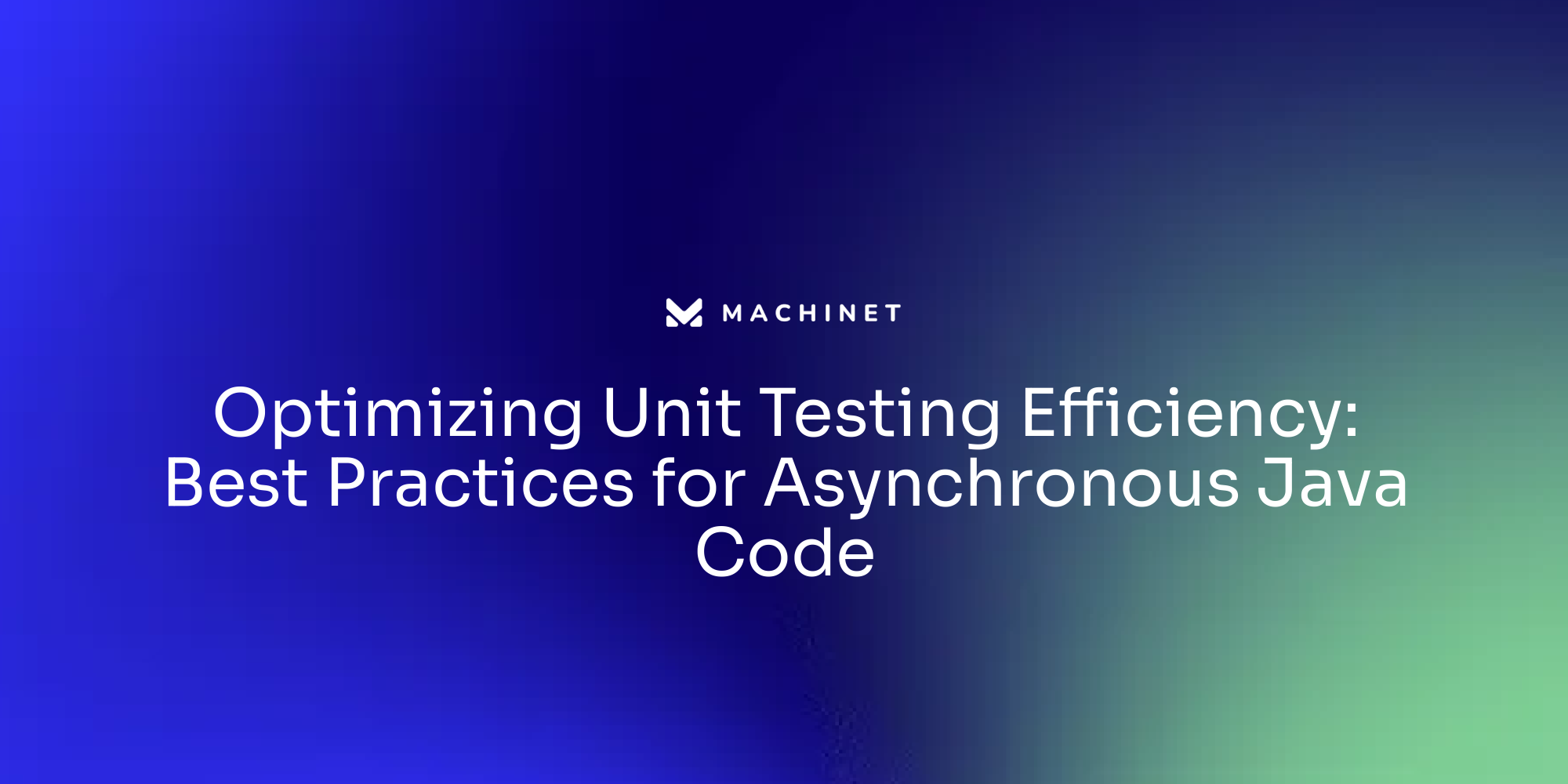
Table of Contents
- Understanding the Challenges of Unit Testing Asynchronous Java Code
- The Role of Automation in Enhancing Efficiency of Asynchronous Unit Tests
- Strategies for Managing Technical Debt in Asynchronous Java Code Testing
- Adapting to Changing Requirements: Implementing Flexible Testing Frameworks
- Balancing Deadlines and Quality Assurance in Asynchronous Java Code Testing
- Best Practices for Streamlining and Optimizing Asynchronous Unit Tests
- Case Study: Successful Implementation of Automated Unit Testing in Asynchronous Java Projects
Introduction
Unit testing is a critical aspect of software development, ensuring code quality and reliability. However, testing asynchronous Java code presents unique challenges due to its non-linear execution pattern and the complexities introduced by callbacks, promises, and futures. These challenges can make the design and implementation of unit tests difficult to write, understand, and maintain.
