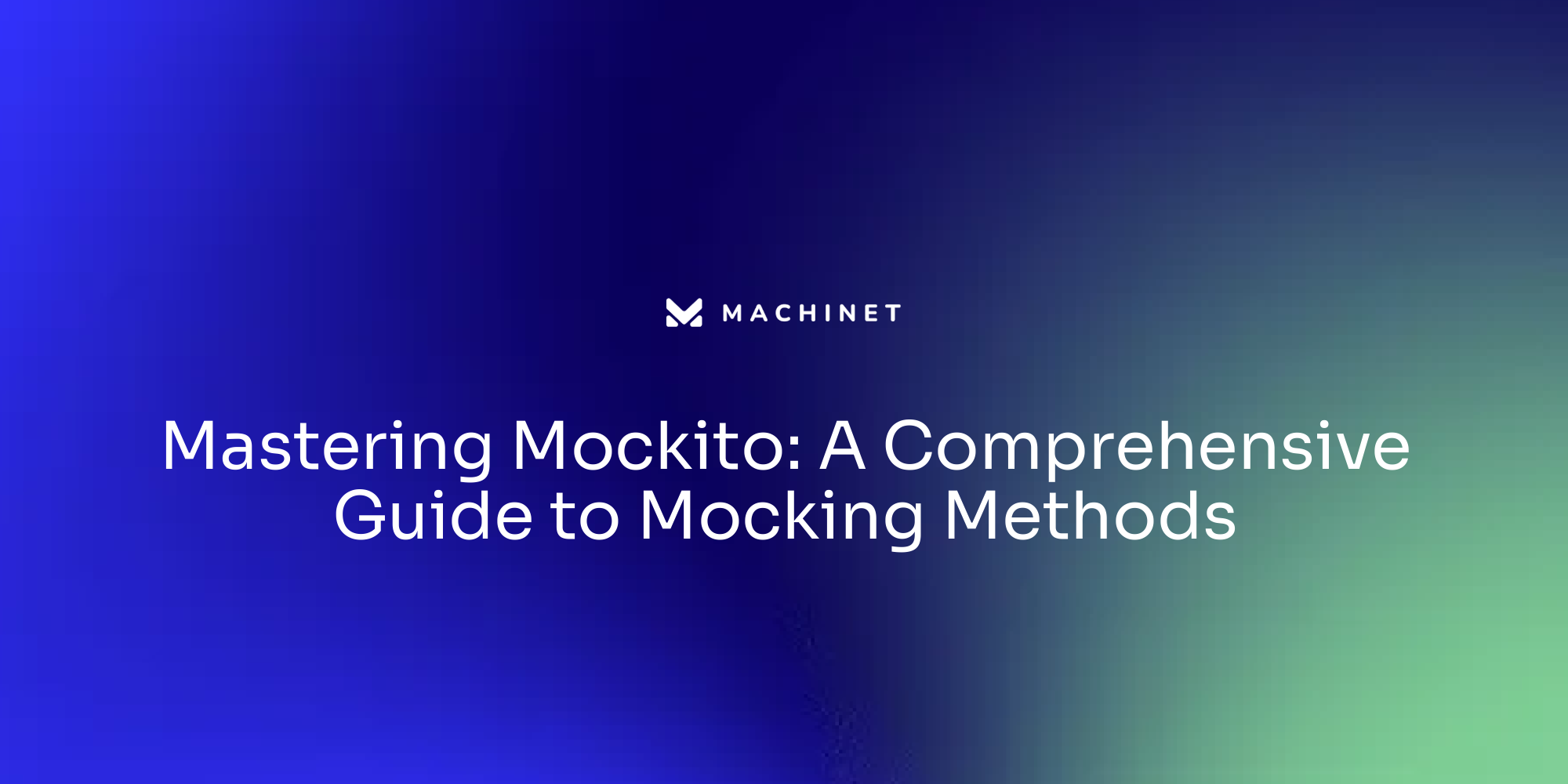
Table of contents
- Understanding the Basics of Mockito
- Exploring Mockito's Mock Methods
- Practical Tutorial: Using Mockito to Mock Some Methods but Not Others
- Advanced Techniques: Mocking a Class Method Inside Another Class with Mockito
- Strategies for Effective Unit Testing with Mockito
- Addressing Common Challenges in Mockito Usage
- Best Practices for Optimizing Your Unit Tests with Mockito
Introduction
Mockito is a powerful Java-based framework that simplifies the process of creating mock objects for unit testing. It enables developers to focus on the behavior of the system under test by providing a way to create 'mock' versions of external dependencies. This isolation of the class under test ensures that test outcomes are solely based on its behavior.
