine. The utility accepts a range of parameters, including the Applitools API key, the application under test, and the match level for comparison. Its functionality is rooted in comparing the PDF file against a captured baseline and reporting any mismatches.
Consider using Selenium WebDriver to enter data into a form and download a PDF invoice. After downloading, the PDF can be moved to a test directory and verified using ImageTester. This verification process involves executing a command using the Java Runtime.exec() method and waiting for the process to complete. You can then check the output of the command for the word "mismatch" to determine the verification status.
Incorporating ImageTester into automated tests by executing it from your code can be highly effective. For instance, a test flow might involve opening the application, entering the relevant information, generating a PDF file, and then performing visual assertions using ImageTester. The results can be validated by checking if the output from ImageTester contains the word "mismatch".
ImageTester also offers additional features that can enhance your PDF validation process. The ability to add ignore regions to PDFs is one such feature. This allows you to exclude specific areas from verification, which can be particularly useful when certain parts of the PDF are not relevant to your test.
While Selenium provides the foundation for navigating to and extracting text from PDF files, tools like ImageTester can further enhance and streamline your PDF validation process. The integration of these tools into your automated tests can lead to more reliable and efficient unit testing efforts.
As part of your toolkit, Selenium WebDriver can be used to navigate to a PDF file, and with the use of the get()
method, you can navigate to the URL of the PDF file. Since PDF files cannot be directly interacted with by WebDriver, you will need to download the file to your local machine. The built-in download functionality of the browser or a library like Apache PDFBox or PDF.js can be used for this purpose. After downloading the PDF file, you can use other libraries or tools to interact with the PDF file as needed.
There are several libraries available for parsing PDF files and extracting text from them. Libraries like Apache PDFBox, iText, and PDFMiner are popular choices for extracting text from PDF files programmatically, making it easier to work with PDF documents in your application.
To extract text from PDF files using PDFBox with Selenium, you can follow a similar procedure. First, you need to install PDFBox and set up Selenium WebDriver to automate the web browser. Then you can use Selenium WebDriver to navigate to the webpage containing the PDF file and download the PDF file. After downloading the PDF file, you can use PDFBox to open the downloaded PDF file and extract the text from it. You can further process the extracted text as per your requirements, such as saving it to a file or performing text analysis.
To validate text in PDF files using Selenium and Java, you can use Apache PDFBox. This open-source Java library allows you to work with PDF documents. Once the PDF file is downloaded, you can use Apache PDFBox to extract the text from the PDF file and perform text validation.
If you are trying to compare extracted text from PDF files with expected values in Selenium, you can use the PDFBox library in Java. This library provides functionality for extracting text from PDF files, which you can then compare with your expected values using Selenium. This way, you can verify that the extracted text matches the expected values, ensuring the accuracy of your tests.
When it comes to validating text in PDF files during unit tests, there are several best practices that can be followed. One approach is to use a PDF parsing library or tool that allows you to extract the text from the PDF file. Once you have extracted the text, you can compare it with the expected text using assertions or other validation techniques provided by your unit testing framework. Another best practice is to have a set of sample PDF files with known text content that can be used as test inputs to verify the correctness of the text extraction process. Additionally, it is important to consider edge cases such as handling different fonts, styles, and formatting in the PDF file. By following these best practices, you can ensure that the text in PDF files is accurately validated during unit tests.
To perform text validation in PDF files using Selenium and PDFBox, you can utilize the capabilities of both tools. Selenium is a popular web automation framework that can be used to interact with web pages, while PDFBox is a Java library for working with PDF documents.
One approach to validate text in PDF files is to first use Selenium to navigate to the web page that contains the PDF file. Then, you can use Selenium to download the PDF file to a local directory. Once the PDF file is downloaded, you can use PDFBox to extract the text from the PDF file and perform the necessary validation.
Here is a high-level overview of the steps involved:
- Use Selenium to navigate to the web page that contains the PDF file.
- Use Selenium to locate and click on the link or button that triggers the download of the PDF file.
- Use Selenium to wait for the download to complete.
- Use PDFBox to load the downloaded PDF file.
- Use PDFBox to extract the text from the PDF file.
- Perform the necessary validation on the extracted text using your preferred validation approach (e.g., comparing against expected values, using regular expressions, etc.).
By combining the capabilities of Selenium and PDFBox, you can automate the process of downloading PDF files and validating the text within them. This can be particularly useful in scenarios where you need to perform automated testing or data extraction from PDF files
5. Advanced Techniques: Reading Content from PDF File using Apache PDFBox and Integrating it with Selenium
The Apache PDFBox library offers a robust open-source solution for handling PDF documents in Java. It allows developers to create, modify, and extract content from PDF files. The library, which is under the Apache License v2.0, is a volunteer-led project as part of the Apache Software Foundation. It encourages contributions from the community.
A key feature of Apache PDFBox is its capacity to extract text from existing PDF documents. This is accomplished using the PDTextStripper
class, which offers methods for obtaining the desired content. In particular, the getText
method is utilized to extract all text from the document, returning it as a string object.