Despite the challenges, unit testing is essential for catching bugs early, providing a safety net for code refactoring, and serving as living documentation for code behavior. In this article, we will explore the significance of unit testing for asynchronous Java code and discuss strategies and best practices for effectively managing these tests. We will also delve into the role of automation in enhancing the efficiency of asynchronous unit tests and examine real-world examples of successful implementation. By understanding the complexities involved and adopting effective strategies and tools, developers can improve the reliability and maintainability of their software applications
1. Understanding the Challenges of Unit Testing Asynchronous Java Code
Unit testing forms a backbone for software development, offering a critical check on the quality and reliability of code. Asynchronous Java code, with its non-linear execution pattern, introduces a new level of complexity, making the design and implementation of unit tests a challenging task. The unpredictability that arises from the asynchronous execution of operations often complicates the testing process.
Asynchronous Java code intricacies are heightened with the inclusion of callbacks, promises, and futures. If not managed appropriately, these elements can lead to a scenario known as 'callback hell,' which further complicates the testing process. As a result, tests become difficult to write, understand, and maintain.
Despite these challenges, unit testing's significance is undeniable. It serves as an early warning system for potential bugs, identifying issues before they progress into larger problems. It also provides a safety net for code refactoring and maintenance, giving developers the confidence to modify the codebase, knowing that existing functionality remains unaffected.
Moreover, unit tests function as living documentation for the codebase, offering an up-to-date guide of the code's intended behavior. This encourages a modular design, making the codebase more maintainable and easier to comprehend. Although writing unit tests may initially seem like an added burden, they ultimately save time by catching bugs early and reducing the need for manual testing.
Regarding continuous integration and deployment (CI/CD), unit tests are imperative for ensuring that code is tested at every stage of the development process. By incorporating unit testing into the development process's core and utilizing automated testing tools, a comprehensive unit testing strategy is promoted, fostering a culture of continuous testing and improvement.
Nonetheless, the challenges of testing asynchronous workloads, such as multi-step workflows, asynchronous expectations, and external dependencies, are significant. These challenges require the use of various components to write effective integration tests for asynchronous workloads, including queues, workers, fake external service dependencies, test runners, and other application dependencies.
It is critical to be mindful of unintentional backward incompatible changes to job parameters and the risks associated with using marshalling as a job parameter encoding. Testing for backward compatibility and reducing marshalling complications are essential for effectively managing asynchronous workloads.
For instance, consider the examples of Rappi, Peacock, and BlaBlaCar, who successfully managed asynchronous workloads and iterated jobs with confidence using Braze. Rappi reactivated lapsed users and increased purchases with WhatsApp, whereas Peacock achieved a 20% reduction in churn through a year-long review campaign. BlaBlaCar leveraged Braze Canvas Flow to design an effective abandoned cart program. These real-world examples highlight the importance of effective testing in managing asynchronous workloads.