In this article, we will explore the basics of Mockito and its capabilities in unit testing. We will discuss how to use Mockito to create mock objects, define their behavior, and verify method calls. We will also delve into advanced techniques such as mocking static methods and constructors, partial mocking using spies, and mocking methods in different classes. Additionally, we will discuss best practices for optimizing unit tests with Mockito and address common challenges that developers may encounter. By understanding and applying these concepts, developers can enhance the effectiveness and reliability of their unit tests using Mockito
1. Understanding the Basics of Mockito
Mockito is a powerful Java-based framework that simplifies the process of creating mock objects for unit testing.
Supercharge your unit testing with Mockito and improve the quality of your code!
It enables developers to focus on the behavior of the system under test by providing a way to create 'mock' versions of external dependencies. This isolation of the class under test ensures that test outcomes are solely based on its behavior.
Mocking, as a technique, allows a class's functionality to be tested in isolation, without the need for a database connection or file reading. Mockito excels in this area by making it easy to create mock objects in Java using reflection. This eliminates the need to write mock objects manually, ensures refactoring safety, supports return values and exceptions, checks the order of method calls, and provides support for annotations.
Imagine a scenario where Mockito is used to create a mock stock service to test a portfolio object. The portfolio object contains a list of stocks and calculates the market value based on the stock prices. The mock stock service provides dummy values for the stock prices, and the portfolio's market value is checked against a specific value.
Mockito goes beyond just mocking objects in software tests using Java and JUnit 5. It provides dummy implementations for interfaces or classes, allowing developers to define the output of certain method calls. Mockito simplifies the development of tests for classes with external dependencies. It's capable of mocking static methods and final classes, but private methods remain invisible for tests and cannot be mocked.
Mockito records interactions with mock objects, letting developers verify whether certain methods have been called on the mocks. It can be incorporated into a project using Maven or Gradley. Mockito provides several methods for creating mock objects, including using annotations and the Mockito API. The return values of method calls on mock objects can be configured using the Mockito API, including using when-thenReturn and doReturn-when.
Mockito provides the capability to wrap real objects with spy and verify the calls on the mock objects. It supports dependency injection via the @InjectMocks annotation and provides the ArgumentCaptor class to capture the arguments of method calls during verification. It supports complex mocks using the Answers interface. The Strict Stubs rule in Mockito aids in maintaining clean test code and checks for common oversights. Mockito can be used to mock final classes and static methods.
Mockito offers the ability to use spies and reflection to modify private fields and call private methods. It provides resources such as the official website, a DZone reference card, and release notes. Mockito offers online training, onsite training, and consulting services.
To use Mockito in Java unit testing, it's important to have the Mockito library added to your project's dependencies. This can be done by adding the appropriate dependency declaration to your build file. The necessary classes from the Mockito library are then imported into your test class. This typically includes the Mockito
class itself and any other classes required for mocking and verification. A mock object for the class or interface to be tested is created using the Mockito.mock()
method.