To illustrate this, consider a Java program that loads a PDF document named "newpdf" from a specified path. The program creates an instance of the PDTextStripper
class and retrieves the text using the getText
method. The extracted text is then displayed as output. This program is compiled and executed from the command prompt, showcasing the power and simplicity of using Apache PDFBox for text extraction in Java.
```javaimport java.io.File;import java.io.IOException;
import org.apache.pdfbox.pdmodel.PDDocument;import org.apache.pdfbox.text.PDFTextStripper;
public class PdfTextExtractor { public static void main(String[] args) { try { // Load PDF document PDDocument document = PDDocument.load(new File("path/to/your/pdf/file.pdf"));
// Create an instance of PDFTextStripper class
PDFTextStripper pdfStripper = new PDFTextStripper();
// Extract text from PDF
String text = pdfStripper.getText(document);
// Print the extracted text
System.out.println(text);
// Close the document
document.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}```Remember to replace "path/to/your/pdf/file.pdf" with the actual path to your PDF file. This code will extract the text from the PDF and print it to the console.
Apache PDFBox can be integrated with Selenium WebDriver to streamline your unit tests. For instance, after downloading a PDF file using Selenium's WebDriver, you can utilize Apache PDFBox to open the file, extract its text, and validate the content as part of your assertions. This combination of tools delivers a powerful method to ensure the accuracy and integrity of PDF content in your software applications.
To integrate PDFBox with Selenium WebDriver, you can use the PDFBox library in your Selenium test scripts. PDFBox is an open-source Java library for working with PDF documents. By integrating PDFBox with Selenium WebDriver, you can automate tasks such as extracting text from PDF files, searching for specific content, and validating the content of PDF documents during your test automation process.
In your unit tests, you can use the PDFTextStripper class provided by the Apache PDFBox library to validate PDF content. This class enables you to extract text content from a PDF document, which you can then compare with the expected content in your unit test. By comparing the extracted text with the expected content, you can verify that the PDF document contains the correct information.
You can also use Apache PDFBox with Selenium by following these steps:
- First, add the Apache PDFBox library to your project. You can download the library from the Apache PDFBox website.
- Once you have downloaded the library, include the JAR file in your project's build path.
- Use the Apache PDFBox API to interact with PDF files in your Selenium tests. The API provides methods for extracting text, images, and other content from PDF files.
- Use the WebDriver provided by Selenium to navigate to the PDF file's URL or open a local file. You can then use the Apache PDFBox API to interact with the PDF file's content.
By following these steps, you can use Apache PDFBox with Selenium to perform various actions on PDF files, such as extracting text, validating content, or interacting with form fields. By leveraging the combined capabilities of Apache PDFBox and Selenium, you can enhance the efficiency and effectiveness of your unit testing efforts, leading to higher quality software products
6. Best Practices for Verifying PDF File Content with Selenium
When it comes to validating PDF file content using Selenium, there are several crucial steps and strategies to consider. Initially, it's vital to ensure that the PDF file has been downloaded successfully before any content extraction is attempted. This can be achieved by using Selenium's find_element method to locate the download link or button on the webpage, and the click method to initiate the download. You can then use Selenium's WebDriverWait to pause the process until the PDF file download is complete. This proactive verification helps in preventing unnecessary errors and guarantees that the correct file is being evaluated.
The subsequent step in the process involves the extraction of text data from the PDF file. For this, leveraging a robust PDF parser library, such as Apache PDFBox, iText, or PDFMiner, becomes essential. For instance, to extract text using Apache PDFBox, you need to add the PDFBox dependency to your project. You can download the JAR file from the Apache PDFBox website or use a dependency management tool like Maven or Gradle. Then, you can use the PDFBox API to load the PDF file and extract the text content. The PDFBox's PDDocument.load()
method can be used to load the PDF file, following which the PDFTextStripper
class can be used to extract the text.
Once the text has been extracted, comparing it with the expected results becomes the next primary task. When performing this comparison, it's advisable to adopt a string comparison approach that disregards differences in case and whitespace. These discrepancies can often result in tests failing without a valid reason. To achieve this, normalization techniques such as removing leading and trailing whitespaces or converting text to lowercase can be applied before performing the comparison. This helps in ensuring that the comparison is not affected by differences in letter casing, such as uppercase and lowercase letters, or by spaces, tabs, or line breaks.
Moreover, the platform used for software testing also significantly contributes to the efficacy of the process. For instance, the Stack Exchange network, a vast resource of QA communities, can be an invaluable asset for developers seeking to learn and share knowledge.
Additionally, tools such as Applitools can hugely benefit the process. This tool can be used to verify websites, mobile applications, and PDFs, and provides functionality like the ImageTester.jar, which can verify PDF forms from the command line. The ImageTester.jar can be integrated into the resources directory of an automation project and executed using commands to execute PDF comparisons. The results can then be inspected in the Test Manager dashboard for evaluation.