Testing asynchronous Java code can be daunting, but it is a crucial component of modern software development. By understanding the complexities involved and employing effective strategies and tools, developers can write, execute, and maintain effective unit tests for asynchronous Java code, thus improving the reliability and maintainability of software applications.
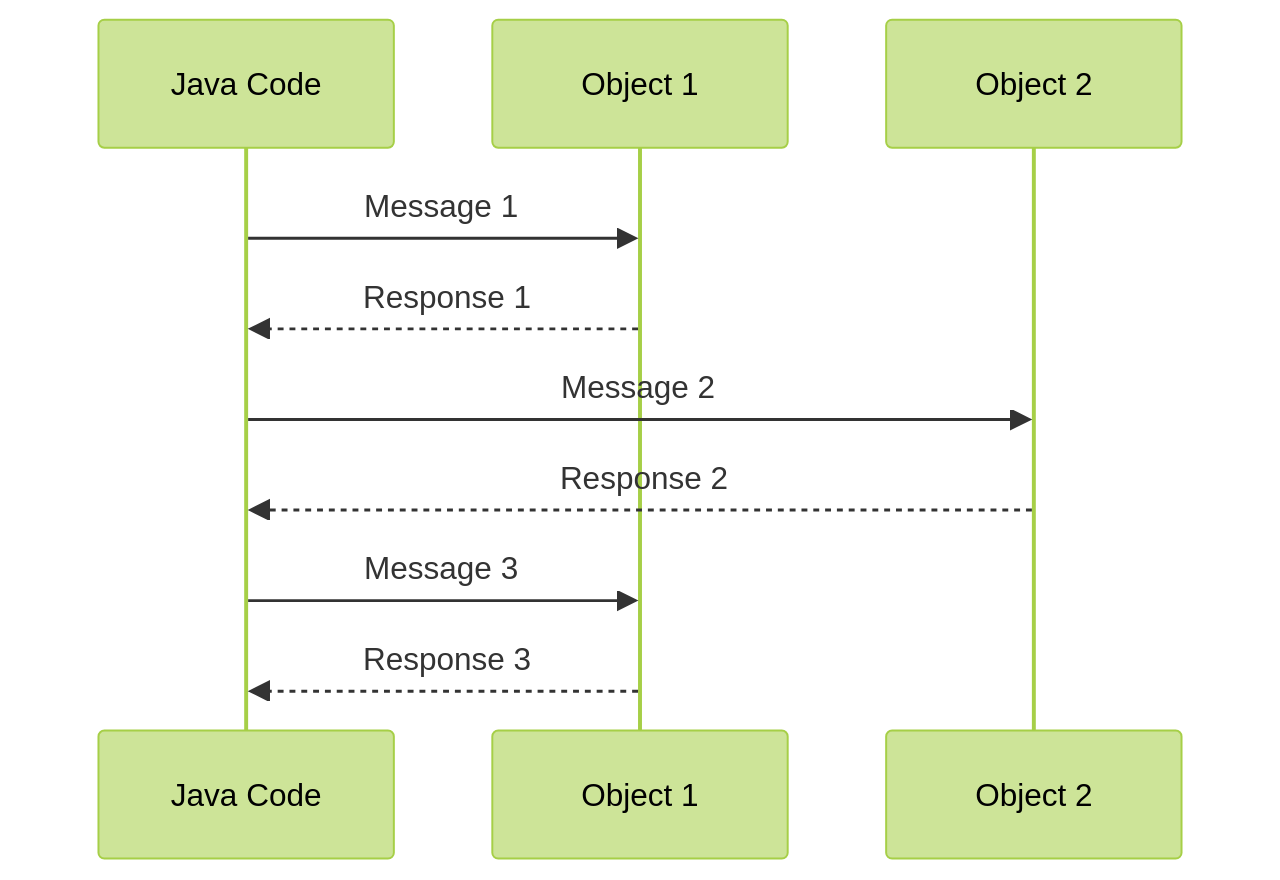
To tackle unit testing for asynchronous Java code, developers can refer to online tutorials, articles, and documentation related to unit testing in Java. Furthermore, books and courses on Java unit testing that cover asynchronous testing can provide invaluable insights. Such resources equip developers with the necessary information and techniques for effectively unit testing asynchronous Java code
2. The Role of Automation in Enhancing Efficiency of Asynchronous Unit Tests
Embracing automation in the realm of asynchronous unit testing is crucial for its efficiency. The value of this approach is highlighted by the benefits offered by automated testing frameworks such as JUnit and TestNG, which streamline the testing of asynchronous code. These tools incorporate methods that effectively tackle the challenges of timeouts and concurrency, two common issues in asynchronous programming.

