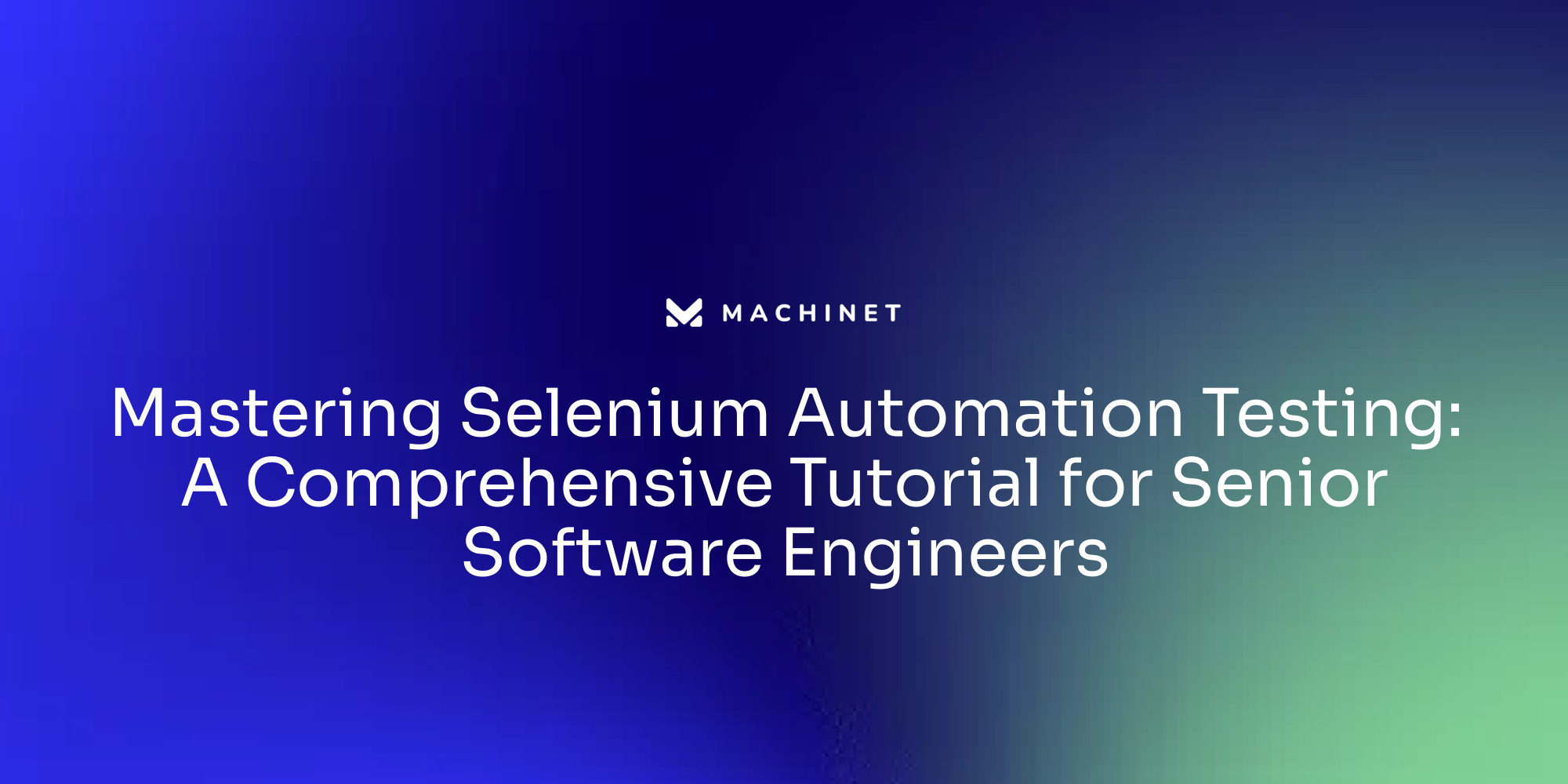
Table of Contents
- Understanding Selenium Automation Testing: An Overview
- Setting Up the Environment for Selenium Automation Testing
- Diving Deep into Selenium WebDriver with Java
- Strategies to Manage Technical Debt and Legacy Code in Selenium Automation Testing
- Implementing Robust and Flexible Testing Frameworks with Selenium
- Workload Management and Deadline Balancing in Selenium Automation Testing
- Addressing Common Challenges in Selenium Automation Testing for Senior Software Engineers
- Leveraging Context-Aware AI to Generate Java Unit Tests in Selenium
Introduction
Selenium automation testing is a vital component of software development, offering a versatile framework to interact with web applications and validate their functionality. However, this powerful tool is not without its challenges. From managing dynamic web elements to handling pop-ups and alerts, navigating iframes, and synchronizing tests, experienced software engineers often face hurdles that can impact the efficiency and effectiveness of their testing efforts.
