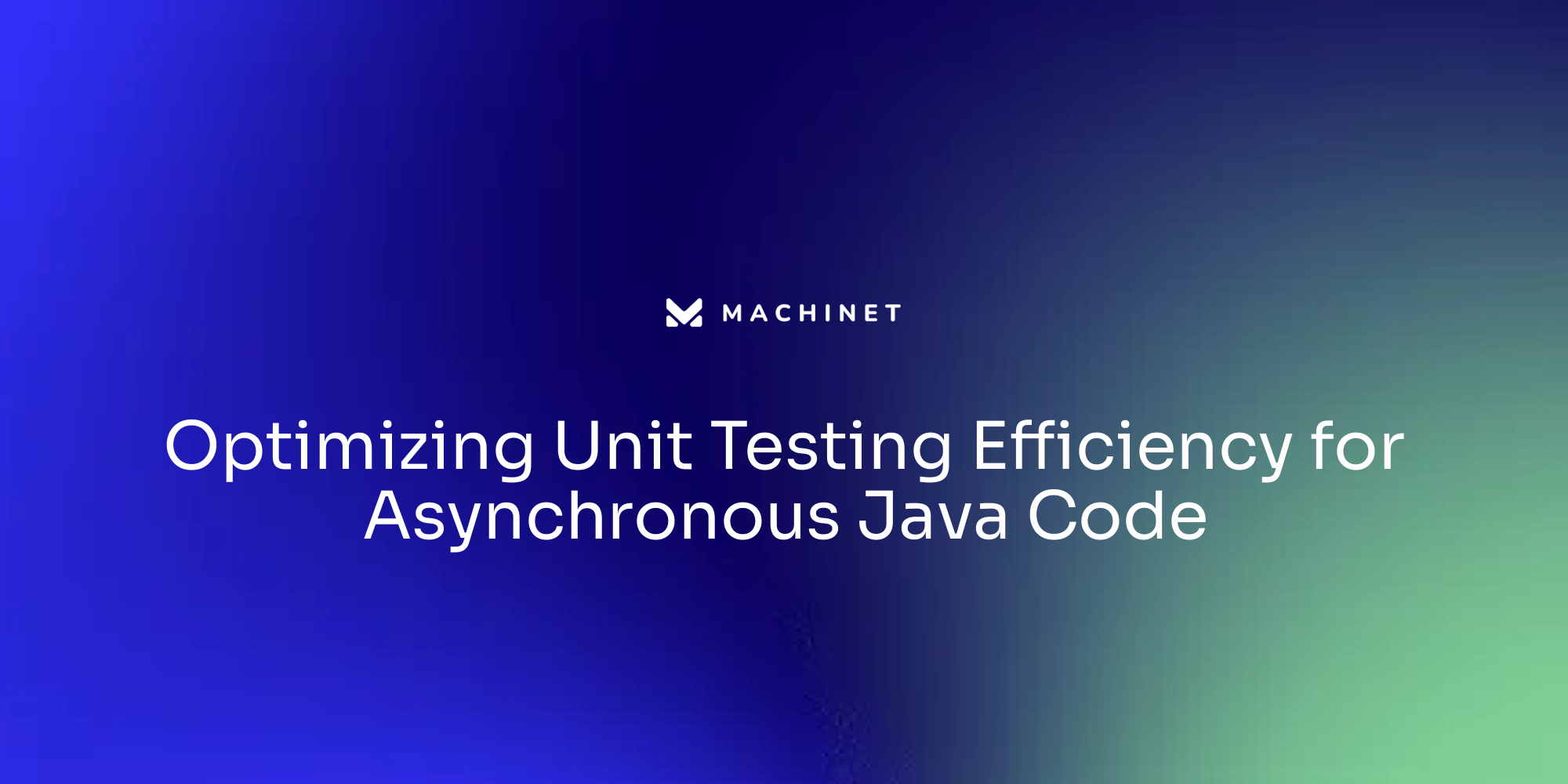
Table of Contents
- Understanding Asynchronous Processes in Java
- Testing Asynchronous Processes with JUnit: An Overview
- Best Practices for Unit Testing Asynchronous Java Code
- Strategies for Managing Multithreading in Unit Tests
- Effective Techniques for Unit Testing CompletableFuture in Java
- Overcoming Common Challenges in Unit Testing Asynchronous Java Code
- Tips and Tricks to Optimize Efficiency of Asynchronous Unit Tests
Introduction
Asynchronous programming in Java has become a fundamental aspect of modern software engineering, enhancing the efficacy and reactivity of applications. However, testing asynchronous code poses unique challenges, such as premature execution of assertions and managing timeouts. To overcome these challenges, developers can utilize techniques like mock listeners and deterministic schedulers. Additionally, frameworks like JUnit and Mockito provide support for unit testing asynchronous Java code, allowing developers to write reliable and efficient tests. In this article, we will explore the best practices and strategies for unit testing asynchronous Java code, including handling exceptions, managing multithreading, and optimizing the efficiency of tests. By implementing these techniques, developers can ensure the reliability and quality of their software while effectively managing the complexities of asynchronous programming in Java
1. Understanding Asynchronous Processes in Java
Java's proficiency in supporting concurrent execution of tasks through asynchronous operations is a fundamental facet of modern software engineering. These operations enhance the overall efficacy and reactivity of an application. Asynchronous programming in Java is facilitated by a variety of tools including threads, executors, and the CompletableFuture class. Mastery of these tools enables proficient unit testing of asynchronous Java code.