The role of automation is to take over repetitive and time-consuming tasks, allowing developers to focus on crafting high-quality code. This transition not only boosts productivity but also reduces the likelihood for errors. However, it's key to remember that the primary aim of automated tests is to speed up the development process, not merely to prevent failures.
Consider the case of a company, strongdm, that used to rely entirely on manual quality assurance testing for each software release. In 2019, they had nearly no automated tests. Fast forward to the present day, and the company now has 56,343 tests. Interestingly, this massive increase in automated tests has not led to a significant reduction in the rate of bugs and failures.
As of September 2023, the company's test suite takes just under 10 minutes to complete. Their CI pipeline initiates 11 concurrent jobs on separate machines. However, these jobs often last between 12-15 minutes. They found that new build servers took longer to run tests. They had their Auto Scaling Group (ASG) set up to keep at least four instances running during working hours. But they discovered that the speed of the slowest job determines the overall speed of the test run.
So, they decided to scale down to zero between test runs to minimize costs and reduce cold starts. They collected data on the desired instance count and actual instance count using CloudWatch. To calculate the cost and the number of cold starts based on different configurations of minimum instance count and shutdown timeout, they used a Jupyter notebook. Their results showed that increasing the minimum instance count to eleven cut the number of cold starts nearly in half for about the same price.
They also implemented other optimizations, such as caching Node modules, optimizing Docker images, and replacing a test analytics tool. They found out they were using a networked EBS volume instead of the physically attached SSDs they had paid for. As a result of these changes, their tests now typically run in under five minutes. This case study highlights the significance of automation in testing, and how it can be optimized to enhance efficiency and reduce costs.
Automated testing frameworks can significantly impact productivity and error reduction in asynchronous unit tests. They provide tools and utilities that help developers write and execute tests more efficiently, leading to quicker development cycles and superior code quality. By automating the testing process, developers can swiftly run tests on their code, identifying and fixing errors early in the development lifecycle. This can help decrease the number of bugs and issues that make it into production, thereby improving the overall quality of the software.
In the context of asynchronous unit tests, where the code being tested involves asynchronous operations like callbacks or promises, automated testing frameworks offer features specifically designed to handle these scenarios. This can include mechanisms for managing timeouts, handling test dependencies, and dealing with asynchronous assertions. Overall, the use of automated testing frameworks in asynchronous unit testing can streamline the testing process, boost developer productivity, and help reduce errors in the tested code
3. Strategies for Managing Technical Debt in Asynchronous Java Code Testing
Addressing technical debt is a crucial aspect of testing asynchronous Java code. One effective approach in handling this is to prioritize refactoring tests that pose comprehension or maintenance challenges. Actions may range from simplifying complex callbacks to utilizing higher-level abstractions. In some cases, it might even require an overhaul of tests using a more advanced and efficient testing framework, although based on the provided context, there is no direct mention of such frameworks for rewriting Java tests.
Regular scrutiny and revision of tests is another useful strategy to ensure their relevance as the codebase evolves. This proactive stance aids in averting an accumulation of obsolete or unnecessary tests, which can impede the testing process and obscure crucial issues. Regular reviews and updates of tests in asynchronous Java code testing are crucial for maintaining relevance and effectiveness in identifying potential issues or bugs in the asynchronous code. It's essential to adapt tests to account for changes or updates in the asynchronous code's complex execution flows and dependencies.
