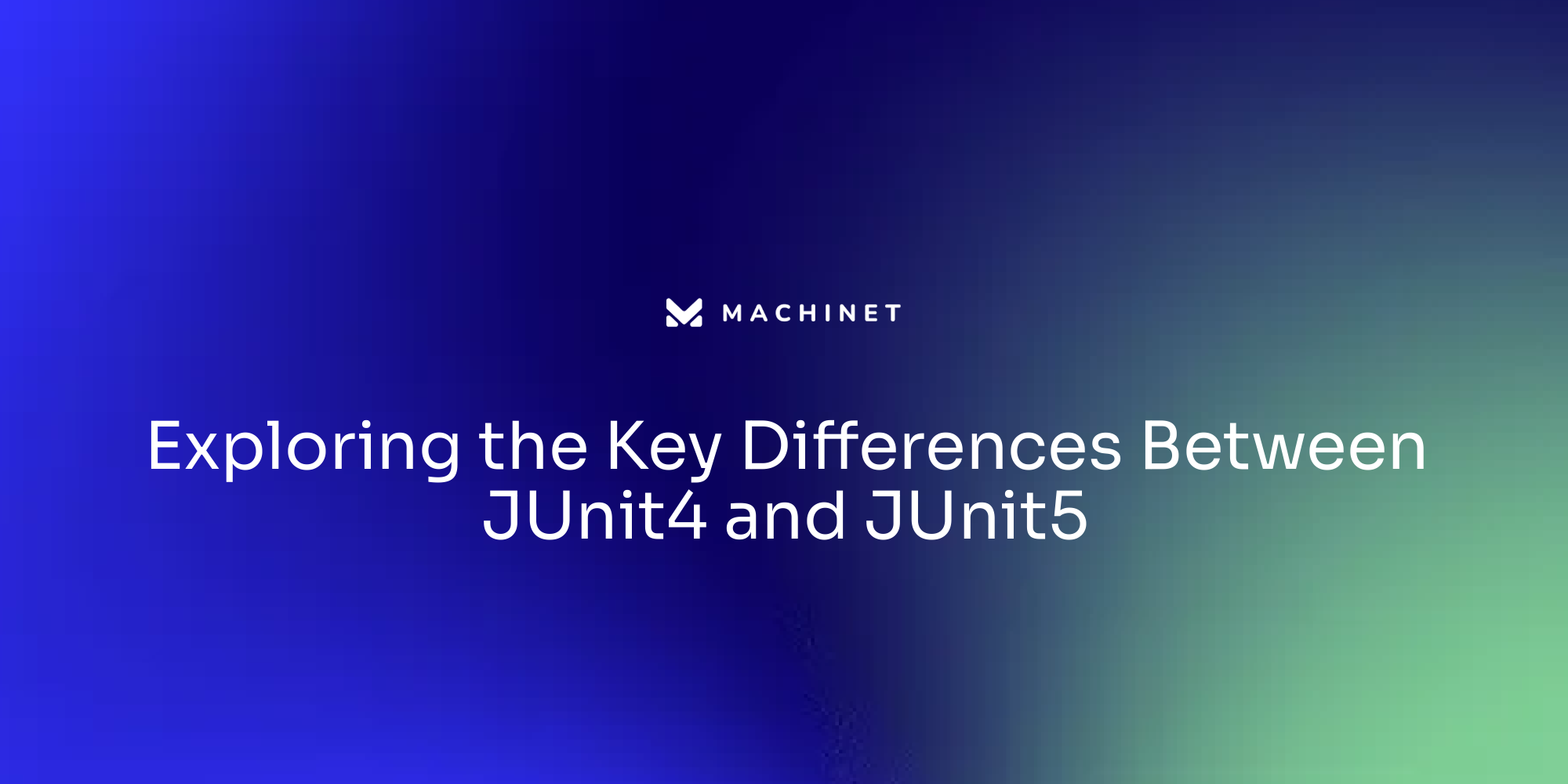
Table of Contents
- Understanding JUnit4: Core Features and Functionality
- Unveiling JUnit5: What's New and Improved
- Transitioning from JUnit4 to JUnit5: A Step-by-Step Guide
- Key Advantages of Migrating to JUnit5
- Dealing with Challenges in Migration from JUnit4 to JUnit5
- Real-world Examples: Applying JUnit5 in Software Testing Scenarios
- Best Practices for Maximizing the Benefits of JUnit5
Introduction
JUnit is a widely used testing framework in the Java community, offering a suite of powerful features for unit testing. In this article, we will explore the core features and functionality of JUnit4, which has been a cornerstone in the Java testing landscape. JUnit4 provides developers with a systematic approach to structuring and organizing their test cases using annotations like @Test, @Before, @After, @BeforeClass, and @AfterClass. We will also discuss the limitations of JUnit4 and the challenges it poses in managing dynamic tests and extensibility.