In conclusion, by following these practices, the efficiency and accuracy of PDF file content verification with Selenium can be significantly enhanced
7. Practical Tips to Assert PDF Text and Validate Contents of Downloaded PDFs
Asserting and validating text in PDF files during Selenium unit testing can be complex, especially when dealing with downloaded PDFs. However, leveraging certain techniques can simplify this process and ensure accurate results.
For asserting the text in a PDF, a string comparison that disregards case and whitespace differences is a preferred method. This can be achieved using text comparison libraries or frameworks that provide options for case-insensitive and whitespace-insensitive matching.
When it comes to validating downloaded PDFs, a checksum comparison is a suggested approach. This technique verifies the integrity of the downloaded file, ensuring that the PDF has been accurately downloaded without corruption. To execute this, one can use various algorithms such as MD5, SHA-1, or SHA-256 to calculate the checksum of the downloaded PDF file.
Extracting text directly from PDFs is a challenging task, but open-source libraries such as Apache PDFBox and PDF.js can aid in transforming the PDF into structured data. For instance, Apache PDFBox can be used in combination with Selenium WebDriver to extract text for assertion during unit testing.
Handling challenges such as over split and under split lines, ligatures, hidden text, and extra spaces can be tackled with specific strategies. For example, over split lines can be resolved by merging lines with sufficient overlap and a low enough gap, while extra spaces can be handled by deduplicating spaces, trimming lines, and performing fuzzy comparisons.
Lastly, it's vital to remember that specific phrases can be found using fuzzy comparisons based on edit distance. Values of particular types can be extracted by stripping out whitespace or using NLP engines like GPT-3.
In conclusion, while asserting and validating PDF content during Selenium unit testing can be complex, with the right methods and tools, it can be effectively managed. This leads to an accurate and reliable unit testing process
8. Balancing Workload and Deadlines: Strategies for Optimizing Your Selenium Testing Efforts
As we navigate the vast realm of software testing, it is crucial to strike a balance between dynamic workloads and looming deadlines. The optimization of testing efforts, particularly when using Selenium, can be significantly enhanced by adopting a few key strategies.
Prioritizing tests is a vital first step. This can be achieved by assessing the criticality of the functionalities they cover. By concentrating on the most crucial aspects first, we ensure that the most important parts of the application are tested thoroughly and efficiently.
Simultaneously, the Machinet AI plugin emerges as a vital tool in this process. This plugin, designed specifically for developers, integrates with the Machinet platform and offers a set of tools that streamline the development process. It provides automated code generation, intelligent code suggestions, and code optimization, which help accelerate workflow, reduce manual coding efforts, and improve overall productivity.
Selenium's parallel test execution feature is another potent strategy to reduce testing time. It allows multiple tests to be run concurrently, significantly reducing the time required to run a suite of tests. This is particularly beneficial when dealing with large applications with numerous features that need to be tested.
Incorporating a continuous integration tool, such as Jenkins, is an effective method to optimize the testing process. These tools automate the testing process, ensuring that tests are run regularly and results are reported promptly. This not only saves time but also ensures that any new changes or additions to the code are tested immediately, keeping the development process agile and efficient.
The use of good coding conventions in your Selenium C# automation is also crucial. This includes using PascalCase for method and class names, camelCase for local variables and parameters, and avoiding abbreviations and underscores in variable names.
The DRY (Don't Repeat Yourself) principle is another key principle to incorporate in your testing strategy. By creating setup methods for common actions like logging in and using variables to store web elements for reuse, you can avoid code duplication and make your automation frameworks more efficient and manageable.
Independent tests that do not rely on the outcome of other tests are important. This can prevent unexpected failures and make your test suite more robust. Additionally, adhering to the Single Responsibility Principle by creating short tests that focus on a single functionality can make your tests more readable and maintainable.
Finally, using appropriate locator strategies in Selenium C# can accelerate the process of finding elements on a webpage. The use of ID as the most preferred locator strategy, followed by className and cssSelector, can significantly improve the efficiency of your tests.
With the integration of the Machinet AI plugin and these strategies, you can optimize your Selenium testing efforts, meet your deadlines, and ensure that the quality of your software remains uncompromised.
Try the Machinet AI plugin and boost your Selenium testing productivity.
The Machinet AI plugin is indeed an essential tool for developers looking to streamline their coding workflow and enhance their coding speed and efficiency
Conclusion
In conclusion, Selenium is a powerful open-source tool that plays a crucial role in software testing, particularly in unit testing. It provides a robust platform to authenticate the functionality of different components within a software application. With its automation capabilities, Selenium simplifies software testing and makes it more reliable by simulating user interactions with a web application. By automating tasks like button clicks, form submissions, and functionality checks, Selenium ensures that every website element operates as expected.
The importance of Selenium in unit testing cannot be overstated. It allows developers and testers to detect issues early on and ensure that websites and applications function correctly. Its compatibility with multiple programming languages, such as Java, makes it a versatile choice for both developers and testers. By following best practices and integrating tools like Apache PDFBox for validating text in PDF files, developers and testers can enhance their testing efforts and ensure the accuracy and reliability of their software applications.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.