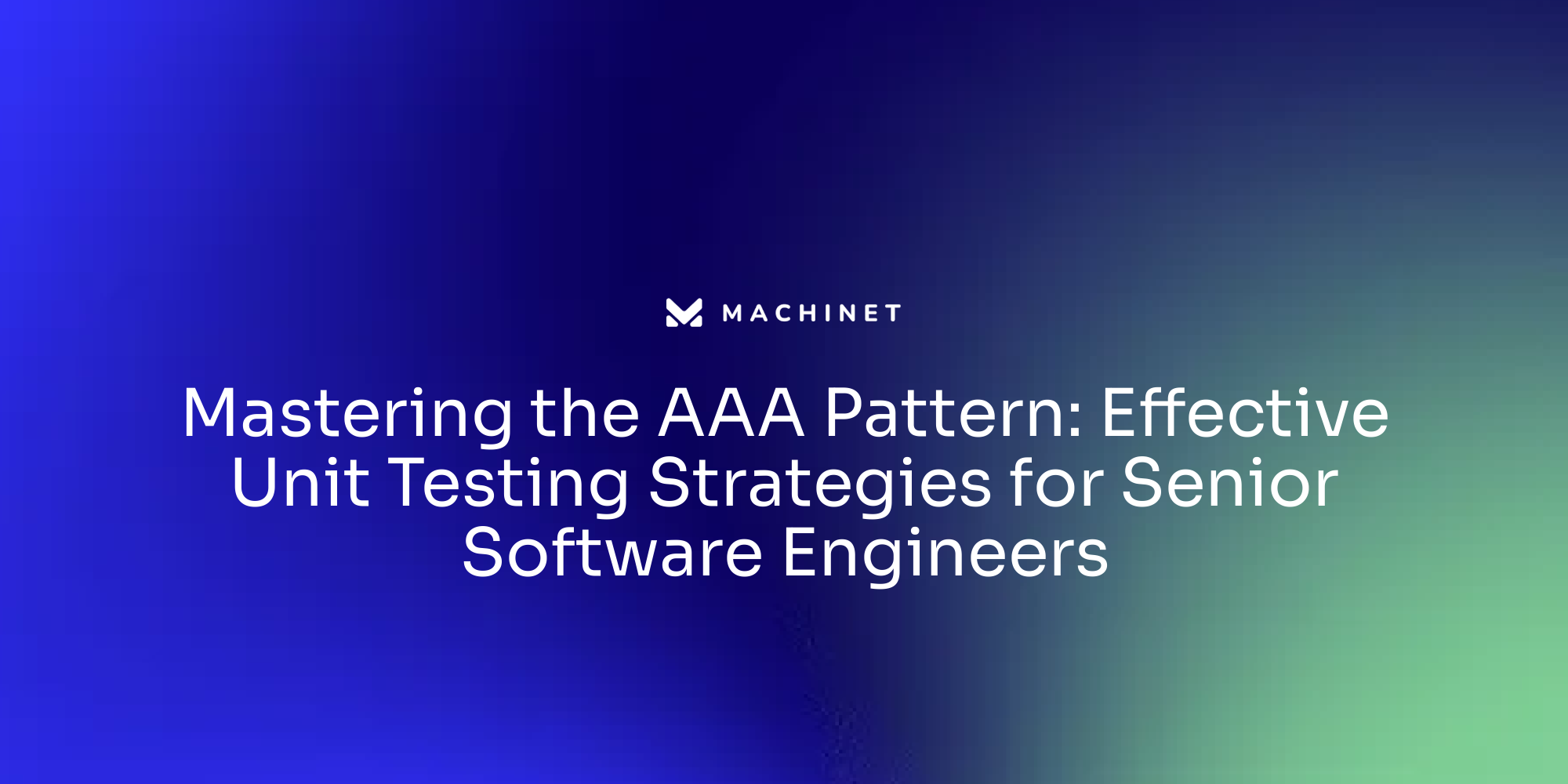
Table of Contents
- Unveiling the AAA Pattern: A Comprehensive Overview
- Implementing the AAA Pattern in Unit Testing: A Step-by-Step Guide
- Best Practices for Unit Testing with AAA Pattern: Tips and Tricks for Success
- The Role of the AAA Pattern in Managing Technical Debt and Legacy Code
- Adapting to Changing Requirements: The Flexibility of the AAA Pattern in Unit Testing
- Balancing Workload Management and Deadlines with Effective Unit Testing Using the AAA Pattern
- Bridging the Gap Between Development and Testing Teams: Communication Strategies with the AAA Pattern
Introduction
The Arrange-Act-Assert (AAA) pattern is a widely recognized and versatile approach to structuring unit tests in software development. It provides a systematic framework that enhances the efficiency and effectiveness of testing. By dividing the testing process into three distinct phases - Arrange, Act, and Assert - the AAA pattern promotes clarity, maintainability, and reliability in unit tests.