Setting up the necessary expectations and behaviors for your mock object is done using Mockito's methods. These include specifying what methods should return, throwing exceptions when certain methods are called, or verifying that certain methods were called with specific arguments. For instance, to specify what a method should return, use the Mockito.when()
method. To throw an exception when a method is called, use the Mockito.doThrow()
method. To verify that a method was called with specific arguments, use the Mockito.verify()
method.
Writing your test cases using the mock object you created allows you to control the behavior of the dependencies of the class you are testing. Running your unit tests and verifying that the expected interactions and behaviors are occurring as defined by your mock objects is the final step.
When using Mockito in unit testing, several best practices should be kept in mind. Utilising Mockito's mocking capabilities to create mock objects for any dependencies that your code relies on is key. This allows you to isolate the code you are testing and focus on its behavior without worrying about the behavior of external dependencies. Using Mockito's verification features to verify that certain interactions with the mock objects occurred during the test is also a good practice. This can help ensure that the code being tested is behaving as expected and interacting correctly with its dependencies
2. Exploring Mockito's Mock Methods
As an experienced software engineer developing advanced software solutions, one of the key tools in your arsenal should be the Mockito framework. Mockito's mock
function offers a foundation of functionality, often employed by developers to create mock instances of specified classes or interfaces. These mock objects can substitute actual entities in the system under test, a feature that becomes invaluable when dealing with complex objects that are challenging to instantiate or have associated side-effects.
For instance, consider a login function in an e-commerce application. In this hypothetical application, a method is designed to save customer data into a session following a successful login. The application's structure includes a mock data access object (DAO) and a service class that leverages the DAO for the login process. The service class also contains a method to save customer data in the session. Mockito can be used to mock this method, enabling you to control its behavior during unit testing.
To do this, first, create a mock object of the class:javaClassName mockObject = Mockito.mock(ClassName.class);
Then, use Mockito's when()
method to specify the behavior of the method when it is called:javaMockito.when(mockObject.methodName(arguments)).thenReturn(returnValue);
Finally, call the method and verify its behavior using verify()
:javamockObject.methodName(arguments);Mockito.verify(mockObject).methodName(arguments);
This way, you can simulate the method's behavior without executing its implementation, allowing you to test it in isolation.
Furthermore, Mockito's mockStatic
method is a useful tool for mocking static methods or constructors, which is occasionally necessary when writing tests. This feature offers a convenient alternative to introducing layers of indirection. The original unmocked behavior can be restored using thenReturn
. Mocking static methods proves beneficial when dealing with hardcoded file paths or performing calculations based on Java time values. In the case of hardcoded file paths, indirection can be introduced with system properties or by converting static methods to instance methods. For calculations based on Java time values, static mocking can provide a fixed constant value for simpler testing. Mocking static methods can also be applied to classes like java.management.Factory
for testing purposes. Mockito's mockConstruction
method can be used to mock constructors, and custom behavior can be applied to the mocked instance using a callback method. However, it is essential to use static and constructor mocking responsibly and judiciously.
In conclusion, Mockito provides a flexible syntax for mocking methods in Java unit testing, allowing developers to simulate different scenarios and verify the behavior of their code. Whether you're dealing with a simple function or a complex object, Mockito's functionality can be invaluable in ensuring your code behaves as expected under a variety of conditions
3. Practical Tutorial: Using Mockito to Mock Some Methods but Not Others
Unit testing often involves scenarios where it's advantageous to mock certain methods of an object while allowing others to function as usual. Mockito, a prevalent mocking framework in the software testing landscape, provides a solution for such situations with its spy
method.
A spy in Mockito is a form of partial mock. It wraps a real object, but with an added control layer that enables you to dictate its behavior as if it were a complete mock. This characteristic allows you to stub a specific method to return a particular value, while other methods continue to execute as usual.
Let's illustrate this concept with a practical example. Suppose an ecommerce application stores customer information in the session after a successful login operation. We can create a spy on the data access object (DAO), focusing on testing the login service. We might want to verify if a method, such as saveInSession
, is called when a customer logs in successfully. This is where the spy proves its worth, allowing us to mock the saveInSession
method and verify its invocation with any customer object using Mockito's verify
method.