Managing technical debt extends beyond refactoring and updating tests. It also includes addressing the challenge of flaky tests - tests that yield inconsistent results in supposedly identical environments. These tests can be especially problematic when testing asynchronous Java code due to the inherent unpredictability of concurrency.
Flaky tests can occur for various reasons. For instance, tests that depend on network connectivity or system load can yield different results under different conditions. Incorrectly used timeouts, often employed in tests to ensure tasks complete within a specified period, can also lead to flaky tests. To mitigate the issue of flaky tests, it's crucial to ensure that the test environment aligns with the needs and expectations of the APIs used. This might involve configuring the test environment to have stable network connectivity and predictable system load.
Another approach to handling flaky tests is the use of indefinite waiting without a timeout. This can prevent tests from failing due to unexpected delays. However, this approach can lead to prolonged executions of broken tests and can cause deadlocks in single-threaded concurrency. Therefore, it's advisable to combine finite waiting and infinite retries to avoid these issues.
Finally, it's key to remember that functional tests and performance tests are fundamentally different and should not be interchanged. Performance tests should be conducted in a representative execution environment and should not be disguised as functional tests. Testing for the absence of events should also be avoided unless the test environment represents the target execution environment, and the operations leading to the event provide timing guarantees.
By employing these strategies, it's possible to effectively manage technical debt and ensure the reliability and robustness of asynchronous Java code. Implementing best practices and techniques for Java unit testing, such as understanding the JUnit framework and asynchronous programming concepts in Java like CompletableFuture and CompletableFuture API, can significantly reduce technical debt over time. Furthermore, regular refactoring and test code improvement, including identifying and removing any code smells or anti-patterns, can maintain a high level of quality and reduce the chances of introducing new issues or bugs
4. Adapting to Changing Requirements: Implementing Flexible Testing Frameworks
In the dynamic realm of software development, the necessity for malleable testing frameworks is paramount. These frameworks equip engineers with the ability to swiftly adapt or expand tests to meet new functionality or modifications in the code. They also cater to a spectrum of testing types, from integration to end-to-end testing, providing a holistic solution when unit tests alone might not suffice. By selecting a flexible testing framework, software engineers can uphold the relevance and effectiveness of their tests, even as project requirements evolve.
However, awareness of potential pitfalls associated with automated testing is crucial. One such pitfall is the occurrence of flaky tests - automated tests that yield inconsistent results, sometimes passing and sometimes failing, without any code changes. Even with a low failure rate, flaky tests can significantly impact large-scale applications under test. Neglecting these tests can lead to squandered continuous integration (CI) time and resources as developers need to rerun tests to address false failures. Additionally, this rerunning of tests leads to wasted development time as developers have to wait for the build and test time again.
Furthermore, flaky tests can be indicative of non-deterministic implementations, leading to inconsistent product behavior in production. This can result in alert fatigue, where developers lose trust in the feedback from flaky tests, leading to a reduction in development velocity and confidence in tests. The causes of flaky tests can vary from poorly written test code, poorly written application code, infrastructural issues, and test tools that are prone to flakiness.
To alleviate the impact of flaky tests, it's crucial to collect data on flakiness and analyze it to understand the scale of the problem and lack of trust in tests. Running new tests multiple times and adjusting them can help reduce flakiness. Setting a benchmark for when a test is considered not flaky, such as running it 100 consecutive times without failure, can help ensure the stability of tests. Tools like CPU throttling emulation and test tracing can help identify and debug flaky tests.
In the sphere of Flutter development, the need for middle-ground integration testing has been acknowledged. The primary hurdle is testing how different features of the app interact without the overhead of setting up UI tests. To address this, one approach was to construct a custom integration testing framework. This framework emphasizes easily runnable tests, a familiar API for developers, and the ability to test integration features without setting up the entire app. It included stubbing problem areas, such as network interactions and native code plugins, using lightweight wrappers. The focus was also on testing "happy paths" or non-exceptional flows to ensure impactful testing.
