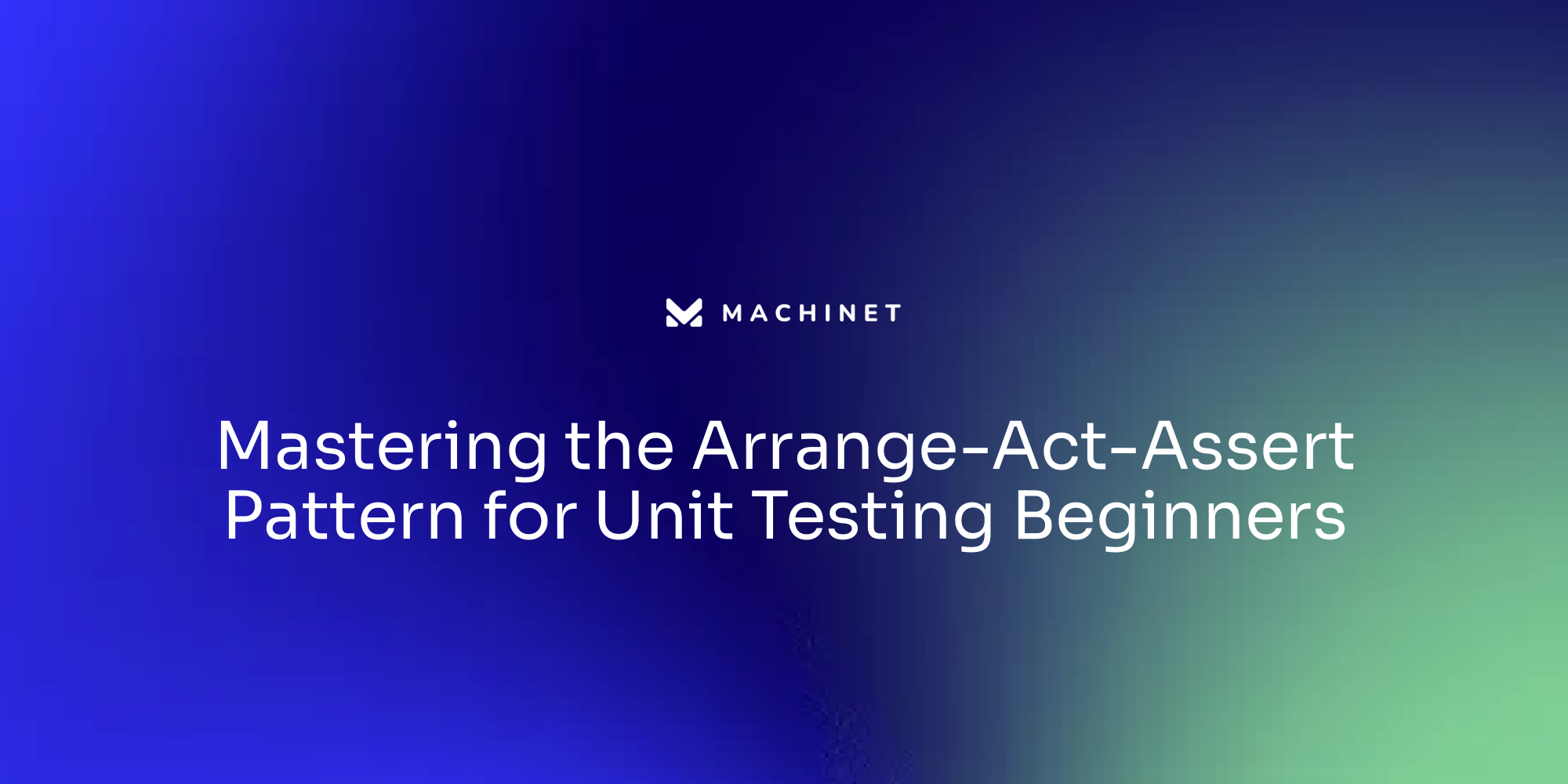
Table of Contents
- Understanding the Basics of Unit Testing
- Deep Dive into the Arrange-Act-Assert (AAA) Pattern
- Implementing the AAA Pattern in Unit Testing: A Step-by-step Guide
- Best Practices for Using the AAA Pattern in Unit Tests
- Real-world Example: Applying the AAA Pattern in a Unit Test Project
- Addressing Common Challenges in Using the AAA Pattern for Unit Testing
- Tips and Techniques to Optimize Unit Test Efforts Using AAA Pattern
Introduction
Unit testing is a crucial aspect of software development, providing a systematic approach to verifying the correctness of individual components within a program. The Arrange-Act-Assert (AAA) pattern serves as a cornerstone in structuring unit tests, offering a clear methodology for setting up test conditions, executing functionality, and validating outcomes. By following best practices and leveraging tools like mocking frameworks and code coverage tools, developers can optimize their unit test efforts and ensure the reliability and quality of their code. In this article, we will delve into the basics of unit testing, explore the AAA pattern in detail, address common challenges faced during unit testing, and provide tips and techniques to enhance the effectiveness of unit tests using the AAA pattern. Whether you are new to unit testing or looking to optimize your testing process, this article will provide valuable insights and guidance to improve your unit testing practices
1. Understanding the Basics of Unit Testing
Unit testing serves as the foundation of software development
Try Machinet to automate unit test generation and save time!
