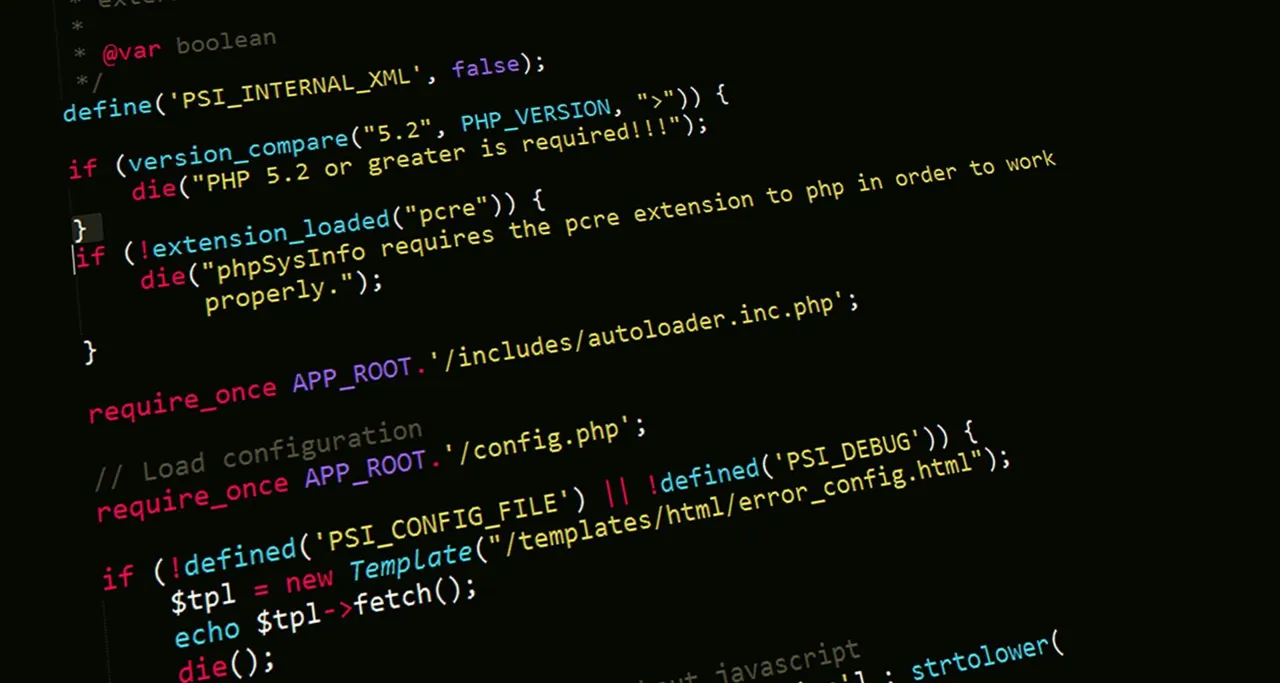
Table of Contents
- Why Unit Testing Matters
- How to Write Testable Code
- Unit Testing Basics and Best Practices
- Unit Testing in Python
- Unit Testing in PHP
- Unit Testing in JavaScript (Vue.js)
- Unit Testing in Swift (Network)
- Creating and Running Unit Tests for Managed Code (C)
- Creating a Project to Test
- Creating a Unit Test Project
- Adding a Reference to the Project Under Test
- Creating the Test Class
- Renaming a File and Class
- Adding a Using Statement
- Test Class Requirements
- Creating the First Test Method
- Test Method Requirements
- Building and Running the Test
- Fixing Failed Tests
- Improving Code with Unit Tests
- Analyzing Issues
- Creating and Running New Test Methods
- Refactoring the Code Under Test
- Refactoring Test Methods
- Retesting, Rewriting, and Reanalyzing
Introduction
Unit testing plays a pivotal role in software development, offering a means to detect and correct bugs early, thus minimizing the time and expense on fixes. It is key to both improving code quality and ensuring that new features or changes don't disrupt existing functionality. Despite its importance, unit testing is often overlooked during the development phase, largely due to time constraints or a rush to market. This can result in a greater number of bugs, escalating bug-fixing costs, and other complications. Machinet.net, an AI software company, offers a solution to the challenges of unit testing.
It provides an AI assistant for developers, automating unit test generation, simplifying mocking with Mockito, and effectively structuring and isolating dependencies in tests. The platform emphasizes the significance of testing processes early in the development cycle to reduce the risk of new bugs, thereby delivering robust software. Ultimately, the role of unit testing in software development is invaluable. It acts as a safety net, ensuring software reliability and contributing to user satisfaction. It is an essential tool for producing robust, error-free software solutions.
Why Unit Testing Matters
Unit testing plays a pivotal role in software development, offering a means to detect and correct bugs early, thus minimizing the time and expense on fixes. It is key to both improving code quality and ensuring that new features or changes don't disrupt existing functionality. Unit testing involves scrutinizing individual components independently to ensure they perform as anticipated. To achieve comprehensive coverage, test cases should encompass typical use cases, edge cases, and boundary conditions. This proactive methodology can greatly diminish the total cost of ownership, making it a worthy investment of resources. Despite its importance, unit testing is often overlooked during the development phase, largely due to time constraints or a rush to market. This can result in a greater number of bugs, escalating bug-fixing costs, and other complications. An alternative to this approach is to embed quality controls from the outset and foster strong cooperation between developers and testers, thereby ensuring a productive development process and superior software.
There are unit testing frameworks for various programming languages that aid in automating performance testing. These not only help confirm that each component functions as intended but also support in segregating the application code into smaller, manageable portions for individual testing. Machinet.net, an AI software company, offers a solution to the challenges of unit testing. It provides an AI assistant for developers, automating unit test generation, simplifying mocking with Mockito, and effectively structuring and isolating dependencies in tests. The platform emphasizes the significance of testing processes early in the development cycle to reduce the risk of new bugs, thereby delivering robust software. Ultimately, the role of unit testing in software development is invaluable. It acts as a safety net, ensuring software reliability and contributing to user satisfaction. It is an essential tool for producing robust, error-free software solutions.
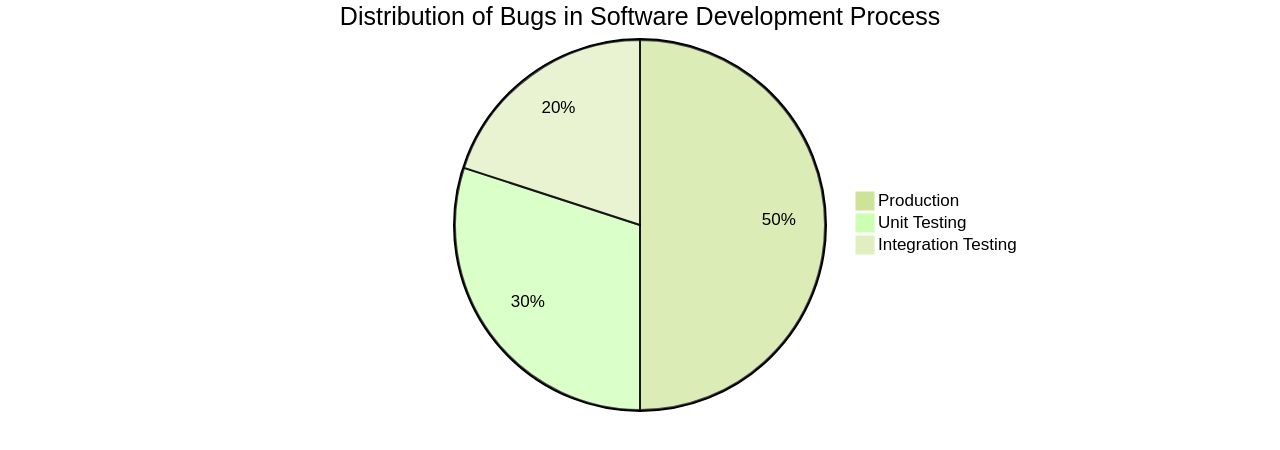
How to Write Testable Code
To achieve effective unit testing, it is essential to focus on developing testable code. This involves creating modular and loosely coupled code, minimizing dependencies, and implementing dependency injection. These practices not only enhance testability but also improve code quality and reliability, enabling quicker defect identification. Understanding controllability, which involves crafting an API that can control the object efficiently, is another pivotal aspect. It offers immediate feedback on the API's usability and its capacity to manage an object. In this scenario, test-driven development (TDD) can be a potent tool, where a failing test is written before implementing the solution.