Regardless of the programming language or platform, the value of flexible and robust testing frameworks is undeniable. They ensure code quality and reliability and help manage technical debt by identifying and addressing issues early in the development cycle. Therefore, investing in a comprehensive and flexible testing strategy is crucial for the successful delivery of high-quality software products. The use of a flexible testing framework allows developers to easily modify and expand their test suites, thus improving the overall quality and coverage of the testing process. It also provides better support for different programming languages and platforms, allowing developers to choose the most suitable framework for their specific needs, further enhancing the efficiency and effectiveness of the testing process
5. Balancing Deadlines and Quality Assurance in Asynchronous Java Code Testing
In the world of asynchronous Java code testing, maintaining a balance between deadline adherence and quality assurance is a delicate dance. The tug-of-war between delivering on time and producing dependable, error-free code is a constant challenge.
One strategy to harmonize these competing demands is to interweave testing into the development process, rather than treating it as a separate stage. This integration can take the form of methodologies like Test-Driven Development (TDD) or Behavior-Driven Development (BDD), both of which advocate for the creation of tests either before or alongside the writing of code. As a result, the code is continually verified throughout its evolution, ensuring its reliability.
In addition to these methodologies, the use of continuous integration tools can be a game-changer. These tools automatically initiate tests whenever changes are made to the codebase, allowing for instantaneous detection and rectification of issues. This continuous testing process reduces the likelihood of unexpected complications arising at the end of the development cycle.
However, it's crucial to be aware of potential stumbling blocks that could compromise your tests' stability, leading to inconsistent results in seemingly identical environments. This issue, known as "flaky tests," can stem from a multitude of factors, such as network connectivity dependencies, system load, or differing library dependencies.
An example of this is when a test initiates an asynchronous operation and waits for its result via a future operation. The test can become flaky if the execution time assumption is incorrect or if the timeout is not set appropriately. To avoid such flaky tests, ensuring that the test environment aligns with the APIs used in the unit under test is critical. If it's not possible to establish such guarantees or contracts for APIs, more permissive timeouts can be a temporary fix, but it's not a long-term solution.
In some situations, waiting indefinitely without a timeout can be a better option as it avoids making assumptions about the operation's execution time. Yet, this approach has its limitations. It can result in prolonged executions of broken tests and may hide test failures as infrastructure failures. Moreover, in single-threaded concurrency, this method can potentially lead to deadlocks.
Venkatesh Prasad Ranganath, an authority in the field, suggests that it's crucial not to masquerade performance tests as functional tests and to perform performance testing in a representative execution environment. Similarly, testing for the absence of events should be done using proxy events or infinite polling and notification, rather than timed waiting.
To effectively integrate testing into the development process for asynchronous Java code, several steps can be followed:1. Identify the asynchronous code: Determine which parts of your Java code are asynchronous, such as methods or functions that use callbacks, futures, or reactive programming libraries.2. Write test cases: Create test cases that cover the different scenarios and edge cases for your asynchronous code. Make sure to include both positive and negative test cases.3. Use testing frameworks: Use testing frameworks like JUnit or TestNG to write and run your test cases. These frameworks provide features for handling asynchronous code, such as timeouts and assertions for expected outcomes.4. Mock dependencies: If your asynchronous code depends on external systems or services, use mocking frameworks like Mockito or PowerMockito to simulate the behavior of these dependencies during testing.5. Handle concurrency issues: Asynchronous code often involves concurrency, so make sure to handle any potential race conditions or synchronization issues in your test cases. Use synchronization mechanisms like locks or semaphores if necessary.6. Use test doubles: When testing asynchronous code, it's important to isolate the code under test from external dependencies. Use test doubles like stubs or mocks to simulate the behavior of these dependencies and make your tests more deterministic.7. Test asynchronous behavior: Test the asynchronous behavior of your code by verifying that callbacks or futures are called or completed as expected. Use assertions or matchers provided by your testing framework to check the results of asynchronous operations.8. Automate testing: Integrate your test cases into your development process by automating them with build tools like Maven or Gradle. Set up continuous integration pipelines to run your tests automatically on each code change.