In this article, we will explore the benefits and applications of the AAA pattern in various aspects of software development. We will discuss how the AAA pattern can accelerate sales cycles, align sales and marketing efforts, manage technical debt, adapt to changing requirements, balance workload management, and bridge the gap between development and testing teams. By understanding and implementing the AAA pattern, software engineers can optimize their testing practices and deliver higher-quality software products
1. Unveiling the AAA Pattern: A Comprehensive Overview
The Arrange-Act-Assert (AAA) pattern is a highly acknowledged technique for structuring unit tests in software development. This systematic approach is categorized into three distinct phases that warrant every test remains focused, easily comprehensible, and maintainable.
In the Arrange phase, the foundation for the test is laid. This could involve initializing objects, creating mock data, or setting up method calls. The Act phase then takes over, executing the functionality that's under test. The final phase, Assert, verifies if the outcome of the Act phase matches our expectations.
Nonetheless, test fragility is a common concern in test-driven development (TDD). Production code changes can unintentionally lead to extensive modifications in the tests.

To counter this, the tests' structure should not directly reflect the production code. Instead, it should be independent, leading to a contra variant design. This separation of structure and behavior makes TDD more effective and less prone to frailty.
As the tests become more specific, the production code should simultaneously become more generic. This allows for a broader range of behaviors and reduces coupling, increasing system flexibility. It's crucial to continue generalizing the production code until no new failing tests can be written.
Despite these advantages, the value of unit testing is sometimes questioned by developers. They contend that it's challenging to perceive the benefits of writing code to prevent defects in code. However, the correlation between lines of code and defects suggests that defects tend to cluster in hotspots. Adding test code can be acceptable if those hotspots are kept bug-free.
Trust in test code can be established by observing its failure and ensuring its simplicity. For instance, the decision to add branching logic to a unit test should be made deliberately, with an assurance that each branch is covered by a test case. However, adding branching logic may increase the test's cyclomatic complexity.
Alternatives to branching in tests could include introducing a test helper or using parameterized tests. Regardless of the approach, it's critical to move deliberately and avoid jumping to conclusions.
When using the AAA pattern, it's pivotal to avoid some common pitfalls. One such mistake is not properly arranging the test data before executing the action, leading to incorrect or unexpected results. Another mistake is not clearly defining the expected outcomes in the assert step. Without clear expectations, it becomes difficult to determine whether the test has passed or failed. Additionally, avoiding coupling the test cases too closely to the implementation details is crucial as this can make the tests brittle and difficult to maintain. Lastly, ensuring that each test case is independent and does not rely on the state of previous tests allows for easier debugging and isolation of issues.
By understanding and implementing the AAA pattern in this way, the readability, maintainability, and effectiveness of unit tests are enhanced, ultimately leading to higher-quality software products. Integrating the AAA pattern into a testing framework is a best practice in software testing that ensures consistent and effective testing practices across your codebase
2. Implementing the AAA Pattern in Unit Testing: A Step-by-Step Guide
The Arrange-Act-Assert (AAA) pattern is a widely recognized strategy for structuring unit tests, offering a simple yet effective way to validate code. This pattern is divided into three clear phases.