This method ensures higher test coverage and more reliable code, minimizing the risk of biased tests and overlooked implementation aspects. The Arrange, Act, and Assert (AAA) technique is another key strategy in unit testing, providing a sturdy foundation for developers aiming to ensure code quality. However, tight coupling in code, where parts of the code are highly dependent on each other, can be a substantial barrier to achieving highly testable and maintainable code. This can lead to a series of unintended consequences, where a minor change in one part of the code triggers a chain reaction of bugs in other parts. Machinet.net, a platform for Java developers, automates the process of writing unit tests using Mockito, which improves productivity and ensures code correctness. It offers a comprehensive unit testing strategy that detects and fixes defects early in the development cycle, enhancing code quality and delivering high-quality software. The platform also offers resources like ebooks, demos, and blog articles, and career opportunities for those interested in AI and software development.
Unit Testing Basics and Best Practices
Unit testing remains a cornerstone in software development, concentrating on validating individual pieces of code separately from the larger system. These self-contained, specific tests aim to be easily understood, maintained, and executed, working to pinpoint potential bugs early in the development cycle and ensuring the code operates as expected. A popular technique in unit testing is the Arrange, Act, and Assert (AAA) method. This technique breaks a test into three distinct segments: setting up the test data, carrying out the action or method, and verifying the results. This method promotes clarity in each phase, facilitating understanding and easing troubleshooting. In generating tests, the focus should be on simplicity. Tests should be designed for straightforward failure identification, aiding in swift issue resolution. Furthermore, every test should serve a specific purpose. It's not about generating tests simply for coverage, but about ensuring they yield significant results. Software tools like Istanbul, Coveralls, and Codecov can assist in pinpointing coverage gaps, enhancing the integrity and trust in your tests.
For those stepping into unit testing, it’s recommended to begin with simpler functions or components, progressively building confidence and expertise. A comprehensive testing strategy, as proposed by the testing pyramid concept, advises for an optimal distribution of different test types in your test suite. When combined with a focus on the assurance each test provides against the time and effort put into it, this can accelerate the delivery of superior software. Lastly, it’s important to remember that tests should comply with principles like the Open/Closed Principle, which ensures they can be extended without modifying existing ones, and the Dependency Inversion Principle, advocating for reliance on abstractions over concrete implementations. These guidelines, relevant regardless of the test type, can enhance your testing efforts. Machinet.net, a software development platform that specializes in unit testing in Java, provides a comprehensive unit testing strategy. It automates the process of writing unit tests using Mockito, aiding in improving code quality and productivity. By utilizing JUnit and effective assertions, Machinet.net offers various features, use cases, and pricing options. It also hosts career opportunities and a blog with beneficial resources. Users can sign up for an account and access a demo of the product.
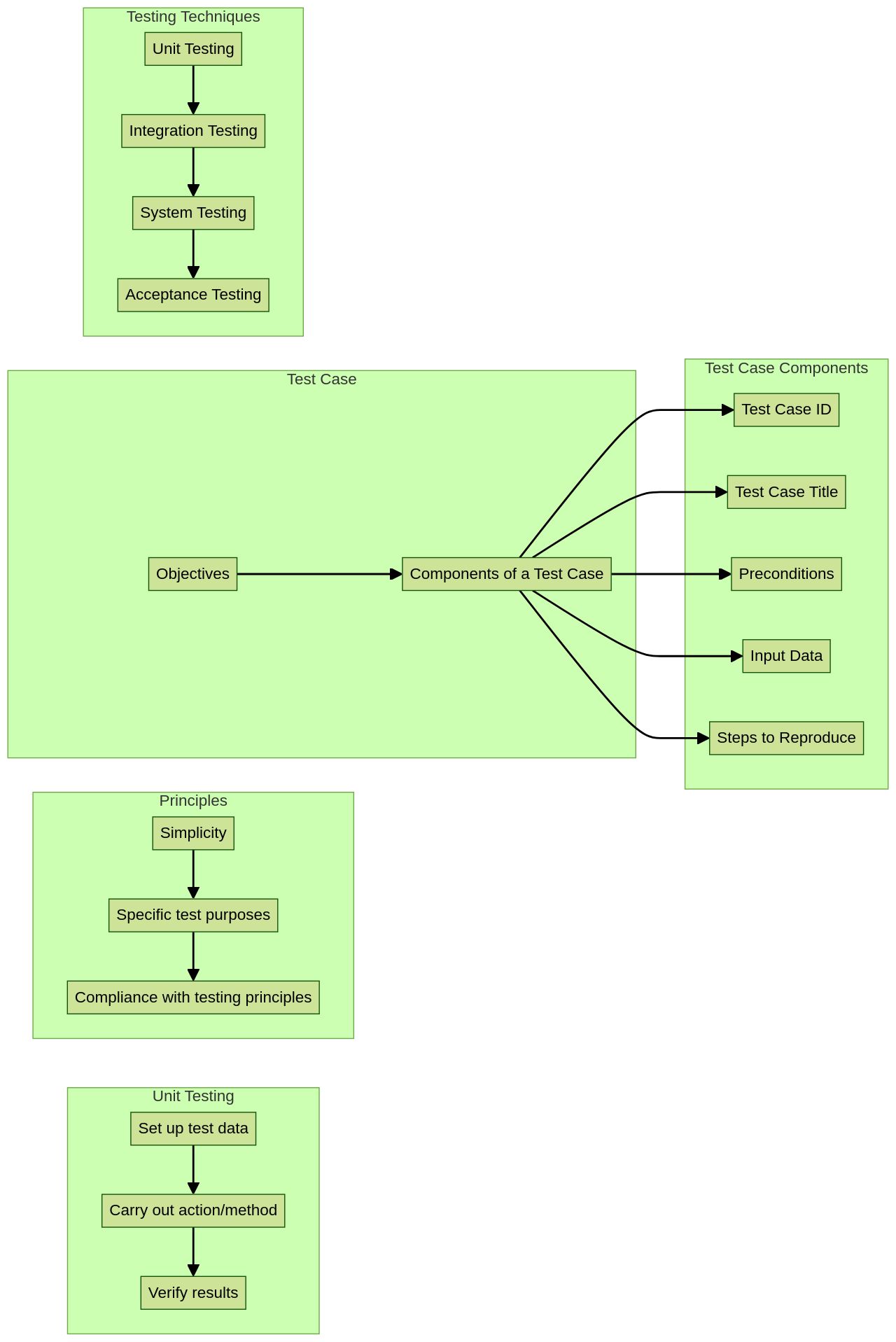
Unit Testing in Python
Python, a widely adopted programming language, boasts a rich arsenal of testing frameworks and tools. Delving into unit testing, a method used to verify the functionality of individual software components in isolation, we explore Python's built-in 'unittest' module and the well-regarded 'pytest' framework. Unit testing is a key responsibility of developers, ensuring that each function or module of the application is functioning correctly and adheres to the required specifications. This is achieved through writing and running test cases, and generating test coverage reports. Tools like the 'unittest' module and 'pytest' are instrumental in this process. An important aspect of unit testing is Test-Driven Development (TDD), a strategy aimed at minimizing software failures and ensuring the software meets intended requirements.
This is accomplished by describing the expected behavior of the code through test cases written upfront, providing developers with a clear understanding of their objectives. Another useful tool in Python's testing suite is 'Coverage.py', a code coverage tool that indicates which parts of your code are being exercised by tests. The latest version, coverage.py 7.3.2, was released in October 2023, keeping in pace with the annual release of new Python versions since 2019. However, testing isn't limited to unit testing. It also helps identify defects, ensure desired functionality, compatibility across different systems, and reduce risks by identifying potential vulnerabilities. Python's versatile testing tools facilitate this process, making it an ideal language for ensuring software quality and maintainability.
