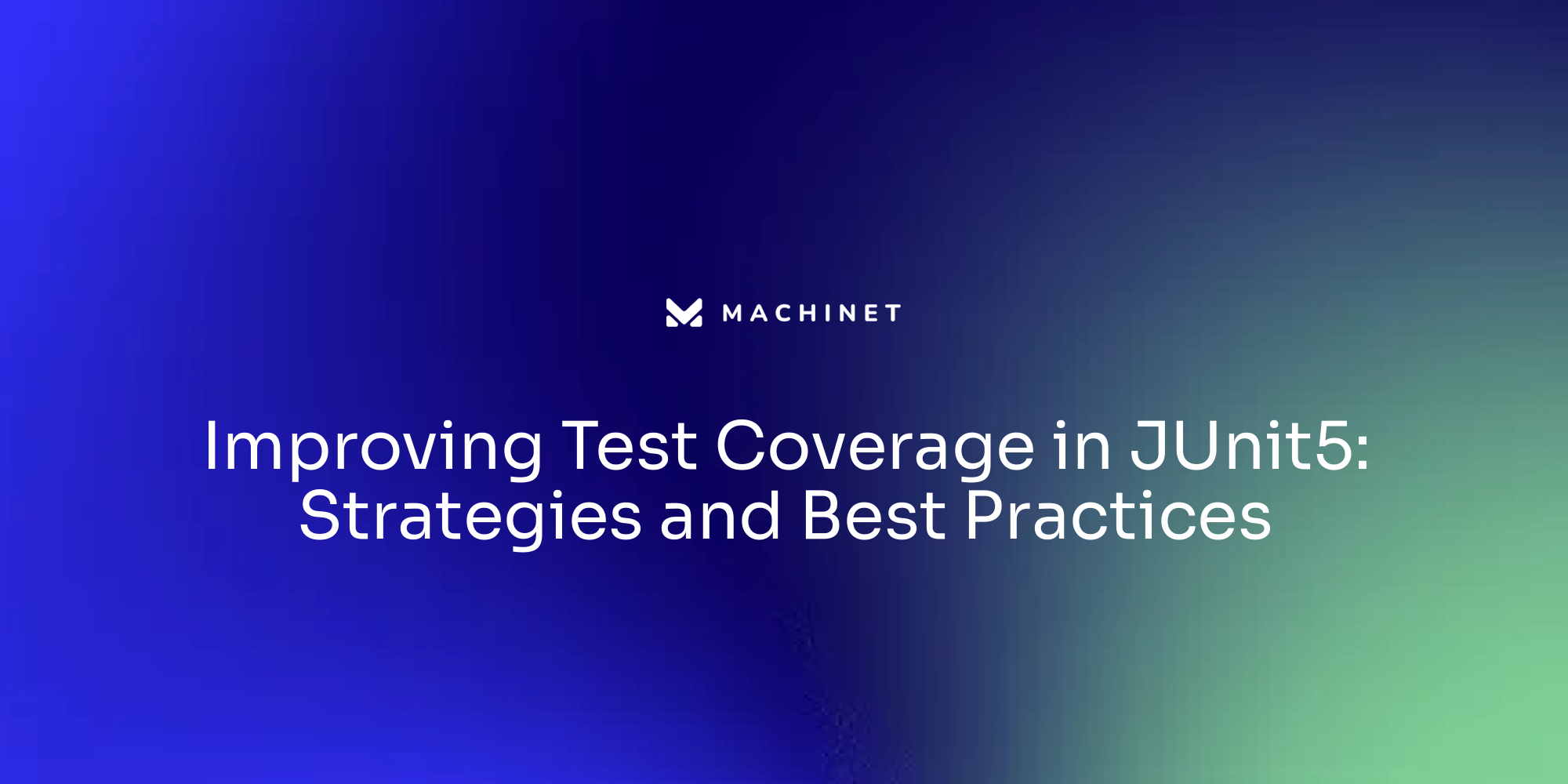
Table of contents:
- Understanding the Importance of Test Coverage in Software Development
- Identifying Challenges in Achieving Optimal Test Coverage with JUnit5
- Analyzing the Differences between JUnit4 and JUnit5 in Terms of Test Coverage
- Implementing Effective Strategies to Improve Test Coverage in JUnit5
- Utilizing Best Practices for Writing Robust and Comprehensive Unit Tests in JUnit5
- Leveraging Advanced Features of JUnit5 to Maximize Test Coverage
- Case Study: Successful Improvement of Test Coverage Using JUnit5
Introduction
Test coverage plays a pivotal role in software development, measuring the breadth of testing conducted and providing insights into the effectiveness of testing efforts. High test coverage indicates a well-tested software system, reducing the probability of unnoticed bugs and enhancing software quality. In this article, we will delve into the importance of test coverage in software development, the differences between code coverage and test coverage, and strategies to improve test coverage using JUnit5. We will also explore the advanced features of JUnit5 that can be leveraged to maximize test coverage and discuss a case study showcasing successful improvements in test coverage using JUnit5. By understanding the significance of test coverage and utilizing JUnit5 effectively, developers can enhance the reliability and quality of their software
1. Understanding the Importance of Test Coverage in Software Development
The pivotal role of test coverage in the software development landscape is undeniable. This metric acts as a barometer, measuring the breadth of testing conducted by a suite of tests. It encompasses a variety of aspects, from the percentage of invoked classes and methods to the number of executed code lines. High test coverage indicates a well-tested software system, reducing the probability of unnoticed bugs and thereby enhancing software quality. It provides a quantifiable method to gauge the success of testing efforts, which aids in efficiently distributing resources.
Code coverage and test coverage are two key concepts within the context of test coverage. Code coverage quantifies the portion of code executed during testing, expressed as a percentage. Its measurement can take various forms, such as statement coverage, branch coverage, condition coverage, and function coverage. For example, statement coverage evaluates the percentage of executed code statements, while branch coverage measures the percentage of executed code branches. Code coverage is a valuable tool in identifying unutilized or untested code, evaluating the quality of test suites, detecting potential bugs, and identifying dead code. Its benefits range from improved software reliability and shorter development cycles to easier debugging and superior code quality.
