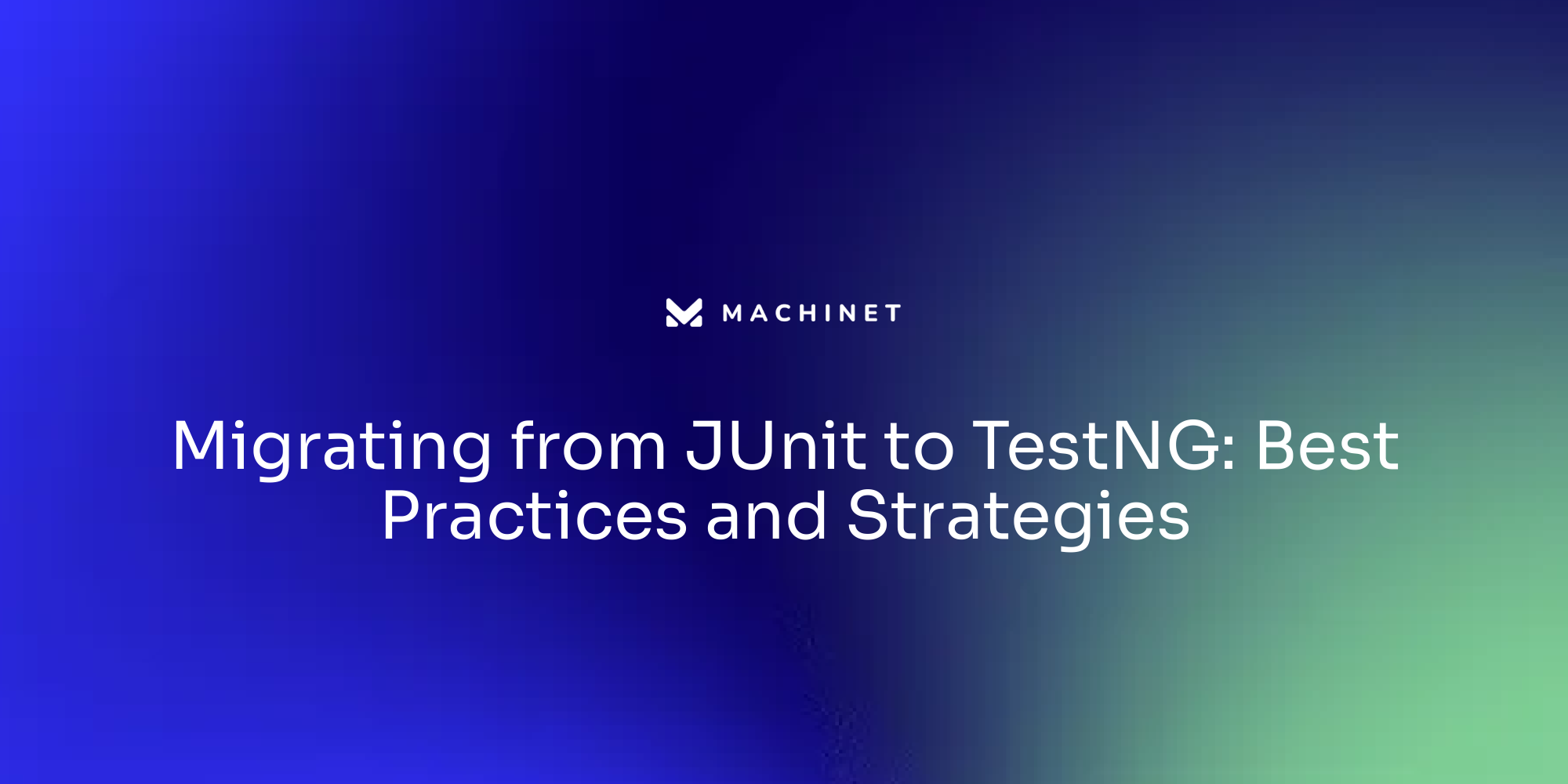
Table of Contents
- Understanding the Need for Migration: JUnit vs TestNG
- Preparing for Migration: Assessing Current JUnit Test Suites
- Setting up TestNG Environment: Installation and Configuration
- Converting JUnit Tests to TestNG: Practical Steps
- Strategies for Seamless Execution of JUnit and TestNG Tests Simultaneously
- Dealing with Challenges During Migration: Tips and Solutions
- Best Practices for Maintaining and Optimizing TestNG Tests After Migration
- Case Study: Successful Migration from JUnit to TestNG in a Large-Scale Project
Introduction
The migration from JUnit to TestNG is a process that many developers undertake to enhance their testing capabilities and take advantage of the advanced features offered by TestNG. This article explores the need for migration from JUnit to TestNG and provides practical tips and solutions for a successful transition. It discusses the benefits of TestNG over JUnit, such as support for data-driven testing, parallel test execution, and flexible test configuration. The article also covers the process of assessing current JUnit test suites, setting up the TestNG environment, converting JUnit tests to TestNG, and strategies for executing JUnit and TestNG tests simultaneously. Additionally, it addresses the challenges faced during migration and offers best practices for maintaining and optimizing TestNG tests after migration. A case study of a successful migration from JUnit to TestNG in a large-scale project is presented to demonstrate the benefits and outcomes of the migration process. Overall, this article provides valuable insights and guidance for developers looking to migrate from JUnit to TestNG and optimize their testing processes
1. Understanding the Need for Migration: JUnit vs TestNG
The switch to TestNG from JUnit is often driven by the need for enhanced testing functionalities. TestNG, a next-gen testing framework, introduces several capabilities that JUnit lacks, such as support for data-driven testing, parallel test execution, and a more flexible approach to test configuration. TestNG also boasts a robust execution model with features like test groups, dependencies, and priorities, making it a more fitting choice for complex, large-scale projects that require advanced testing approaches.
Influenced by JUnit and NUnit frameworks, TestNG surpasses JUnit 5 in parameterized testing, suite testing, and dependency testing. It provides features like test annotations, prioritization, grouping, and parameterization that simplify the process of writing and maintaining test cases, allowing for a higher degree of control over test cases and their execution.
TestNG's annotation system includes @BeforeMethod
, @AfterMethod
, @BeforeClass
, @AfterClass
, @BeforeTest