The challenge in testing asynchronous methods is primarily due to the potential of the assert statement executing before the task initiated by the asynchronous method completes. The solution to this challenge lies in the use of a mock listener. This mock listener waits for the task to finish before executing the assert statement. This can be illustrated using a search model interface that performs an asynchronous search and notifies a listener upon completion. The listener, in this case, is a mock listener class that waits for the completion of the search and verifies the results.
Unit testing bridges this gap by creating a mock listener, executing the search, waiting for the search to finish, and then verifying the results.
Try Machinet and automate your unit tests effortlessly.
A crucial part of this process is the implementation of a timeout period. This prevents the test from waiting indefinitely if the asynchronous method fails to notify the caller. For example, the code may call an asynchronous method that runs in its own thread. A mock listener is used to wait for the asynchronous method to finish. The search model interface and the search model listener interface are demonstrated in this context. The mock search model listener class and the unit test for the asynchronous search explain the use of synchronized blocks and wait/notifyAll. The importance of incorporating a timeout period in the unit test is emphasized here.
Moreover, it's crucial to handle exceptions efficiently when writing asynchronous Java code to maintain application stability and reliability. This can be achieved by using try-catch blocks to catch and handle any exceptions that may occur during the execution of asynchronous tasks. Additionally, the CompletableFuture's exceptionally() method can be used to handle exceptions in a more streamlined way. By chaining the exceptionally() method to a CompletableFuture, it allows you to specify a callback function that will be executed if an exception occurs during the CompletableFuture's execution. This helps in handling exceptions and performing necessary error handling or logging.
When writing asynchronous Java code, there are several common pitfalls that developers should be aware of to avoid potential issues. These pitfalls include race conditions, blocking operations, and improper error handling. Race conditions occur when multiple threads access and modify shared data simultaneously, leading to unpredictable results. To avoid race conditions, it's crucial to synchronize access to shared resources properly using techniques such as locks or atomic variables. Blocking operations, such as network or file I/O, can significantly impact the performance of asynchronous code. To mitigate this, developers should consider using non-blocking or asynchronous alternatives for I/O operations, such as NIO or CompletableFuture. Improper error handling can also be a pitfall in asynchronous Java programming. It is necessary to handle exceptions appropriately and ensure that errors are propagated and handled correctly across different threads and asynchronous tasks. This can be achieved by using try-catch blocks or by using error-handling mechanisms provided by asynchronous frameworks, such as CompletableFuture's exceptionally() method.
JUnit 5 plays a significant role in implementing unit tests in Java, particularly in the context of test-driven development (TDD), given the importance of testing code for time-saving and reliability improvement.
A step-by-step guide is provided to explain the process of writing testable code and unit tests for a specific example, such as a username validation feature. The basic process of unit testing includes preparing test inputs, calling the code to be tested, and verifying the behavior and results. The article also discusses the use of abstractions and dependency injection to make code more testable and decoupled from specific implementations. The creation of test doubles for dependencies using interfaces and their use in unit tests is also covered.
The article also addresses controversial topics such as 100% code coverage and test-driven development, providing different perspectives on these subjects. It concludes by encouraging readers to experiment with different testing approaches and emphasizes the importance of regularly writing tests to enhance code quality
2. Testing Asynchronous Processes with JUnit: An Overview
JUnit has established itself as a reliable framework for unit testing in the Java ecosystem, thanks to its comprehensive set of annotations and assertions.