Conversely, test coverage quantifies the extent to which the tests encapsulate the software's requirements or functionality. It is imperative in ensuring all crucial business requirements are tested. Test coverage metrics encompass specifications coverage, product coverage, risk coverage, and functional coverage. It augments software quality, decreases the risk of new defects, identifies untested portions of the product, saves time and money, and fosters product confidence.
The distinction between code coverage and test coverage stems from their measurement approach, focus, testing methodology, and testing stage. Code coverage is a quantitative measurement focusing on code execution, employing a white box testing approach during unit tests. In contrast, test coverage is a qualitative measurement focusing on the testing process, employing a black box testing methodology during integration, system, and acceptance tests.
The choice between code coverage and test coverage is circumstantial, but both are integral parts of a well-rounded testing strategy. Code coverage is preferred during development to ensure all code lines are tested, while test coverage is more suitable for evaluating test effectiveness and overall code quality. Therefore, both code coverage and test coverage are critical metrics for determining software correctness and should be used based on the specific requirements of the software system.
Furthermore, studies have demonstrated the significant role of test coverage in ensuring software quality. A positive correlation exists between test coverage and software quality, as high test coverage aids in early defect detection and resolution. These studies also emphasize the need to consider different levels of coverage, including statement coverage, branch coverage, and path coverage, to achieve comprehensive testing and improve software quality.
Boosting test coverage in software can be achieved via various strategies. Prioritizing the writing of unit tests for critical and complex code sections ensures thorough testing and early detection of potential bugs. Utilizing code coverage tools can identify code areas not adequately covered by tests, allowing developers to concentrate their efforts on increasing test coverage in these areas. Incorporating automated testing into the development process, using continuous integration tools to automatically run tests after each code change, can catch any regressions or emerging issues.
Try Machinet's automated unit test generation to catch regressions and emerging issues
Techniques such as boundary value analysis and equivalence partitioning help identify test cases covering a wide range of input values, thereby increasing overall test coverage. Cultivating a culture of testing within the development team, with regular code reviews and peer testing, also contributes to improving test coverage in software.