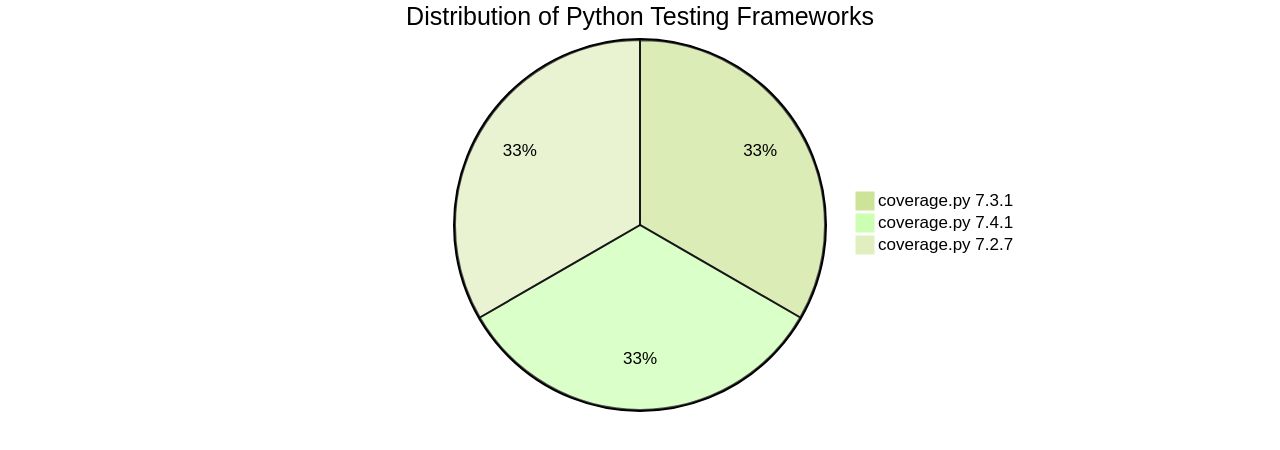
Unit Testing in PHP
PHP, a prevalent scripting language, is widely adopted for web development, with unit testing being a crucial component of its development process. The 'PHPUnit' testing framework provides a robust platform for such testing. This includes setting up a conducive testing environment, crafting test cases, and utilizing assertions to confirm expected behavior. Unit tests are primarily written to assist developers in identifying and fixing bugs, refactoring code, and serving as software documentation. These tests should ideally cover all possible paths in a program. One such example is a test that depends on 'DependencyFailureTest::testOne' to pass. PHPUnit ensures that the dependencies of a test can be met before it gets a fixture from the first producer as the first argument, a fixture from the second producer as the second argument, and so on.
This forms an integral part of the testing process as a test method can accept arbitrary arguments. The importance of PHPUnit is further emphasized with the recent release of PHPUnit 9.6.0. Meanwhile, the PHP team is preparing for the release of a new minor version of PHP, with numerous improvements and features. This evolution in PHP development, coupled with the continuous improvements and support provided by the PhpStorm team for the Pest plugin, underscores the importance of PHPUnit in the PHP development landscape. A study measuring test effectiveness found that the actual bug fixes associated with a test case in software's history is a crucial factor. This highlights the importance of testing in maintaining code quality and preventing future errors. Lastly, remember that the approach to writing tests can vary, but the importance of good test coverage and testable code remains paramount.

Unit Testing in JavaScript (Vue.js)
JavaScript, known for its versatility, is a key player in both front-end and back-end development realms. It's particularly invaluable when it comes to unit testing, a fundamental pillar of robust software applications. Unit testing aids in early bug detection and guarantees code functionality as anticipated. One of the prevalent strategies in unit testing is the Arrange, Act, and Assert (AAA) technique, which segments a test into three distinct phases. In the context of JavaScript, we'll be delving into the Vue.js framework, a favorite among developers due to its simplicity and flexibility. Particularly, we'll explore Vitest, a rising star in the testing framework sphere, brought to you by the Vue team. This framework is designed to test almost all other frameworks used in unison with its predecessor, Vite.
In addition to using Vitest, we'll also discuss utilizing testing libraries like 'Jest' and 'Vue Test Utils'. Jest stands out for its user-friendly API, making it convenient to write and manage tests. It's also one of the fastest JavaScript testing frameworks, courtesy of its virtual DOM and parallel test execution capability. The importance of unit testing in maintaining the stability of growing applications cannot be overstated. Therefore, we'll also touch on the use of a code coverage tool, an instrumental resource in identifying untested code sections. Remember, start small and simple if you're new to unit testing, gradually enhancing your skills and confidence. This exploration into JavaScript unit testing will provide you with an understanding of best practices and effective tools to make testing an integral part of your development process.
Unit Testing in Swift (Network)
Swift serves as the core language for app development across iOS, macOS, watchOS, and tvOS. This section deep dives into the nuances of unit testing in Swift, particularly focusing on the testing of network functionalities. We delve into the use of mock network requests, the application of test doubles, and the intricacies of asynchronous testing. Unit testing plays a pivotal role in continuous integration and deployment pipelines, forming the bedrock of automated testing. It allows organizations to ensure the quality of the code at every stage of the development process, setting a standard for code quality. In certain industries, regulatory requirements necessitate comprehensive testing to ensure the accuracy and safety of software. Unit tests serve as evidence of code correctness, meeting these compliance standards. Unit tests also act as a bridge between developers, testers, and other stakeholders, fostering collaboration and communication.
Everyone involved can understand the expected behavior of the code through unit tests, instilling confidence in the codebase. With a robust suite of unit tests, developers can be assured that their changes will not inadvertently break existing functionality, leading to a more reliable and stable software product. In the realm of Swift programming, understanding various types of test doubles and their applications can greatly enhance your testing strategies. Test doubles simulate the behavior of real components or services, enabling developers to isolate and test individual parts of their code more effectively. In the context of performance, even small variations in response time can significantly impact outcomes. This is particularly crucial in fast-paced environments where high throughput, low latency, and predictable performance are required. Performance is an integral part of the overall product, and instant response to user operations truly helps provide a delightful end user experience. After analyzing the existing infrastructure within the Swift ecosystem, we identified a gap for multi-platform and rich metrics support, CI integration, and developer-friendliness. This led to the development of an open-source Benchmark package, aiming to advance performance for the Swift community.
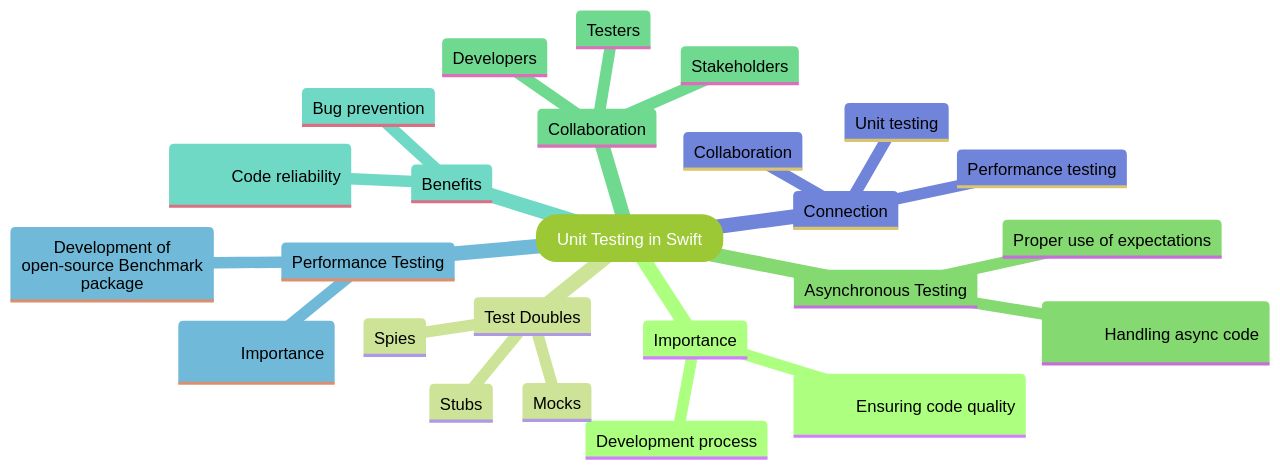
Creating and Running Unit Tests for Managed Code (C)
In the realm of C# and .NET development, the role of unit testing is key. It serves as a mechanism to verify the accuracy of individual code units, typically methods or functions, in isolation from the rest of the system. This practice ensures that each code unit performs as expected, aiding in the early detection of potential bugs or issues. The tests are automated, self-contained, and focused on specific aspects of a unit, making them readily understandable, maintainable, and executable. The NUnit testing framework is a pivotal tool for .NET developers. Its 'Arrange-Act-Assert' pattern facilitates the identification of problems in the event of a test failure, thus aiding developers in isolating errors and generating effective debug reports. NUnit tests are automated, enabling quick and easy execution.