However, the task of testing asynchronous processes can often prove to be a complex puzzle due to their concurrent nature. It is imperative for developers to possess an in-depth understanding of both the JUnit framework and the Java concurrency model to successfully write tests for asynchronous code. The ultimate goal is to ensure that the test case waits for the asynchronous process to complete before validating any assertions.
One of the key challenges in testing asynchronous processes is the concurrent setup. Typically, the test case and the program under scrutiny operate on separate threads, introducing an additional layer of complexity. A prevalent workaround is to make the test case wait for the asynchronous processes to complete using the Thread.sleep method. However, this method does not guarantee consistent results across different environments.
To navigate these challenges, consider the insights offered in the book "Growing Object-Oriented Software Guided by Tests". Chapters 27 and 28 provide a deep dive into testing and multi-threaded code, yielding valuable knowledge. A key takeaway is the concept of separating concurrency policy and functionality. This can be achieved by making the executor service a part of the injected dependencies. This enables the test case to wait for the pool to terminate before making any assertions. For example, in a program that sends emails asynchronously using a notification handler class and a notification gateway, injecting the executor service allows the test case to wait for the pool to terminate before asserting the system under test.
The book also proposes a testing strategy for asynchronous code that comprises two techniques: listening and sampling. Listening involves capturing events dispatched by the system under test and evaluating criteria based on these events. For instance, a notification trace class could be employed to capture events and provide assertion methods. Conversely, sampling involves periodically observing the system under test's state and validating assertions based on that state. The book introduces a poller and a probe as constructs for state sampling and assertion validation. The poller continues to wait until the specified assertion criteria are met or a timeout is reached.
Testing asynchronous processes with JUnit can be a daunting task that demands a comprehensive understanding of the JUnit framework and the Java concurrency model. However, with the correct strategies and tools, such as those outlined in the book "Growing Object-Oriented Software Guided by Tests", these challenges can be surmounted. It is worth noting that waiting, listening, and sampling are not mutually exclusive mechanisms, and they can be used in combination depending on the situation and objectives of the system. By implementing these strategies, developers can ensure their tests are robust and reliable, regardless of the complexities inherent in asynchronous processes.
Additionally, when writing JUnit tests for concurrent execution, there are several best practices to follow. Ensuring your tests are thread-safe is crucial. This means they can be executed concurrently without interference. Techniques such as locks or semaphores can be used to control access to shared resources and achieve this. Designing your tests for parallel execution is also recommended. This involves minimizing dependencies between test cases and ensuring they can be executed independently, which can boost your test suite's overall performance.
Another valuable tool to consider is the CompletableFuture
class when testing asynchronous processes in JUnit. This class offers a way to work with asynchronous computations, allowing you to wait for their completion in a non-blocking manner. By using CompletableFuture
, you can test the asynchronous behavior of your code and verify the expected outcomes. To use CompletableFuture
in your JUnit tests, create a CompletableFuture
instance and pass it to the method or code block that performs the asynchronous operation. Then, you can use methods like get()
or join()
to wait for the CompletableFuture
to complete and assert the expected results.
Moreover, JUnit allows for the creation of test cases for concurrent execution using the @RunWith
annotation and the Thread
class. By creating multiple threads and running them concurrently, you can simulate concurrent execution in a JUnit test case. By adopting these strategies and tools, developers can write robust and reliable tests capable of handling the complexities of asynchronous and concurrent execution
3. Best Practices for Unit Testing Asynchronous Java Code
The process of crafting unit tests for asynchronous Java code demands a specialized approach to ensure that the tests are dependable and sturdy. The main focus should be on isolating asynchronous behavior, which aids in creating tests that are modular and easy to comprehend. This strategy subsequently leads to a more maintainable and understandable codebase.
A significant part of writing any unit test is managing time. It's crucial to incorporate timeouts in the tests to avert them from indefinitely hanging. This is particularly vital when dealing with asynchronous code, as the tests could potentially wait forever for a response that may never come. A well-placed timeout ensures that the test fails instead of indefinitely waiting, thereby providing valuable feedback promptly.
Exception handling is another critical factor contributing to the effectiveness of unit testing. Proper exception handling not only ensures that the test fails when it should, but it also offers crucial information about why the test failed. This makes it easier to identify and fix bugs in the codebase, thereby enhancing the overall quality of the software.