Understanding test coverage metrics and their interpretation is crucial in the context in which these metrics are employed. They provide a quantitative measure of the extent to which a program's source code has been tested, helping assess testing thoroughness and identify inadequately tested code areas. Different test coverage metrics measure different code coverage aspects. For instance, line coverage measures the percentage of executed code lines during testing, while branch coverage measures the percentage of exercised decision points. Other commonly used metrics include statement coverage, condition coverage, and path coverage.
Interpreting test coverage metrics requires considering the specific goals and software requirements. A high test coverage percentage does not guarantee completely error-free code or that all possible scenarios have been tested. These metrics should be used as tools for identifying areas that may require further testing and prioritizing testing efforts accordingly. Test coverage metrics can provide valuable insights into the effectiveness of testing efforts and help improve software quality. However, they should be used in conjunction with other testing techniques and considerations, such as code reviews, static analysis, and manual testing, to ensure comprehensive test coverage and identify potential issues
2. Identifying Challenges in Achieving Optimal Test Coverage with JUnit5
Addressing the challenges of achieving high test coverage with JUnit5, particularly in complex codebases, requires a blend of strategic practices and the effective use of the framework's features. The complexity of the codebase can often make comprehensive testing an intimidating task, leading to gaps in test coverage. This complexity is further compounded by the dynamic nature of project requirements, necessitating continual updates or complete rewrites of test cases.
Balancing the necessity for thorough testing with the constraints of time and resources is a common challenge. However, this balance can be achieved through a combination of diligent practices and the efficient use of JUnit5.4, the latest version of this widely used Java testing library. JUnit5.4 offers an array of enhancements that simplify the testing process and boost the efficiency of test coverage.
To maintain high test coverage amidst evolving requirements, it is crucial to regularly review and update test cases to align with changes in the system's functionality. Prioritizing test cases based on their criticality and impact on the system is another important practice. This approach ensures that the most crucial functionalities are thoroughly tested, even when faced with changing requirements. Automating the testing process where possible can save time and effort, allowing for quick and efficient testing whenever there are changes in the requirements.
JUnit5.4 has significantly improved the process of initiation by including a single JUnit Jupiter dependency, which simplifies the setup. It also introduces support for temporary directories during testing, which assists in managing temporary files. Parameterized tests now support null and empty values as input, allowing for a more comprehensive and diverse range of testing scenarios.
The display name of test methods can now be dynamically generated based on the nested class or method name, which improves the organization and readability of tests. This version also allows developers to specify the order of test method execution using the @TestMethodOrder
annotation, providing more control over the test execution process.
While these improvements and features address the challenges of optimal test coverage, the nuances of balancing test coverage and resource constraints require careful planning, prioritization, and optimization of testing efforts. One approach is to focus on testing critical and high-risk areas first, ensuring these areas are thoroughly tested. Prioritizing tests based on the likelihood and impact of failure is another approach. Automated tests can be run repeatedly and quickly, allowing for faster feedback on the software's stability and functionality.
Regular communication and collaboration between the development and testing teams can help identify areas where test coverage can be optimized, and resources can be utilized more effectively. Despite the complexities involved, these practices, combined with the features of JUnit5.4, can make the process of achieving high test coverage more efficient and manageable. Upgrading from JUnit4 to JUnit5 is therefore highly recommended due to these new features and improvements
3. Analyzing the Differences between JUnit4 and JUnit5 in Terms of Test Coverage
As a latest iteration in the respected Java testing framework series, JUnit 5.4 comes packed with numerous enhancements that significantly elevate test coverage. Among the key introductions, dynamic testing stands out, as it enables test generation during runtime based on specific conditions. This feature proves to be a game changer in bolstering test coverage for complex scenarios.
JUnit 5.4 has also streamlined the process of including dependencies by allowing the use of a single junit-jupiter dependency. This has simplified the task for developers in managing their dependencies during test writing.
The framework introduces an extension for creating and cleaning temporary files during testing, contributing to the efficiency of tests that necessitate file operations. JUnit 5.4 also extends support for parameterized tests with null and empty input values, thereby offering a robust testing environment for edge cases. This feature can minimize boilerplate code and boost test coverage by allowing different data sets to be used in the same test method.
Another notable feature in JUnit 5.4 is the capability to generate the display name of tests dynamically, depending on the nested class or method name. This feature improves the readability of test reports and simplifies the process of tracing test failures.