By adopting these tactics, developers can strike a balance between meeting deadlines and ensuring quality in asynchronous Java code testing.
Optimize your asynchronous unit tests with these best practices.
With a strategic approach, it's possible to deliver robust, high-quality software within the stipulated deadlines
6. Best Practices for Streamlining and Optimizing Asynchronous Unit Tests
Asynchronous unit testing in Java presents unique challenges, but with the right practices and tools, the efficiency and maintainability of your test scripts can be significantly enhanced. This, in turn, improves the quality of your asynchronous Java code.
In optimizing asynchronous unit tests, Java provides several tools. For instance, the CompletableFuture
class is a valuable tool that allows handling of asynchronous computations and their results. The CountDownLatch
class is another crucial resource that can synchronize the test execution with the completion of asynchronous tasks.
To prevent tests from running indefinitely, the @Timeout
annotation can be used to set a limit for the execution of asynchronous tests. The @BeforeEach
and @AfterEach
annotations are additionally recommended for setting up and tearing down any resources needed for the asynchronous tests.
Unit testing of asynchronous code can be enhanced through assertion libraries, which offer functionalities and features specifically designed for handling asynchronous code. AssertJ, a popular assertion library, provides a fluent and expressive API for making assertions on asynchronous code. It simplifies handling of asynchronous operations, such as waiting for a certain condition to be met or verifying the completion of tasks.
JUnit, another widely-used assertion library, offers built-in support for asynchronous testing through the use of the CompletableFuture
class. It allows writing assertions that wait for asynchronous tasks to complete and verify their results.
Mockito, primarily known for its mocking capabilities, also supports asynchronous testing. It enables the creation of mock objects that simulate asynchronous behavior, and the writing of assertions to verify interactions with these objects.
In terms of test data, it's essential to isolate the data used by each test to avoid conflicts when running tests concurrently. Creating resources like users or records in a database as part of tests should be done using unique identifiers to avoid conflicts.
In relation to testing web applications, running tests in headless mode can speed up execution times. Writing tests as if they will be running in parallel can help avoid flaky tests and make scripts easier to maintain. It's also beneficial to prioritize user-facing attributes and use locators with auto waiting and retry ability.
To keep up with the latest browser versions and catch failures early, it's important to keep Playwright, a valuable tool for generating tests and picking locators, up to date. Integrating Playwright tests with CI/CD and running tests frequently is also recommended.
In conclusion, the quality and efficiency of your asynchronous Java code can be significantly enhanced by implementing these practices and leveraging the functionalities of tools and libraries such as CompletableFuture, CountDownLatch, AssertJ, JUnit, Mockito, and Playwright
7. Case Study: Successful Implementation of Automated Unit Testing in Asynchronous Java Projects
A perfect exemplification of the powerful impact of automation in unit testing for asynchronous Java code can be found in a joint venture between Altexsoft and Snaptracs Inc. Snaptracs Inc., being a worldwide manufacturer of pet tracking wearable devices, aimed for an upgrade in their product, the Tagg GPS Pet Tracker, via the creation of a robust QA automation framework.
Altexsoft, a company renowned for their software solutions, was their go-to for executing meticulous testing and QA automation tasks. This project was time-critical and necessitated close collaboration with the team at Snaptracs Inc. Altexsoft assigned a team comprising two QA engineers and a project manager for the task, employing a variety of tools such as Java, Git, Eclipse IDE, Saucelabs, Bamboo Cloud, Jira, and Bitbucket.