Regularly running these tests can help catch errors and spot issues early in the development lifecycle, thus ensuring code quality. Moreover, the functionality of an application can change with the addition or modification of code. By adhering to good practices, such as avoiding multiple assertions in a single test and ensuring tests are open for extension, the integrity of the application can be maintained. In the process of test creation, we utilize mocks to write tests, write code along the way, refactor the code, and then test the code to ensure we refactored correctly and everything is functioning properly. An example of this process can be seen in the creation of the TranslatorDataSourceTest, where a translator loaded from an external data source is tested. In conclusion, unit testing in C# and .NET is a crucial practice for maintaining code quality and catching bugs early in the development lifecycle. The use of testing frameworks such as NUnit and adherence to good testing practices can help developers ensure the functionality and integrity of their applications.
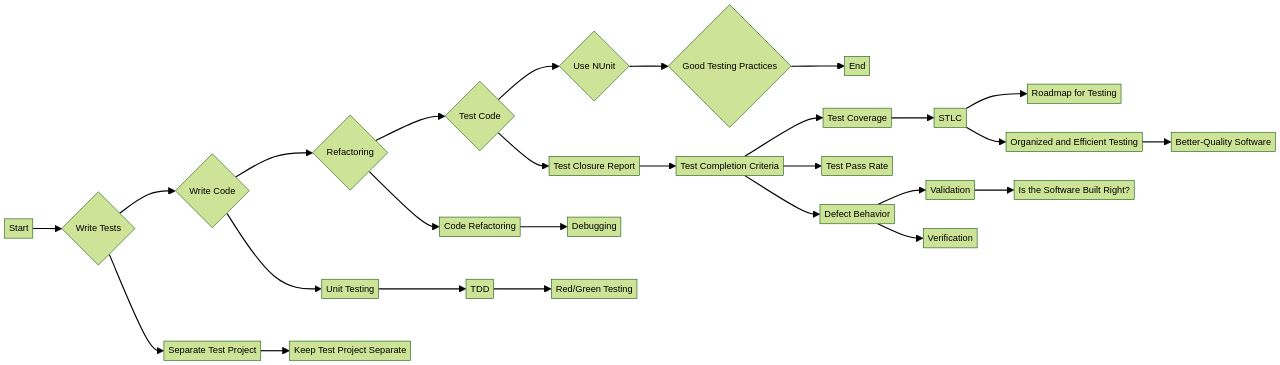
Creating a Project to Test
The first step in the testing journey is to prepare a project for validation. This involves establishing dependencies, setting up your build system, and organizing your codebase efficiently. The principle of Test-driven development (TDD) suggests that before you write any code, you should write a test that defines a new function or improvement, which should initially fail as the function doesn't exist or the improvement hasn't been made. This process ensures that your test is correctly written and is testing the desired functionality. The approach in an integration testing project is to minimize the time required for validating changes within the test suites and corresponding libraries. The automation team typically runs the same test suites that developers use to ensure that changes in tests won't impact the continuous integration (CI) processes for developers. However, the challenge arises when these test suites require substantial infrastructure resources and take a significant amount of time to complete. An organized and well-designed codebase is crucial for productivity and long-term maintainability of the project.
It requires clear communication, shared understanding, and discipline from all members of the team. Over time, without a concerted effort to maintain consistency, the codebase can become a patchwork of different styles and patterns, making it difficult to understand and maintain. Unit testing is a crucial practice in modern software development that ensures functionality and enhances maintainability. Well-written tests act as a safety net, allowing developers to refactor or change the code with confidence. Yet, it's important to note that the value of unit tests in the early stages of a project's lifetime may be limited. In such cases, integration tests can often prove more useful. Innovation in software applications is rapid, and quality assurance (QA) teams constantly strive to ensure functionality, quality, and speed of release for their digital products. The perception of software testing is shifting from being a financial liability to providing substantial cost savings and return on investment, provided modern methods are utilized.

Creating a Unit Test Project
Unit testing is an essential step in the software development process that scrutinizes the smallest, testable parts of an application to ensure they function as expected. This process calls for the creation and configuration of a unique unit test project, designed to critically examine individual code units in isolation. Understanding the expected behavior of the unit under test is an essential first step in setting up a unit test project. This knowledge comes from a detailed analysis of the specifications, requirements, and design documents, which clarifies the intended functionality. Once this is established, test cases are created covering a range of scenarios, including regular use cases, edge cases, and boundary conditions. The goal is to test the unit with various input combinations and error scenarios, ensuring comprehensive coverage. The naming of test cases is also vital, as each test case should be descriptively named to accurately reflect its purpose. This practice aids in quickly identifying and addressing issues when they arise, making the debugging process more efficient.
Additionally, a makefile can be integrated to manage the tests, simplifying the compilation and execution of all tests in the directory that follow a particular naming convention. The reporting functionality of the framework is equally important, producing clear, concise, and informative reports that detail each test's outcome. This detailed reporting allows for easy pinpointing of issues, enhancing the overall testing process. While unit testing focuses on individual code units, integration tests are also beneficial, examining how different components interact. Employing a multi-layered approach to testing, which includes both unit and integration tests, ensures more robust code and instills greater confidence in the software development process. Incorporating Machinet.net into this process can simplify unit testing. Machinet.net, a platform designed for Java developers, provides tips, techniques, and best practices for unit testing. It emphasizes the benefits of automated unit test generation, catching and fixing issues early in the development cycle, and reducing the likelihood of introducing new bugs. It also offers an AI assistant for developers, making the testing process even more efficient.
Adding a Reference to the Project Under Test
In the realm of software development, crafting unit tests for a specific project necessitates the inclusion of a reference to that project within the unit test project. This action ensures that the unit test project can access the code under test, thereby verifying the correctness of individual units of code, often methods or functions, independently from the rest of the system. This practice of unit testing aids in catching potential bugs or issues early in the development process, contributing to the overall quality and maintainability of the code. Moreover, unit tests are automated, self-contained, and focused on specific aspects of a unit, making them easy to understand, maintain, and execute. They form a robust foundation for any developer aiming to confirm the quality of their code. Notably, unit testing saves time by reducing debugging time.
It's simpler to locate the source of a problem in a module with unit test coverage than in one without. Furthermore, unit tests play a vital role in Continuous Integration and Deployment (CI/CD) pipelines, forming the bedrock for automated testing and enabling organizations to guarantee the quality of the code at every stage of the development process. In industries with stringent regulatory requirements, unit tests help meet compliance standards by offering proof of code correctness. Finally, it's crucial to keep your tests simple and easy to understand, focusing on testing a single, specific functionality or feature. This approach allows for quick identification and resolution of issues when they arise. Remember, tests are not just for coverage; they should always serve a purpose.

Creating the Test Class
A test class is a crucial component in the realm of software testing, encapsulating individual test methods specific to a particular unit or component. The creation of a test class involves defining essential setup and teardown methods, along with organizing test code in a systematic manner. Each test case within this class serves a specific objective, targeting a particular aspect of the software's functionality or performance. These test cases are identifiable through unique IDs, each carrying a concise and descriptive title reflecting the purpose of the test. Before executing a test case, certain preconditions must be met. Test cases also require specific data inputs or conditions. The execution process of each test case is guided by a step-by-step roadmap, known as 'Steps to Reproduce'.
The testing process utilizes techniques such as Equivalence Partitioning and Boundary Value Analysis. The former involves dividing a range of input values into classes or partitions, with each class exhibiting similar behavior. This helps optimize test coverage by reducing redundancy. The latter technique focuses on testing at the boundaries of input domains, where software is more likely to fail, thereby uncovering issues related to boundary conditions. Testing isn't just a task, but an integral part of the development process. It ensures the robustness, dependability, and adaptability of the code to the evolving development landscape. The process of unit testing verifies the correctness of individual units of code, independent from the rest of the system, catching potential bugs or issues early in the development process. This forms a solid foundation for developers ensuring the quality of their code.

Renaming a File and Class
In the realm of software development, the necessity to rename a file or class within an ongoing project is not uncommon. This process is part of the refactoring techniques that aim to reduce complexity and increase the comprehensibility of the codebase. Renaming enhances the understanding of the problem domain and aligns class names with their intended functions, making the code more intuitive for developers. However, this renaming process should be done carefully to ensure that the associated unit tests are updated accordingly, preserving the old functionality. This is crucial especially in projects undergoing redesign, where changes can yield significant improvements in performance and revenue, as observed in a Ruby on Rails application redesign that resulted in a 30% increase in project revenue.