, @AfterTest
, @BeforeSuite
, @AfterSuite
, @BeforeGroups
, @AfterGroups
, and @Test
. These annotations provide extra information about test methods or classes. Moreover, TestNG allows for the grouping of tests using XML configuration, in contrast to JUnit 5's annotation-based grouping using @Tag
.
TestNG supports parameterized testing using the @DataProvider
annotation, allowing you to pass multiple sets of test data to a test method and execute it multiple times with different data inputs. Here's an example of data-driven testing with TestNG using the @DataProvider
annotation:
```javaimport org.testng.annotations.DataProvider;import org.testng.annotations.Test;
public class DataDrivenTest {
@DataProvider(name = "testData") public Object[][] testData() { return new Object[][] { { "Data1" }, { "Data2" }, { "Data3" } }; }
@Test(dataProvider = "testData") public void testMethod(String testData) { System.out.println("Test Data: " + testData); // Perform test steps using the test data }}```
In this example, the testData
method returns a 2D array of test data, and the testMethod
is annotated with @Test
and dataProvider = "testData"
. The testMethod
will be executed three times, each time with a different set of test data ("Data1", "Data2", "Data3").
TestNG also allows for setting the order of test execution using the priority
attribute in the @Test
annotation. By assigning different priority values to your test methods, you can control the order in which they are executed. Test methods with lower priority values will be executed first.
Another feature of TestNG is the creation of test dependencies using the dependsOnMethods
attribute in the @Test
annotation. This attribute allows you to specify which test methods should be executed before the current test method. By using dependsOnMethods
, you can ensure that certain tests are executed in a specific order, based on their dependencies.
While TestNG does not offer built-in support for customizing test names, it does support parallel test execution using the parallel attribute in the testng.xml file. To execute tests in parallel using TestNG, you can use the "parallel" attribute in the TestNG XML configuration file. This attribute allows you to specify the level of parallelism for test execution. TestNG provides three options for parallel execution: "none" - No parallel execution, "methods" - Parallel execution at the method level, and "tests" - Parallel execution at the test level. By setting the "parallel" attribute to either "methods" or "tests", TestNG will automatically create and manage threads for parallel test execution. You can also specify the number of threads to be used for parallel execution using the "thread-count" attribute, allowing you to control the level of concurrency in your test execution.