In this article, we will explore the common challenges faced by senior software engineers in Selenium automation testing and discuss strategies and tools to overcome these obstacles. We will delve into topics such as managing dynamic web elements, handling pop-ups and alerts, navigating iframes, and synchronizing tests. Additionally, we will explore the role of AI-driven testing platforms and their potential to enhance the automation testing process.
By understanding these challenges and leveraging appropriate strategies and tools, senior software engineers can optimize their Selenium automation testing efforts and deliver high-quality software products efficiently and effectively
1. Understanding Selenium Automation Testing: An Overview
Selenium, an open-source tool for automating web browsers, is an integral part of software development. It's a versatile testing framework that supports various programming languages, including Java - one of the most prevalent languages in software development. Selenium interacts with websites and applications similar to a virtual assistant, executing tasks such as clicking buttons, filling out forms, and verifying operation.
Operating by launching a web browser, navigating to a specified website, and performing actions like clicking and typing, Selenium verifies the correctness of these actions. It's ability to automate repeatable tests, execute tests rapidly, and ensure consistent testing makes it a vital asset in the software testing process.
Unique to Selenium is its ability to test various segments of a website, ensuring no element remains unchecked, guaranteeing a flawless user experience. Its integration with other tools in the software development process extends its utility and versatility.
However, Selenium focuses on testing the underlying code, not the visual layer of an application, potentially resulting in missed visual bugs or regressions. Crafting and maintaining Selenium tests can be time-consuming and require specialized coding skills. Debugging test failures in Selenium can also be lengthy, as it lacks built-in features to identify the cause of failures.
Rainforest QA, a tool that interacts with the visual layer of the application, validates the user's experience. It's a no-code platform that enables swift creation and maintenance of tests, without the need for coding skills. Rainforest QA has built-in features to render tests resilient to changes in the application and offers text matching for flexible testing options. It provides video replays of test failures, simplifying the process of understanding and troubleshooting issues.
Additional features of Rainforest QA such as testing actions outside the browser, managing manual and automated testing from the same platform, and scaling up testing without additional tools make it an effective solution to Selenium's limitations.
In essence, while Selenium is an essential tool in the software testing process, its limitations can be effectively addressed by platforms like Rainforest QA, leading to a more comprehensive and efficient testing process
2. Setting Up the Environment for Selenium Automation Testing
Setting up a Selenium automated testing environment involves several key steps. The first step is installing the Java Development Kit (JDK) on your machine. Selenium uses Java for script writing, so this is a crucial step. Download the JDK installer from the official Oracle website, run it, and follow the on-screen instructions to complete the installation process. Once installed, set up the environment variables by adding the JDK bin directory to your system's PATH variable. You can verify the installation by opening a command prompt or terminal window and typing "java -version". This should display the installed JDK version.
The next step is setting up an Integrated Development Environment (IDE). There are several popular IDEs for Selenium automation testing, such as Eclipse, IntelliJ IDEA, and Visual Studio Code. Download and install your chosen IDE, then configure it for Selenium automation testing, which typically involves adding the necessary plugins or extensions specific to Selenium. After that, set up Selenium WebDriver, a crucial component for Selenium automation testing. Download the WebDriver for the browser you intend to automate (e.g., ChromeDriver for Google Chrome) and configure it with the IDE.
Another key step is creating a new project for your Selenium automation testing. This project will contain your test scripts and related files. Add the necessary Selenium dependencies to your project. This can be done by adding the Selenium WebDriver libraries to your project's build path or by using a dependency management tool like Maven or Gradle. You can then start writing your Selenium test scripts using your chosen programming language. Once the test scripts are ready, execute them from within the IDE, which will provide feedback on the test execution, including any failures or errors encountered.