JUnit4 has been the go-to testing framework for Java developers practicing Test-Driven Development (TDD), seamlessly integrating into the standard Java development workflow. It is supported by major Java IDEs and build tools, making it accessible to developers worldwide. We will delve into the structure of a test class in JUnit4, the use of annotations to mark test methods, and the assertion methods available for validating expected outcomes. Additionally, we will touch upon the transition from JUnit4 to JUnit5, which introduces new annotations and enhancements for improved testing capabilities. By understanding the core features and limitations of JUnit4, developers can make informed decisions and explore new testing paradigms to enhance the quality of their code
1. Understanding JUnit4: Core Features and Functionality
JUnit4 has served as a fundamental pillar in the Java community, providing a robust suite of unit testing capabilities. The core functionalities of JUnit4 are centered around a variety of annotations, including @Test, @Before, @After, @BeforeClass, and @AfterClass.

These annotations play a crucial role in guiding developers in structuring and organizing their test cases, reinforcing a systematic approach to unit testing.
In addition to this, JUnit4 offers support for exception testing and timeout, equipping developers with the tools to assess the resilience of their code under a range of conditions. However, JUnit4 does have its limitations, particularly when it comes to managing dynamic tests and extensibility.
In the era of Test-Driven Development (TDD), an integral part of contemporary software engineering, JUnit4 has established itself as the preferred testing framework for Java developers.
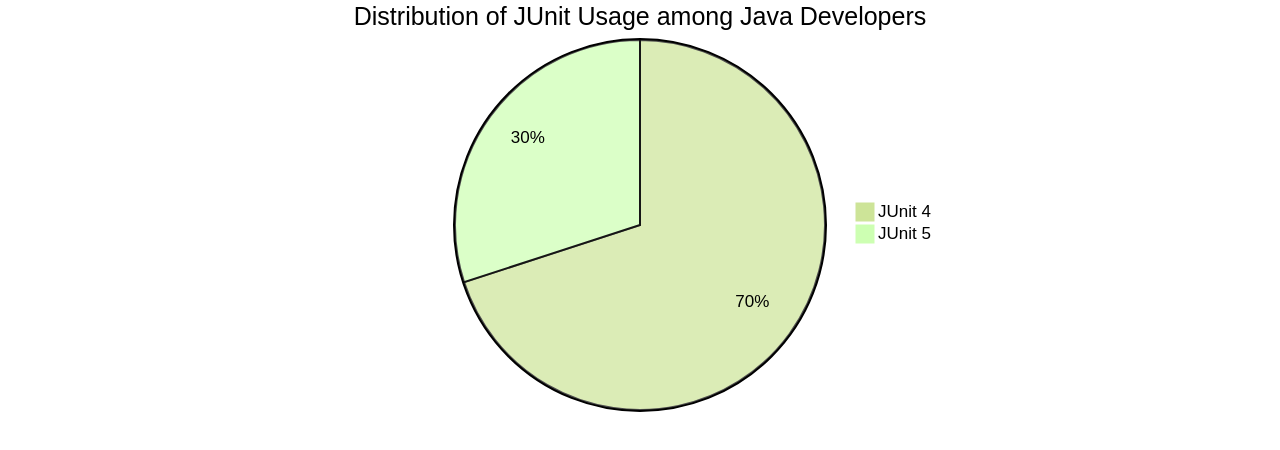
It is widely supported by almost all Java IDEs and build tools, making it a mainstay in the standard Java development workflow.