In the context of unit testing for asynchronous Java code, synchronization is a vital element. By synchronizing access to shared resources, we can prevent race conditions that could otherwise lead to unreliable and unpredictable test results. This is particularly crucial when the code being tested involves multiple threads that could potentially access and modify shared resources simultaneously.
Using a testing library that supports asynchronous testing can greatly simplify the process of writing unit tests. For instance, JUnit 5, a popular testing framework in the Java ecosystem, provides robust support for asynchronous testing. By leveraging such a framework, you can focus more on the logic of your tests and less on the mechanics of asynchronous testing.
When dealing with asynchronous methods, it's vital to note that the assert statement may be executed before the method has had a chance to complete due to the non-blocking nature of asynchronous code. To address this issue, a mock listener can be utilized to wait for the asynchronous method to complete before proceeding with the assert statement.
In this approach, an asynchronous method would execute in its own thread and notify a listener upon completion. The unit test would then wait for the completion of the method using a synchronized block and the wait() method. If the method completes within the specified timeout period, the results are then checked for correctness. If the method does not complete within the timeout period, the test fails. This ensures that the test does not hang indefinitely while waiting for the method to complete.
Finally, the process of unit testing asynchronous Java code can indeed be complex, but by adhering to these best practices and utilizing the appropriate tools, you can create tests that are reliable, maintainable, and effective in ensuring the quality of your software.
Furthermore, to enhance the efficiency of unit testing asynchronous Java code, it is advised to refer to various resources available online. These resources provide several techniques and tips that can help you effectively test asynchronous code in Java. Some common best practices include using frameworks like Mockito for mocking dependencies, using timeouts or explicit wait mechanisms to handle asynchronous operations, using callback mechanisms or CompletableFuture to handle async code, and properly managing test data and test environments. Additionally, writing clear and descriptive test cases, properly handling exceptions, and using assertions to verify the expected outcomes of the asynchronous code are also recommended. By following these best practices, you can ensure that your unit tests for asynchronous Java code are robust and provide accurate results
4. Strategies for Managing Multithreading in Unit Tests
Multithreading is an essential part of asynchronous Java programming, and managing it effectively is crucial for successful unit testing. This management includes controlling the number of threads, their execution sequence, and their interaction with shared resources. One strategy for this is to use a single-threaded executor for testing, which allows the tests to run in a predetermined order. Another technique involves the use of thread-safe data structures and synchronization methods to prevent race conditions. Additionally, Java Concurrency Utilities can be helpful in managing complex multithreading scenarios.
There are, however, challenges along the way. One such instance involved an embedded software engineer who faced a segmentation fault in a unit test project that had been running successfully for a year. The fault was located not in the code being tested, but within the test framework itself, traced back to a race condition caused by the sequence in which objects using inheritance were destroyed. This led to a complete revamp of the codebase to fix the race condition, and a shift towards using composition and dependency injection over inheritance to prevent similar issues in the future.
This incident underscores the importance of careful management of multithreading in unit tests. Even a seemingly minor race condition can lead to major problems, as the engineer discovered. This experience serves as an important reminder of the need for thorough testing and careful management of multithreading in unit tests.
In another situation, an Android app developer using the popular concurrency framework RxJava faced a challenging situation. The developer was working on a side project involving a RecyclerView that displays a list of elements obtained from two different API calls. Initially, the developer used concatMapEager to execute the API calls for the second endpoint concurrently while maintaining the order of the elements. However, the developer decided to optimize their code further by displaying the data as it becomes available instead of waiting for all API calls to complete. This change led to intermittent failure of the unit tests due to the intermittent content states. To rectify this, the developer updated their tests to include the intermittent states, and they passed. However, the developer discovered that the tests were flaky due to concurrency issues. The state updates were not thread-safe, leading to inconsistent state.
This case study emphasizes the importance of using real schedulers in testing to catch concurrency issues. It also highlights the necessity of making a conscious choice about whether to replace schedulers or not.
To manage multithreading in asynchronous Java code, various techniques can be utilized, such as the Executor framework, CompletableFuture, or reactive programming libraries like RxJava. These tools provide ways to execute tasks concurrently, handle thread synchronization, and manage thread pools for efficient execution of asynchronous code.
For thread-safe data structures and synchronization mechanisms for unit testing, there are several options available. One commonly used method is to use the synchronized keyword in Java, ensuring that only one thread can access a particular method or a block of code at a time. Another option is to use the concurrent data structures provided by the Java.util.concurrent package, such as ConcurrentHashMap or CopyOnWriteArrayList, which are designed to be thread-safe. Additionally, frameworks like JUnit provide built-in support for running tests in parallel, allowing for concurrent execution of test cases while ensuring thread safety.