Remember, Selenium is not limited to just Java. It can also work with Node.js, which can be particularly beneficial if you are proficient in JavaScript. To set up Selenium with Node.js, initialize an npm project by creating a project folder and running the command "npm init". Install the necessary npm packages, which include "selenium-webdriver" and "chromedriver". Then, create a folder called "test" and add a "test.js" file inside it. This file will contain your test, which involves opening a Chrome browser, navigating to Google's website, inputting a search term, pressing enter to search, and then closing the browser.
For Python enthusiasts, Selenium can also be set up with Python using the Python unittest framework. This process involves installing the latest Python build, ensuring that pip is installed, and downloading the latest Selenium client and its webdriver bindings. The tutorial recommends using virtualenv to isolate the test environment from other setups and ensure that the tests run with the specified versions of the modules. After setting up your Lambdatest credentials, you can run test automation scripts on the Lambdatest Selenium grid.
Remember, the specific steps and procedures may vary based on the IDE you choose and your project requirements. Always refer to the official documentation and resources available for your chosen IDE and Selenium WebDriver for detailed instructions
3. Diving Deep into Selenium WebDriver with Java
Selenium WebDriver, a cornerstone of the Selenium suite, provides an intuitive API which enables the creation of functional tests that interact with web applications. This tool is language-agnostic, supporting various programming languages, but we will focus on Java for this tutorial. The WebDriver operates at the operating system level, interacting with web elements in a manner akin to human interaction. This makes it more effective and versatile than other Selenium components. It is capable of managing a diverse range of web elements and can perform numerous operations including clicking, typing, selecting from dropdowns, and more. Developing a comprehensive understanding of WebDriver is a vital step towards mastering Selenium automation testing.
The WebDriver is an object-oriented automation API that operates a browser as a user would. Its versatility and efficiency are enhanced by its compatibility with multiple programming languages, with the emphasis on the Java implementation in this tutorial. The WebDriver allows for a diverse range of interactions with web elements, including the ability to click, type, and select from dropdowns. It can also handle pop-ups, alerts, and file uploads, as well as work with iframes. More advanced interactions such as hovering and sending alternative keys are also supported.
The WebDriver can also be integrated with a test assertion library and can be customized to run headless tests or navigate multiple open tabs within a browser. Additionally, it offers the ability to manage cookies and write custom actions using JavaScript, making it a highly powerful tool for test automation.
This tutorial will guide you through topics such as installing Selenium WebDriver and its dependencies, launching and interacting with websites using the WebDriver API, finding elements within a web application, and more. To reinforce learning, optional independent exercises are included. This course is available on Test Automation University and is offered by Angie Jones. It is copyrighted by Applitools.
In tandem with this, it's pertinent to note that automated UI tests simulate the actions of a manual tester, which is why Selenium WebDriver is an indispensable tool for automated testing. Setting up the Selenium UI testing environment involves installing the IDE, creating a Maven/Gradle project, and downloading the correct WebDriver for the desired browser. The WebDriver allows you to identify web elements in Chrome and Firefox using CSS and XPath selectors, and it's crucial to understand the differences between the two.
Creating and running automated Selenium UI tests involve writing test classes, identifying web elements, and performing actions on them. To compare the actual and expected results of the tests, creating assertions is essential. Finally, the tests can be executed from the IDE, command line, or with BlazeMeter. The tutorial also covers creating a custom test report using a reporting library like Extent and the benefits of integrating the project with Git and Jenkins. The blog post concludes by providing related resources for further learning
4. Strategies to Manage Technical Debt and Legacy Code in Selenium Automation Testing
The complexities that come with working with legacy code and managing technical debt in Selenium automation testing are significant, yet not insurmountable. Legacy code, often referred to as "spaghetti code," can be challenging due to its complicated nature, slow feedback cycles, and lack of automated tests, among other issues. However, there are effective strategies to handle these challenges.
One approach to managing legacy code is to break it down into smaller, more manageable parts. This can be achieved by analyzing the existing test cases, identifying common functionalities, and refactoring these into separate methods or classes to reduce code duplication and enhance modularity. Using data-driven testing techniques, where test inputs are parameterized and separated from the test logic, can also aid in maintenance and reusability. Test automation frameworks like TestNG or JUnit, which offer features for test grouping, parallel execution, and test dependency management, can further assist in making legacy tests more manageable.
Technical debt, a metaphor for the future cost of making the code maintainable again, can accumulate due to suboptimal choices and changes made in an unsustainable way. There are several strategies to manage technical debt in Selenium automation testing, such as regular maintenance to keep automation test scripts updated, code refactoring to improve maintainability and readability, following good test design principles like the Single Responsibility Principle (SRP) and Don't Repeat Yourself (DRY) principle, and implementing a continuous integration (CI) and continuous testing (CT) approach. Regular communication and feedback between developers and testers can lead to better test coverage and reduced technical debt.