TestNG also offers built-in HTML reports generated after test execution, a feature JUnit 5 lacks.
Try TestNG's HTML reports for comprehensive test result analysis.
However, JUnit 5 can be integrated with plugins to generate reports. The decision between JUnit 5 and TestNG often depends on the project requirements and the features each provides. Both can be used for parallel test execution and offer options for running tests in parallel across different browsers. They can also be integrated with the LambdaTest Selenium Grid for parallel test execution.
In conclusion, both JUnit 5 and TestNG can be employed for a variety of tasks in Selenium automation, including cross-browser testing, parameterizing tests, grouping tests, prioritizing tests, creating test dependencies, and generating reports
2. Preparing for Migration: Assessing Current JUnit Test Suites
The initial step in the migration process is a thorough evaluation of your existing JUnit test suites. This process entails a detailed examination of your current tests, identifying any dependencies, and gaining a deep understanding of your test suites' structure and organization.
The complexity of the tests and the degree of their coverage are significant aspects to consider in this process. Unit testing forms a crucial part of software development, ensuring the quality and reliability of individual code components. It aids in catching and preventing bugs, refactoring and maintaining code, and providing living documentation.
Integrating unit testing into the development process and using automated testing tools helps assure the delivery of high-quality software products. Automated unit testing tools offer a wealth of benefits, including test frameworks and runners, code coverage analysis, integration with CI/CD pipelines, mocking and test doubles, parameterized testing, test execution reporting, test data management, test prioritization, and integration with IDEs.
Evaluating the current state of your unit tests will provide invaluable insights into the breadth of the migration and will assist in creating an effective strategy for the migration process. Remember, manual unit test writing involves arranging, acting, and asserting the expected outcomes. Effective unit testing requires adhering to best practices, maintaining test coverage, refactoring tests, integrating tests with CI, reviewing and collaborating, and balancing the quantity and quality of tests.
In essence, this initial assessment will streamline the development process, promote continuous testing and improvement, and enhance code reliability and maintainability.
When assessing your JUnit test suites for migration, it's important to carefully review the test cases and evaluate their compatibility with the target environment. This includes considering any dependencies, frameworks, or specific features used in the current test suite that may require modifications or updates during migration.
Analyzing the coverage and effectiveness of the existing test suite can help identify areas that may need improvement or additional testing in the new environment. It's also crucial to examine the test coverage to ensure that all critical parts of the code are being tested.
Review the test naming conventions for clarity, and check for any duplicated or redundant tests. Furthermore, analyze the use of test fixtures to ensure they are properly set up and torn down to avoid any side effects between tests. Lastly, consider the readability and maintainability of the tests, ensuring they are easy to understand and modify if needed.
The structure of JUnit test suites in Java typically involves the use of test classes and test methods, with annotations like @Test
, @Before
, @After
, @BeforeClass
, and @AfterClass
to define the test methods and their execution order. These annotations allow for the setup and teardown of resources before and after each test method or the entire test suite.

To measure test coverage in JUnit test suites, tools like JaCoCo can be used, which provides a code coverage library for Java applications. JaCoCo can be integrated into your JUnit test suites to measure the code coverage of your tests and can generate reports that provide detailed information about the coverage achieved by your tests.
Finally, to plan the migration process for JUnit tests, consider the current state of the JUnit tests and identify any dependencies or frameworks that are being used. Research and understand the capabilities, syntax, and any differences in testing methodologies of the target framework or testing tool that you want to migrate to. Create a migration plan that outlines the steps involved in the migration process. This plan should include tasks such as identifying and updating test dependencies, rewriting test cases using the new framework or tool, and ensuring that the migrated tests are still able to provide the same level of coverage and functionality as the original JUnit tests.
In conclusion, assessing the effectiveness of a JUnit test suite involves ensuring that the test suite provides comprehensive coverage of the code being tested, is easily maintainable and modular, regularly reviewed and updated, and provides clear and informative feedback on test results
3. Setting up TestNG Environment: Installation and Configuration
Setting up TestNG, a vibrant Java application testing framework, requires a few key steps. First, you need to ensure the Java Development Kit (JDK) is installed on your machine. You can check this by running the "java version" command in the console. The JAVA_HOME environment variable should be set to point to the base directory where Java is installed, and the Java compiler location should be appended to your system path.