To manage complex multithreading scenarios in Java, it is important to follow best practices for Java unit testing. This includes understanding annotations and assertions in the JUnit framework. Using annotations effectively can control the execution order and dependencies of multithreaded tests. Assertions allow you to verify expected outcomes and handle exceptions in a structured manner. Proper synchronization techniques, such as locks and semaphores, ensure thread safety and prevent race conditions.
These examples and solutions underline that effective management of multithreading in unit tests is crucial for the successful testing of asynchronous Java code
5. Effective Techniques for Unit Testing CompletableFuture in Java
Asynchronous programming in Java has evolved significantly with the introduction of CompletableFuture in Java 8. This advanced tool marks a significant enhancement over Java's Future API, addressing its limitations like the inability to manually complete a future or handle exceptions, the absence of completion notifications, and the incapability to chain or combine multiple futures.
CompletableFuture facilitates asynchronous programming by allowing developers to write non-blocking code. This code executes a task on a separate thread and communicates its progress, completion, or failure to the primary thread. Creating a CompletableFuture can be done using the no-argument constructor or via the runAsync or supplyAsync methods. The former method allows for the execution of a background task asynchronously without returning a result, while the latter enables the same but includes a returned result.
When there is a requirement to chain multiple futures, CompletableFuture provides several methods. The thenApply, thenAccept, and thenRun methods permit the attachment of callback functions to CompletableFuture. The async variants of these methods enable callback functions to be executed asynchronously. For combining two dependent CompletableFuture, the thenCompose method is used, and the thenCombine method is employed for independent CompletableFuture.
In scenarios where multiple CompletableFuture need to be combined, the allOf method runs them in parallel, while the anyOf method obtains the results of the first completed future. Exception handling in CompletableFuture is made possible with the exceptionally and handle methods.
When unit testing CompletableFuture, it is vital to test both successful and failure scenarios. The complete() and completeExceptionally() methods can be used to manually complete the CompletableFuture in the test. The thenApply() and exceptionally() methods can also be employed to test the chaining and error handling behavior.
To test success scenarios with CompletableFuture, the CompletableFuture.completedFuture
method can be used to create a CompletableFuture that is already completed with a specific value. This approach is useful for testing your code's behavior when the CompletableFuture completes successfully. The thenApply
method can be chained to a function that performs assertions on the result of the CompletableFuture, verifying the expected result and performing any necessary assertions. Additionally, the join
method can be used to block until the CompletableFuture completes, followed by assertions to verify the result.
In the broader context of JVM and system resources, understanding how CompletableFuture impacts thread creation is crucial, especially in containerized environments such as Kubernetes. This knowledge is key to avoiding potential performance issues related to excessive thread creation and CPU resource allocation, and developers can mitigate these issues by passing an executor or adjusting the system parallelism.
Overall, CompletableFuture offers a robust and flexible API for managing asynchronous programming in Java, with a variety of methods to create, chain, combine, and handle exceptions in futures. However, understanding its behavior in the context of system resources is crucial for effectively managing performance in various environments, including containerized ones like Kubernetes
6. Overcoming Common Challenges in Unit Testing Asynchronous Java Code
Unit testing asynchronous Java code does indeed pose unique challenges, particularly when it comes to dealing with indeterminacy, managing timeouts and exceptions, and ensuring thread safety. However, with the right strategies and tools, these challenges can be effectively managed.
One common issue when testing asynchronous methods is the premature execution of assertion statements before the completion of the method under test. This can be addressed by using a mock listener, which waits for the asynchronous method to finish before asserting the results. This mock listener is predicated on the concept that another thread, in this case, the unit test, waits for the completion of the task by calling notifyAll. This allows the unit test to resume once the task is completed.
Additionally, a deterministic scheduler can be employed to manage indeterminacy. This scheduler ensures that the assertxxx is executed only after the thread started by some asynchronous method has completed its work. Essentially, it regulates the order in which tasks are executed, minimizing the effects of indeterminacy.
The task of testing timeouts in unit testing for asynchronous Java code is another crucial aspect. A mock clock can prove to be a valuable tool here. By using a mock clock, time progression can be simulated, ensuring that our code correctly handles timeouts.