Another key strategy is to prioritize technical debt reduction based on its severity and impact on the automation process. For example, high priority technical debt, which has the most significant impact on the automation framework, should be addressed first. This may involve fixing critical defects, refactoring code, or improving the stability and maintainability of the automation framework. Medium and low priority technical debt, which have a moderate and minimal impact respectively, can be addressed subsequently.
Additionally, regular code reviews play a crucial role in managing technical debt. They provide a platform for team members to review and analyze the code, identify potential issues, bugs, or design flaws early in the development process, and promote collaboration and knowledge sharing. This can help maintain code quality and consistency, and ensure the maintainability and scalability of the automation codebase.
In conclusion, managing technical debt and legacy code in Selenium automation testing is a complex task that requires careful planning and execution. By implementing these strategies, organizations can effectively manage technical debt and legacy code, ensuring the long-term success of their testing efforts
5. Implementing Robust and Flexible Testing Frameworks with Selenium
Testing frameworks, such as Selenium, have been instrumental in enhancing the testing process. The components of Selenium, such as Selenium WebDriver, offer the ability to interact with elements on a webpage and perform operations. Similarly, Selenium Grid provides the capability to execute tests across multiple machines and browsers simultaneously, broadening the scope of test coverage and boosting efficiency.
To set up a Selenium Grid infrastructure, a hub and multiple nodes need to be established. The hub acts as a central point to distribute test cases to the available nodes, which then execute the tests in parallel on different browsers and operating systems. This parallel execution can significantly reduce the overall test execution time and improve test efficiency.
In addition, testing frameworks like TestNG or JUnit can be used to manage tests, generate reports, and even integrate advanced features such as parallel test execution. TestNG provides a robust framework for organizing and generating test reports, making it a popular choice for Java unit testing.
However, Selenium, while a powerful tool, has its limitations. Conceived before the emergence of single-page applications and intricate HTML structures, Selenium faces challenges in the current landscape of web development. The heavy reliance of Selenium on XPath locators does not cater well to the complexities of modern web pages, and debugging tests in Selenium can be a formidable task due to the often cryptic nature of error descriptions.
To overcome these limitations, AI-driven testing platforms like TestRigor offer promising solutions. TestRigor not only offers AI testing, including generative AI in software testing but also provides features for both code and codeless testing. This includes testing across various platforms such as web, mobile, and desktop, as well as Salesforce automation and accessibility testing.
TestRigor also ensures compliance with regulatory standards such as 21 CFR Part 11. In addition, TestRigor also provides resources like documentation, certification, integrations, whitepapers, blogs, FAQs, tutorials, and events. Furthermore, it allows users to view recent public tests and provides case studies.
One of the unique aspects of TestRigor is its emphasis on end-to-end testing from the user's perspective. It proposes a different approach to end-to-end testing, focusing on elements from the user's perspective, such as labels and placeholders.
In light of these considerations, while Selenium does offer a robust and flexible framework for testing, it's important to consider its limitations and the potential benefits of AI-driven testing platforms like TestRigor. Leveraging these components and understanding their strengths and weaknesses can help create a testing framework that is not only robust and flexible but also more efficient and effective
6. Workload Management and Deadline Balancing in Selenium Automation Testing
Selenium automation testing, when deployed strategically, can significantly aid in workload management, deadline balancing, and ensuring the delivery of top-tier software. The key to effective workload management lies in prioritizing testing tasks based on their potential impact and associated risk. This approach necessitates focusing on areas of the application that pose the highest risk or are subject to frequent modifications.
The automation feature of Selenium can significantly lighten the workload, especially for repetitive tests. This not only conserves time but also minimizes the effort required, leading to a more efficient distribution of resources. Selenium's compatibility with all modern web browsers and operating systems adds to its versatility as a tool for managing testing workloads. The three main components of Selenium - Selenium IDE, Selenium WebDriver, and Selenium Grid - each play a critical role in this process.
Selenium IDE simplifies the recording and playback of test scripts, while Selenium WebDriver interacts with browsers and manipulates DOM elements. Selenium Grid facilitates parallel testing on multiple devices and browsers. These components work in harmony to streamline the testing process, thereby reducing the time required for test creation, execution, and maintenance.