```java// Create a spy objectYourClass yourClass = spy(new YourClass());
// Use the spy object just like you would use a regular object. You can stub methods on the spy object using the when
method:
when(yourClass.methodToStub()).thenReturn(value);```Spies are especially useful when testing methods that interact with external systems or produce side effects. By mocking these methods, we can isolate the behavior we want to test, making our tests more reliable and predictable.
Mockito offers a variety of tools besides a spy. It provides several other methods to create mock objects, configure their return values, and verify interactions with them. It also supports mocking static methods, final classes, and features like the @InjectMocks
annotation for dependency injection of mock objects and the ArgumentCaptor class for capturing the arguments of method calls. However, Mockito encourages caution when mocking final classes and static methods, often suggesting code refactoring as a better approach.
Mockito's spy
method is an effective way to create partial mocks, offering the flexibility to mock some methods while leaving others untouched. This feature can be invaluable in complex testing scenarios. Remember, the ultimate goal is to write tests that are reliable, maintainable, and meaningful. Mockito, with its rich feature set, can be a powerful ally in achieving this goal
4. Advanced Techniques: Mocking a Class Method Inside Another Class with Mockito
Mockito, a renowned open-source testing framework, presents the ability to mimic the functionality of a method within a different class. This is particularly handy when there's a need to isolate a class's behavior from its dependencies. Consider, for instance, a scenario where a class A
invokes a method from class B
. Mockito allows you to replace the method in class B
with a mock version that returns a predefined value when called by class A
. This enables the independent testing of class A
's behavior, regardless of class B
's behavior.
The steps to mock a method in a different class using Mockito are as follows:
- First, create an instance of the class containing the method you need to mock.
- Use the
mock()
method provided by Mockito to create a mock object of the class. - The
when()
method from Mockito is then used to specify the method you want to mock and what it should return when invoked. - Call the method on the mock object in your test code.
- Finally, employ assertions or verifications to check the behavior of the method.
This process allows you to effectively mock a method in another class using Mockito. It's a prime example of how Mockito amplifies the efficiency and effectiveness of unit testing in software development. By isolating the behavior of classes, Mockito simplifies the identification of issues, comprehension of functionality, and enhancement of your code quality.
Here's an example of how you can use Mockito to mock a method in a different class:
```java// Create a mock object of the desired classSomeClass someObject = Mockito.mock(SomeClass.class);
// Define the behavior of the mocked methodMockito.when(someObject.someMethod()).thenReturn(someValue);
// Call the method on the mock objectsomeObject.someMethod();
// Verify that the method was calledMockito.verify(someObject).someMethod();```
By using Mockito, you can easily control the behavior of the mocked method during unit testing. This is a crucial aspect of managing technical debt and ensuring the delivery of high-quality software solutions
5. Strategies for Effective Unit Testing with Mockito
Unit testing with Mockito goes beyond the mere creation of mock objects and spies; it's about understanding their application and significance. Mockito, a popular Java mocking framework, is commonly used for unit testing. It allows developers to create mock objects, which mimic the behavior of real objects, to test the functionality of their code.
Take for instance, an ecommerce application where customer information is stored in the session after a successful login. In unit tests, you may not have direct access to certain objects like HttpSession, hence the need to verify that the method for saving customer data in the session was called. This can be achieved by creating a mock data access object (DAO) and a service that uses the DAO to perform the login and save customer data in the session.
In this scenario, a spy is used instead of a mock in the test code. The 'verify' method is critical for checking if the 'saveInSession' method was called. This method requires the spy or mock object as a parameter and in this example, it checks if the 'saveInSession' method was called with any customer object.