The first phase, known as "Arrange", is about setting up the test environment. This phase may involve initializing objects, setting up dependencies, and providing necessary input data. The goal is to ensure the system is in a known state before proceeding to the next phase, thereby laying the groundwork for the test.
The second phase, "Act", is about executing the functionality being tested. This phase involves calling the method or performing the action that is under scrutiny. It is a critical phase as it facilitates the observation of the expected behavior and the validation of the test results.
The third and final phase, "Assert", is where the results of the action undertaken in the "Act" phase are validated. This could involve checking an object's state, confirming a method's return value, or asserting that a particular exception was thrown. The goal is to ensure the test outcome is consistent with the expected result.
The AAA pattern is versatile and can be implemented across a variety of testing frameworks, including Jasmine. For instance, when testing a user model in JavaScript, the "Arrange" phase might involve setting up the user model with specific data. The "Act" phase could involve calling the getFullName
method on the user model, and the "Assert" phase would then check that the returned value is the user's full name.
Adhering to the AAA pattern allows you to create unit tests that are clear, concise, and effective. It promotes thorough testing, covering all aspects of a code module. It's worth noting that the internal state of the code being tested is not the primary concern of unit testing. Instead, the focus is on the 'contact surface' with the external world.
The AAA pattern encourages the use of concrete values in tests, reducing uncertainty and making debugging easier. It also emphasizes the importance of assertions or validations to ensure the effectiveness of tests. The pattern is applicable not only to unit testing but also relevant for all types of testing, from automation to manual testing. Keep in mind that a test without proper assertion or validation is a common pitfall to avoid.
Experts in the field, like Jay Cruz and Timothy Foster, have expressed their appreciation for the AAA pattern. Cruz states, "Testing is an essential part of building software" and "the AAA pattern provides simple but effective steps for testing our code." Foster shares his enthusiasm for the AAA pattern, saying, "I use it often when teaching to devs unfamiliar with testing." He also highlights the importance of concrete values in tests, stating, "The test should have concrete values so you don't have to test the test."
By following the AAA pattern, you can ensure your unit tests are clear, concise, and effective. It provides straightforward and efficient steps for testing code, covering all aspects of a code module. This results in reliable and well-tested code with fewer bugs, making it an invaluable tool for any software engineer
3. Best Practices for Unit Testing with AAA Pattern: Tips and Tricks for Success
The AAA pattern, a structured approach to unit testing, assures comprehensive and reliable tests that are easy to maintain. To fully reap the benefits of this methodology, it's imperative to adhere to certain best practices.
Emphasizing the independence of each test is a fundamental tenet of the AAA pattern. This means one test's outcome does not influence another, eliminating any unexpected side effects. An example of this principle in action can be found in the Refactoring Ninja GitHub project. In this project, the code for Divinity: Original Sin's turn queue bar was divided into separate visual and logic components, upholding the Single Responsibility Principle (SRP) and facilitating independent testing for each code unit.
The practice of keeping tests small and focused is also crucial. Each test should concentrate on a single behavior, simplifying the process of identifying and resolving issues. This was demonstrated during the refactoring of the turn queue bar in the Divinity: Original Sin project, where the code was split into two classes, "TurnQueueBar" and "TurnQueue", each with its own unique functionality and testable behavior.
Assigning meaningful names to your tests is another key practice. The test names should articulate what the test is evaluating, providing clarity for future code reviewers. This was illustrated when an interface named "ITurnQueue" was introduced in the refactored code of the Divinity: Original Sin project. The name "ITurnQueue" succinctly conveys the purpose of the interface, enhancing its comprehensibility and testability.
Avoiding the introduction of logic into your tests is essential to keep them simple and straightforward. This principle was underscored during the testing process of the Divinity: Original Sin project, which revealed the challenges of testing dynamic, frequently changing visual components. The coder concluded it would be more beneficial to focus on testing the business logic, which is less variable and easier to verify.
Lastly, using mock objects and stubs to isolate the code under test can be invaluable. This ensures the tests are assessing only the intended code, without interference from other components. This principle was demonstrated in the Divinity: Original Sin project, where the "TurnQueue" class was isolated via the "ITurnQueue" interface, thus simplifying testing.