For effective deadline balancing, it is crucial to consider the time it takes to create, execute, and maintain tests. Cloud-based testing platforms like LambdaTest can be integrated with Selenium to provide a scalable and cost-effective solution for testing web applications across multiple browsers and platforms. This further aids in deadline management by providing an online Selenium grid for running tests, which saves time and enhances test coverage.
However, it's crucial to remember that the ultimate goal extends beyond merely meeting deadlines. The focus should always be on delivering software of the highest quality. Techniques such as early testing of planned functionality and setting priorities can be employed to manage uneven workloads and prevent burnout, a common issue in the software industry.
In summary, Selenium automation testing, when leveraged effectively, can greatly assist in workload management and deadline balancing, while also ensuring the delivery of high-quality software. By implementing effective workload management strategies such as parallel test execution, test case prioritization based on business impact, and resource utilization monitoring, testers can optimize their testing efforts and maximize the value of their selenium automation testing.
Furthermore, to balance deadlines in Selenium automation testing, it is important to prioritize tasks and manage time effectively. This can be achieved by identifying critical test cases that need to be executed first and focusing on them, breaking down the testing tasks into smaller, manageable chunks for parallel execution, and setting achievable deadlines for each testing phase or iteration. Regular communication with stakeholders, developers, and other team members also plays a vital role in keeping everyone informed about the progress and any challenges faced during testing.
Lastly, to manage workload and deadlines in Selenium automation testing, it is important to prioritize tasks, set realistic deadlines, and allocate resources effectively. Additionally, implementing proper test case management and using test automation frameworks can help streamline the testing process and improve efficiency. Regular communication and collaboration with the development team and stakeholders can also ensure that everyone is on the same page regarding expectations and deadlines
7. Addressing Common Challenges in Selenium Automation Testing for Senior Software Engineers
In the sphere of Selenium automation testing, experienced software developers frequently face a series of challenges. Among these hurdles are the management of dynamic web elements, handling pop-ups and alerts, navigating iframes, and synchronizing tests. Yet, Selenium, a powerful testing tool, provides various strategies to mitigate these challenges.
To manage dynamic web elements, developers can utilize techniques such as XPath or CSS selectors, which locate elements based on their attributes or properties. Implicit or explicit waits can be used to ensure that elements are fully loaded before interaction, contributing to the robustness of the automation scripts. Dynamic element locators like partial link texts or regular expressions can also be employed to locate elements with changing attributes or values.
Handling pop-ups and alerts in Selenium automation testing can be efficiently done using the Alert
class. This class facilitates interaction with JavaScript alerts, confirmations, and prompts that might occur during test case execution. Methods such as accept()
, dismiss()
, getText()
, and sendKeys(String text)
can be utilized to handle pop-ups and alerts effectively.
Dealing with iframes in Selenium automation testing is another common challenge. Selenium provides methods like switchTo()
and frame()
to switch the driver's focus to the specified iframe, enabling interaction with the elements inside. After interacting with the elements inside the iframe, developers can switch back to the default content using the defaultContent()
method.
Synchronization of tests in Selenium automation testing is another crucial aspect. Techniques such as explicit waits, which pause the automation script until a certain condition is met, and implicit waits, which instruct Selenium to wait for a specified time before proceeding, can be applied. Synchronization methods like Thread.sleep() can also be effective in controlling the timing of test steps, and the power of Selenium's built-in wait methods, such as WebDriverWait, can provide more precise control over synchronization in automation testing.
In the context of API testing, which involves verifying the health and performance of every API within a software system, the challenges include unfamiliarity with APIs, ensuring the framework is suited for APIs, prioritizing scenarios, using correct testing approaches, and designing test cases properly. Comprehensive training on APIs, continuous testing throughout the development cycle, scenario prioritization based on user experience, and proper design of test cases can effectively address these challenges, leading to successful API testing and obstacle prevention.
A case in point is QASource, a seasoned provider of QA services, which offers a strategic guide for overcoming API testing challenges. By implementing best practices such as comprehensive training, continuous testing of APIs, prioritizing scenarios, using correct testing approaches, and designing test cases properly, QASource has been able to successfully navigate API testing challenges and adopt best practices.
Stack Overflow, a mature and complex product serving 100 million developers every month, found their test automation solution in Mabl, a tool known for its easy test creation and fast test execution capabilities. Mabl's integration with Stack Overflow's engineering workflow, including CI/CD pipelines, was a powerful feature that allowed them to make significant progress towards their testing goals.