Eclipse, a leading Java IDE, offers robust support for JUnit4, featuring it as its default testing library. The structure of a test class in JUnit4 is shaped through the use of specific annotations. For example, @BeforeClass and @AfterClass annotations are used to execute a set of instructions once before and after all test methods in a class, respectively. Likewise, @Before and @After annotations execute code before and after each test method.
Methods marked with the @Test annotation are recognized as test methods by the framework. The test methods can utilize various assert
methods provided by JUnit to validate the expected outcomes. For instance, the assertEquals()
method compares two values and throws an AssertionError if they are unequal. Other available assert
methods include assertNotEquals()
, assertTrue()
, assertFalse()
, assertNull()
, assertNotNull()
, assertSame()
, and assertNotSame()
.
As a practical example, the @Test annotation is used in JUnit4 to mark a method as a test method. Let's consider a few examples of how the @Test annotation can be used in JUnit4:
- Basic usage:```javaimport org.junit.Test;
public class MyTestClass { @Test public void testMethod() { // Test logic goes here }}```
- Testing expected exceptions:```javaimport org.junit.Test;
public class MyTestClass { @Test(expected = ArithmeticException.class) public void testMethod() { // Test logic that throws an ArithmeticException goes here }}```
- Testing with timeout:```javaimport org.junit.Test;
public class MyTestClass { @Test(timeout = 1000) public void testMethod() { // Test logic that should complete within 1 second goes here }}```
In terms of handling dynamic tests in JUnit4, you can utilize the DynamicTest
class and the @TestFactory
annotation.
Try using dynamic tests in JUnit4 to enhance your test cases!
This enables the generation of tests at runtime based on certain conditions or parameters. By implementing the TestFactory
interface, you can define a method that returns a list of DynamicTest
instances. Each DynamicTest
represents a single test case that can be executed by JUnit.
While JUnit4 has proven to be a reliable tool for Java developers across the globe, it's vital to stay abreast of the latest developments in the testing framework landscape.

The introduction of JUnit5, which comes with numerous new annotations and enhancements, including @BeforeAll, @BeforeEach, @AfterEach, and @AfterAll, offers developers more flexibility and control over the testing lifecycle. Transitioning from JUnit4 to JUnit5, however, warrants a separate discussion.
In essence, while JUnit4 has been a dependable resource, the advent of JUnit5 presents an opportunity for developers to explore new testing paradigms and enhance the overall quality of their code
2. Unveiling JUnit5: What's New and Improved
The upgraded version of the JUnit framework, JUnit5, delivers a range of enhancements and groundbreaking features, which specifically address the limitations encountered with JUnit4.

One of the notable improvements is the initiation of a novel extension model, enhancing its resilience and flexibility.
JUnit5 has been architecturally structured as an assembly of numerous modules, such as JUnit Platform, JUnit Jupiter, and JUnit Vintage. This modular architecture facilitates a more adaptable testing environment in comparison to its antecedents.
JUnit5 now backs dynamic tests and parameterized tests, providing developers with increased flexibility in formulating test cases. This adaptability proves to be a significant advantage for developers as it enables the creation of a more diversified and exhaustive suite of tests.
Further enhancing the testing experience, JUnit5 introduces novel annotations like @BeforeEach, @AfterEach, @Tag, and @DisplayName. These annotations grant developers greater control over the testing process and enable the creation of more descriptive and well-organized tests.
For instance, the @BeforeEach and @AfterEach annotations can be utilized to denote methods that should be executed before and after every test. This proves to be advantageous in establishing and dismantling testing environments or conditions that are critical for each test.
The @Tag annotation, conversely, enables tests to be classified in a manner that is beneficial to the developer, such as by functionality or by requirement. This simplifies the process of running a specific subset of tests.
Lastly, the @DisplayName annotation allows for more descriptive and human-readable test names. This simplifies the process of understanding what a test does without the need to delve into the implementation details.