Downloading the TestNG jar file from the TestNG website is the next step. You should set the TESTNG_HOME environment variable to the directory where the TestNG jar is stored. Also, the classpath environment variable should be set to the TestNG jar location.
To install TestNG using Maven, add the TestNG dependency to your project's pom.xml file, as shown below:
xml<dependency> <groupId>org.testng</groupId> <artifactId>testng</artifactId> <version>7.4.0</version> <scope>test</scope></dependency>
If you're using Gradle, the necessary dependencies should be added to your build.gradle file:
dependencies { testCompile 'org.testng:testng:<version>'}
Replace <version>
with the version of TestNG you wish to install.
TestNG can also be installed in IDEs such as Eclipse, NetBeans, and IntelliJ. For instance, in your IDE, you can navigate to the "Help" menu, select the option to install new software or plugins, and follow the prompts to complete the installation.
To align TestNG with project specifications, you can utilize the TestNG XML configuration file. This file allows you to define various settings and configurations for your tests, like specifying the test classes or packages to include in the test suite, setting the test execution order, defining test groups, configuring test listeners, and more.
Test groups can be created to organize and execute tests based on different criteria such as functionality, priority, or environment. TestNG provides the "groups" attribute in the @Test annotation for this very purpose.
Test listeners can also be configured using the @Listeners
annotation. Listener classes, which can implement various interfaces provided by TestNG, can be invoked during the test execution to perform tasks like logging test results, capturing screenshots, or generating custom reports.
You can integrate TestNG with your continuous integration pipeline using tools like Jenkins, Travis CI, or CircleCI. Configure your CI pipeline to trigger a build whenever changes are pushed to your version control system and to execute TestNG tests as part of the build process.
While setting up, pay attention to the JDK version requirement. For TestNG, JDK version 17 or above is needed. The TestNG jar file version used in this tutorial is 7.4. The TestNG environment variable "TESTNG_HOME" should be set to the base directory location where the TestNG jar is stored, and the classpath environment variable should be set to the TestNG jar location.
Finally, be sure to troubleshoot common issues in TestNG installation and configuration. Verify that the TestNG library is properly installed and configured in the project, check for any mismatch between the TestNG version and the project dependencies, and review the TestNG XML file for errors or inconsistencies. Also, ensure that all required dependencies are included in the classpath settings and that the project build and compilation settings are correct.
By following these steps, you can ensure a smooth and successful setup of your TestNG environment
4. Converting JUnit Tests to TestNG: Practical Steps
Transitioning from JUnit to TestNG involves a methodical process that calls for a thorough understanding of both frameworks. The first step of this process is replacing JUnit annotations with their TestNG counterparts. To illustrate, the JUnit @Test
annotation is replaced with TestNG's @Test
annotation, and @Before
in JUnit is replaced with @BeforeMethod
in TestNG. Additionally, @After
in JUnit is replaced with @AfterMethod
in TestNG, and @Ignore
in JUnit is replaced with @Test(enabled = false)
in TestNG. These replacements are crucial as they mark the methods as test cases and indicate which methods should be run before and after each test.

The following step involves recognizing and substituting JUnit-exclusive features, such as assertions or test runners, with alternatives provided by TestNG. TestNG provides a more robust and flexible assertion framework with a wide range of assertion methods that can be used to verify the expected behavior of the code under test. These assertions can be utilized to check conditions such as equality, inequality, nullity, and exception handling. TestNG also provides advanced features like grouping and dependency management, which are useful in organizing and controlling the execution of test cases.
The final step of the transition process is reconfiguring the tests to fit into TestNG's suite and test structure. While this may seem challenging, tools like the junit-to-testng converter can significantly simplify this task. The converter automates the conversion process, saving valuable time and effort. The process involves identifying the JUnit tests to be converted, installing and configuring the converter, running the converter, and verifying the converted tests.
TestNG has gained significant traction among developers and is widely used, as shown by data from GitHub where it has over 19K stars and is being used by over 463,072 users. Open for contributions and encouraging pull requests for new features and bug fixes, TestNG demonstrates its adaptability and continuous growth.