By adhering to these best practices, developers can enhance the effectiveness of their unit tests, resulting in superior software products. These practices are exemplified in the Refactoring Ninja GitHub project and the work of companies like Sweet Mustard, which regularly hosts Innovation Days to explore new technologies and methodologies, including unit testing with the AAA pattern.
The solution context underscores the importance of writing small, focused unit tests for ensuring efficient and effective testing. It offers several tips to achieve this, such as focusing each unit test on a specific code functionality, keeping tests simple and concise, testing boundary conditions, maintaining test case independence, using appropriate assertions, testing error handling, and regularly refactoring unit tests. These tips further reinforce the best practices associated with the AAA pattern and can significantly enhance the quality of your code testing process
4. The Role of the AAA Pattern in Managing Technical Debt and Legacy Code
Managing technical debt and outdated code can be a daunting task for software engineers. The Arrange-Act-Assert (AAA) pattern emerges as a potent tool to handle these complexities. It provides a systematic framework to craft tests, fostering the development of maintainable, high-quality tests. These tests act as a safety net during the process of refactoring or updating legacy code, significantly minimizing the chances of new bugs or regressions.
The AAA pattern champions the creation of targeted and self-contained tests. This approach ensures the test suite remains robust and reliable, even as the codebase evolves and expands over time. The AAA pattern's ability to uphold the test suite's integrity, regardless of the codebase's growth, underscores its critical role in managing technical debt and preserving a codebase's health.
Technical debt can significantly impede a project's progress in the realm of software development. Such debt, often a result of hurried or poorly planned coding efforts, can lead to a codebase that's challenging to maintain and upgrade. Agile development methodologies, like the AAA pattern, can help manage and reduce this debt.
Automated testing and continuous integration are two other vital elements in maintaining high-quality agile development. The AAA pattern, with its emphasis on clear, maintainable tests, plays a significant role in this process. By ensuring that each unit test is well-structured and focuses on a single functionality, the AAA pattern contributes to a more manageable and healthier codebase.
The AAA pattern also aids in modernizing legacy systems, in addition to managing technical debt. The process of replacing or upgrading outdated systems can be fraught with challenges. However, by breaking the problem into smaller, more manageable parts, the AAA pattern can facilitate the delivery of new requirements while improving technology. This approach, coupled with the necessary organizational changes and a shift in culture, can pave the way for successful legacy modernization.
Here is how the AAA pattern can be implemented for legacy code:
- Arrange: Set up the necessary preconditions for the test. This may involve creating objects, setting up dependencies, and initializing any required data.
- Act: Perform the action that you want to test. This could be calling a method, invoking a function, or triggering a specific behavior.
- Assert: Verify the outcome of the action and check if it matches the expected results. This could involve comparing values, checking for exceptions, or validating the state of objects.
By adhering to the AAA pattern, you can organize your tests in a structured manner, making them more readable and maintainable. With its emphasis on structure, maintainability, and focus in unit testing, the AAA pattern is undoubtedly an invaluable tool in a software engineer's arsenal for managing technical debt, maintaining the health of a codebase, and facilitating the modernization of legacy systems
5. Adapting to Changing Requirements: The Flexibility of the AAA Pattern in Unit Testing
The AAA (Arrange-Act-Assert) pattern, a fundamental component of unit testing, is celebrated for its inherent adaptability. This adaptability becomes particularly advantageous when dealing with evolving software requirements, a common occurrence in the dynamic landscape of software development.
The AAA pattern distinguishes itself with its focus on individual behavior. This focus equips the pattern with the ability to effectively adapt to alterations in the requirements of that behavior. To mirror these changes, updating the corresponding test is all that's needed, ensuring that the test suite remains current as the software continues to evolve.
Adapting the AAA pattern to changing software requirements can be complicated. The AAA pattern is widely used in unit testing to shape test cases, promoting clarity in the setup, execution, and verification steps of a test. When software requirements transform, it may necessitate modifications to the arrangement or assertion steps of the AAA pattern. This could encompass updating the setup of test data or fine-tuning the expected outcomes. By adhering to good coding practices and maintaining modular and flexible code, the process of adapting the AAA pattern to changing software requirements becomes more manageable.