On the whole, these new features and enhancements make JUnit5 a more potent and adaptable testing framework, enabling developers to write more effective and efficient tests. The JUnit Platform module acts as a foundation for launching various testing frameworks on the JVM, providing a TestEngine API for discovering and executing tests. The JUnit Platform also defines the TestDescriptor and TestExecutionListener APIs that can be leveraged by other testing frameworks to integrate with the platform
3. Transitioning from JUnit4 to JUnit5: A Step-by-Step Guide
The transition from JUnit4 to JUnit5 is a process that requires careful thought and meticulous execution.
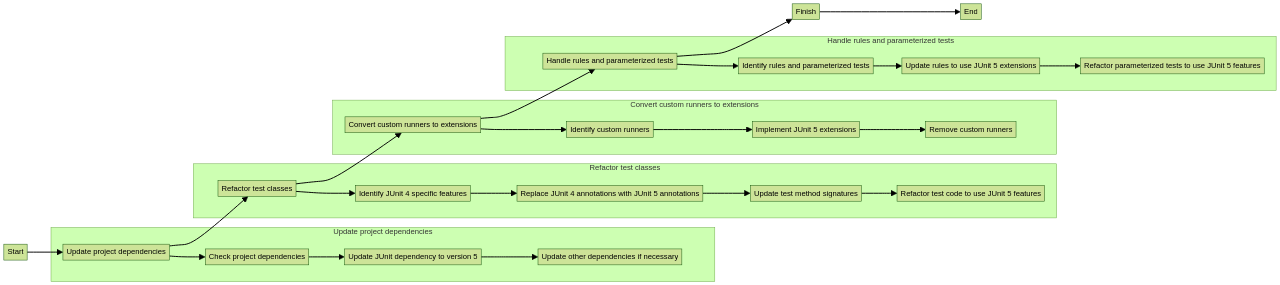
The first step in this process is to update the project dependencies to include JUnit5 libraries. This can be achieved by modifying the project's build configuration file, often named "pom.xml" for Maven projects or "build.gradle" for Gradle projects. Within this file, locate the section that specifies the dependencies for the project. Here, you can find the entry for JUnit, which may be labeled as "junit" or "junit-jupiter" depending on the specific JUnit version you are using. Update this to the desired version of JUnit5, save the changes, and rebuild your project.
Once the dependencies are set, the next step involves refactoring of test classes and methods to adapt to the annotations provided by JUnit5. While the context information does not provide specific details about the test classes and methods in the project that need refactoring, it is important to understand that JUnit5 offers new annotations such as @Test
, @BeforeEach
, @AfterEach
, @BeforeAll
, and @AfterAll
. These annotations play a crucial role in defining test methods, setup and teardown methods, and methods that need to run before or after all tests in a test class.
The JUnit5 extension model replaces the runners of JUnit4. Hence, any custom runners should be converted into extensions. The conversion process involves identifying the specific behavior provided by the custom runner and implementing it as an extension. This approach allows you to leverage the benefits of JUnit5's extension model and ensures compatibility with your project.
Additionally, any rules or parameterized tests in JUnit4 must be restructured using the new constructs offered by JUnit5. To aid in this process, tools like OpenRewrite can be used to automate code migrations and refactoring. For Spring or Spring Boot projects, the "rewrite-spring" dependency should be added and the "springboot2junit4to5migration" recipe activated. For non-Spring projects, the "rewrite-testing-frameworks" dependency should be added and the "junit5bestpractices" recipe activated. The migration can be executed using the "mvn rewriterun" or "gradlew rewriterun" commands.
After executing these steps, it's crucial to inspect the results using "git diff" or a similar tool. This allows for any necessary manual fixes to be made before committing the changes. It is important to note that there may be known limitations and unsupported functionality in the migration process, such as certain JUnit4 features or libraries which do not have a direct JUnit5 counterpart. Custom JUnit4 rules or runners may not be migrated automatically and may require manual handling or a custom recipe.