, facilitating the verification of individual components within a program. This process involves crafting unique pieces of code to examine the behavior and output of specific functions or methods in isolation, therefore confirming their correctness. Automated unit tests, a common practice in contemporary development environments, play a crucial role in early detection of regression errors or bugs.
Unit tests are instrumental in uncovering potential issues during code refactoring, bug fixing, or the integration of new features. They should be executed rapidly, within seconds rather than minutes, to maintain efficiency in the development process. The independence of unit tests is an important aspect - each test should not share state with others, and their execution should not depend on external systems such as databases or file systems.
Unit test results should be self-validating, producing a boolean value that signifies the test result as pass or fail. This process eliminates the necessity for developers to trawl through logs to determine the outcome of the tests. As the application code evolves, so should the unit test suite, reflecting the changes and additions made to the codebase.
The timely creation of unit tests is crucial
Sign up for Machinet and generate unit tests effortlessly!
- they should not be an afterthought or hastily composed just prior to launching into production. Non-determinism, a condition that can lead to inconsistent test results, should be avoided in unit tests. This necessitates meticulous examination of the unit test code and logic to identify and eliminate any sources of non-deterministic behavior.
Unit tests not only aid in regression but also function as executable documentation
Improve code quality with Machinet's context-aware AI chat and unit test generation!
, clearly demonstrating the expected output for a given input. They also contribute to good design by assisting in decoupling the code, making it more testable and easier to maintain.
Understanding the distinction between "mock" and "stub" in the context of testing is crucial. A mock object is a fake object used to determine whether a test has passed or failed, while a stub serves as a controllable replacement for an existing dependency.
When crafting unit tests, developers should follow certain best practices: write tests that are focused and cover a specific piece of functionality or behavior, ensure that tests are independent and do not rely on the state of other tests, use meaningful and descriptive test names, incorporate test-driven development (TDD) principles where tests are written before the implementation code, include both positive and negative test cases, mock or stub external dependencies to isolate the code being tested, regularly re-run tests to catch any regressions or breaking changes, write tests that are easy to understand and maintain with clear assertions and minimal test setup.
While code coverage is an essential aspect of unit testing, it should not be the sole indicator of code quality. Setting ambitious coverage goals can sometimes be counterproductive and lead to tests that are more focused on achieving coverage than verifying functionality. The unit test suite should evolve alongside the application code, reflecting changes and updates over time.
Finally, unit testing is an invaluable tool for software developers, assisting in catching regression issues, providing executable documentation, and facilitating good design. By adhering to best practices, developers can create a robust and efficient unit test suite that ensures the quality and integrity of their code
2. Deep Dive into the Arrange-Act-Assert (AAA) Pattern
The Arrange-Act-Assert (AAA) pattern, a cornerstone of unit testing, provides a systematic methodology for structuring tests, enhancing their comprehensibility and updatability. This pattern is particularly beneficial for those new to unit testing. The AAA pattern is divided into three distinct phases: Arrange, Act, and Assert.
During the 'Arrange' phase, the conditions for the test are established, setting the stage for the testing process. The 'Act' phase involves executing the functionality under test, while the 'Assert' phase verifies the outcome to see if it aligns with the expected results.