Transitioning from JUnit to TestNG, while requiring technical knowledge and effort, can be made more efficient through the use of resources and tools such as the junit-to-testng converter. The growing popularity and active contributions to TestNG make it a viable and promising alternative for unit testing in Java
5. Strategies for Seamless Execution of JUnit and TestNG Tests Simultaneously
Transitioning between testing environments, such as JUnit to TestNG, requires the concurrent operation of multiple test types. A common approach to accomplish this is to adapt the build system to run both test types simultaneously. For instance, in a Maven setting, you can establish separate profiles for both JUnit and TestNG, which allows for their concurrent execution within the same build process.
To configure Maven to run JUnit and TestNG tests concurrently, you can utilize the Maven Surefire Plugin. This plugin facilitates the simultaneous execution of both JUnit and TestNG tests within the same Maven build. To set up the Surefire Plugin for running JUnit and TestNG tests concurrently, you must configure the plugin in the project's pom.xml file. You can do this by adding the appropriate dependencies to the <dependencies>
section of the pom.xml file.
For JUnit, you can add the following dependency:
xml<dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.12</version> <scope>test</scope></dependency>
For TestNG, you can add the following dependency:
xml<dependency> <groupId>org.testng</groupId> <artifactId>testng</artifactId> <version>7.3.0</version> <scope>test</scope></dependency>
Once the dependencies are added, you can configure the Surefire Plugin to run both JUnit and TestNG tests by adding the following configuration to the <build>
section of the pom.xml file:
xml<plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-surefire-plugin</artifactId> <version>3.0.0-M5</version> <configuration> <includes> <include>**/*Test.java</include> <include>**/*Tests.java</include> </includes> </configuration> </plugin></plugins>
This configuration instructs the Surefire Plugin to include all JUnit and TestNG test classes that match the specified patterns. With this setup, Maven can run both JUnit and TestNG tests concurrently during the build process. You can use the command mvn test
to execute the tests, which will run all the JUnit and TestNG tests in the project. The test results will be displayed in the console and also saved in the target/surefire-reports directory.
Alternatively, you can leverage TestNG's JUnit compatibility mode. This mode allows JUnit tests to be executed within a TestNG environment, which is particularly beneficial during a phased migration. This strategy enables the incremental conversion of JUnit tests to TestNG, while maintaining the ability to execute all tests concurrently. To use TestNG's JUnit compatibility mode for running JUnit tests, you need to ensure that you have TestNG and JUnit dependencies added to your project, create a new TestNG XML configuration file, specify the classes or packages containing your JUnit tests using the <class>
or <package>
tags, set the attribute junit="true"
for the <test>
tag to enable JUnit compatibility mode, and then run the TestNG XML configuration file as you would typically run a TestNG test suite.
The principle of unit testing is vital to software development, as it verifies the proper functionality of individual code components and their seamless integration within larger applications. In this context, unit tests act as an early warning system, aiding in the detection of bugs and issues within the codebase before they escalate into significant problems.
Moreover, unit tests provide a safety net for code refactoring and maintenance, ensuring that the existing functionality remains intact. They also act as living documentation, describing the expected behavior of the code and providing up-to-date information that traditional written documentation may lack. Therefore, by running JUnit and TestNG tests simultaneously during a migration process, it ensures that the unit tests continue to perform their crucial role effectively.
Automated unit testing tools, such as JUnit and TestNG, are instrumental in streamlining the unit testing process and promoting continuous testing and improvement. These tools integrate with IDEs, provide test execution and reporting capabilities, and support parameterized testing and test data management. Therefore, the ability to run both JUnit and TestNG tests concurrently during a migration process is a testament to the flexibility and versatility of these automated unit testing tools
6. Dealing with Challenges During Migration: Tips and Solutions
The pathway from JUnit to TestNG can seem labyrinthine, littered with potential pitfalls and complexities. One of the primary challenges lies in navigating JUnit-specific features that don't have direct counterparts in TestNG. In addition, the management of dependencies between tests and ensuring that the tests run correctly in the new environment can feel like a Herculean task.
The key to a seamless transition lies in understanding the distinct features and disparities between JUnit and TestNG. Moreover, to replicate equivalent functionality, it becomes essential to fully harness the advanced features of TestNG. But the journey doesn't end here, comprehensive testing and validation must be carried out to ensure that the migrated tests yield the same results as before.
Communities such as SmartBear and Stack Overflow can be valuable allies in this process. SmartBear, with its vast member base, offers an array of open-source tools and resources. Stack Overflow, known for its developer-friendly environment, is a treasure trove of knowledge and solutions to common issues faced during migration.