Mockito is a powerful tool for unit testing, but the effectiveness of your tests depends on your understanding of the code you're testing and the behavior you're trying to verify. It is crucial to properly set up the mock or spy object, define the behavior using Mockito's when-then syntax, and verify the interactions using Mockitoβs verification capabilities. You can check if certain methods were called, how many times they were called, and with what arguments.
While Mockito serves as a robust solution, alternative tools such as WireMock can be used for HTTP APIs. WireMock provides flexibility and can be extended to handle delayed callbacks for asynchronous APIs. Furthermore, tools like Code With Me, developed by JetBrains, facilitate collaborative development and pair programming in real time, adding another dimension to your testing environment.
In terms of tracing asynchronous code, New Relic presents a solution. This tool uses an implementation of java.util.concurrent.Executor
that wraps a delegate instance and calls the New Relic trace methods before executing the delegate's runnable.
Lastly, consider Octopus Deploy for deploying to on-premises environments with polling tentacles. This tool secures connections using self-signed certificates and can be configured to accept connections from polling tentacles when deployed in AWS.
Remember, the power of Mockito lies not just in its use, but in understanding and applying it appropriately in your unit tests. It's also important to avoid common mistakes such as not properly setting up the mock or spy objects, not verifying the interactions, overusing mocks and spies, and using mocks and spies as a substitute for proper integration testing. By following these best practices, you can ensure that your unit tests with Mockito are effective and reliable
6. Addressing Common Challenges in Mockito Usage
As a Senior Software Engineer, you may encounter challenges when implementing unit testing with Mockito. These hurdles can range from managing an overuse of mocks, ensuring accurate mimicry of objects by the mocks, to maintaining the robustness of your tests. However, with a proper understanding and use of Mockito's capabilities, these challenges can be effectively managed.
When using Mockito, it's essential to recognize the importance of not overusing mocks in your tests. Overuse can lead to complex test setups and a reduction in test clarity. To manage this, consider only mocking dependencies directly relevant to the behavior being tested. This helps keep the tests focused and reduces the complexity of the test setup. Favor using real objects whenever possible, as they provide a more accurate representation of how the system behaves. You can also use test doubles, which are simplified versions of real dependencies that only implement the necessary behavior for the test.
When setting up your mocks, use Mockito's when()
method to define the desired behavior of the mock objects. This includes specifying the return values of methods, defining the order of method invocations, and verifying that certain methods are called with specific arguments. Mockito's verify()
method is particularly useful for this purpose. Using the verify()
method allows you to check if certain methods were called on the mocks during the execution of your unit tests, ensuring the code under test is interacting correctly with the dependencies.
For testing static methods and constructors, Mockito provides the mockStatic
and mockConstruction
methods respectively. These methods allow you to modify the behavior of static methods and constructors within a try scope using thenReturn
. However, remember to use static and constructor mocking judiciously and responsibly.
A key aspect of maintaining the robustness of your tests is to avoid brittleness. This can be achieved by using the verify()
method with specific verification modes, allowing you to verify that certain methods were called on your mock objects, without specifying the exact order in which the calls occurred. Use argument matchers to make your verifications more flexible and less prone to breaking when the implementation details change.
In terms of real-world applications, Mockito's capabilities can be beneficial in various scenarios. For example, you might need to test code that uses the paths.get
static method from java.nio.Path
with hardwired file paths, or the Instant.now
static method from java.time.Instant
for calculations based on the current time. In such cases, mocking these methods would be useful.
Overall, Mockito encourages developers to write effective tests by adhering to principles such as keeping the test code concise and readable, avoiding coding tautologies, and achieving as much coverage as possible. By understanding and implementing these practices, you can improve the quality of your unit tests and overcome the challenges associated with Mockito
7. Best Practices for Optimizing Your Unit Tests with Mockito
As a seasoned software engineer, utilizing Mockito to its fullest potential is a strategic step in creating more robust, reliable, and maintainable Java unit tests. This involves adherence to certain best practices, which include defining the behavior of the dependencies you are mocking clearly. This helps in creating focused and effective unit tests. Furthermore, Mockito's verification capabilities should be utilized to ensure that your code interacts correctly with the dependencies.