To further assist in the migration process and to delve into the depths of JUnit5, the Test Automation University (TAU) offers an in-depth tutorial on JUnit5. This tutorial includes various aspects of writing tests such as setup, lifecycle methods, parameterized tests, and more. It also provides code examples and encourages the use of AI-powered tools like Applitools for testing resources. The tutorial emphasizes the use of annotations in JUnit5 and mentions that JUnit5 has experimental features that may be improved or discarded based on community feedback. The tutorial covers 18 chapters and includes topics such as setup, writing tests, lifecycle methods, parameterized tests, test run order, assumptions, disabling tests, repeating tests, tags, assertions, running tests, test watcher, timeouts, nested tests, custom annotations, and migrating from JUnit4
4. Key Advantages of Migrating to JUnit5
Embracing JUnit5 introduces a plethora of advantages. The framework's modular architecture fosters adaptability and control over the testing environment. The new extension model is a powerful tool for extending tests, enabling customization of test execution. Moreover, JUnit5's compatibility with state-of-the-art Java features positions it as the go-to choice for projects that require modern Java versions.
Significant enhancements have been made to the annotation system in JUnit5. Dynamic and parameterized tests are now supported, which boosts the efficiency of test writing and organization. For example, the "before" and "after" annotations have been renamed to "beforeEach" and "afterEach", respectively, while "beforeAll" and "afterAll" have been renamed to "beforeClass" and "afterClass". The "ignore" annotation in JUnit 4 has transitioned to the "disabled" annotation in JUnit 5.
The "runWith" annotation, previously used for integrating tests with other frameworks in JUnit4, has been replaced by the "extendWith" annotation in JUnit5. This development in annotations illustrates one of the many ways JUnit5 has enhanced its predecessor.
Importantly, switching from JUnit4 to JUnit5 doesn't necessitate a complete rewriting of all your tests. The JUnit Vintage engine allows JUnit 4 tests to be executed within the JUnit 5 environment. To start leveraging the advanced features of JUnit 5, simply include the JUnit Jupiter dependencies in your project.
For an easier transition to JUnit 5, consider utilizing the Machinet AI plugin. This tool facilitates the migration process by providing automated utilities, ensuring compatibility with JUnit 5. With the Machinet AI plugin, updating your existing JUnit 4 tests to JUnit 5 becomes a breeze, allowing you to benefit from the new features and improvements.
To use Machinet for JUnit5 migration, ensure that your project dependencies are updated with the necessary JUnit5 components, including any additional extensions or libraries. Convert your existing JUnit4 test classes to JUnit5 format by updating the annotations and assertions, and adapt any JUnit4-specific features that are no longer supported in JUnit5. Depending on your build system (e.g., Maven, Gradle), your configuration may need to be updated to include the necessary dependencies and plugins for JUnit5. Upon completing the migration steps, run your tests to confirm they are functioning properly.
In a nutshell, JUnit5 is a potent testing framework that supports cutting-edge Java features and offers improved test organization and increased flexibility in test execution. Its user guide serves as a comprehensive introduction to writing tests with JUnit 5, proving to be an essential resource for developers
5. Dealing with Challenges in Migration from JUnit4 to JUnit5
Embarking on the journey from JUnit4 to JUnit5 may initially seem complex, given the changes in the annotation system, the new extension model, and the shift from parameterized tests and rules. But with a clear understanding of the differences between the two versions and careful planning, these changes can be navigated with ease.
JUnit5, released around two years ago, has now become the preferred testing framework due to the myriad enhancements it offers over JUnit4. One of the key advantages is that there's no need to overhaul all your existing JUnit4 tests at once while migrating to JUnit5. Thanks to the JUnit Vintage engine, your older JUnit4 tests can run within the JUnit5 context.
To fully leverage JUnit5, it's important to add certain dependencies to your project. For crafting new tests, the JUnit Jupiter API and JUnit Jupiter engine dependencies are needed. For running older JUnit4 tests, the JUnit Vintage engine dependency becomes essential.