To ensure thread safety and test all code paths, including exception handling code, a code coverage tool can be used. This tool will analyze our code and provide a report indicating which parts of our code have been tested and which have not.
In the specific case of testing a search model that uses asynchronous methods, a mock listener would be required to wait for the search to complete. The unit test would then wait for the completion of the search using a synchronized block and a timeout period. If the search times out, the test fails. Otherwise, the test checks the results for correctness.
Frameworks like JUnit and Mockito can be used to unit test asynchronous Java code. One approach is to use the CompletableFuture class in Java, which provides a way to handle asynchronous computations. A CompletableFuture object can be created, and a series of operations can be chained together using thenApply, thenAccept, or thenCompose methods to represent your asynchronous code.
JUnit's CompletableFutureAssertions class can be used to test this asynchronous code. This class provides various methods to assert the completion, result, or exception of a CompletableFuture, verifying that the expected behavior of the asynchronous code is correct.
Mockito's asynchronous testing support can also be used. Mockito provides methods like whenCompleteAsync and whenCompleteAsync to stub and verify asynchronous calls. These methods can be used to simulate and test the behavior of asynchronous code.
The choice of which method to use for unit testing asynchronous Java code will depend on the specific requirements, personal preferences, and familiarity with the APIs. Therefore, it is always best to choose the method that best suits your specific needs and situation
7. Tips and Tricks to Optimize Efficiency of Asynchronous Unit Tests
Enhancing the efficiency of asynchronous unit tests in software development is paramount. This enhancement not only curtails the execution time but also bolsters the reliability of the tests. One of the methods to achieve this is by controlling the actual concurrency within the tests. Unbridled concurrency could potentially lead to unforeseen results and sluggish execution. However, this does not imply the total elimination of concurrency; rather, a controlled concurrency with deterministic scheduling is more beneficial.
Selecting the right assertions for testing asynchronous code is another crucial aspect. For example, CompletableFuture can be tested using assertThat().isDone(). Additionally, it is essential to manage resources effectively and clean up after each test to prevent memory leaks and other potential issues. This includes releasing any allocated memory, closing connections, and disposing of any objects that are no longer needed. Moreover, employing suitable synchronization mechanisms can help circumvent race conditions and ensure that tests are executed in the preferred order.
In the software development landscape, the speed of tests can significantly influence the development process. The slower the tests, the less frequently they are run, slowing down the development process. Fast testing is not just a design challenge but also a testament to the team's experience and professionalism. Slow tests could often indicate a design flaw, underscoring the importance of quick testing tools. For instance, a client experienced slow build and link times for their unit tests due to their testing tool, highlighting the importance of fast testing tools and the need to avoid anything that could decelerate the tests.
Test-Driven Development (TDD) advocates for fearless and cost-free refactoring. It promotes the creation of decoupled architectures that support quick test doubles and the stubbing out of slow operations. For example, the testing framework Fitnesse, which has been in development for over a decade, was designed to have quick test times. It can stub out slow operations such as the persistence framework and web framework. This practical example exhibits how decoupled architectures and stubbing can contribute to achieving quick test times.
Ultimately, adopting a systematic and professional approach to asynchronous unit testing is essential. By managing concurrency, selecting suitable assertions, and ensuring resource cleanup after each test, software developers can amplify the efficiency of their unit tests. Moreover, by adhering to the principles of TDD and concentrating on the design challenge of quick testing, they can ensure the regularity and reliability of their tests, thereby augmenting the overall software development process
Conclusion
In conclusion, unit testing asynchronous Java code poses unique challenges due to the concurrent nature of asynchronous operations. However, by implementing best practices and utilizing tools like mock listeners and deterministic schedulers, developers can overcome these challenges and ensure reliable and efficient tests. Techniques such as handling exceptions, managing multithreading, and optimizing test efficiency are crucial in ensuring the quality and reliability of software that incorporates asynchronous programming in Java. By following these strategies, developers can effectively manage the complexities of asynchronous programming and improve the overall efficacy of their applications.
The ideas discussed in this article have significant implications for software engineering. Asynchronous programming has become a fundamental aspect of modern software development, and being able to effectively test asynchronous Java code is essential for ensuring the reliability and quality of software. By following best practices for unit testing asynchronous code, developers can identify and fix bugs early in the development process, leading to more robust and stable applications. Additionally, optimizing the efficiency of tests can save time and resources, allowing developers to iterate faster and deliver high-quality software more efficiently.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.