For example, the topic of running TestNG tests on Jenkins, a popular automation server, has been extensively deliberated on Stack Overflow. Users have exchanged experiences and sought assistance on this subject. Several solutions have been proposed, including the use of Ant scripts, Gradle, and custom batch commands. Challenges like configuring Jenkins, specifying the JDK path, and setting up custom workspaces have also been discussed.
Users have also shared insights on running TestNG tests as Ant tasks and reporting test failures back to Jenkins. They have recommended Gradle and TestNG documentation for more in-depth information. Plugins like TestNG, ReportNG, and HTML Publisher have been suggested for improved reporting and formatting of test results in Jenkins.
Migration from JUnit to TestNG is no small feat, but by understanding the differences between the two, leveraging TestNG's advanced features, and conducting extensive testing and validation, this challenge can be met head-on. Utilizing resources from platforms like SmartBear Community and Stack Overflow can provide additional support and solutions to common issues encountered during the process.
Join the SmartBear Community and Stack Overflow to get expert advice on JUnit to TestNG migration.
To tackle JUnit-specific features in TestNG, the TestNG JUnit compatibility layer comes in handy. This allows JUnit tests to run using the TestNG framework, enabling the use of TestNG's advanced features while working with existing JUnit tests. This compatibility layer is instrumental in ensuring a smooth migration from JUnit tests to TestNG without losing any functionality.
Managing test dependencies in TestNG can be achieved using the dependsOnMethods
attribute in the @Test
annotation. This attribute allows specification of which test methods should be executed before a particular test method, ensuring that dependencies are met before running a specific test.
It is crucial to ensure that tests run correctly post-migration. Best practices like updating necessary dependencies, ensuring proper test environment configuration, and conducting thorough testing to identify and resolve any issues are recommended. Having a comprehensive test suite to cover all aspects of the migrated system is beneficial, and running these tests regularly ensures ongoing correctness.
Leveraging TestNG's advanced features like data providers, parameterization, and dependency management can help achieve equivalent functionality. Data providers allow test data from external sources to be used, parameterization enables passing parameters to test methods, and dependency management allows control over the execution order of test methods. Using these features effectively ensures efficient and effective testing of your code.
The challenges of migrating from JUnit to TestNG may seem daunting, but understanding the differences between the two frameworks and careful planning can help overcome them. Whether it's adapting to different annotations and assertions, understanding the different test execution flow, or integrating TestNG with existing build systems, with careful planning and understanding, these challenges can be navigated successfully
7. Best Practices for Maintaining and Optimizing TestNG Tests After Migration
In the process of optimizing and maintaining tests after migration, TestNG proves to be a crucial tool. It allows for the segregation of tests into distinct groups, which enhances the management efficiency. This can be achieved by using the "groups" attribute in TestNG and assigning tests to specific groups. This allows for running specific groups of tests based on certain criteria, making management and execution easier. Additionally, the "dependsOnGroups" attribute can be utilized to define dependencies between different test groups, ensuring tests are executed in the desired sequence.
Data-driven testing is made possible with TestNG's data providers. A data provider method can be defined in the test class, returning a two-dimensional object array where each row represents a set of test data. The test method is then annotated with the @DataProvider
annotation and the name of the data provider method is specified. TestNG automatically invokes the data provider method and passes the test data to the test method for each iteration. This allows for running the same test method with different input data, enhancing the maintainability and reusability of tests.
Parallel execution is another powerful feature of TestNG that can be harnessed to reduce test execution time. By configuring TestNG to run tests in parallel and specifying the desired parallel execution mode, tests can be distributed across multiple threads or processes. This efficient utilization of computing resources significantly reduces the overall test execution time, especially when dealing with large test suites.
The quality of the tests is maintained through regular code reviews. This practice helps identify potential issues, ensures best practices are adhered to, and improves the overall quality of tests. Early detection of mistakes allows for necessary improvements, leading to more reliable and efficient tests.
The implementation of continuous integration is also instrumental in automatic execution of tests with every code modification. Tools such as Jenkins, Travis CI, or GitLab CI/CD can be utilized to automate the testing and deployment process. This ensures that tests are run consistently and timely, leading to improved code quality and faster development cycles.