Maintaining a coherent and clean codebase is fundamental to fostering collaboration among team members and making the codebase more maintainable. Adopting and adhering to a naming convention across all projects allows for consistency, which is key to maintaining a coherent codebase. To ensure that your code is well formatted, it is advisable to choose a set of simple rules that govern your code and then apply them consistently. Leveraging semantic naming conventions and community-accepted coding standards can aid in maintaining consistency in naming conventions, spacing, and code structure. In conclusion, renaming is a vital practice in software development, but it should be performed cautiously to ensure the associated unit tests are updated, thereby retaining the old functionality while simultaneously enhancing the maintainability and readability of the codebase.

Adding a Using Statement
Unit testing, a vital aspect of software development, involves examining individual components of an application during creation. The primary objective is to confirm each unit operates as anticipated. Unit testing frameworks can streamline this process, enabling standardized test script writing, and providing reusable testing modules and libraries. These frameworks also help in analyzing test and requirement coverage. Consider the scenario of unit testing in Node.js using the NestJS framework. Here, the emphasis is on testing a BookService class and its methods for creating, retrieving, updating, and deleting books. The process starts with environment setup, dependency importing, and BookService class construction. Writing unit tests, however, can be lengthy, particularly for complex systems. Therefore, a code coverage tool is crucial to measure the extent of test coverage on your code. Such tools can highlight testing gaps, leading to more comprehensive and confident tests.
The testing pyramid concept, which describes the ideal distribution of various test types in your test suite, is also essential. These range from unit tests to integration tests and end-to-end tests. Beginning with small functions or components and writing basic tests can help familiarize with testing tools and techniques, thereby gradually building confidence and skills. In terms of testability, the principle of separation of concerns is critical, allowing each codebase part to be tested individually. This method enables developers to isolate problems effectively and make changes without unexpected outcomes. Remember, unit tests are not just for coverage—they should always serve a purpose. They are crucial in ensuring the tests are meaningful and that issues can be swiftly identified and rectified. In this context, Machinet.net comes into play, a platform that automates unit test writing. It uses Mockito, a mocking framework for Java, to help developers enhance productivity and ensure code correctness. This automation of unit test generation, along with resources on test structuring, dependency isolation, and effective assertion use, can significantly ease the unit testing process. Machinet.net also covers JUnit annotations and assertions for writing efficient unit tests, offering a robust solution to the time-consuming nature of writing unit tests.

Test Class Requirements
A well-structured unit test class is fundamental to ensuring the reliability and efficiency of software applications. This involves a clear understanding of the unit's expected behavior, drawn from the specifications, requirements, and design documents. The design of test cases should cover a range of scenarios including normal use, edge cases, and boundary conditions, testing the unit with different input combinations and error scenarios. Each test case should have a specific objective, evaluating a particular aspect of the software’s functionality or performance. Key components of a test case include a unique ID for easy tracking, a descriptive title that reflects the purpose of the test, preconditions that must be met before executing the test case, specific data inputs or conditions required for the test, and a step-by-step guide on how to execute the test case.
Descriptive test names are essential, providing clarity and ease of understanding. It's also beneficial to group input values into classes that are expected to exhibit similar behavior, a technique known as Equivalence Partitioning. In the world of software development, unit testing plays a crucial role in ensuring the quality and reliability of applications. It verifies that each unit of code works as expected, helping to catch potential bugs or issues early in the development process. Well-written tests act as a safety net, enhancing maintainability and allowing developers to refactor or change the code with confidence.

Creating the First Test Method
Embarking on the journey of unit testing begins with crafting your maiden test method. This process involves setting up the required preconditions, executing the action or behavior to be scrutinized, and validating the anticipated outcome. It's akin to setting up a stage for a performance, where the preconditions are the props, the action or behavior is the performance itself, and the expected outcome is the applause. Unit testing, while sometimes perceived as mundane, plays a critical role in software development. It's like a safety net, catching defects before they escalate to integration, consequently saving time and reducing costs. It's a task that can be undertaken by anyone with access to the source code, from developers to QA engineers. Applying the Arrange, Act, and Assert (AAA) technique is a common practice in unit testing.
It's like preparing a three-course meal: arranging the ingredients (test data), cooking the meal (performing the action), and finally, tasting the results (asserting the outcome). This methodical approach ensures that each test case focuses on a single functionality, making it easier to pinpoint any issues. It's crucial to remember that tests should be more than just a coverage exercise; they should serve a purpose. Keeping them simple and easy to comprehend allows for swift issue identification and resolution. So, think of each test as a story - it should be clear, concise, and meaningful, allowing anyone to understand what went wrong if it fails. Moreover, unit tests are not just about catching bugs; they also help ensure compatibility across different operating systems and hardware devices. This broadens the user base of the software system, enhances user satisfaction, and minimizes risks such as data loss or system crashes. In conclusion, writing your first test method may seem daunting, but with the right approach and mindset, it can be a stepping stone towards producing high-quality, reliable software.
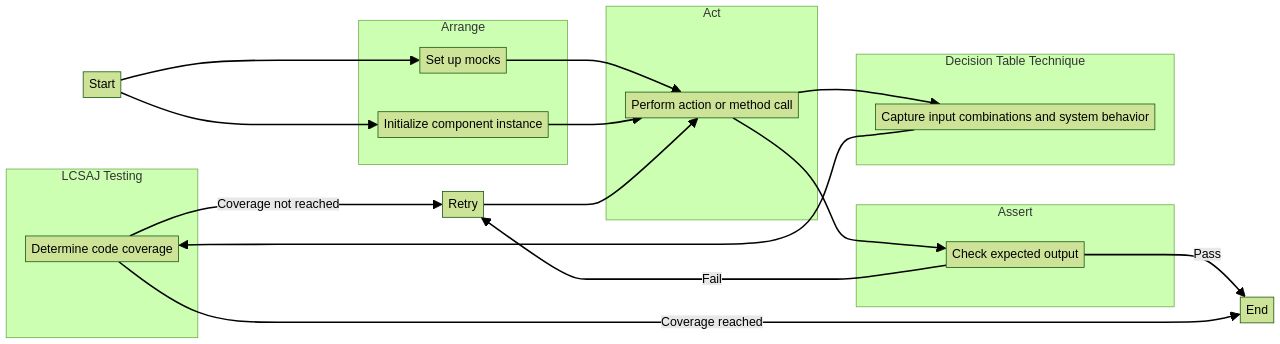
Test Method Requirements
Creating a test method involves more than just understanding its function in the testing process. It's also about the application of specific requirements such as naming conventions, attribute usage, and the inclusion of assertions. These elements are not only based on developers' beliefs or software engineering advice but are also backed by empirical studies conducted on open software repositories. The design of a test case should be concise, focusing on a single expected result to maintain simplicity and clarity. This not only enhances the stability of applications but also ensures that the introduction of new features doesn't negatively impact the application’s functionality. Moreover, a well-crafted test case should include preconditions, test steps/actions, and test inputs.
These elements work together to organize tests into groups called test suites, which are crucial in maintaining straightforward communication during testing. The quality of a test case is vital to the software development process. It serves as the first defensive line in bug prevention and plays a significant role in program comprehension and maintenance. Furthermore, it is essential to focus on testing individual units in isolation from other components, covering different scenarios, including normal use cases, edge cases, and boundary conditions. In conclusion, the creation of a good test case is a critical and complex task that requires a thorough understanding of the software's expected behavior, the ability to cover different scenarios, and the use of descriptive test names. This not only ensures the effectiveness of the test but also contributes significantly to the software's overall quality and reliability.