The team's primary goal was to augment the overall quality of the software, utilizing both manual and automated testing. They established automated feature testing to replace manual work, a benefit that mirrors the Machinet AI plugin's advantages. The outcome was an entirely automated QA process that operates independently, leading to significant time and cost savings. Consequently, the client received immediate feedback on issues arising from code modifications, enabling the development team to promptly rectify any problems.
The QA process was comprehensive, encompassing meticulous manual testing, automated tests running post each build, and complete automation of all system features. The team's hard work over a span of five months resulted in considerable enhancements in the final product's quality.
This case study beautifully demonstrates the profound impact of automation in enhancing the efficiency and effectiveness of unit testing for asynchronous Java code. However, it's crucial to note that the case's success was not solely due to automation but also because of the team's adherence to best practices in unit testing.
In addition to using the Machinet AI plugin, it's important to follow general best practices for unit testing in Java. This includes writing testable code, using appropriate test fixtures, and ensuring test coverage for all code paths. Furthermore, understanding and effectively using Mockito, a popular framework for mocking in Java unit testing, can help you mock dependencies and test asynchronous code more easily. Mockito provides features like CompletableFuture
and thenApplyAsync
for testing asynchronous code.
By integrating the Machinet AI plugin into your unit testing workflow, you can save time and improve the effectiveness of your tests. It provides features such as understanding annotations and assertions for Java unit testing, as well as best practices and tips for effective unit testing in Java. By following these guidelines and leveraging the capabilities of Machinet AI plugin, you can significantly improve the quality of your unit tests for asynchronous Java code
Conclusion
In conclusion, unit testing for asynchronous Java code presents unique challenges due to its non-linear execution pattern and the complexities introduced by callbacks, promises, and futures. However, despite these challenges, unit testing is crucial for catching bugs early, providing a safety net for code refactoring, and serving as living documentation for code behavior. By understanding the complexities involved and adopting effective strategies and tools, developers can improve the reliability and maintainability of their software applications.
The significance of automation in enhancing the efficiency of asynchronous unit tests cannot be overstated. Automated testing frameworks like JUnit and TestNG streamline the testing process by handling timeouts and concurrency issues. Automation allows developers to focus on crafting high-quality code while reducing errors. By automating the testing process, developers can identify and fix issues early in the development lifecycle, ultimately improving the overall quality of the software. To boost your productivity with Machinet, experience the power of AI-assisted coding and automated unit test generation here.
Conclusion
In conclusion, managing technical debt in asynchronous Java code testing is crucial for ensuring reliable and robust software applications. Prioritizing refactoring tests that pose comprehension or maintenance challenges, regular scrutiny and revision of tests, and addressing flaky tests are effective strategies for managing technical debt. Additionally, implementing flexible testing frameworks that can adapt to changing requirements is essential for streamlining the testing process.
Furthermore, balancing deadlines with quality assurance in asynchronous Java code testing requires integrating testing into the development process, leveraging methodologies like Test-Driven Development (TDD) or Behavior-Driven Development (BDD), and utilizing continuous integration tools. By striking a balance between meeting deadlines and ensuring quality assurance through strategic approaches, developers can deliver high-quality software within stipulated timeframes.
To optimize asynchronous unit tests, best practices include utilizing tools like CompletableFuture and CountDownLatch in Java, using assertion libraries such as AssertJ or JUnit for handling asynchronous code assertions efficiently, mocking dependencies using frameworks like Mockito to isolate the code under test from external dependencies during testing.
Implementing these strategies and best practices can significantly enhance the efficiency and maintainability of asynchronous unit tests in Java. By investing in comprehensive automated testing frameworks, adapting to changing requirements, managing technical debt effectively, balancing deadlines with quality assurance considerations, and following best practices for optimizing asynchronous unit tests; developers can ensure reliable software applications that meet customer needs effectively.Boost your productivity with Machinet. Experience the power of AI-assisted coding and automated unit test generation here
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.