In JUnit5, there's been a revamp of the annotations. For instance, the 'before' and 'after' annotations from JUnit4 have been replaced by 'beforeEach' and 'afterEach' in JUnit5. Similarly, 'beforeAll' and 'afterAll' have been swapped with 'beforeClass' and 'afterClass'. The 'ignore' annotation in JUnit4 is now represented by the 'disabled' annotation in JUnit5. JUnit5 has also done away with the 'RunWith' annotation for specifying different test runners, choosing instead to use the 'ExtendWith' annotation for integrating tests with other frameworks.
The shift from JUnit4 to JUnit5 may seem daunting at first, but it need not be so. As John Ring, a noted figure in the software testing community, once pointed out, "To migrate from JUnit 4.x to JUnit 5, you don't have to rewrite all your tests at once." This stands true and is a testament to the seamless transition facilitated by JUnit5.
To assist in this transition, the JUnit5 user guide offers a detailed introduction to writing tests with JUnit5. Additional resources are also available to provide more practical JUnit5 tips. So, while the transition may initially seem challenging, the benefits of JUnit5 make it a worthwhile undertaking.
However, the migration from JUnit4 to JUnit5 does come with its own set of challenges. Dealing with the changes in API and annotations, integrating JUnit5 with the build and test infrastructure, and adapting existing tests to the new test engine architecture are some of the hurdles to be overcome.
Moreover, rewriting parameterized tests in JUnit5 can be done more efficiently by leveraging the new features and enhancements in JUnit5. With JUnit5, you can use the @ParameterizedTest annotation to define parameterized tests and the @ValueSource annotation to supply the input values. JUnit5 also offers support for dynamic tests, which allows for the generation of tests at runtime based on different input values. This makes the test code more concise and readable, thereby simplifying the writing and maintenance of parameterized tests in JUnit5.
When planning the migration to JUnit5, understanding the differences and new features in JUnit5 compared to JUnit4 is a key first step. The process involves evaluating compatibility, updating dependencies, converting test classes, handling breaking changes, running compatibility tests, and training your team. By following these steps, you can effectively plan and execute the migration to JUnit5, ensuring a smooth transition and improved testing capabilities
6. Real-world Examples: Applying JUnit5 in Software Testing Scenarios
JUnit5 introduces a multitude of practical features beneficial for software testing.
Explore the practical features of JUnit5 for software testing!
A standout among these is the dynamic testing capability, which allows for the creation of test cases at runtime based on certain conditions. This proves particularly useful when testing data-driven applications.
Another significant addition to JUnit5 is the parameterized tests feature. This enables the execution of a single test case with a variety of different inputs, which is an invaluable asset when testing functions that accept a range of input values. The process of setting up parameterized tests with JUnit5 involves configuring the necessary dependencies using Maven and Gradle. A basic parameterized test accepting a single method parameter can be established, and to distinguish each method invocation in a parameterized test, the display name can be personalized.
JUnit5 offers a range of argument sources for parameterized tests. This includes the ValueSource annotation for simple types and the EnumSource annotation for enum values. To further leverage this feature, test data can be created using the CSV format and loaded from a CSV file. While the default argument converter in JUnit5 has its constraints, it is feasible to create custom argument converters. This enables the creation of test data using factory methods and custom argument providers. Moreover, custom argument converters can be employed to provide test data as strings.
The new extension model of JUnit5 is a powerful instrument. Although there is no direct context information about creating custom extensions in JUnit5, referring to the official JUnit5 documentation and GitHub repository can provide detailed guidance and examples. Online tutorials and blog posts also provide practical examples of implementing custom extensions in JUnit5.
Furthermore, resources such as the 'bonigarcia/mastering-junit5' repository on GitHub serve as comprehensive reference materials for mastering JUnit5. This repository, licensed under the Apache 2.0 license, offers examples from the book 'Mastering Software Testing with JUnit 5'. It features branches, tags, and has received contributions from various contributors, making it a valuable resource for understanding software testing scenarios with JUnit5.
In essence, JUnit5 presents a comprehensive set of features that cater to diverse testing scenarios, marking it as an indispensable tool for software engineers
7. Best Practices for Maximizing the Benefits of JUnit5
The key to maximizing the benefits of JUnit5 lies in adopting certain developer best practices. A crucial aspect of this is harnessing JUnit5's revamped features such as dynamic and parameterized tests. These tools offer the adaptability required to build more comprehensive and adaptable test cases.