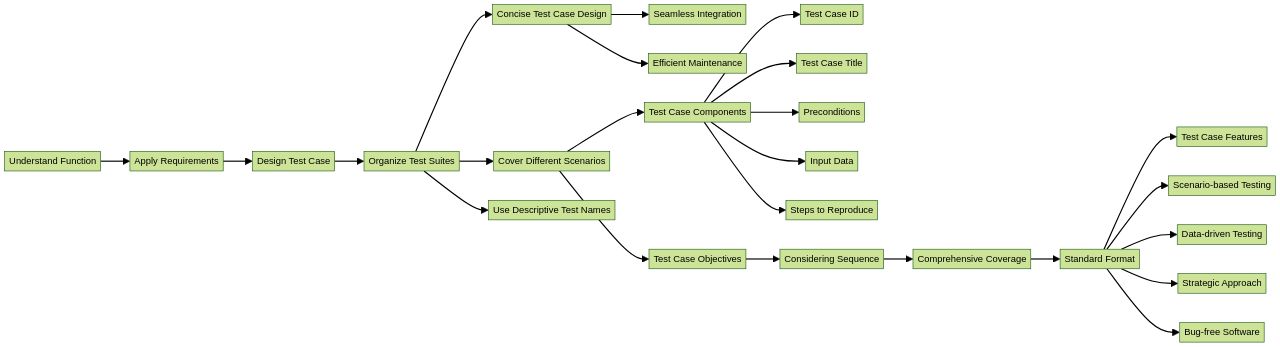
Building and Running the Test
Ensuring the functionality of your code through unit tests is crucial. A variety of tools and frameworks are available to help you build and run these tests. Each test is a unique program verifying a specific part of the software. A makefile aids in managing these tests for easy compilation and execution. Reports from these tests are clear and concise, and the reporting process includes functions like 'tstrun', 'tstcase', 'tstcheck', 'tstassert', and 'tstgroup'. A code coverage tool can measure how much of your code is covered by tests, helping to identify any testing gaps. For those new to unit testing, starting with simple functions or components can be beneficial. The testing pyramid concept can guide the distribution of different tests in your suite.
However, writing unit tests can be time-consuming and may not catch every bug. As the system evolves, maintaining and updating unit tests can require significant effort. Tests should be small and focused, making debugging easier. Descriptive test names help developers understand the test code's purpose. Enter Machinet.net, a platform that aims to improve productivity and code correctness through automated unit test generation for Java developers. It offers features such as mock creation and unit testing, and provides resources like ebooks and blog articles to help developers get started with Java unit testing. Machinet.net emphasizes the importance of code quality and catching and fixing issues early in the development cycle. The platform also offers career opportunities and encourages users to join their team to shape the future of AI software. With Machinet.net, writing unit tests becomes less of a chore and more of a streamlined process.
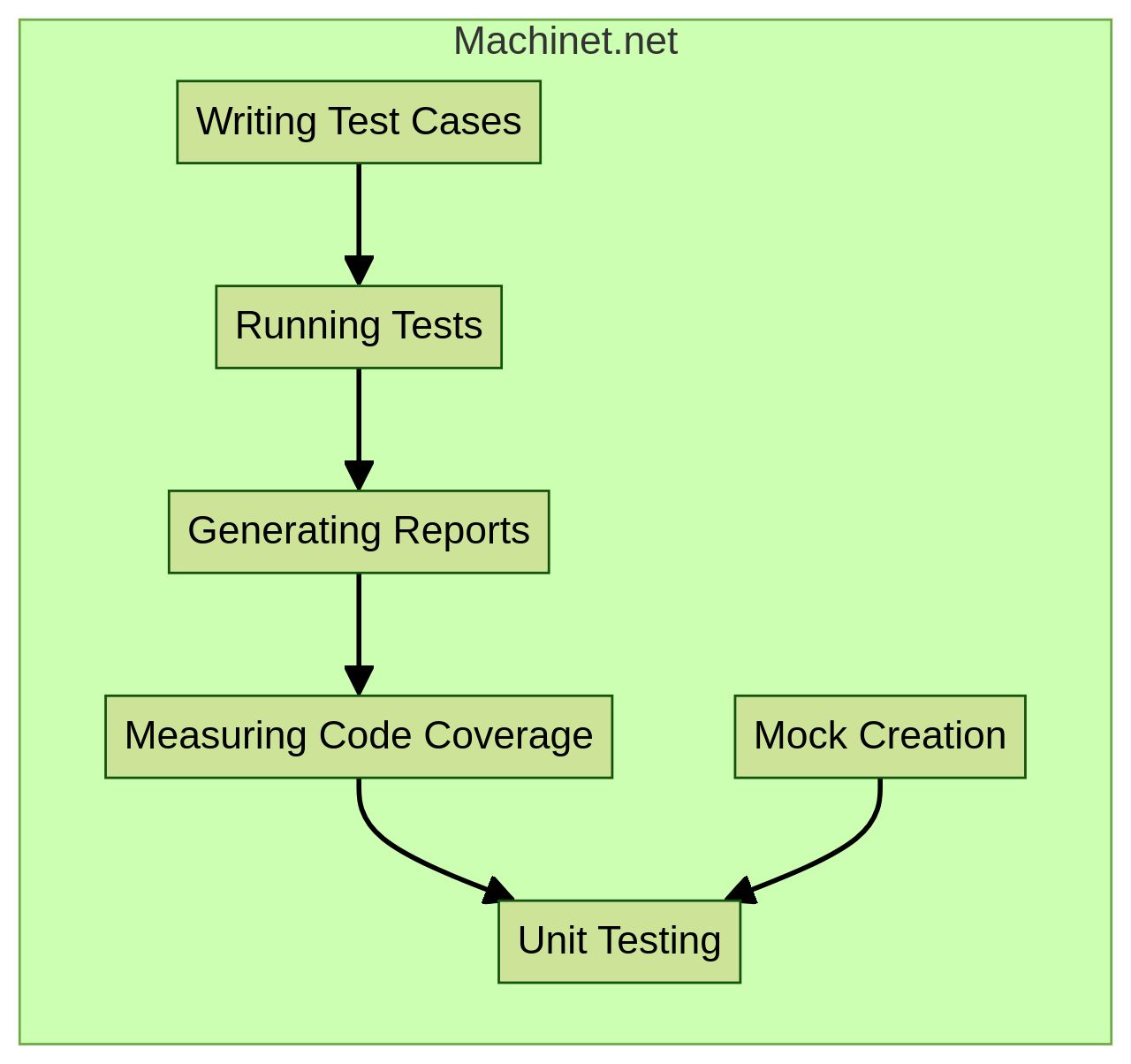
Fixing Failed Tests
Unit testing is a crucial part of software development, ensuring that each piece of code functions as intended. The debugging process can be complicated, especially when tests fail intermittently or disappear after logging. To mitigate these issues, developers are turning to tools like Replay, which records test failures for a more efficient debugging process. However, merely fixing failing tests is not enough. Periodic review and revision of existing tests, like Shopify's comprehensive review of decade-old tests, ensures that all tests remain relevant and effective. While unit tests are invaluable for their ease and speed of debugging, they may not provide sufficient coverage, especially in areas where modules interact. Therefore, integration tests are often crucial for ensuring overall application quality.
Test automation is another strategy that can significantly improve software quality by identifying bugs earlier in the development cycle. This approach allows for more test scenarios to be covered and tests to be run more frequently, enhancing the reliability of software applications and boosting customer satisfaction. To further ease the process of unit testing, platforms like Machinet.net have emerged. This platform aids Java developers in automating the writing of unit tests using Mockito, thereby improving productivity and ensuring code correctness. It provides resources such as mockito mocks, unit testing tips, and techniques, and best practices for Java unit testing. It also offers features like an AI assistant for developers, emphasizing code quality, reducing bugs, and delivering robust and reliable software. In essence, fixing failed tests, though challenging, is a vital part of software development. It involves not only debugging and updating assertions but also ensuring that tests continue to serve their purpose and contribute to the overall quality of the software.