The Mockito when-then
syntax is a powerful tool that allows you to stub the behavior of the mocked dependencies. This helps you simulate different scenarios and test different paths of execution in your code. It's recommended to use the @Mock
and @InjectMocks
annotations to simplify the creation and injection of mock objects.
While the goal is to keep your unit tests simple and focused on testing a single unit of functionality, Mockito's mocking capabilities can help create mock objects that simulate the behavior of real objects. This allows you to isolate the code being tested and focus on specific scenarios without having to deal with complex dependencies. By mocking dependencies, you can control their behavior and ensure that your tests are focused on the unit of code being tested.
In addition, Mockito's fluent API helps define the behavior of the mock objects and verify the interactions, making your tests more readable. Annotations such as @Mock
, @InjectMocks
, and @Spy
can simplify the setup of the test environment, allowing for easier creation and injection of mock objects into the test class.
It's important to remember to avoid unnecessary mocking in Mockito unit tests. Consider which dependencies need to be mocked and which can be left as real objects. Following the principle of "mock only what you own" will help avoid unnecessary mocking and keep your tests focused on the specific behavior being tested.
Another essential practice is to use meaningful names for your mock objects. This enhances the readability and maintainability of your test code. Descriptive names for your mock objects make it easier for other developers to understand the intent of the test and how the mock objects are being used.
To ensure the correctness of method calls, the Mockito verify
method is a powerful tool. It allows you to confirm that a specific method of a mock object has been called with the expected parameters. For example, you can use Mockito.verify(mockObject).doSomething("param1", "param2");
to verify that the doSomething
method was called with the parameters "param1"
and "param2"
. If the method was called with the wrong parameters or not called at all, the verify
method will throw an exception, and the test will fail.
However, Mockito's power doesn't replace the need for sound coding practices. Writing clean, testable code should always be your primary goal. Mockito's features, such as mocking dependencies and verifying method calls, can help you write tests that are more focused and isolated, leading to more robust and reliable code. Despite its limitations, such as the inability to mock final classes or enums, Mockito remains a powerful tool for unit testing in Java.
Avoid common mistakes when using Mockito, such as not properly setting up the test environment, overusing or misusing mocking, failing to verify the behavior of the code being tested, and neglecting to handle exceptions properly. These mistakes can lead to incorrect test results and make it harder to effectively test your code.
For those who wish to delve deeper into testing and development, adopting advanced techniques like mocking dependencies, stubbing method calls, verifying method calls, using argument matchers, and spying on real objects can significantly enhance the effectiveness of Mockito in your Java projects.
As a final note, remember to write small and focused tests that cover different scenarios and edge cases. This allows for easier debugging and maintenance of the tests, providing a clear understanding of the behavior of the code being tested. With these practices, your unit tests can be kept simple and focused with Mockito, leading to more reliable and maintainable code
Conclusion
In conclusion, Mockito is a powerful Java-based framework that simplifies the process of creating mock objects for unit testing. By creating 'mock' versions of external dependencies, developers can focus on the behavior of the system under test and ensure that test outcomes are solely based on its behavior. Mockito provides various capabilities such as mocking static methods and constructors, partial mocking using spies, and mocking methods in different classes. It also offers best practices for optimizing unit tests and addresses common challenges that developers may encounter.
The ideas discussed in this article have significant implications for software development. By using Mockito, developers can enhance the effectiveness and reliability of their unit tests. This leads to improved code quality, faster development cycles, and easier maintenance. Mockito's features enable developers to isolate code under test, simulate different scenarios, define desired behaviors of mock objects, and verify method calls. By understanding and applying these concepts, developers can boost their productivity and confidence in their code.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.