Furthermore, the AAA pattern's emphasis on the utilization of mock objects and stubs enhances its adaptability. This strategy simplifies the process of modifying the setup of a test to accommodate changes in the dependencies of the code being tested. The use of mock objects and stubs in the AAA pattern is a common practice in unit testing. By creating mock objects and stubbing dependencies, developers can isolate the code under test and simulate different scenarios to ensure its correctness.
To reflect changes in dependencies, developers can update the behavior of the mock objects or stubs. This can be achieved by setting up the desired behavior using methods like when
or given
to define the expected inputs and outputs. By updating the behavior, developers can simulate different scenarios and observe how the code under test reacts to those changes.
Another approach involves the use of a mocking framework like Mockito. This framework offers utilities for creating mock objects and stubbing their behavior. With Mockito, developers can easily define the behavior of the mock objects and stubs, and also verify that certain interactions with the dependencies have occurred.
Additionally, the flexibility of the AAA pattern extends to reducing the coupling between tests and production code. This is achieved through the design of independent tests using the AAA pattern, which means that refactoring the production code won't break all the tests if they are designed properly.
In essence, the AAA pattern is an indispensable tool for maintaining an effective and relevant test suite, especially in the face of changing requirements. Its flexibility aids in managing the evolving landscape of software development, thus proving to be an invaluable asset for contemporary software engineers
6. Balancing Workload Management and Deadlines with Effective Unit Testing Using the AAA Pattern
The Arrange-Act-Assert (AAA) pattern is a recognized approach to structuring unit tests, enhancing the efficiency of software development. This pattern introduces a systematic structure to the testing process, which optimizes the time spent on writing and maintaining tests. This optimization allows for the allocation of time to other vital tasks within the development lifecycle, aiding in workload balance and deadline adherence.
The AAA pattern is especially advantageous when dealing with time-dependent classes, which are known to be difficult in unit testing. Traditional testing methods for these classes can result in slow and unreliable tests. However, using the AAA pattern alongside tools like the NodaTime and NodaTimeTesting packages can alleviate these issues. For example, the FakeClock class from NodaTimeTesting enables the simulation of different points in time, a crucial aspect when testing time-dependent classes, such as a video game combat skill with a cooldown period.
The use of these tools within the AAA pattern framework helps create reliable and consistent unit tests, enabling early bug detection in the development process. This early detection reduces the need for extensive debugging and bug fixing, further contributing to workload management and efficient time utilization.
Consider a case where xUnit is used to write unit tests for a skill class in a video game. The NodaTime package is utilized to inject the concept of time as a dependency, while the FakeClock class simulates different points in time for testing. The FakeClock class even allows for advancing the clock by a specific duration to simulate the passage of time, essential for testing a combat skill with a cooldown period. This approach, using the AAA pattern, results in reliable unit tests for time-dependent classes, emphasizing the importance of NodaTime and its FakeClock class in projects dealing with DateTime.
Roy Osherove, a respected figure in software development, is known for his on-site training, consulting, and agile XP consulting services. His expertise and views on effective software development, including test-driven development (TDD) and unit testing, are widely respected. He emphasizes the importance of writing for 5 minutes, a practice that aligns with the efficiency and time-saving aspects of the AAA pattern. His work, such as the online unit testing and TDD training content made free during the COVID-19 pandemic, or his discussions about unit testing entry and exit points, further underscore the significance of efficient and reliable unit testing practices.
In summary, the AAA pattern's systematic approach to unit testing, when used with the right tools and methodologies, can significantly enhance the efficiency of software development. It can lead to the creation of high-quality tests that catch bugs early, thereby reducing debugging time and effort, managing the workload effectively, and ensuring timely delivery of software products."