JUnit 5.4 also presents a new test method order annotation for designating the execution order of test methods. This feature offers more control over the test execution flow, which can be especially beneficial in scenarios where the order of test execution is crucial.
With regard to integration, the modular architecture of JUnit 5 allows for enhanced integration with other tools and frameworks, thereby improving test coverage. The modular architecture distributes concerns into different modules, making it easier to use only the parts of JUnit that are needed without being overloaded by unnecessary dependencies.
The comprehensive documentation for JUnit 5.4, replete with illustrative code examples, simplifies the task for developers to understand and utilize these new features. The documentation also attests to the high quality of this new iteration of JUnit, making it a recommended upgrade for all Java developers.
The transition from JUnit 4 to JUnit 5 can be carried out incrementally, courtesy of the JUnit Vintage engine. This engine enables JUnit 4 tests to run within the JUnit 5 context, allowing developers to migrate their tests gradually.
In essence, JUnit 5.4 brings several enhancements over JUnit 4, including lambda support, test method parameter injection, and many other features that augment test coverage. These improvements make it an enticing choice for developers seeking to upgrade their testing framework
4. Implementing Effective Strategies to Improve Test Coverage in JUnit5
Enhancing test coverage in JUnit5 can be achieved by adopting a few strategic approaches and adhering to industry best practices. Ensuring comprehensive test coverage for all critical code paths is one such strategy, as it ensures the integral functionalities are thoroughly validated.
Identifying the untested areas of your codebase can be a challenging task. However, this task can be made easier by using code coverage tools or by manually reviewing your code. These tools provide invaluable insights into the sections of your code that may have been overlooked during testing.
Incorporating testing into the development process from the outset, rather than treating it as an afterthought, can lead to higher test coverage. This approach allows for a more streamlined and efficient process where tests and code evolve together, creating more robust coverage.
Keeping your tests in sync with the changes in your codebase is also crucial. Regularly reviewing and updating your tests ensures that they remain effective and relevant, even as the codebase evolves.
JUnit5, especially its 5.4 version, provides a host of features that can significantly aid in improving test coverage.
Boost your test coverage with JUnit5. Try Machinet now!
Lambda support, test method parameter injection, and the introduction of the @TempDir annotation for handling temporary directories during testing make it a more modern and efficient version.
JUnit 5.4 also provides support for parameterized tests with null and empty values, which can be essential in increasing test coverage. The @Order annotation is another new feature introduced in JUnit 5.4, allowing you to specify the order of test method execution for more control and predictability in your testing process.
The ability to customize test display names in JUnit 5.4 offers a smarter way to identify your tests, especially when you have a large number of them. This feature, coupled with the high-quality documentation and illustrative code examples provided, makes the upgrade to JUnit 5.4 a recommended move.
Incorporating parameterized tests in your testing strategy can be highly beneficial. Not only does it make your tests more concise and readable, but it also ensures that your code is tested against a wider range of inputs, thereby increasing your test coverage.
Increase your test coverage with parameterized tests. Try Machinet today!
JUnit5 provides several argument sources for parameterized tests, such as ValueSource, EnumSource, CSVSource, MethodSource, and custom ArgumentProviders, offering a high degree of flexibility.
In essence, improving test coverage in JUnit5 is a multi-faceted process that requires strategic planning, regular review, and the effective use of the robust features provided by the framework. With JUnit 5.4, you have a powerful tool at your disposal to help you achieve optimal test coverage
5. Utilizing Best Practices for Writing Robust and Comprehensive Unit Tests in JUnit5
In the journey of building robust and comprehensive unit tests using JUnit5, embracing certain best practices is pivotal. One of the key aspects is the independence of tests, ensuring they do not hinge on the state of other tests or any external variables. Consistency is another crucial element, as tests should yield identical results every time they are executed, demonstrating their repeatability and determinism.
JUnit5 offers several mechanisms to ensure repeatability and determinism in unit tests. Annotations like @BeforeEach
and @AfterEach
allow you to designate setup and teardown methods that are executed before and after each test method. By using these annotations, each test starts with a clean and consistent state, making the tests repeatable.
Another powerful feature of JUnit5 is the ability to control the test execution order. Using the @TestMethodOrder
annotation, you can specify the sequence in which test methods are executed. This ensures that the tests are executed in a deterministic manner, irrespective of the order in which they are defined in the test class.