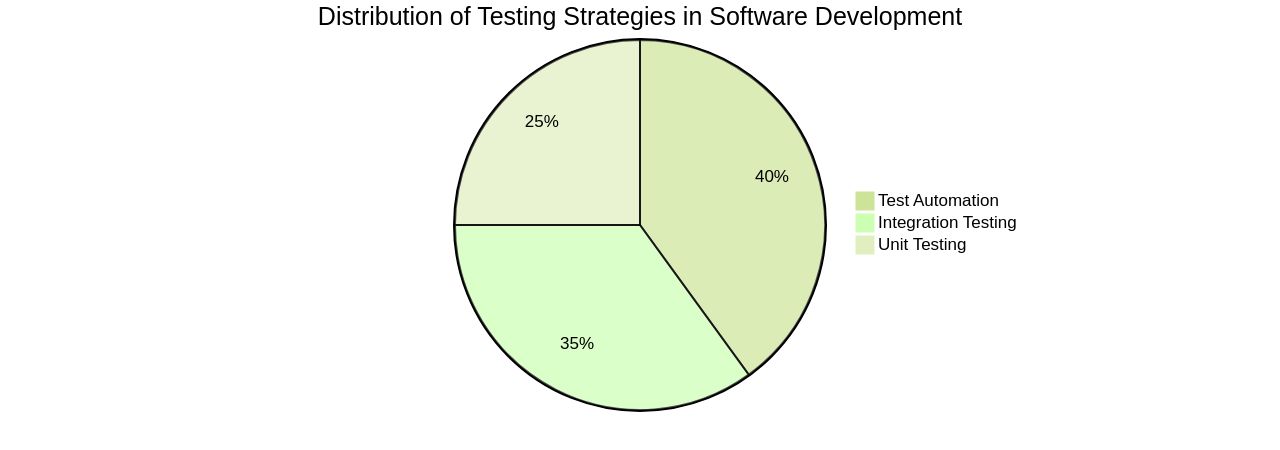
Improving Code with Unit Tests
Unit tests are a vital asset in the software development process, playing a key role in maintaining and enhancing code quality. They adhere to the Arrange, Act, and Assert (AAA) method, which divides the test into three stages: setting up the test data, performing the action, and verifying the results. This approach ensures tests align with the code's expected behavior, mitigating the risk of false positives. Unit tests also foster highly testable code, crucial for efficient defect detection. However, it is essential to avoid tight coupling in the code, where changes in one segment might impact other parts, causing a cascade of bugs. While unit tests are essential, they also pose challenges. They can be brittle, overemphasize specific details, and possibly miss integration issues.
Managing a large number of unit tests can also be challenging. Research shows that only 10% of teams release at least daily due to testing often causing delays. This emphasizes the need for successful continuous testing strategies, including unit testing, to maintain confidence in code quality. Machinet.net is a platform dedicated to unit testing in software development, especially in Java. It offers an AI assistant for developers and features such as code quality and unit test generation. The platform emphasizes catching and fixing issues early in the development cycle, reducing the likelihood of bugs, and delivering robust software. It also provides resources on JUnit annotations and assertions for effective and efficient unit testing. Machinet.net offers various resources, including ebooks, demos, and use cases, and encourages individuals to join their team to shape the future of AI software.

Analyzing Issues
Unit tests are a crucial part of software development, assisting in identifying performance bottlenecks, resource leaks, and security vulnerabilities. However, it's important to note that while unit tests are beneficial for debugging due to their simplicity and speed, they often fall short in covering the interconnectivity between modules. In fact, many complex bugs emerge in these interconnections, emphasizing the importance of integration tests for overall application quality. Static code analysis, a process of scrutinizing code without executing it, is another powerful tool in a developer's toolbox. While limited in scope to unit and technology levels, it's excellent for 'microscope' analysis, catching unused variables and overcomplicated expressions. However, it's worth mentioning that these tools are less effective at the integration level, especially with third-party tools or libraries. Interestingly, some developers have found success with generated tests, boasting an acceptance rate of 75%, significantly exceeding the anticipated 40-50% range.
The high acceptance rate suggests that these tests are both accurate and useful, alleviating the often time-consuming task of writing tests. However, it's worth noting that testing is not a panacea. As some developers have pointed out, throwing more resources at testing doesn't necessarily result in bug-free code. It's also possible to write biased tests that conform to the code's current behavior, leading to false positives. Furthermore, overemphasis on unit tests can lead to dead code and added complexity. In conclusion, while unit tests, profiling tools, static analysis tools, and code coverage analysis are all valuable, they are part of a larger picture. A structured approach to debugging, effective communication, and a robust testing strategy are all essential components of efficient and high-quality software development.
Creating and Running New Test Methods
In the realm of software evolution, the addition of new features and functionalities often calls for the creation of more unit tests. This can be quite an intimidating task, particularly when working with a pre-existing project that lacks complete test coverage. It's not feasible to pause development until every aspect of the project is exhaustively tested. A more pragmatic strategy is to develop the requested features or bug fixes simultaneously while implementing tests for the specific components being altered. Over time, this will result in a large part of the codebase being covered by tests, thereby assuring code quality. This process, however, presents a challenge when these test suites demand significant infrastructure resources and time to complete. The answer to this lies in running only those tests that were affected by corresponding changes in the test repository. This method can be applied not only to Python-written frameworks and libraries but can also be generalized to other programming languages. Machinet.net, a platform dedicated to software development with a focus on unit testing and AI operations, offers a solution to this challenge.
With features such as code quality and unit testing tips, Machinet.net eases the process of generating effective and efficient unit tests. Its AI assistant aids developers in automating the unit testing process, making it applicable across various use cases including security and pricing. The ultimate goal of software testing is to ensure that the application functions as intended and meets the set requirements. This includes ensuring compatibility across different operating systems and hardware devices, identifying potential risks and vulnerabilities, and detecting defects early in the development process. Software testing is not just about identifying errors; it's about ensuring that the product will perform well under all conditions and will be well-received by its intended users. Code Coverage, a form of White Box Testing that measures the percentage of code that is tested, is fundamental in testing the quality of the test suite. A program with high test coverage is likely to have fewer bugs than a program with low test coverage. Lastly, empirical data plays a key role in illustrating the business advantage of a well-maintained codebase. Code that is easy to understand and maintain (Green Code) allows for a faster implementation of capabilities compared to unhealthy code (Red Code), providing businesses with a competitive advantage. Machinet.net's platform, with its focus on unit testing and AI operations, can be instrumental in achieving this.

Refactoring the Code Under Test
Refactoring is a key part of software development, allowing developers to enhance existing code without changing its external functionality. This process refines the structure of the codebase, making it more understandable, testable, and extendable. A prime example of this can be seen in the 'CustomerService' class, where validation logic might be dispersed and challenging to maintain. Refactoring can help extract this logic into a separate method, enhancing readability and maintainability. Dependency injection (DI) is a design pattern that promotes the principle of Inversion of Control (IoC), encouraging a separation of concerns. This principle is vital for testability, allowing each part of the codebase to be tested separately. By segmenting the code into sections like business logic, user interface, and data access, developers can isolate problems more efficiently and make changes without unexpected outcomes. Unit tests are essential in verifying the functionality of refactored code.
However, writing tests after the code can lead to biased tests that align with the code's current behavior, potentially resulting in false positives. Writing tests first can solidify requirements before implementation, leading to a more focused and effective implementation. This approach ensures that testing is an integral part of the development process, leading to higher test coverage and more reliable code. Machinet.net is a platform that supports these practices, providing resources and tools to improve code quality and productivity through unit testing. It emphasizes the importance of unit testing in catching and fixing issues early in the development cycle, ensuring reliable software delivery. The platform also offers an AI assistant that aids developers with automated unit test generation and understanding concepts like mocking. In the context of refactoring, it's crucial to remember that it involves making many small changes across a codebase. Therefore, adopting Continuous Integration techniques and merging your branch into the mainline at the end of each day can pinpoint exactly which refactoring caused an issue, enabling an easy rollback when a test fails.

Refactoring Test Methods
Refactoring test methods is essential in maintaining their efficiency, just as it is with the code under scrutiny. Refactoring techniques are pivotal for optimizing the structure of your codebase, which improves readability, testing, and extendibility. For instance, the validation logic in a CustomerService class may be scattered throughout the AddCustomer method. This can make it hard to maintain and test, but by extracting the validation logic into a separate method, we can improve readability and maintainability. Test-driven development (TDD) is a popular approach where tests are written before the code they are meant to validate. This process clarifies the purpose and expected behavior of the code, leading to more focused and effective implementation. Writing tests first also ensures higher test coverage, resulting in more reliable code. However, writing tests after the code can lead to overlooked aspects of implementation and potentially lower test coverage.
There's also the risk of biased tests that conform to the code's current behavior, rather than independently calculating the expected output. This could result in false positives. In terms of testability in code, key characteristics include modularity, clarity, and independence. Code that is organized into discrete units, understandable, and can be tested in isolation without reliance on external systems or states is highly testable. However, tight coupling in code can be a barrier to achieving highly testable and maintainable code. If a change in one part of the code impacts or breaks the functionality in another part, this indicates a high degree of coupling. Finally, the concept of separation of concerns is crucial for testability. This design principle suggests dividing the code into sections such as business logic, user interface, and data access. This allows for each part of the codebase to be tested independently, isolating problems more effectively and minimizing unintended consequences from changes.