Testing multiple failure conditions and exceptions, in addition to the expected working condition, is crucial when writing unit tests for a method. Custom domain exceptions should also be tested to ensure proper behavior. The role of guard clauses in maintaining clean methods and ensuring input adherence to method expectations cannot be underscored.
The xUnit framework provides an Assert.Throws
method to test for expected exceptions. Alongside this, the use of an action can aid in separating the act step from assertions in tests, thereby enhancing their readability. FluentAssertions serve as an alternative to built-in assertions for testing libraries. Local functions can be used instead of actions to represent the operation expected to throw an exception.
Steve, an experienced software architect and trainer, advocates for the action approach for testing exceptions. Ardalis, another seasoned software architect, has recently discussed topics such as scaling software teams and process bloat. He also emphasizes the robust support that the xUnit test framework provides for testing exceptions and how using actions can help make tests cleaner.
In real-world scenarios, developers often utilize xUnit and actions to test for expected exceptions in their code. The Assert.Throws<T>
method provided by xUnit is commonly used to test for expected exceptions. Alternatives like FluentAssertions and local functions are also employed to test for expected exceptions in their code. By adopting these approaches, developers can enhance code quality and ensure the successful delivery of software products.
The AAA pattern helps to structure and organize your unit tests, making them more readable and maintainable. Its implementation can be achieved by using testing frameworks like JUnit or TestNG for Java, which provide annotations and assertions that can be used to clearly separate the different stages of the test. In the Arrange stage, necessary dependencies are set up, test objects are created, and the test environment is prepared. This usually involves creating mock objects or stubs, initializing variables, and configuring required dependencies. In the Act stage, the method or code under test is invoked, passing the necessary inputs or triggering the desired behavior. Finally, in the Assert stage, the expected outcomes or behaviors of the code under test are verified. This involves making assertions to ensure that the result of the action matches expectations. Various assertion methods provided by the testing framework can be used to check for specific conditions or values. By following the AAA pattern, unit tests become more organized, easier to understand, and more maintainable. It helps to clearly separate the different stages of the test, making it easier to identify any issues or failures. Overall, the implementation of the AAA pattern in unit tests leads to more reliable and effective testing practices
3. Implementing the AAA Pattern in Unit Testing: A Step-by-step Guide
As a proficient software engineer, understanding the Arrange-Act-Assert (AAA) pattern's application in unit testing is critical to developing robust software solutions. The AAA pattern is a structured approach that guides the testing process through three distinct stages.
The first stage, Arrange, sets the groundwork for the test. This involves preparing the required objects, inputs and expected outcomes. Activities in this stage might include the creation of mock objects, the formation of test data, or configuring the system for the test.
The second stage, Act, involves executing the function or method that you're testing. This could mean calling a method with specific parameters or triggering a specific event.
Lastly, the Assert stage is where you validate that the actual outcomes align with the expected results. This verification might involve checking a function's return value, evaluating an object's state, or confirming that a specific event has occurred.
While the AAA pattern provides a systematic approach to unit testing, other testing frameworks like Unreal's Gauntlet and functional tests supplement this process by offering additional tools for testing gameplay code. Gauntlet, for instance, is designed for gameplay testing and is capable of running on full builds. Functional tests, on the other hand, can run on any platform. These frameworks use latent functions, which wait for certain conditions to be met before proceeding. This feature can be especially valuable when testing larger sections of gameplay code.
Furthermore, principles of writing maintainable software, such as the concept of 'page objects' from the Android testing course by Protech, should also be applied to automated test code. Page objects are an abstraction layer that defines an interface for triggering actions and obtaining results. They can significantly improve the readability and maintainability of test code by facilitating code reuse and a clear separation of actions.
As such, the effective utilization of the AAA pattern, supported by strategic use of testing frameworks and principles of maintainable software, can significantly enhance the efficiency and maintainability of unit tests. To implement the AAA pattern effectively in your unit testing, it's advisable to refer to reliable sources for general unit testing best practices and documentation
4. Best Practices for Using the AAA Pattern in Unit Tests
Venturing into the realm of unit testing, the Arrange-Act-Assert (AAA) pattern has the potential to significantly enhance the quality of your test cases. Harnessing the full potential of this pattern requires adherence to several best practices.