Clarity in test naming provides easier comprehension of its purpose and functionality. This is particularly beneficial when troubleshooting a failed test. Beyond merely verifying the correctness of results, tests should also validate the correctness of the behavior. By using JUnit5's built-in assertion methods, you can make assertions about the expected behavior of your code and provide descriptive names for the assertions. For instance, the assertEquals
method allows you to compare expected and actual values and provide a descriptive name for the assertion. This aids in swiftly identifying which test has failed and what was the expected behavior.
JUnit5's parameterized tests offer a comprehensive way to write tests. It involves setting up the display name and providing method parameters using the valuesource annotation. Customizing the display name of each method invocation in a parameterized test can be advantageous. JUnit5 supports various argument sources, such as the valuesource annotation for primitive types and the enumsource annotation for enum values. You can pass multiple method parameters to a parameterized test using the csvsource annotation, or even load test data from a CSV file.
Advanced techniques include creating test data using a factory method and a custom arguments provider. A custom argument converter can be used to convert source objects into instances of another type. Examples and code snippets provide practical illustrations of these concepts.
The benefits of testing are numerous, and it starts with writing testable code. The basic process of testing includes preparing test inputs, triggering the code to be tested, and verifying the behavior and outcomes. Utilizing abstractions and dependency injection can make the code more testable and decoupled from external dependencies.
Creating fake implementations of interfaces for testing purposes, or test doubles, is a common practice. Running unit tests using JUnit5 involves setting up and running different test scenarios. Testing practices like 100% code coverage and test-driven development (TDD) can be controversial, but they offer differing perspectives that can be beneficial.
Testing should be a regular practice, and it is encouraged to experiment with different testing approaches. Writing effective unit tests using JUnit5 can lead to catching errors earlier and faster test runs. The use of regular expressions (regex) for testing can also be efficient and faster than writing validation logic in pure Java.
Mocking is another important concept in testing, allowing the creation of test doubles using mocking frameworks. Utilizing dependency injection can make the code more testable by passing dependencies to a class via a constructor method. Abstractions, such as interfaces, can be used to separate code from platform APIs and third-party libraries, making the code more testable.
In the end, the importance of regularly writing tests cannot be overstated, and various testing approaches should be explored to find the ones that best suit your needs
6. Leveraging Advanced Features of JUnit5 to Maximize Test Coverage
JUnit5, in its current version, 5.4, introduces several enhanced features that significantly improve test coverage. Among these features is the ability to generate dynamic tests at runtime based on specific conditions, which is beneficial when dealing with complex software applications requiring robust testing strategies.
Dynamic tests in JUnit 5 are implemented using the @TestFactory
annotation. You need to create a method annotated with @TestFactory
that returns a Stream
or Iterable
of DynamicTest
objects. Each DynamicTest
represents a single test case. The method can contain any logic to generate the dynamic tests, such as iterating over a collection of data, reading from a file, or making API calls to get the test data. For each test case, you create a DynamicTest
object using the DynamicTest.dynamicTest()
method. Once you have generated all the dynamic tests, you return them as a stream or iterable from the @TestFactory
method. JUnit 5 will then execute each dynamic test and report the results.
```javaimport org.junit.jupiter.api.DynamicTest;import org.junit.jupiter.api.TestFactory;import org.junit.jupiter.api.function.Executable;
import java.util.stream.Stream;
import static org.junit.jupiter.api.Assertions.assertEquals;import static org.junit.jupiter.api.Assertions.assertTrue;
public class DynamicTestsExample {
@TestFactory
public Stream<DynamicTest> dynamicTests() {
return Stream.of(
DynamicTest.dynamicTest("Test 1", () -> assertTrue(true)),
DynamicTest.dynamicTest("Test 2", () -> assertEquals(4, 2 + 2)),
DynamicTest.dynamicTest("Test 3", () -> {
// Custom assertion logic
int result = 5 * 5;
assertTrue(result > 0);
})
);
}
}```
JUnit Jupiter dependency in JUnit 5.4 simplifies dependency inclusion, which is a testament to JUnit5's commitment to enhancing developers' experience and productivity. The @TempDir
annotation introduces temporary directories for testing, further simplifying the creation and cleanup of temporary files during testing.