TestNG's reporting capabilities equip you with the ability to generate comprehensive test reports, which are instrumental for analysis and troubleshooting. This is achieved by specifying the appropriate listeners in the TestNG XML configuration file. Detailed HTML reports generated provide valuable insights into test execution, aiding in identifying issues and making informed decisions about the test suite.
In the broader industry context, software quality assurance plays a critical role in various sectors including communications, fintech, banking, hardware, IoT, security, authentication, travel, hospitality, healthcare, gaming, and artificial intelligence. A company like TestDevLab, for instance, offers a wide array of services including QA management, performance testing, test automation, manual testing, accessibility testing, ISO advisory services, security testing, UX testing, and more.
Their expertise extends to testing platforms such as mobile apps, web apps, desktop apps, APIs, SDKs, and AR/VR. They also provide audio/video quality testing, location testing, and battery and data usage testing in their laboratories. Products like Loadero and TestRay, developed by TestDevLab, underscore the importance of software quality assurance and the need for effective QA strategies. This philosophy aligns with the approach outlined above for managing and optimizing TestNG tests after migration
8. Case Study: Successful Migration from JUnit to TestNG in a Large-Scale Project
The success story of a large-scale project migrating from JUnit to TestNG serves as a testament to the benefits of transitioning between these testing frameworks. The project team underwent a thorough review of their existing JUnit tests and devised a detailed plan for the migration process.
In the journey from JUnit to TestNG, it's crucial to understand the differences between the two frameworks in terms of annotations and assertions used for unit testing. The team refactored their existing JUnit tests to align with TestNG's features and capabilities, modifying test case methods, handling dependencies between test methods, and utilizing TestNG's advanced features such as parameterization and grouping.
Their use of an automated conversion tool, junit-to-testng, expedited the process by handling the batch conversion of tests, annotations, and assertions, while maintaining the overall structure of the tests. This tool was vital in ensuring a seamless transition, eliminating the need for manual effort, and saving valuable time.
To enable concurrent execution of JUnit and TestNG tests during the migration phase, the team adjusted their build system. They used a build tool, such as Maven or Gradle, configuring the dependencies, build configurations, and test execution settings in the project configuration file. This configuration allowed them to run both JUnit and TestNG tests simultaneously and review the combined test results, providing a comprehensive view of their project's test coverage.
The team leveraged TestNG's advanced features to implement intricate testing strategies that were unfeasible with JUnit. The migration resulted in a more robust and adaptable test suite, significantly enhancing the project's testing capabilities.
In a parallel narrative, the Georgia Lottery Corporation (GLC) also underwent a significant transition. They adopted SmartBear's QAComplete and TestComplete Web solutions to automate their testing processes, leading to enhanced application usability and performance.
Prior to this implementation, the GLC was releasing only one or two software updates per quarter. However, after implementing SmartBear's solutions, the number of quarterly releases increased dramatically to 10 or more. The solutions not only accelerated the testing of web and mobile applications but also expedited the software development process, leading to a five-fold increase in application rollouts.
Just like the migration from JUnit to TestNG, the deployment of SmartBear's solutions at the Georgia Lottery Corporation exemplifies how a well-planned and executed transition can drastically bolster an organization's testing capabilities and overall efficiency
Conclusion
The migration from JUnit to TestNG offers developers the opportunity to enhance their testing capabilities and take advantage of the advanced features provided by TestNG. TestNG provides support for data-driven testing, parallel test execution, and flexible test configuration, making it a more suitable choice for complex, large-scale projects. The process of migration involves assessing current JUnit test suites, setting up the TestNG environment, converting JUnit tests to TestNG, and executing both JUnit and TestNG tests simultaneously. By migrating to TestNG, developers can optimize their testing processes and improve the reliability and efficiency of their software.
In conclusion, migrating from JUnit to TestNG is a beneficial move for developers looking to enhance their testing capabilities. TestNG offers advanced features that are not available in JUnit, such as support for data-driven testing and parallel test execution. By following the recommended steps for migration and leveraging the features of TestNG, developers can optimize their testing processes and achieve more reliable and efficient software testing. Boost your productivity with Machinet. Experience the power of AI-assisted coding and automated unit test generation. Boost your productivity with Machinet. Experience the power of AI-assisted coding and automated unit test generation.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.