One fundamental principle is to keep your tests concise and focused. Each test should be designed to verify a single behavior or outcome. This approach not only simplifies understanding and maintaining your tests, but it also aids in locating the source of a failure when a test does not pass.
Another important practice is to eliminate dependencies between tests. Every test should be a self-contained entity that can be executed independently of others. This ensures that the order in which tests are run does not influence their outcome and facilitates the isolation and resolution of issues when they emerge.
Moreover, the naming of your tests is critical. Consistent naming conventions improve the maintainability of your tests and facilitate understanding of their purpose. Unit tests are often named using a combination of the method or class being tested and the specific scenario being evaluated. A format such as "testMethodName_ExpectedBehavior" or "testClassName_MethodName_ExpectedOutcome" can be employed. By including the expected behavior or outcome in the test name, the purpose of each test case can be easily identified. Using descriptive names that clearly indicate the functionality being tested further enhances understandability. Following such naming conventions, developers can improve the overall readability and maintainability of their codebase.
The DRY (Don't Repeat Yourself) principle is another crucial practice in unit testing. If a pattern of duplicating setup or assertion code across multiple tests is observed, consider refactoring it into a shared method or class. This can not only reduce redundancy but also enhance the readability and maintainability of your tests.
Testing failure conditions, not just the expected working condition, is equally important. For instance, if a method throws exceptions under certain conditions, these exceptions should be tested as well. Tools like xUnit have built-in methods like Assert.Throws
which can be used to test for expected exceptions.
Utilizing actions to represent operations that are expected to throw exceptions can help you adhere to the AAA test pattern and make your test code cleaner. Considering the use of local functions as an alternative to actions for representing these operations could be beneficial.
Ultimately, effective application of the AAA pattern in unit testing demands a thoughtful approach. By adhering to these best practices and continually seeking ways to improve your test code, you can ensure that your unit tests are robust, maintainable, and valuable
5. Real-world Example: Applying the AAA Pattern in a Unit Test Project
Unit testing, a fundamental discipline in software development, relies heavily on the Arrange-Act-Assert (AAA) pattern. This pattern provides a systematic approach that increases clarity and coherence in the process. Let's delve into its application using a practical example of unit-testing an iOS MVP (Model-View-Presenter) project using Swift, through a project named "The Dragon Rides App".
The AAA pattern initiates with the Arrange phase, where the prerequisites for the test are set up. In our case, this could involve generating a new instance of a class, such as a Calculator, and outlining the test inputs. Supplementary elements, such as mock objects, are created to interact with the system under test. For example, a mock flight service or a mock list flights view controller might be created.
The Act phase follows, involving the execution of the operation that is being tested. In our example, this could be invoking the Add method with the defined inputs or setting up unit tests for the ListFlightsPresenter using the generated mock objects.
The final stage is the Assert phase, where the outcome of the test is validated. This could involve verifying that the result of the Add method aligns with the expected outcome or confirming that the interaction between the presenter and view controller is as anticipated. This phase may also involve testing failing scenarios, such as a service request failure, to ensure that the system responds appropriately under adverse conditions.
Following the AAA pattern, the unit testing process becomes more streamlined and efficient. It aids in identifying potential problems and contributes to code refactoring and improved extensibility. Upon completion, the test suite is ready to be run, offering a tangible measure of the software's quality and functionality.
In summary, the effective application of the AAA pattern can significantly improve the readability, maintainability, and overall effectiveness of unit tests, ultimately leading to the delivery of high-quality software products
6. Addressing Common Challenges in Using the AAA Pattern for Unit Testing
Unit testing, particularly with the Arrange-Act-Assert (AAA) pattern, can become complex, especially when dealing with intricate setup code or asynchronous functions. The Arrange phase, which sets up the necessary conditions for testing, can become convoluted when the test necessitates extensive setup. However, there are strategies to manage this complexity.
The first strategy involves breaking down the setup into smaller, more manageable parts. This can be done by deconstructing the setup code into distinct methods or classes. For instance, Doctolib, a healthcare software solutions provider, releases new versions of their native software weekly. Testing these installers and executables require complete installation, functionality validation, and verification of files and registry entries across various operating systems and architectures. To manage this, Doctolib developed tools and protocols to automate end-to-end testing of installers and executables on their Continuous Integration (CI) system. They set up a stateless environment for testing to ensure isolated and consistent results. This demonstrates the importance of refactoring setup code into separate methods or classes.