Parameterized tests in JUnit 5.4 now support null and empty values as input, reducing boilerplate code and improving test coverage. The @DisplayName
annotation generates dynamic display names based on nested classes or method names, enhancing the readability and organization of tests.
JUnit 5.4 introduces the @TestMethodOrder
annotation, allowing developers to specify the order of test method execution. This feature, along with support for nested tests, provides better test organization and improved test coverage. Moreover, JUnit5's modular architecture ensures seamless integration with other tools and frameworks, further enhancing test coverage.
JUnit 5.4 also offers experimental support for parallel test execution, utilizing multiple CPU cores to shorten the software development feedback loop. The ParallelizableTest
annotation controls the parallel execution of test classes or test methods. This approach not only prevents excessive use of built-in annotations but also ensures "separate instance per method" semantics.
The test pyramid can guide the selection of tests for parallelization, with unit tests being the easiest to parallelize. Parallelizing other test classes may require more effort, especially for tests with shared resources or complex synchronization requirements. However, the proposed approach allows for incremental parallelization of tests over time.
Consequently, with the introduction of these advanced features, JUnit5 has further solidified its position as a robust and comprehensive unit testing framework in the Java ecosystem. Upgrading to JUnit 5 is highly recommended due to the multitude of new features and improvements it offers
7. Case Study: Successful Improvement of Test Coverage Using JUnit5
The challenge of low test coverage in software development, compounded by a complex codebase and the constant evolution of requirements, is a familiar scenario for many development teams, including the one at Machinet. To tackle this issue head-on, the team decided to transition from JUnit4 to JUnit5. This latest iteration of the JUnit testing framework, designed for Java 8 and beyond, was birthed from the JUnit Lambda project and was made possible through an Indiegogo crowdfunding campaign.
JUnit5 brought with it a plethora of advanced features that the Machinet team was keen to leverage. Its focus on facilitating various styles of testing styles offered a modern foundation for developer-side testing. To bolster their test coverage, the team adopted several effective strategies and best practices, including writing unit tests for critical and complex code sections, using JUnit5's parameterized tests feature, leveraging JUnit5's support for dynamic tests, and regularly reviewing and updating test suites.
The team found the dynamic tests, parameterized tests, and nested tests features of JUnit5 especially useful. These features enabled them to write more comprehensive tests, significantly reducing the risk of undetected bugs and thus improving the overall quality of their software.
Additionally, the team used JaCoCo, a popular code coverage tool for Java, alongside JUnit5. This tool measures the effectiveness of tests by identifying areas of code that aren't addressed during testing. By integrating JaCoCo with JUnit5, the team could generate detailed reports showing the percentage of code covered by tests, helping to identify potential gaps in the test suite and improve the overall quality of the code.
The Machinet team's transition to JUnit5 played a crucial role in helping them deliver their projects on time. Indeed, it underscored the potential of JUnit5 in real-world scenarios, where complex codebases and ever-changing requirements are par for the course. By exploiting the advanced features of JUnit5, the team didn't just improve their test coverage; they also enhanced the reliability of their software and kept their project timelines on track
Conclusion
In conclusion, test coverage plays a vital role in software development by measuring the breadth of testing conducted and providing insights into the effectiveness of testing efforts. High test coverage indicates a well-tested software system, reducing the probability of unnoticed bugs and enhancing software quality. Code coverage and test coverage are two key concepts within the context of test coverage, with code coverage focusing on code execution during testing and test coverage ensuring that all crucial business requirements are tested. By understanding the significance of test coverage and utilizing JUnit5 effectively, developers can enhance the reliability and quality of their software.
The advanced features introduced in JUnit5, such as dynamic tests, streamlined dependency inclusion, support for parameterized tests with null and empty values, dynamic display names for tests, and more control over test method execution order, contribute to maximizing test coverage. These features enable developers to write more comprehensive tests, simplify the testing process, and improve the readability and organization of tests. By adopting effective strategies like prioritizing critical code sections for testing, regularly reviewing and updating tests, leveraging automation tools, and fostering a culture of testing within development teams, developers can further enhance their test coverage. Boost your productivity with Machinet. Experience the power of AI-assisted coding and automated unit test generation.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.