The AAA pattern is implemented by following the Arrange, Act, and Assert approach. During the Arrange phase, the necessary preconditions for the test are set up. This includes creating objects, initializing variables, and configuring the environment. In the Act phase, the specific action or behavior to be tested is performed. This typically involves calling a method or function and passing in the necessary inputs. Lastly, in the Assert phase, the expected outcome of the test is verified by comparing the actual result with the expected result to see if they match.
To balance workload management with unit testing, it is crucial to prioritize your workload and allocate sufficient time for unit testing. Planning ahead, breaking down tasks into manageable segments, and allocating time for unit testing within each task can help manage the workload more effectively. Utilizing automation tools and frameworks can save time and ensure consistent and reliable testing. Involving team members in the unit testing process, implementing continuous integration practices, and keeping track of your unit testing progress are also essential tips to balance workload management with unit testing
7. Bridging the Gap Between Development and Testing Teams: Communication Strategies with the AAA Pattern
The AAA pattern is not just a methodology for structuring unit tests, but it also serves as a robust communication and collaboration tool between development and testing teams. This pattern's standardized structure for tests fosters mutual comprehension of the test's purpose and validation points, paving the way for improved synergy. It also enables the testing team to gain an in-depth understanding of the software's behavior and requirements, thereby providing them with the ability to offer valuable feedback and insights.
A clear exemplification of the AAA pattern's potential as a communication tool was witnessed at the Agile Testing Days 2023 conference. This event, held at the Dorint Hotel in Potsdam, Germany, highlighted the criticality of establishing effective relationships between developers and testing teams to enhance software quality. Not only did the conference feature insightful speakers, but it also facilitated wider participation through virtual passes.
A standout moment from the conference was a blog post by Tristan Lombard titled "How to Build More Effective Relationships with Your Developers". Lombard emphasized the need to reevaluate traditional testing strategies and adopt a whole-team approach to quality. He suggested that probing questions and a "show me" approach could encourage developers to expose areas of code that may require additional scrutiny. This approach to testing mirrors the principles of the AAA pattern, underlining its efficacy.
Lombard also touched upon the shift towards a DevOps culture and the necessity of aligning software testing with development teams. He introduced the DORA metrics (Deployment Frequency, Lead Time, Change Failure Rate, and Mean Time to Recovery) as a measure of developer output and production quality. This discussion further accentuated the relevance of the AAA pattern in today's dynamic software development landscape.
The relationship between developers and testers in an agile team can be likened to characters in buddy cop films. Despite initial differences, they eventually find common ground and work towards a shared objective. This analogy perfectly encapsulates the essence of the AAA pattern, which aims to foster collaboration and dissolve traditional silos to improve software quality.
The AAA pattern, with its emphasis on clarity and focus, serves as a valuable tool for communication and collaboration between development and testing teams. By adopting this pattern, teams can ensure that software is thoroughly tested, issues are quickly identified and addressed, and the final product is of the highest quality. However, it's important to remember that the AAA pattern is not a silver bullet, and its effectiveness depends on the team's ability to adapt and apply it correctly
Conclusion
The AAA pattern, with its systematic approach to structuring unit tests, offers numerous benefits in software development. It enhances the efficiency and effectiveness of testing, promoting clarity, maintainability, and reliability in unit tests. By dividing the testing process into three distinct phases - Arrange, Act, and Assert - the AAA pattern provides a clear framework for developers to follow when writing tests.
The main idea presented in the article is that by understanding and implementing the AAA pattern, software engineers can optimize their testing practices and deliver higher-quality software products. The AAA pattern helps manage technical debt, adapt to changing requirements, balance workload management, and bridge the gap between development and testing teams. It promotes clear communication and collaboration between teams, improves the efficiency of unit testing, and ensures that software is thoroughly tested.
The broader significance of the ideas discussed in the article is that by using the AAA pattern, developers can overcome common challenges in software development such as managing technical debt and dealing with changing requirements. The AAA pattern provides a structured approach to unit testing that enhances the effectiveness of testing practices. By following this pattern, developers can improve their productivity and deliver high-quality software products.
Boost your productivity with Machinet. Experience the power of AI-assisted coding and automated unit test generation. Start optimizing your testing practices today
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.