The second strategy is to use test fixtures, reusable objects that serve as a baseline for running tests. Test fixtures simplify the setup code by providing a predefined state for the unit under test. Using setup methods or fixtures to initialize common test data ensures that the setup process is repeatable and doesn't have any side effects on other tests.
The third strategy is to use mocking frameworks, such as Mockito for Java unit testing. Mockito creates mock objects that simulate the behavior of dependencies, allowing you to isolate the unit under test and focus on testing its specific behavior. This eliminates the need for setting up complex arrangements of dependencies.
Asynchronous code presents another common challenge in unit testing. If the function you are testing operates asynchronously, the test needs to pause and wait for the function to finish before moving on to the assertion phase. This can be accomplished through techniques like async/await or other asynchronous testing methods. In the context of Doctolib, they utilize Jest for snapshot testing to verify that files, registry entries, and config files match the expected values. The binary architecture is also tested to ensure compatibility with the target system. This highlights the need for asynchronous testing techniques, particularly when dealing with complex software systems.
While the AAA pattern offers a structured approach to unit testing, it's essential to be aware of and prepared for the potential challenges that may arise, particularly when dealing with complex setup code and asynchronous functions. By implementing strategies such as refactoring setup code, using test fixtures and mocking frameworks, and employing asynchronous testing techniques, these challenges can be effectively managed, leading to more efficient and reliable unit tests
7. Tips and Techniques to Optimize Unit Test Efforts Using AAA Pattern
The AAA (Arrange, Act, Assert) pattern is a widely recognized and effective approach to structuring unit tests. The application of several strategies can enhance the implementation of the AAA pattern in your testing process.
Firstly, it is crucial to select a unit testing framework that aligns with the AAA pattern. A prime example is the xUnit testing framework, which offers built-in mechanisms that facilitate the arranging, acting, and asserting in your tests. This not only simplifies the process of drafting tests but also makes their maintenance easier.
Secondly, the incorporation of mocking or stubbing methodologies to isolate the functionality under test can significantly reduce the complexity of your setup code, thereby enhancing the reliability of your tests. A tool like the JustMock framework by Telerik can facilitate this process. It provides methods such as mockArrange
and mockAssert
for setting up and validating the behavior of mock objects, which enables developers to test code without modifying the original codebase.
The third strategy is to utilize a code coverage tool to ensure the comprehensiveness of your tests. Such tools measure the extent to which your program's source code has been tested, offering insights into which parts of the code have been executed during testing and which parts have not. This is particularly useful in identifying gaps in your testing, ensuring every line of code is tested, a critical aspect of managing technical debt and legacy code.
In addition to these strategies, the importance of appropriately naming your unit tests cannot be understated. While common naming practices may have their limitations, alternative guidelines can aid in creating readable and meaningful test names, thereby improving the overall maintainability of your tests.
Lastly, these strategies are not confined to any specific programming language, making them universally applicable to unit testing. By understanding and implementing these techniques, developers can enhance the readability, maintainability, and effectiveness of their unit tests, ultimately leading to the delivery of high-quality software products
Conclusion
In conclusion, the article emphasizes the importance of unit testing in software development and introduces the Arrange-Act-Assert (AAA) pattern as a structured approach to writing effective unit tests. The AAA pattern provides clear guidelines for setting up test conditions, executing functionality, and validating outcomes. It promotes best practices such as writing focused tests, ensuring test independence, using meaningful test names, and incorporating test-driven development principles. The article also highlights the significance of code coverage and the role of mocking frameworks in isolating dependencies during testing. By following these techniques and leveraging tools like xUnit and JustMock, developers can create robust and maintainable unit test suites that enhance the reliability and quality of their code.
The broader significance of implementing the AAA pattern in unit testing lies in its ability to improve software development practices. Unit tests not only catch regression issues but also serve as executable documentation and contribute to good design by decoupling code and making it more testable. By adopting the AAA pattern and adhering to best practices, developers can optimize their unit testing efforts, leading to higher code quality, increased productivity, and improved software reliability. To boost your productivity with Machinet, experience the power of AI-assisted coding and automated unit test generation.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.