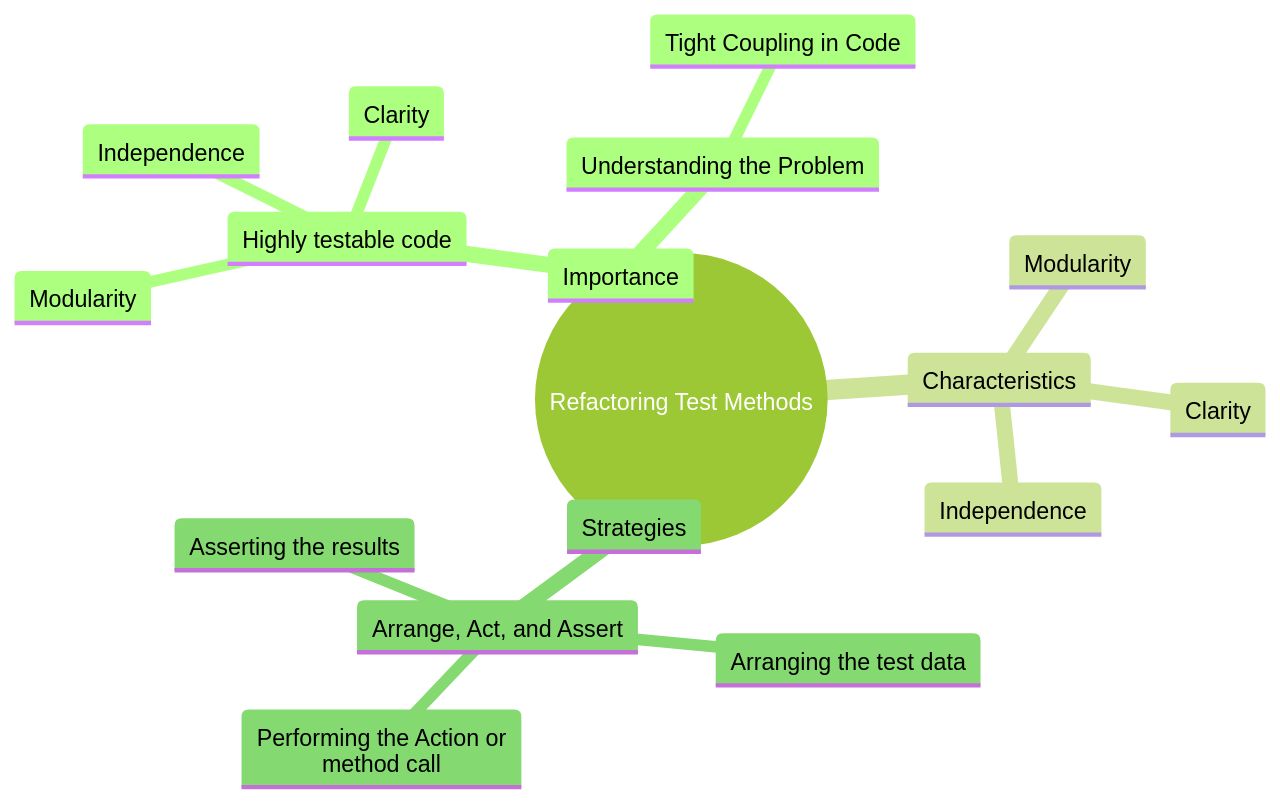
Retesting, Rewriting, and Reanalyzing
Unit testing is not a static process, but a dynamic one that evolves in tandem with the codebase. As the codebase expands and transforms, so too must the unit tests adapt to remain effective. It's crucial to stay vigilant in retesting, rewriting, or reanalyzing tests to ensure they align with the current state of the codebase. This task, however, may prove labor-intensive, particularly for those who are inexperienced. Undergoing this process may also cause disruptions in the user experience. Changes in interface, functionality, or performance can cause confusion or frustration among end users. Therefore, it's essential for product managers to lead the effort in defining the functional requirements needed in the new platform. The efficiency and accuracy of the testing process can significantly be enhanced by automating regression testing, especially for large and complex applications. However, there is always a possibility of biased tests when tests are written after the code.
This can lead to false positives and a reduced emphasis on API design. In the context of testability, the principle of separation of concerns is critical. It allows each part of the codebase to be tested independently, enabling developers to isolate problems more effectively and make changes without unintended consequences. The key to successful unit testing is thoroughness. Adhering to this principle ensures the quality, reliability, and overall effectiveness of the software being tested. This can also improve user satisfaction by ensuring compatibility across different systems and hardware devices, and by identifying potential risks and vulnerabilities early. However, the retest process can be costly and time-consuming, and it may not be justifiable for minor changes in the software. Thus, in the face of time constraints, a manual approach might be the best option. Remember, the goal is to maintain high confidence in your software through effective and efficient testing.
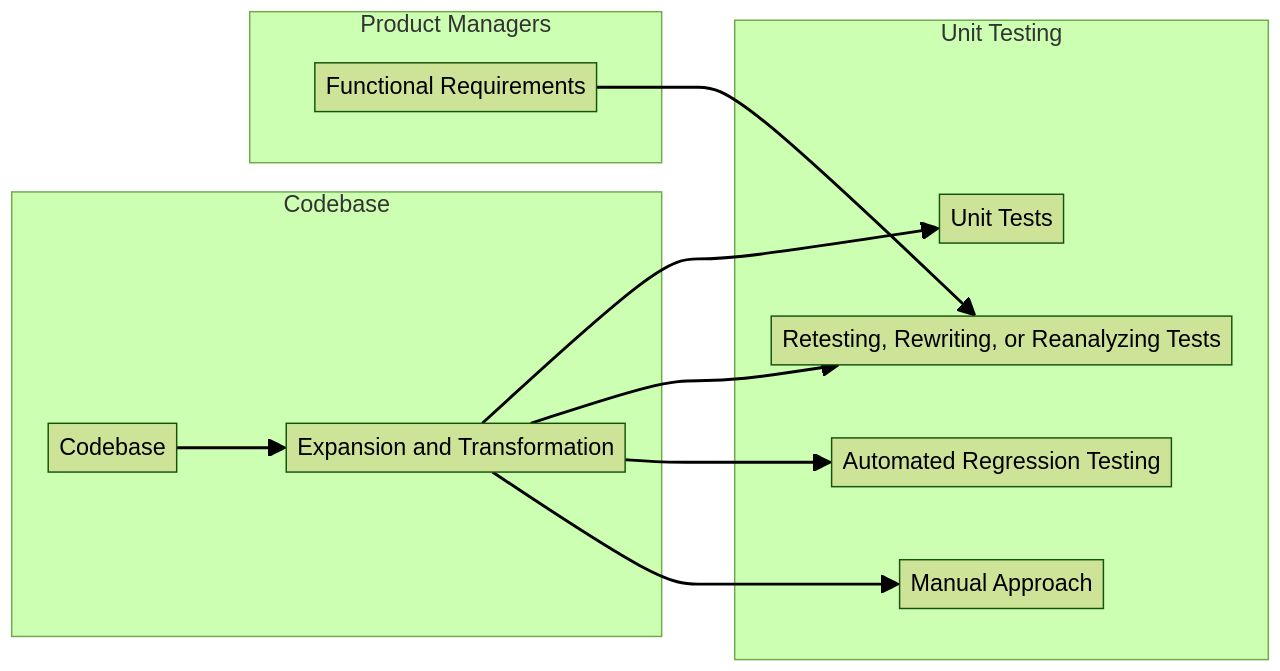
Conclusion
In conclusion, unit testing plays a crucial role in software development by detecting and correcting bugs early, improving code quality, and ensuring the smooth functioning of new features or changes. However, it is often overlooked due to time constraints or a rush to market. Machinet.net offers a solution to these challenges with its AI assistant for developers. It automates unit test generation, simplifies mocking, and effectively structures and isolates dependencies in tests. By emphasizing the significance of testing processes early in the development cycle, Machinet.net helps reduce the risk of new bugs and deliver robust software. Unit testing acts as a safety net, ensuring software reliability and contributing to user satisfaction.
It is an essential tool for producing robust and error-free software solutions. The broader significance of unit testing lies in its ability to minimize bug-fixing costs, prevent complications, and reduce the total cost of ownership. It also improves code quality and fosters strong cooperation between developers and testers. Unit testing frameworks automate performance testing and help segregate application code into manageable portions for individual testing. As software development evolves rapidly, unit testing becomes even more crucial in maintaining stability, ensuring compatibility across different systems, reducing risks, and enhancing user satisfaction. To harness the benefits of unit testing, developers are encouraged to start now with Machinet.net's AI assistant for automated unit test generation Start now.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.