In essence, whether it's Selenium automation testing, API testing, or test automation with tools like Mabl, understanding and effectively leveraging the tools and strategies available can help software engineers overcome common challenges and deliver high-quality software products
8. Leveraging Context-Aware AI to Generate Java Unit Tests in Selenium
As we delve deeper into the realm of Java programming and Selenium automation testing, the emergence of context-aware AI as a transformative force becomes clear. Such AI comprehends the scope of software applications and constructs appropriate test cases, leading to significant savings in time and resources. An exemplary platform in this arena is ContextQA, which offers advanced feature integrations and extensions, facilitating a streamlined testing process.
Covering various testing domains such as UI testing, mobile app testing, API testing, and Salesforce testing, ContextQA ensures comprehensive test coverage and peak performance across different software interfaces. For instance, its automated mobile app testing employs advanced algorithms and AI technology to detect glitches and enhance performance across various mobile devices and operating systems.
The adaptability of ContextQA is a key feature, allowing the generated tests to become more resilient and adaptable to evolving requirements. This adaptability proves particularly beneficial when dealing with changes in software projects and codebases, as the generated test cases dynamically adjust to accommodate these changes.
A real-world example of the effectiveness of ContextQA is the Techicom company, which was able to achieve 30% faster product releases by automating regression testing with ContextQA. This case study, along with testimonials from users, highlights the benefits of using ContextQA, including faster releases, improved productivity, and enhanced testing capabilities.
The question of whether AI can write better tests is raised in the Java programming ecosystem. In this context, the use of AI in generating unit tests is seen as a game-changer. AI-powered testing frameworks capable of analyzing and understanding the context of the application under test are leveraged. These frameworks use techniques such as natural language processing, machine learning, and computer vision to interpret the application's context and make intelligent decisions during test execution. This AI-driven approach can quickly identify potential edge cases, boundary conditions, and error-prone areas within the code, ensuring comprehensive test coverage.
Furthermore, context-aware AI techniques can help improve code quality in Selenium unit tests. These techniques analyze the code and provide insights and suggestions for improvement. They can detect potential issues, such as incorrect assertions, redundant or duplicate code, and inefficient test cases, and provide recommendations for best practices, such as proper use of annotations and assertions, and suggest ways to enhance the maintainability and readability of the code.
The integration of context-aware AI tools like Machinet into Selenium automation testing can be a valuable addition to the testing process. By leveraging Machinet's AI capabilities, the accuracy and efficiency of tests can be enhanced. From generating realistic and context-aware test data to simulating user behavior based on real-world usage patterns, or identifying and prioritizing test cases based on the impact on end users, Machinet's context-aware AI offers numerous benefits.
In summary, harnessing the power of context-aware AI can significantly improve the Selenium automation testing process, enhance code quality, and increase productivity. The benefits of using such AI in generating Java unit tests are numerous, and by leveraging this advanced approach, developers can enhance the efficiency and effectiveness of their unit testing efforts, leading to higher quality software products
Conclusion
In conclusion, Selenium automation testing is a vital component of software development, offering a versatile framework to interact with web applications and validate their functionality. However, experienced software engineers often face challenges such as managing dynamic web elements, handling pop-ups and alerts, navigating iframes, and synchronizing tests. These challenges can impact the efficiency and effectiveness of testing efforts. To overcome these obstacles, strategies and tools such as Rainforest QA can be utilized. Rainforest QA provides a no-code platform that simplifies test creation and maintenance, offers features to handle changes in applications, and provides video replays of test failures for easier troubleshooting. By understanding these challenges and leveraging appropriate strategies and tools, senior software engineers can optimize their Selenium automation testing efforts and deliver high-quality software products efficiently and effectively.
In addition to addressing specific challenges in Selenium automation testing, the article also highlights the broader significance of AI-driven testing platforms. Platforms like TestRigor offer promising solutions to overcome limitations in Selenium, such as difficulties in handling modern web development techniques and debugging tests. These AI-driven platforms provide advanced features like generative AI in software testing, codeless testing, cross-platform compatibility, Salesforce automation, accessibility testing, compliance with regulatory standards, documentation resources, case studies, and more. By considering the potential benefits of AI-driven testing platforms like TestRigor alongside Selenium's capabilities, organizations can create a more comprehensive and efficient testing process.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.