Dynamic tests offer the capability to generate and run tests at runtime. This proves useful when dealing with a set of tests that can only be determined at runtime or when you want to generate tests based on certain conditions or data.
Parameterized tests, on the other hand, allow for the execution of the same test logic with different input values. This is especially beneficial when you have a test case that needs to be executed with multiple sets of input data. Utilizing dynamic tests and parameterized tests in JUnit 5 can make your unit tests more flexible and efficient while reducing code duplication.
The new extension model introduced in JUnit5 is another resource developers can utilize. This model allows developers to add more depth and versatility to their testing process by extending their tests' functionality.
Another crucial practice is maintaining concise and focused tests.
Learn how to maintain concise and focused tests for better test efficiency!
This involves ensuring that each test focuses on a single behavior or functionality, keeping tests isolated from each other, managing test data effectively, using clear and descriptive names for your tests, avoiding test duplication, and avoiding unnecessary dependencies in tests. These practices make tests easier to comprehend and manage, thereby enhancing their efficiency.
JUnit5, which was released a couple of years ago, is generally advised for transition from JUnit4. However, this shift does not require the complete rewriting of tests. The JUnit Vintage engine allows developers to execute their existing JUnit4 tests within the JUnit5 context.
One of the significant changes from JUnit4 to JUnit5 is the renaming of certain annotations. For instance, 'before' and 'after' annotations are now referred to as 'beforeEach' and 'afterEach', respectively. Similarly, 'beforeAll' and 'afterAll' have been renamed to 'beforeClass' and 'afterClass'. In JUnit4, the 'ignore' annotation has been substituted with the 'disabled' annotation in JUnit5. JUnit5 also provides annotations for conditional execution, such as 'enabledOnOs' and 'disabledOnJre'.
Developers who integrated their tests with other frameworks using the 'RunWith' annotation in JUnit4 can now use the 'ExtendWith' annotation in JUnit5. The JUnit 5 User Guide serves as a valuable resource for developers looking to get started with writing tests in JUnit5.
Understanding parameterized tests in JUnit5 is also important. This involves understanding the process of obtaining the necessary dependencies for writing parameterized tests, customizing the display name of each method invocation in a parameterized test, understanding the different argument sources that can be used in parameterized tests, such as valuesource, enumsource, csvsource, methodsource, and argumentsprovider.
Furthermore, creating test data using the csv format, loading test data from a csv file, understanding the limitations of the default argument converter, creating custom argument converters, creating test data using a factory method, and employing a custom arguments provider can all prove beneficial.
In essence, these best practices' implementation can significantly enhance the testing process's efficiency and effectiveness, leading to the development of more robust and reliable software solutions
Conclusion
In conclusion, JUnit4 has been a widely used and trusted testing framework in the Java community. Its core features, such as the use of annotations like @Test, @Before, and @After, have provided developers with a systematic approach to structuring and organizing their test cases. JUnit4 has seamlessly integrated into the standard Java development workflow, making it accessible to developers worldwide. However, JUnit4 does have its limitations when it comes to managing dynamic tests and extensibility. The introduction of JUnit5 offers new annotations and enhancements that address these limitations and provide developers with more flexibility and control over the testing lifecycle. By understanding the core features and limitations of JUnit4, developers can make informed decisions about transitioning to JUnit5 and explore new testing paradigms to enhance the quality of their code.
The broader significance of the ideas discussed in this article is that software testing is a crucial aspect of software development. Testing frameworks like JUnit4 and JUnit5 play a vital role in ensuring the reliability and quality of software applications. By utilizing these frameworks effectively, developers can improve their testing processes, identify bugs or issues early on, and deliver more robust software solutions. The transition from JUnit4 to JUnit5 presents an opportunity for developers to embrace new features and enhancements that enhance their testing capabilities. It is important for developers to stay updated with the latest developments in testing frameworks and continuously improve their testing practices to ensure the delivery of high-quality software products.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.